KDEUI
#include <kdatetable.h>
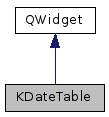
Public Types | |
enum | BackgroundMode { NoBgMode = 0, RectangleMode, CircleMode } |
Signals | |
void | aboutToShowContextMenu (KMenu *menu, const QDate &date) |
void | dateChanged (const QDate &date) |
void | dateChanged (const QDate &cur, const QDate &old) |
void | tableClicked () |
Public Member Functions | |
KDateTable (QWidget *parent=0) | |
KDateTable (const QDate &, QWidget *parent=0) | |
~KDateTable () | |
const KCalendarSystem * | calendar () const |
const QDate & | date () const |
bool | popupMenuEnabled () const |
bool | setCalendar (KCalendarSystem *calendar=0) |
bool | setCalendar (const QString &calendarType) |
bool | setCalendarSystem (KLocale::CalendarSystem calendarSystem) |
void | setCustomDatePainting (const QDate &date, const QColor &fgColor, BackgroundMode bgMode=NoBgMode, const QColor &bgColor=QColor()) |
bool | setDate (const QDate &date) |
void | setFontSize (int size) |
void | setPopupMenuEnabled (bool enable) |
virtual QSize | sizeHint () const |
void | unsetCustomDatePainting (const QDate &date) |
Protected Member Functions | |
virtual QDate | dateFromPos (int pos) |
virtual bool | event (QEvent *e) |
virtual void | focusInEvent (QFocusEvent *e) |
virtual void | focusOutEvent (QFocusEvent *e) |
virtual void | keyPressEvent (QKeyEvent *e) |
virtual void | mousePressEvent (QMouseEvent *e) |
virtual void | paintEvent (QPaintEvent *e) |
virtual int | posFromDate (const QDate &date) |
virtual void | wheelEvent (QWheelEvent *e) |
Properties | |
QDate | date |
bool | popupMenu |
Detailed Description
Date selection table.
This is a support class for the KDatePicker class. It just draws the calendar table without titles, but could theoretically be used as a standalone.
When a date is selected by the user, it emits a signal: dateSelected(QDate)
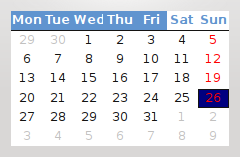
Definition at line 139 of file kdatetable.h.
Member Enumeration Documentation
Enumerator | |
---|---|
NoBgMode | |
RectangleMode | |
CircleMode |
Definition at line 235 of file kdatetable.h.
Constructor & Destructor Documentation
|
explicit |
The constructor.
Definition at line 253 of file kdatetable.cpp.
|
explicit |
The constructor.
Definition at line 246 of file kdatetable.cpp.
KDateTable::~KDateTable | ( | ) |
The destructor.
Definition at line 260 of file kdatetable.cpp.
Member Function Documentation
|
signal |
A popup menu for a given date is about to be shown (as when the user right clicks on that date and the popup menu is enabled).
Connect the slot where you fill the menu to this signal.
const KCalendarSystem * KDateTable::calendar | ( | ) | const |
Returns the currently selected calendar system.
- Returns
- a KCalendarSystem object
Definition at line 788 of file kdatetable.cpp.
const QDate& KDateTable::date | ( | ) | const |
- Returns
- the selected date.
|
signal |
The selected date changed.
|
signal |
This function behaves essentially like the one above.
The selected date changed.
- Parameters
-
cur The current date old The date before the date was changed
|
protectedvirtual |
calculate the date that is displayed at a given cell in the matrix.
pos is the 0-based index in the matrix. Inverse function to posForDate().
Definition at line 328 of file kdatetable.cpp.
|
protectedvirtual |
Cell highlight on mouse hovering.
Definition at line 665 of file kdatetable.cpp.
|
protectedvirtual |
Definition at line 811 of file kdatetable.cpp.
|
protectedvirtual |
Definition at line 816 of file kdatetable.cpp.
|
protectedvirtual |
Definition at line 591 of file kdatetable.cpp.
|
protectedvirtual |
React on mouse clicks that select a date.
Definition at line 699 of file kdatetable.cpp.
|
protectedvirtual |
Definition at line 341 of file kdatetable.cpp.
bool KDateTable::popupMenuEnabled | ( | ) | const |
Returns if the popup menu is enabled or not.
Definition at line 837 of file kdatetable.cpp.
|
protectedvirtual |
calculate the position of the cell in the matrix for the given date.
The result is the 0-based index.
Definition at line 314 of file kdatetable.cpp.
bool KDateTable::setCalendar | ( | KCalendarSystem * | calendar = 0 | ) |
Changes the calendar system to use.
Can use its own local locale if set.
- Parameters
-
calendar the calendar system object to use, defaults to global
- Returns
true
if the calendar system was successfully set,false
otherwise
Definition at line 793 of file kdatetable.cpp.
Changes the calendar system to use.
Will always use global locale.
- Parameters
-
calendarType the calendar system type to use
- Returns
true
if the calendar system was successfully set,false
otherwise
Definition at line 800 of file kdatetable.cpp.
bool KDateTable::setCalendarSystem | ( | KLocale::CalendarSystem | calendarSystem | ) |
- Since
- 4.6
Changes the calendar system to use. Will always use global locale.
- Parameters
-
calendarSystem the calendar system to use
- Returns
true
if the calendar system was successfully set,false
otherwise
Definition at line 805 of file kdatetable.cpp.
void KDateTable::setCustomDatePainting | ( | const QDate & | date, |
const QColor & | fgColor, | ||
BackgroundMode | bgMode = NoBgMode , |
||
const QColor & | bgColor = QColor() |
||
) |
Makes a given date be painted with a given foregroundColor, and background (a rectangle, or a circle/ellipse) in a given color.
Definition at line 842 of file kdatetable.cpp.
bool KDateTable::setDate | ( | const QDate & | date | ) |
Select and display this date.
Definition at line 764 of file kdatetable.cpp.
void KDateTable::setFontSize | ( | int | size | ) |
Set the font size of the date table.
Definition at line 639 of file kdatetable.cpp.
void KDateTable::setPopupMenuEnabled | ( | bool | enable | ) |
Enables a popup menu when right clicking on a date.
When it's enabled, this object emits a aboutToShowContextMenu signal where you can fill in the menu items.
Definition at line 832 of file kdatetable.cpp.
|
virtual |
Returns a recommended size for the widget.
To save some time, the size of the largest used cell content is calculated in each paintCell() call, since all calculations have to be done there anyway. The size is stored in maxCell. The sizeHint() simply returns a multiple of maxCell.
Definition at line 821 of file kdatetable.cpp.
|
signal |
A date has been selected by clicking on the table.
void KDateTable::unsetCustomDatePainting | ( | const QDate & | date | ) |
Unsets the custom painting of a date so that the date is painted as usual.
Definition at line 859 of file kdatetable.cpp.
|
protectedvirtual |
Definition at line 659 of file kdatetable.cpp.
Property Documentation
|
readwrite |
Definition at line 142 of file kdatetable.h.
|
readwrite |
Definition at line 144 of file kdatetable.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.