KDEUI
#include <keditlistwidget.h>
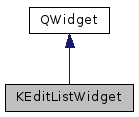
Classes | |
class | CustomEditor |
Public Types | |
enum | Button { Add = 0x0001, Remove = 0x0002, UpDown = 0x0004, All = Add | Remove | UpDown } |
Signals | |
void | added (const QString &text) |
void | changed () |
void | removed (const QString &text) |
Public Member Functions | |
KEditListWidget (QWidget *parent=0) | |
KEditListWidget (const CustomEditor &customEditor, QWidget *parent=0, bool checkAtEntering=false, Buttons buttons=All) | |
virtual | ~KEditListWidget () |
QPushButton * | addButton () const |
Buttons | buttons () const |
bool | checkAtEntering () |
void | clear () |
int | count () const |
int | currentItem () const |
QString | currentText () const |
QPushButton * | downButton () const |
bool | eventFilter (QObject *o, QEvent *e) |
void | insertItem (const QString &text, int index=-1) |
void | insertStringList (const QStringList &list, int index=-1) |
QStringList | items () const |
KLineEdit * | lineEdit () const |
QListView * | listView () const |
QPushButton * | removeButton () const |
void | setButtons (Buttons buttons) |
void | setCheckAtEntering (bool check) |
void | setCustomEditor (const CustomEditor &editor) |
void | setItems (const QStringList &items) |
QString | text (int index) const |
QPushButton * | upButton () const |
Properties | |
Buttons | buttons |
bool | checkAtEntering |
QStringList | items |
Detailed Description
An editable listbox.
This class provides an editable listbox, this means a listbox which is accompanied by a line edit to enter new items into the listbox and pushbuttons to add and remove items from the listbox and two buttons to move items up and down.
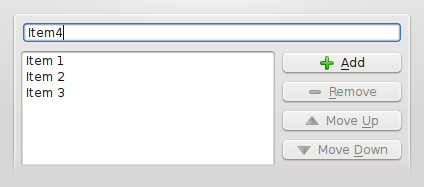
- Since
- 4.6
Definition at line 48 of file keditlistwidget.h.
Member Enumeration Documentation
Enumeration of the buttons, the listbox offers.
Specify them in the constructor in the buttons parameter, or in setButtons.
Enumerator | |
---|---|
Add | |
Remove | |
UpDown | |
All |
Definition at line 90 of file keditlistwidget.h.
Constructor & Destructor Documentation
|
explicit |
Create an editable listbox.
Definition at line 238 of file keditlistwidget.cpp.
KEditListWidget::KEditListWidget | ( | const CustomEditor & | customEditor, |
QWidget * | parent = 0 , |
||
bool | checkAtEntering = false , |
||
Buttons | buttons = All |
||
) |
Constructor which allows to use a custom editing widget instead of the standard KLineEdit widget.
E.g. you can use a KUrlRequester or a KComboBox as input widget. The custom editor must consist of a lineedit and optionally another widget that is used as representation. A KComboBox or a KUrlRequester have a KLineEdit as child-widget for example, so the KComboBox is used as the representation widget.
- See also
- KUrlRequester::customEditor(), setCustomEditor
Definition at line 244 of file keditlistwidget.cpp.
|
virtual |
Definition at line 254 of file keditlistwidget.cpp.
Member Function Documentation
QPushButton * KEditListWidget::addButton | ( | ) | const |
Return a pointer to the Add button.
Definition at line 274 of file keditlistwidget.cpp.
|
signal |
This signal is emitted when the user adds a new string to the list, the parameter is the added string.
Buttons KEditListWidget::buttons | ( | ) | const |
Returns which buttons are visible.
|
signal |
bool KEditListWidget::checkAtEntering | ( | ) |
Returns true if check at entering is enabled.
void KEditListWidget::clear | ( | ) |
Clears both the listbox and the line edit.
Definition at line 581 of file keditlistwidget.cpp.
int KEditListWidget::count | ( | ) | const |
See Q3ListBox::count()
Definition at line 294 of file keditlistwidget.cpp.
int KEditListWidget::currentItem | ( | ) | const |
See Q3ListBox::currentItem()
Definition at line 512 of file keditlistwidget.cpp.
QString KEditListWidget::currentText | ( | ) | const |
See Q3ListBox::currentText()
Definition at line 619 of file keditlistwidget.cpp.
QPushButton * KEditListWidget::downButton | ( | ) | const |
Return a pointer to the Down button.
Definition at line 289 of file keditlistwidget.cpp.
Reimplented for interal reasons.
The API is not affected.
Definition at line 653 of file keditlistwidget.cpp.
void KEditListWidget::insertItem | ( | const QString & | text, |
int | index = -1 |
||
) |
See Q3ListBox::insertItem()
Definition at line 600 of file keditlistwidget.cpp.
void KEditListWidget::insertStringList | ( | const QStringList & | list, |
int | index = -1 |
||
) |
See Q3ListBox::insertStringList()
Definition at line 588 of file keditlistwidget.cpp.
QStringList KEditListWidget::items | ( | ) | const |
- Returns
- a stringlist of all items in the listbox
KLineEdit * KEditListWidget::lineEdit | ( | ) | const |
Return a pointer to the embedded KLineEdit.
Definition at line 269 of file keditlistwidget.cpp.
QListView * KEditListWidget::listView | ( | ) | const |
Return a pointer to the embedded QListView.
Definition at line 264 of file keditlistwidget.cpp.
QPushButton * KEditListWidget::removeButton | ( | ) | const |
Return a pointer to the Remove button.
Definition at line 279 of file keditlistwidget.cpp.
|
signal |
This signal is emitted when the user removes a string from the list, the parameter is the removed string.
void KEditListWidget::setButtons | ( | Buttons | buttons | ) |
Specifies which buttons should be visible.
Definition at line 299 of file keditlistwidget.cpp.
void KEditListWidget::setCheckAtEntering | ( | bool | check | ) |
If check
is true, after every character you type in the line edit KEditListWidget will enable or disable the Add-button, depending whether the current content of the line edit is already in the listbox.
Maybe this can become a performance hit with large lists on slow machines. If check
is false, it will be checked if you press the Add-button. It is not possible to enter items twice into the listbox. Default is false.
Definition at line 349 of file keditlistwidget.cpp.
void KEditListWidget::setCustomEditor | ( | const CustomEditor & | editor | ) |
Allows to use a custom editing widget instead of the standard KLineEdit widget.
E.g. you can use a KUrlRequester or a KComboBox as input widget. The custom editor must consist of a lineedit and optionally another widget that is used as representation. A KComboBox or a KUrlRequester have a KLineEdit as child-widget for example, so the KComboBox is used as the representation widget.
Definition at line 259 of file keditlistwidget.cpp.
void KEditListWidget::setItems | ( | const QStringList & | items | ) |
Clears the listbox and sets the contents to items
.
Definition at line 633 of file keditlistwidget.cpp.
QString KEditListWidget::text | ( | int | index | ) | const |
See Q3ListBox::text()
Definition at line 612 of file keditlistwidget.cpp.
QPushButton * KEditListWidget::upButton | ( | ) | const |
Return a pointer to the Up button.
Definition at line 284 of file keditlistwidget.cpp.
Property Documentation
|
readwrite |
Definition at line 53 of file keditlistwidget.h.
|
readwrite |
Definition at line 55 of file keditlistwidget.h.
|
readwrite |
Definition at line 54 of file keditlistwidget.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.