KDEUI
KFontAction Class Reference
#include <kfontaction.h>
Inheritance diagram for KFontAction:
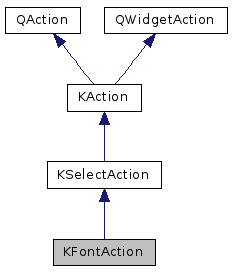
Public Member Functions | |
KFontAction (uint fontListCriteria, QObject *parent) | |
KFontAction (QObject *parent) | |
KFontAction (const QString &text, QObject *parent) | |
KFontAction (const KIcon &icon, const QString &text, QObject *parent) | |
virtual | ~KFontAction () |
virtual QWidget * | createWidget (QWidget *parent) |
QString | font () const |
void | setFont (const QString &family) |
![]() | |
KSelectAction (QObject *parent) | |
KSelectAction (const QString &text, QObject *parent) | |
KSelectAction (const KIcon &icon, const QString &text, QObject *parent) | |
virtual | ~KSelectAction () |
QAction * | action (int index) const |
QAction * | action (const QString &text, Qt::CaseSensitivity cs=Qt::CaseSensitive) const |
QList< QAction * > | actions () const |
virtual void | addAction (QAction *action) |
KAction * | addAction (const QString &text) |
KAction * | addAction (const KIcon &icon, const QString &text) |
void | changeItem (int index, const QString &text) |
void | clear () |
int | comboWidth () const |
QAction * | currentAction () const |
int | currentItem () const |
QString | currentText () const |
bool | isEditable () const |
QStringList | items () const |
bool | menuAccelsEnabled () const |
virtual QAction * | removeAction (QAction *action) |
void | removeAllActions () |
QActionGroup * | selectableActionGroup () const |
void | setComboWidth (int width) |
bool | setCurrentAction (QAction *action) |
bool | setCurrentAction (const QString &text, Qt::CaseSensitivity cs=Qt::CaseSensitive) |
bool | setCurrentItem (int index) |
void | setEditable (bool) |
void | setItems (const QStringList &lst) |
void | setMaxComboViewCount (int n) |
void | setMenuAccelsEnabled (bool b) |
void | setToolBarMode (ToolBarMode mode) |
void | setToolButtonPopupMode (QToolButton::ToolButtonPopupMode mode) |
ToolBarMode | toolBarMode () const |
QToolButton::ToolButtonPopupMode | toolButtonPopupMode () const |
![]() | |
KAction (QObject *parent) | |
KAction (const QString &text, QObject *parent) | |
KAction (const KIcon &icon, const QString &text, QObject *parent) | |
virtual | ~KAction () |
KAuth::Action * | authAction () const |
bool | event (QEvent *) |
void | forgetGlobalShortcut () |
const KShortcut & | globalShortcut (ShortcutTypes type=ActiveShortcut) const |
bool | globalShortcutAllowed () const |
bool | isGlobalShortcutEnabled () const |
bool | isShortcutConfigurable () const |
KRockerGesture | rockerGesture (ShortcutTypes type=ActiveShortcut) const |
void | setAuthAction (KAuth::Action *action) |
void | setAuthAction (const QString &actionName) |
void | setGlobalShortcut (const KShortcut &shortcut, ShortcutTypes type=ShortcutTypes(ActiveShortcut|DefaultShortcut), GlobalShortcutLoading loading=Autoloading) |
void | setGlobalShortcutAllowed (bool allowed, GlobalShortcutLoading loading=Autoloading) |
void | setHelpText (const QString &text) |
void | setRockerGesture (const KRockerGesture &gest, ShortcutTypes type=ShortcutTypes(ActiveShortcut|DefaultShortcut)) |
void | setShapeGesture (const KShapeGesture &gest, ShortcutTypes type=ShortcutTypes(ActiveShortcut|DefaultShortcut)) |
void | setShortcut (const KShortcut &shortcut, ShortcutTypes type=ShortcutTypes(ActiveShortcut|DefaultShortcut)) |
void | setShortcut (const QKeySequence &shortcut, ShortcutTypes type=ShortcutTypes(ActiveShortcut|DefaultShortcut)) |
void | setShortcutConfigurable (bool configurable) |
void | setShortcuts (const QList< QKeySequence > &shortcuts, ShortcutTypes type=ShortcutTypes(ActiveShortcut|DefaultShortcut)) |
KShapeGesture | shapeGesture (ShortcutTypes type=ActiveShortcut) const |
KShortcut | shortcut (ShortcutTypes types=ActiveShortcut) const |
Properties | |
QString | font |
![]() | |
int | comboWidth |
QAction | currentAction |
int | currentItem |
QString | currentText |
bool | editable |
QStringList | items |
ToolBarMode | toolBarMode |
QToolButton::ToolButtonPopupMode | toolButtonPopupMode |
![]() | |
KShortcut | globalShortcut |
bool | globalShortcutAllowed |
bool | globalShortcutEnabled |
KShortcut | shortcut |
bool | shortcutConfigurable |
Additional Inherited Members | |
![]() | |
enum | ToolBarMode { MenuMode, ComboBoxMode } |
![]() | |
enum | GlobalShortcutLoading { Autoloading = 0x0, NoAutoloading = 0x4 } |
enum | ShortcutType { ActiveShortcut = 0x1, DefaultShortcut = 0x2 } |
![]() | |
void | triggered (QAction *action) |
void | triggered (int index) |
void | triggered (const QString &text) |
![]() | |
void | authorized (KAuth::Action *action) |
void | globalShortcutChanged (const QKeySequence &) |
void | triggered (Qt::MouseButtons buttons, Qt::KeyboardModifiers modifiers) |
![]() | |
virtual void | actionTriggered (QAction *action) |
void | slotToggled (bool) |
![]() | |
KSelectAction (KSelectActionPrivate &dd, QObject *parent) | |
virtual void | deleteWidget (QWidget *widget) |
virtual bool | event (QEvent *event) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
![]() | |
KSelectActionPrivate * | d_ptr |
Detailed Description
An action to select a font family.
On a toolbar this will show a combobox with all the fonts on the system.
Definition at line 36 of file kfontaction.h.
Constructor & Destructor Documentation
KFontAction::KFontAction | ( | uint | fontListCriteria, |
QObject * | parent | ||
) |
Definition at line 67 of file kfontaction.cpp.
|
explicit |
Definition at line 76 of file kfontaction.cpp.
Definition at line 85 of file kfontaction.cpp.
Definition at line 94 of file kfontaction.cpp.
|
virtual |
Definition at line 103 of file kfontaction.cpp.
Member Function Documentation
Reimplemented from.
- See also
- QWidgetAction.
Reimplemented from KSelectAction.
Definition at line 113 of file kfontaction.cpp.
QString KFontAction::font | ( | ) | const |
void KFontAction::setFont | ( | const QString & | family | ) |
Definition at line 138 of file kfontaction.cpp.
Property Documentation
|
readwrite |
Definition at line 39 of file kfontaction.h.
The documentation for this class was generated from the following files:
This file is part of the KDE documentation.
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.