KDEUI
#include <kstatusnotifieritem.h>
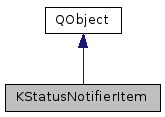
Public Types | |
enum | ItemCategory { ApplicationStatus = 1, Communications = 2, SystemServices = 3, Hardware = 4, Reserved = 129 } |
enum | ItemStatus { Passive = 1, Active = 2, NeedsAttention = 3 } |
Public Slots | |
virtual void | activate (const QPoint &pos=QPoint()) |
Signals | |
void | activateRequested (bool active, const QPoint &pos) |
void | scrollRequested (int delta, Qt::Orientation orientation) |
void | secondaryActivateRequested (const QPoint &pos) |
Protected Member Functions | |
bool | eventFilter (QObject *watched, QEvent *event) |
Detailed Description
KDE Status notifier Item protocol implementation
This class implements the Status notifier Item Dbus specification. It provides an icon similar to the classical systemtray icons, with some key differences:
- the actual representation is done by the systemtray (or the app behaving like it) itself, not by this app. Since 4.5 this also includes the menu, which means you cannot use embed widgets in the menu.
- there is communication between the systemtray and the icon owner, so the system tray can know if the application is in a normal or in a requesting attention state.
- icons are divided in categories, so the systemtray can represent in a different way the icons from normal applications and for instance the ones about hardware status.
Whenever possible you should prefer passing icon by name rather than by pixmap because:
- it is much lighter on Dbus (no need to pass all image pixels).
- it makes it possible for the systemtray to load an icon of the appropriate size or to replace your icon with a systemtray specific icon which matches with the desktop theme.
- some implementations of the system tray do not support passing icons by pixmap and will show a blank icon instead.
- Since
- 4.4
Definition at line 72 of file kstatusnotifieritem.h.
Member Enumeration Documentation
Different kinds of applications announce their type to the systemtray, so can be drawn in a different way or in a different place.
Definition at line 110 of file kstatusnotifieritem.h.
All the possible status this icon can have, depending on the importance of the events that happens in the parent application.
Definition at line 95 of file kstatusnotifieritem.h.
Constructor & Destructor Documentation
|
explicit |
Construct a new status notifier item.
- Parameters
-
parent the parent object for this object. If the object passed in as a parent is also a QWidget, it will be used as the main application window represented by this icon and will be shown/hidden when an activation is requested.
- See also
- associatedWidget
Definition at line 101 of file kstatusnotifieritem.cpp.
Construct a new status notifier item with a unique identifier.
If your application has more than one status notifier item and the user should be able to manipulate them separately (e.g. mark them for hiding in a user interface), the id can be used to differentiate between them.
The id should remain consistent even between application restarts. Status notifier items without ids default to the application's name for the id. This id may be used, for instance, by hosts displaying status notifier items to associate configuration information with this item in a way that can persist between sessions or application restarts.
- Parameters
-
id the unique id for this icon parent the parent object for this object. If the object passed in as a parent is also a QWidget, it will be used as the main application window represented by this icon and will be shown/hidden when an activation is requested.
- See also
- associatedWidget
Definition at line 109 of file kstatusnotifieritem.cpp.
KStatusNotifierItem::~KStatusNotifierItem | ( | ) |
Definition at line 116 of file kstatusnotifieritem.cpp.
Member Function Documentation
KActionCollection * KStatusNotifierItem::actionCollection | ( | ) | const |
All the actions present in the menu.
Definition at line 517 of file kstatusnotifieritem.cpp.
Shows the main widget and try to position it on top of the other windows, if the widget is already visible, hide it.
- Parameters
-
pos if it's a valid position it represents the mouse coordinates when the event was triggered
Definition at line 569 of file kstatusnotifieritem.cpp.
Inform the host application that an activation has been requested, for instance left mouse click, but this is not guaranteed since it's dependent from the visualization.
- Parameters
-
active if it's true the application asked for the activatin of the main window, if it's false it asked for hiding pos the position in the screen where the user clicked to trigger this signal, QPoint() if it's not the consequence of a mouse click.
QWidget * KStatusNotifierItem::associatedWidget | ( | ) | const |
Access the main widget associated with this StatusNotifierItem.
Definition at line 512 of file kstatusnotifieritem.cpp.
QString KStatusNotifierItem::attentionIconName | ( | ) | const |
- Returns
- the name of the icon to be displayed when the application is requesting the user's attention if attentionImage() is not empty this will always return an empty string
QIcon KStatusNotifierItem::attentionIconPixmap | ( | ) | const |
- Returns
- a pixmap of the requesting attention icon
Definition at line 294 of file kstatusnotifieritem.cpp.
QString KStatusNotifierItem::attentionMovieName | ( | ) | const |
- Returns
- the name of the movie to be displayed when the application is requesting the user attention
Definition at line 318 of file kstatusnotifieritem.cpp.
ItemCategory KStatusNotifierItem::category | ( | ) | const |
- Returns
- the application category
KMenu * KStatusNotifierItem::contextMenu | ( | ) | const |
Access the context menu associated to this status notifier item.
Definition at line 466 of file kstatusnotifieritem.cpp.
Definition at line 691 of file kstatusnotifieritem.cpp.
QString KStatusNotifierItem::iconName | ( | ) | const |
- Returns
- the name of the main icon to be displayed if image() is not empty this will always return an empty string
QIcon KStatusNotifierItem::iconPixmap | ( | ) | const |
- Returns
- a pixmap of the icon
Definition at line 207 of file kstatusnotifieritem.cpp.
QString KStatusNotifierItem::id | ( | ) | const |
- Returns
- The id which was specified in the constructor. This should be guaranteed to be consistent between application starts and untranslated, as host applications displaying items may use it for storing configuration related to this item.
Definition at line 128 of file kstatusnotifieritem.cpp.
QString KStatusNotifierItem::overlayIconName | ( | ) | const |
- Returns
- the name of the icon to be used as overlay fr the main one
QIcon KStatusNotifierItem::overlayIconPixmap | ( | ) | const |
- Returns
- a pixmap of the icon
Definition at line 259 of file kstatusnotifieritem.cpp.
|
signal |
Inform the host application that the mouse wheel (or another mean of scrolling that the visualization provides) has been used.
- Parameters
-
delta the amount of scrolling, can be either positive or negative orientation direction of the scrolling, can be either horizontal or vertical
|
signal |
Alternate activate action, for instance right mouse click, but this is not guaranteed since it's dependent from the visualization.
- Parameters
-
pos the position in the screen where the user clicked to trigger this signal, QPoint() if it's not the consequence of a mouse click.
void KStatusNotifierItem::setAssociatedWidget | ( | QWidget * | parent | ) |
Sets the main widget associated with this StatusNotifierItem.
If you pass contextMenu() as a parent then the menu will be displayed when the user activate the icon. In this case the activate() method will not be called and the activateRequested() signal will not be emitted
- Parameters
-
parent the new main widget: must be a top level window, if it's not parent->window() will be used instead.
Definition at line 471 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setAttentionIconByName | ( | const QString & | name | ) |
Sets a new icon that should be used when the application wants to request attention (usually the systemtray will blink between this icon and the main one)
- Parameters
-
name KIconLoader-compatible name of icon to use
Definition at line 266 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setAttentionIconByPixmap | ( | const QIcon & | icon | ) |
Sets the pixmap of the requesting attention icon.
Use setAttentionIcon(const QString) instead when possible.
- Parameters
-
icon QIcon to use for requesting attention.
Definition at line 282 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setAttentionMovieByName | ( | const QString & | name | ) |
Sets a movie as the requesting attention icon.
This overrides anything set in setAttentionIcon()
Definition at line 299 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setCategory | ( | const ItemCategory | category | ) |
Sets the category for this icon, usually it's needed to call this function only once.
- Parameters
-
category the new category for this icon
Definition at line 134 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setContextMenu | ( | KMenu * | menu | ) |
Sets a new context menu for this StatusNotifierItem.
the menu will be shown with a contextMenu(int,int) call by the systemtray over dbus usually you don't need to call this unless you want to use a custom KMenu subclass as context menu
Definition at line 432 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setIconByName | ( | const QString & | name | ) |
Sets a new main icon for the system tray.
- Parameters
-
name it must be a KIconLoader compatible name, this is the preferred way to set an icon
Definition at line 172 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setIconByPixmap | ( | const QIcon & | icon | ) |
Sets a new main icon for the system tray.
- Parameters
-
pixmap our icon, use setIcon(const QString) when possible
Definition at line 191 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setOverlayIconByName | ( | const QString & | name | ) |
Sets an icon to be used as overlay for the main one.
- Parameters
-
icon name, if name is and empty QString() (and overlayIconPixmap() is empty too) the icon will be removed
Definition at line 212 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setOverlayIconByPixmap | ( | const QIcon & | icon | ) |
Sets an icon to be used as overlay for the main one setOverlayIconByPixmap(QIcon()) will remove the overlay when overlayIconName() is empty too.
- Parameters
-
pixmap our overlay icon, use setOverlayIcon(const QString) when possible.
Definition at line 237 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setStandardActionsEnabled | ( | bool | enabled | ) |
Sets whether to show the standard items in the menu, such as Quit.
Definition at line 522 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setStatus | ( | const ItemStatus | status | ) |
Sets a new status for this icon.
Definition at line 154 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setTitle | ( | const QString & | title | ) |
Sets a title for this icon.
Definition at line 149 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setToolTip | ( | const QString & | iconName, |
const QString & | title, | ||
const QString & | subTitle | ||
) |
Sets a new toolTip or this icon, a toolTip is composed of an icon, a title ad a text, all fields are optional.
- Parameters
-
iconName a KIconLoader compatible name for the tootip icon title tootip title subTitle subtitle for the toolTip
Definition at line 325 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setToolTip | ( | const QIcon & | icon, |
const QString & | title, | ||
const QString & | subTitle | ||
) |
Sets a new toolTip or this status notifier item.
This is an overloaded member provided for convenience
Definition at line 345 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setToolTipIconByName | ( | const QString & | name | ) |
Set a new icon for the toolTip.
- Parameters
-
name the name for the icon
Definition at line 366 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setToolTipIconByPixmap | ( | const QIcon & | icon | ) |
Set a new icon for the toolTip.
Use setToolTipIconByName(QString) if possible.
- Parameters
-
pixmap representing the icon
Definition at line 382 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setToolTipSubTitle | ( | const QString & | subTitle | ) |
Sets a new subtitle for the toolTip.
Definition at line 417 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::setToolTipTitle | ( | const QString & | title | ) |
Sets a new title for the toolTip.
Definition at line 399 of file kstatusnotifieritem.cpp.
void KStatusNotifierItem::showMessage | ( | const QString & | title, |
const QString & | message, | ||
const QString & | icon, | ||
int | timeout = 10000 |
||
) |
Shows the user a notification.
If possible use KNotify instead
- Parameters
-
title message title message the actual text shown to the user icon icon to be shown to the user timeout how much time will elaps before hiding the message
Definition at line 551 of file kstatusnotifieritem.cpp.
bool KStatusNotifierItem::standardActionsEnabled | ( | ) | const |
- Returns
- if the standard items in the menu, such as Quit
Definition at line 546 of file kstatusnotifieritem.cpp.
ItemStatus KStatusNotifierItem::status | ( | ) | const |
- Returns
- the current application status
QString KStatusNotifierItem::title | ( | ) | const |
- Returns
- the title of this icon
QString KStatusNotifierItem::toolTipIconName | ( | ) | const |
- Returns
- the name of the toolTip icon if toolTipImage() is not empty this will always return an empty string
QIcon KStatusNotifierItem::toolTipIconPixmap | ( | ) | const |
- Returns
- a serialization of the toolTip icon data
Definition at line 394 of file kstatusnotifieritem.cpp.
QString KStatusNotifierItem::toolTipSubTitle | ( | ) | const |
- Returns
- the subtitle of the main icon toolTip
QString KStatusNotifierItem::toolTipTitle | ( | ) | const |
- Returns
- the title of the main icon toolTip
Property Documentation
|
readwrite |
Definition at line 83 of file kstatusnotifieritem.h.
|
readwrite |
Definition at line 78 of file kstatusnotifieritem.h.
|
readwrite |
Definition at line 81 of file kstatusnotifieritem.h.
|
readwrite |
Definition at line 82 of file kstatusnotifieritem.h.
|
readwrite |
Definition at line 80 of file kstatusnotifieritem.h.
|
readwrite |
Definition at line 79 of file kstatusnotifieritem.h.
|
readwrite |
Definition at line 84 of file kstatusnotifieritem.h.
|
readwrite |
Definition at line 86 of file kstatusnotifieritem.h.
|
readwrite |
Definition at line 85 of file kstatusnotifieritem.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.