KDEUI
#include <kuniqueapplication.h>
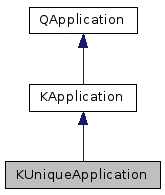
Public Types | |
enum | StartFlag { NonUniqueInstance = 0x1 } |
Public Member Functions | |
KUniqueApplication (bool GUIenabled=true, bool configUnique=false) | |
KUniqueApplication (Display *display, Qt::HANDLE visual=0, Qt::HANDLE colormap=0, bool configUnique=false) | |
virtual | ~KUniqueApplication () |
virtual int | newInstance () |
bool | restoringSession () |
![]() | |
KApplication (bool GUIenabled=true) | |
KApplication (Display *display, Qt::HANDLE visual=0, Qt::HANDLE colormap=0) | |
KApplication (Display *display, int &argc, char **argv, const QByteArray &rAppName, bool GUIenabled=true) | |
virtual | ~KApplication () |
void | clearStartupId () |
void | commitData (QSessionManager &sm) |
void | disableSessionManagement () |
void | enableSessionManagement () |
void | iceIOErrorHandler (_IceConn *conn) |
void | installX11EventFilter (QWidget *filter) |
bool | notify (QObject *receiver, QEvent *event) |
void | removeX11EventFilter (const QWidget *filter) |
void | saveState (QSessionManager &sm) |
KConfig * | sessionConfig () |
bool | sessionSaving () const |
void | setStartupId (const QByteArray &startup_id) |
void | setSynchronizeClipboard (bool synchronize) |
void | setTopWidget (QWidget *topWidget) |
QByteArray | startupId () const |
void | updateRemoteUserTimestamp (const QString &service, int time=0) |
unsigned long | userTimestamp () const |
int | xErrhandler (Display *, void *) |
int | xioErrhandler (Display *) |
Static Public Member Functions | |
static void | addCmdLineOptions () |
static void | setHandleAutoStarted () |
static bool | start (StartFlags flags) |
static bool | start () |
![]() | |
static QString | checkRecoverFile (const QString &pFilename, bool &bRecover) |
static KApplication * | kApplication () |
static QString | tempSaveName (const QString &pFilename) |
Additional Inherited Members | |
![]() | |
Q_SCRIPTABLE void | quit () |
Q_SCRIPTABLE void | reparseConfiguration () |
Q_SCRIPTABLE void | updateUserTimestamp (int time=0) |
![]() | |
void | saveYourself () |
![]() | |
static bool | loadedByKdeinit = false |
![]() | |
KApplication (bool GUIenabled, const KComponentData &cData) | |
KApplication (Display *display, Qt::HANDLE visual, Qt::HANDLE colormap, const KComponentData &cData) | |
bool | x11EventFilter (XEvent *) |
![]() | |
static KApplication * | KApp = 0L |
Detailed Description
KUniqueApplication is a KApplication which only uses a single process.
When a KUniqueApplication is started, it attempts to contact an existing copy of the application. If successful, the program asks the existing process to create a new instance by calling its newInstance() method and then exits. If there is no existing process then the program forks and calls the newInstance() method. When newInstance() is called, the application will typically create a new window or activate an existing one.
Instances of KUniqueApplication can be made to behave like a normal application by passing the StartFlag::NonUniqueInstance flag to start().
Please note that this supports only one process per KDE session. If your application can only be opened once per user or once per host, you need to ensure this independently of KUniqueApplication.
The .desktop file for the application should state X-DBUS-StartupType=Unique, see ktoolinvocation.h
If you use command line options before start() is called, you MUST call addCmdLineOptions() so that the KUniqueApplication-specific command-line options can still work.
If your application is used to open files, it should also support the –tempfile option (see KCmdLineArgs::addTempFileOption()), to delete tempfiles after use. Add X-KDE-HasTempFileOption=true to the .desktop file to indicate this.
- See also
- KApplication
Definition at line 55 of file kuniqueapplication.h.
Member Enumeration Documentation
These flags can be used to specify how new instances of unique applications are created.
Enumerator | |
---|---|
NonUniqueInstance |
Create a new instance of the application in a new process and do not attempt to re-use an existing process. With this flag set, the new instance of the application will behave as if it were a plain KApplication rather than a KUniqueApplication. This is useful if you have an application where all instances are typically run in a single process but under certain circumstances new instances may require their own process. |
Definition at line 106 of file kuniqueapplication.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
Takes command line arguments from KCmdLineArgs
- Parameters
-
GUIenabled Set to false to disable all GUI stuff. This implies no styles either. configUnique If true, the uniqueness of the application will depend on the value of the "MultipleInstances" key in the "KDE" group of the application config file.
Definition at line 312 of file kuniqueapplication.cpp.
|
explicit |
Constructor.
Takes command line arguments from KCmdLineArgs
- Parameters
-
display Will be passed to Qt as the X display. The display must be valid and already opened. visual Pointer to the X11 visual that should be used by the application. If NULL, the default visual will be used instead. colormap The colormap that should be used by the application. If 0, the default colormap will be used instead. configUnique If true, the uniqueness of the application will depend on the value of the "MultipleInstances" key in the "KDE" group of the application config file.
Definition at line 329 of file kuniqueapplication.cpp.
|
virtual |
Destructor.
Definition at line 347 of file kuniqueapplication.cpp.
Member Function Documentation
|
static |
Adds command line options specific for KUniqueApplication.
Should be called before calling KUniqueApplication constructor and / or start().
Definition at line 85 of file kuniqueapplication.cpp.
|
virtual |
Creates a new "instance" of the application.
Usually this will involve making some calls into the GUI portion of your application asking for a new window to be created, possibly with some data already loaded based on the arguments received.
Command line arguments have been passed to KCmdLineArgs before this function is called and can be checked in the usual way.
The default implementation ensures the mainwindow of the already running instance is shown and activated if necessary. If your application has only one mainwindow, you should call this default implementation and only add your special handling if needed.
Note that newInstance() is called also in the first started application process.
For applications that share one process for several mainwindows, the reimplementation could be:
- Returns
- An exit value. The calling process will exit with this value.
Definition at line 390 of file kuniqueapplication.cpp.
bool KUniqueApplication::restoringSession | ( | ) |
Returns whether newInstance() is being called while session restoration is in progress.
Definition at line 385 of file kuniqueapplication.cpp.
|
static |
Definition at line 418 of file kuniqueapplication.cpp.
|
static |
Forks and registers with D-Bus.
The command line arguments are being sent via D-Bus to newInstance() and will be received once the application enters the event loop.
Typically this is used like:
Note that it's not necessary to call start() explicitly. It will be called automatically before creating KUniqueApplication if it hasn't been called yet, without any performance impact.
Also note that you MUST call KUniqueApplication::addCmdLineOptions(), if you use command line options before start() is called.
- Parameters
-
flags Optional flags which control how a new instance of the application is started.
- Returns
- true if registration is successful. false if another process was already running.
Definition at line 114 of file kuniqueapplication.cpp.
|
static |
Definition at line 108 of file kuniqueapplication.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.