KDEUI
#include <netwm_def.h>
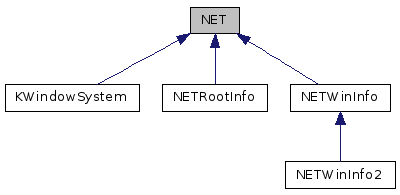
Static Public Member Functions | |
static int | timestampCompare (unsigned long time1, unsigned long time2) |
static int | timestampDiff (unsigned long time1, unsigned long time2) |
static bool | typeMatchesMask (WindowType type, unsigned long mask) |
Detailed Description
Base namespace class.
The NET API is an implementation of the NET Window Manager Specification.
This class is the base class for the NETRootInfo and NETWinInfo classes, which are used to retrieve and modify the properties of windows. To keep the namespace relatively clean, all enums are defined here.
Definition at line 283 of file netwm_def.h.
Member Enumeration Documentation
anonymous enum |
Sentinel value to indicate that the client wishes to be visible on all desktops.
Enumerator | |
---|---|
OnAllDesktops |
Definition at line 704 of file netwm_def.h.
enum NET::Action |
Actions that can be done with a window (_NET_WM_ALLOWED_ACTIONS).
Enumerator | |
---|---|
ActionMove | |
ActionResize | |
ActionMinimize | |
ActionShade | |
ActionStick | |
ActionMaxVert | |
ActionMaxHoriz | |
ActionMax | |
ActionFullScreen | |
ActionChangeDesktop | |
ActionClose |
Definition at line 553 of file netwm_def.h.
Starting corner for desktop layout.
Enumerator | |
---|---|
DesktopLayoutCornerTopLeft | |
DesktopLayoutCornerTopRight | |
DesktopLayoutCornerBottomLeft | |
DesktopLayoutCornerBottomRight |
Definition at line 736 of file netwm_def.h.
enum NET::Direction |
Direction for WMMoveResize.
When a client wants the Window Manager to start a WMMoveResize, it should specify one of:
- TopLeft
- Top
- TopRight
- Right
- BottomRight
- Bottom
- BottomLeft
- Left
- Move (for movement only)
- KeyboardSize (resizing via keyboard)
- KeyboardMove (movement via keyboard)
Enumerator | |
---|---|
TopLeft | |
Top | |
TopRight | |
Right | |
BottomRight | |
Bottom | |
BottomLeft | |
Left | |
Move | |
KeyboardSize | |
KeyboardMove | |
MoveResizeCancel |
Definition at line 512 of file netwm_def.h.
enum NET::MappingState |
Client window mapping state.
The class automatically watches the mapping state of the client windows, and uses the mapping state to determine how to set/change different properties. Note that this is very lowlevel and you most probably don't want to use this state.
Enumerator | |
---|---|
Visible |
indicates the client window is visible to the user. |
Withdrawn |
indicates that neither the client window nor its icon is visible. |
Iconic |
indicates that the client window is not visible, but its icon is. This can be when the window is minimized or when it's on a different virtual desktop. See also NET::Hidden. |
Definition at line 533 of file netwm_def.h.
enum NET::Orientation |
Orientation.
Enumerator | |
---|---|
OrientationHorizontal | |
OrientationVertical |
Definition at line 728 of file netwm_def.h.
enum NET::Property |
Supported properties.
Clients and Window Managers must define which properties/protocols it wants to support.
Root/Desktop window properties and protocols:
- Supported
- ClientList
- ClientListStacking
- NumberOfDesktops
- DesktopGeometry
- DesktopViewport
- CurrentDesktop
- DesktopNames
- ActiveWindow
- WorkArea
- SupportingWMCheck
- VirtualRoots
- CloseWindow
- WMMoveResize
Client window properties and protocols:
- WMName
- WMVisibleName
- WMDesktop
- WMWindowType
- WMState
- WMStrut (obsoleted by WM2ExtendedStrut)
- WMGeometry
- WMFrameExtents
- WMIconGeometry
- WMIcon
- WMIconName
- WMVisibleIconName
- WMHandledIcons
- WMPid
- WMPing
ICCCM properties (provided for convenience):
- XAWMState
Definition at line 612 of file netwm_def.h.
enum NET::Property2 |
Supported properties.
This enum is an extension to NET::Property, because them enum is limited only to 32 bits.
Client window properties and protocols:
- WM2UserTime
- WM2StartupId
- WM2TransientFor mainwindow for the window (WM_TRANSIENT_FOR)
- WM2GroupLeader group leader (window_group in WM_HINTS)
- WM2AllowedActions
- WM2RestackWindow
- WM2MoveResizeWindow
- WM2ExtendedStrut
- WM2TemporaryRules internal, for kstart
- WM2WindowClass WM_CLASS
- WM2WindowRole WM_WINDOW_ROLE
- WM2ClientMachine WM_CLIENT_MACHINE
- WM2ShowingDesktop
- WM2Opacity _NET_WM_WINDOW_OPACITY
- WM2DesktopLayout _NET_DESKTOP_LAYOUT
- WM2FullPlacement _NET_WM_FULL_PLACEMENT
- WM2FullscreenMonitors _NET_WM_FULLSCREEN_MONITORS
Definition at line 675 of file netwm_def.h.
enum NET::RequestSource |
Source of the request.
Definition at line 710 of file netwm_def.h.
enum NET::Role |
Application role.
This is used internally to determine how several action should be performed (if at all).
Enumerator | |
---|---|
Client |
indicates that the application is a client application. |
WindowManager |
indicates that the application is a window manager application. |
Definition at line 290 of file netwm_def.h.
enum NET::State |
Window state.
To set the state of a window, you'll typically do something like:
for example to not show the window on the taskbar and desktop pager. winId() is a function of QWidget()
Note that KeepAbove (StaysOnTop) and KeepBelow are meant as user preference and applications should avoid setting these states themselves.
Enumerator | |
---|---|
Modal |
indicates that this is a modal dialog box. The WM_TRANSIENT_FOR hint MUST be set to indicate which window the dialog is a modal for, or set to the root window if the dialog is a modal for its window group. |
Sticky |
indicates that the Window Manager SHOULD keep the window's position fixed on the screen, even when the virtual desktop scrolls. Note that this is different from being kept on all desktops. |
MaxVert |
indicates that the window is vertically maximized. |
MaxHoriz |
indicates that the window is horizontally maximized. |
Max |
convenience value. Equal to MaxVert | MaxHoriz. |
Shaded |
indicates that the window is shaded (rolled-up). |
SkipTaskbar |
indicates that a window should not be included on a taskbar. |
KeepAbove |
indicates that a window should on top of most windows (but below fullscreen windows). |
StaysOnTop |
|
SkipPager |
indicates that a window should not be included on a pager. |
Hidden |
indicates that a window should not be visible on the screen (e.g. when minimised). Only the window manager is allowed to change it. |
FullScreen |
indicates that a window should fill the entire screen and have no window decorations. |
KeepBelow |
indicates that a window should be below most windows (but above any desktop windows). |
DemandsAttention |
there was an attempt to activate this window, but the window manager prevented this. E.g. taskbar should mark such window specially to bring user's attention to this window. Only the window manager is allowed to change it. |
Definition at line 425 of file netwm_def.h.
enum NET::WindowType |
Window type.
Enumerator | |
---|---|
Unknown |
indicates that the window did not define a window type. |
Normal |
indicates that this is a normal, top-level window |
Desktop |
indicates a desktop feature. This can include a single window containing desktop icons with the same dimensions as the screen, allowing the desktop environment to have full control of the desktop, without the need for proxying root window clicks. |
Dock |
indicates a dock or panel feature |
Toolbar |
indicates a toolbar window |
Menu |
indicates a pinnable (torn-off) menu window |
Dialog |
indicates that this is a dialog window |
Override |
|
TopMenu |
indicates a toplevel menu (AKA macmenu). This is a KDE extension to the _NET_WM_WINDOW_TYPE mechanism. |
Utility |
indicates a utility window |
Splash |
indicates that this window is a splash screen window. |
DropdownMenu |
indicates a dropdown menu (from a menubar typically) |
PopupMenu |
indicates a popup menu (a context menu typically) |
Tooltip |
indicates a tooltip window |
Notification |
indicates a notification window |
ComboBox |
indicates that the window is a list for a combobox |
DNDIcon |
indicates a window that represents the dragged object during DND operation |
Definition at line 305 of file netwm_def.h.
enum NET::WindowTypeMask |
Values for WindowType when they should be OR'ed together, e.g.
for the properties argument of the NETRootInfo constructor.
Enumerator | |
---|---|
NormalMask |
|
DesktopMask |
|
DockMask |
|
ToolbarMask |
|
MenuMask |
|
DialogMask |
|
OverrideMask |
|
TopMenuMask |
|
UtilityMask |
|
SplashMask |
|
DropdownMenuMask |
|
PopupMenuMask |
|
TooltipMask |
|
NotificationMask |
|
ComboBoxMask |
|
DNDIconMask |
|
AllTypesMask |
All window types. |
Definition at line 384 of file netwm_def.h.
Member Function Documentation
|
static |
Compares two X timestamps, taking into account wrapping and 64bit architectures.
Return value is like with strcmp(), 0 for equal, -1 for time1 < time2, 1 for time1 > time2.
- Deprecated:
- Moved to KXUtils namespace.
|
static |
Returns a difference of two X timestamps, time2 - time1, where time2 must be later than time1, as returned by timestampCompare().
- Deprecated:
- Moved to KXUtils namespace.
|
static |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.