KDEUI
#include <netwm.h>
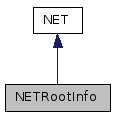
Public Member Functions | |
NETRootInfo (Display *display, Window supportWindow, const char *wmName, const unsigned long properties[], int properties_size, int screen=-1, bool doActivate=true) | |
NETRootInfo (Display *display, const unsigned long properties[], int properties_size, int screen=-1, bool doActivate=true) | |
NETRootInfo (Display *display, unsigned long properties, int screen=-1, bool doActivate=true) | |
NETRootInfo (const NETRootInfo &rootinfo) | |
virtual | ~NETRootInfo () |
void | activate () |
Window | activeWindow () const |
const Window * | clientList () const |
int | clientListCount () const |
const Window * | clientListStacking () const |
int | clientListStackingCount () const |
void | closeWindowRequest (Window window) |
int | currentDesktop (bool ignore_viewport=false) const |
NETSize | desktopGeometry (int desktop) const |
QSize | desktopLayoutColumnsRows () const |
NET::DesktopLayoutCorner | desktopLayoutCorner () const |
NET::Orientation | desktopLayoutOrientation () const |
const char * | desktopName (int desktop) const |
NETPoint | desktopViewport (int desktop) const |
void | event (XEvent *event, unsigned long *properties, int properties_size) |
unsigned long | event (XEvent *event) |
bool | isSupported (NET::Property property) const |
bool | isSupported (NET::Property2 property) const |
bool | isSupported (NET::WindowType type) const |
bool | isSupported (NET::State state) const |
bool | isSupported (NET::Action action) const |
void | moveResizeRequest (Window window, int x_root, int y_root, Direction direction) |
void | moveResizeWindowRequest (Window window, int flags, int x, int y, int width, int height) |
int | numberOfDesktops (bool ignore_viewport=false) const |
const NETRootInfo & | operator= (const NETRootInfo &rootinfo) |
const unsigned long * | passedProperties () const |
void | restackRequest (Window window, RequestSource source, Window above, int detail, Time timestamp) |
Window | rootWindow () const |
int | screenNumber () const |
void | sendPing (Window window, Time timestamp) |
void | setActiveWindow (Window window, NET::RequestSource src, Time timestamp, Window active_window) |
void | setActiveWindow (Window window) |
void | setClientList (const Window *windows, unsigned int count) |
void | setClientListStacking (const Window *windows, unsigned int count) |
void | setCurrentDesktop (int desktop, bool ignore_viewport=false) |
void | setDesktopGeometry (int desktop, const NETSize &geometry) |
void | setDesktopLayout (NET::Orientation orientation, int columns, int rows, NET::DesktopLayoutCorner corner) |
void | setDesktopName (int desktop, const char *desktopName) |
void | setDesktopViewport (int desktop, const NETPoint &viewport) |
void | setNumberOfDesktops (int numberOfDesktops) |
void | setShowingDesktop (bool showing) |
void | setSupported (NET::Property property, bool on=true) |
void | setSupported (NET::Property2 property, bool on=true) |
void | setSupported (NET::WindowType property, bool on=true) |
void | setSupported (NET::State property, bool on=true) |
void | setSupported (NET::Action property, bool on=true) |
void | setVirtualRoots (const Window *windows, unsigned int count) |
void | setWorkArea (int desktop, const NETRect &workArea) |
bool | showingDesktop () const |
const unsigned long * | supportedProperties () const |
Window | supportWindow () const |
void | takeActivity (Window window, Time timestamp, long flags) |
const Window * | virtualRoots () const |
int | virtualRootsCount () const |
const char * | wmName () const |
NETRect | workArea (int desktop) const |
Display * | x11Display () const |
Protected Member Functions | |
virtual void | addClient (Window window) |
virtual void | changeActiveWindow (Window window, NET::RequestSource src, Time timestamp, Window active_window) |
virtual void | changeCurrentDesktop (int desktop) |
virtual void | changeDesktopGeometry (int desktop, const NETSize &geom) |
virtual void | changeDesktopViewport (int desktop, const NETPoint &viewport) |
virtual void | changeNumberOfDesktops (int numberOfDesktops) |
virtual void | changeShowingDesktop (bool showing) |
virtual void | closeWindow (Window window) |
virtual void | gotPing (Window window, Time timestamp) |
virtual void | gotTakeActivity (Window window, Time timestamp, long flags) |
virtual void | moveResize (Window window, int x_root, int y_root, unsigned long direction) |
virtual void | moveResizeWindow (Window window, int flags, int x, int y, int width, int height) |
virtual void | removeClient (Window window) |
virtual void | restackWindow (Window window, RequestSource source, Window above, int detail, Time timestamp) |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
static int | timestampCompare (unsigned long time1, unsigned long time2) |
static int | timestampDiff (unsigned long time1, unsigned long time2) |
static bool | typeMatchesMask (WindowType type, unsigned long mask) |
Detailed Description
Common API for root window properties/protocols.
The NETRootInfo class provides a common API for clients and window managers to set/read/change properties on the root window as defined by the NET Window Manager Specification..
- See also
- NET
- NETWinInfo
Member Enumeration Documentation
anonymous enum |
Constructor & Destructor Documentation
NETRootInfo::NETRootInfo | ( | Display * | display, |
Window | supportWindow, | ||
const char * | wmName, | ||
const unsigned long | properties[], | ||
int | properties_size, | ||
int | screen = -1 , |
||
bool | doActivate = true |
||
) |
Window Managers should use this constructor to create a NETRootInfo object, which will be used to set/update information stored on the rootWindow.
The application role is automatically set to WindowManager when using this constructor.
- Parameters
-
display An X11 Display struct. supportWindow The Window id of the supportWindow. The supportWindow must be created by the window manager as a child of the rootWindow. The supportWindow must not be destroyed until the Window Manager exits. wmName A string which should be the window manager's name (ie. "KWin" or "Blackbox"). properties An array of elements listing all properties and protocols the window manager supports. The elements contain OR'ed values of constants from the NET base class, in the following order: [0]= NET::Property, [1]= NET::WindowTypeMask (not NET::WindowType!), [2]= NET::State, [3]= NET::Property2, [4]= NET::Action. In future versions, the list may be extended. In case you pass less elements, the missing ones will be replaced with default values. properties_size The number of elements in the properties array. screen For Window Managers that support multiple screen (ie. "multiheaded") displays, the screen number may be explicitly defined. If this argument is omitted, the default screen will be used. doActivate true to activate the window
NETRootInfo::NETRootInfo | ( | Display * | display, |
const unsigned long | properties[], | ||
int | properties_size, | ||
int | screen = -1 , |
||
bool | doActivate = true |
||
) |
Clients should use this constructor to create a NETRootInfo object, which will be used to query information set on the root window.
The application role is automatically set to Client when using this constructor.
- Parameters
-
display An X11 Display struct. properties An array of elements listing all protocols the client is interested in. The elements contain OR'ed values of constants from the NET base class, in the following order: [0]= NET::Property, [1]= NET::Property2. properties_size The number of elements in the properties array. screen For Clients that support multiple screen (ie. "multiheaded") displays, the screen number may be explicitly defined. If this argument is omitted, the default screen will be used. doActivate true to call activate() to do an initial data read/update of the query information.
NETRootInfo::NETRootInfo | ( | Display * | display, |
unsigned long | properties, | ||
int | screen = -1 , |
||
bool | doActivate = true |
||
) |
This constructor differs from the above one only in the way it accepts the list of supported properties.
The properties argument is equivalent to the first element of the properties array in the above constructor, and therefore you cannot read all root window properties using it.
NETRootInfo::NETRootInfo | ( | const NETRootInfo & | rootinfo | ) |
Creates a shared copy of the specified NETRootInfo object.
- Parameters
-
rootinfo the NETRootInfo object to copy
|
virtual |
Destroys the NETRootInfo object.
Member Function Documentation
void NETRootInfo::activate | ( | ) |
Window Managers must call this after creating the NETRootInfo object, and before using any other method in the class.
This method sets initial data on the root window and does other post-construction duties.
Clients must also call this after creating the object to do an initial data read/update.
Window NETRootInfo::activeWindow | ( | ) | const |
|
inlineprotectedvirtual |
A Client should subclass NETRootInfo and reimplement this function when it wants to know when a window has been added.
- Parameters
-
window the id of the window to add
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to change the active (focused) window.
- Parameters
-
window the id of the window to activate src the source from which the request came timestamp the timestamp of the user action causing this request active_window active window of the requesting application, if any
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to change the current desktop.
- Parameters
-
desktop the number of the desktop
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to change the specified desktop geometry.
- Parameters
-
desktop the number of the desktop geom the new size
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to change the specified desktop viewport.
- Parameters
-
desktop the number of the desktop viewport the new position of the viewport
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to change the number of desktops.
- Parameters
-
numberOfDesktops the new number of desktops
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a pager made a request to change showing the desktop.
See _NET_SHOWING_DESKTOP for details.
- Parameters
-
showing whether to activate the showing desktop mode
const Window * NETRootInfo::clientList | ( | ) | const |
Returns an array of Window id's, which contain all managed windows.
- Returns
- the array of Window id's
- See also
- clientListCount()
int NETRootInfo::clientListCount | ( | ) | const |
Returns the number of managed windows in clientList array.
- Returns
- the number of managed windows in the clientList array
- See also
- clientList()
const Window * NETRootInfo::clientListStacking | ( | ) | const |
Returns an array of Window id's, which contain all managed windows in stacking order.
- Returns
- the array of Window id's in stacking order
- See also
- clientListStackingCount()
int NETRootInfo::clientListStackingCount | ( | ) | const |
Returns the number of managed windows in the clientListStacking array.
- Returns
- the number of Window id's in the client list
- See also
- clientListStacking()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to close a window.
- Parameters
-
window the id of the window to close
void NETRootInfo::closeWindowRequest | ( | Window | window | ) |
int NETRootInfo::currentDesktop | ( | bool | ignore_viewport = false | ) | const |
Returns the current desktop.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. They are however mapped to virtual desktops if needed.
- Parameters
-
ignore_viewport if false, viewport is mapped to virtual desktops
- Returns
- the number of the current desktop
NETSize NETRootInfo::desktopGeometry | ( | int | desktop | ) | const |
Returns the desktop geometry size.
The desktop argument is ignored. Early drafts of the NET WM Specification were unclear about the semantics of this property.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. You should use calls for virtual desktops, viewport is mapped to them if needed.
- Parameters
-
desktop the number of the desktop
- Returns
- the size of the desktop
QSize NETRootInfo::desktopLayoutColumnsRows | ( | ) | const |
NET::DesktopLayoutCorner NETRootInfo::desktopLayoutCorner | ( | ) | const |
NET::Orientation NETRootInfo::desktopLayoutOrientation | ( | ) | const |
const char * NETRootInfo::desktopName | ( | int | desktop | ) | const |
NETPoint NETRootInfo::desktopViewport | ( | int | desktop | ) | const |
Returns the viewport of the specified desktop.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. You should use calls for virtual desktops, viewport is mapped to them if needed.
- Parameters
-
desktop the number of the desktop
- Returns
- the position of the desktop's viewport
void NETRootInfo::event | ( | XEvent * | event, |
unsigned long * | properties, | ||
int | properties_size | ||
) |
This function takes the passed XEvent and returns an OR'ed list of NETRootInfo properties that have changed in the properties argument.
The new information will be read immediately by the class. The elements of the properties argument are as they would be passed to the constructor, if the array is not large enough, changed properties that don't fit in it won't be listed there (they'll be updated in the class though).
- Parameters
-
event the event properties properties that changed properties_size size of the passed properties array
unsigned long NETRootInfo::event | ( | XEvent * | event | ) |
This function takes the passed XEvent and returns an OR'ed list of NETRootInfo properties that have changed.
The new information will be read immediately by the class. This overloaded version returns only a single mask, and therefore cannot check state of all properties like the other variant.
- Parameters
-
event the event
- Returns
- the properties
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to receive replies to the _NET_WM_PING protocol.
- Parameters
-
window the window from which the reply came timestamp timestamp of the ping
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to receive replies to the _NET_WM_TAKE_ACTIVITY protocol.
- Parameters
-
window the window from which the reply came timestamp timestamp of the ping flags flags passed in the original message
bool NETRootInfo::isSupported | ( | NET::Property | property | ) | const |
Returns true if the given property is supported by the window manager.
Note that for Client mode, NET::Supported needs to be passed in the properties argument for this to work.
bool NETRootInfo::isSupported | ( | NET::Property2 | property | ) | const |
bool NETRootInfo::isSupported | ( | NET::WindowType | type | ) | const |
bool NETRootInfo::isSupported | ( | NET::State | state | ) | const |
bool NETRootInfo::isSupported | ( | NET::Action | action | ) | const |
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to start a move/resize.
- Parameters
-
window The window that wants to move/resize x_root X position of the cursor relative to the root window. y_root Y position of the cursor relative to the root window. direction One of NET::Direction (see base class documentation for a description of the different directions).
Clients (such as pagers/taskbars) that wish to start a WMMoveResize (where the window manager controls the resize/movement, i.e.
_NET_WM_MOVERESIZE) should call this function. This will send a request to the Window Manager.
- Parameters
-
window The client window that would be resized/moved. x_root X position of the cursor relative to the root window. y_root Y position of the cursor relative to the root window. direction One of NET::Direction (see base class documentation for a description of the different directions).
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a pager made a request to move/resize a window.
See _NET_MOVERESIZE_WINDOW for details.
- Parameters
-
window the id of the window to more/resize flags Flags specifying the operation (see _NET_MOVERESIZE_WINDOW description) x Requested X position for the window y Requested Y position for the window width Requested width for the window height Requested height for the window
void NETRootInfo::moveResizeWindowRequest | ( | Window | window, |
int | flags, | ||
int | x, | ||
int | y, | ||
int | width, | ||
int | height | ||
) |
Clients (such as pagers/taskbars) that wish to move/resize a window using WM2MoveResizeWindow (_NET_MOVERESIZE_WINDOW) should call this function.
This will send a request to the Window Manager. See _NET_MOVERESIZE_WINDOW description for details.
- Parameters
-
window The client window that would be resized/moved. flags Flags specifying the operation (see _NET_MOVERESIZE_WINDOW description) x Requested X position for the window y Requested Y position for the window width Requested width for the window height Requested height for the window
int NETRootInfo::numberOfDesktops | ( | bool | ignore_viewport = false | ) | const |
Returns the number of desktops.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. They are however mapped to virtual desktops if needed.
- Parameters
-
ignore_viewport if false, viewport is mapped to virtual desktops
- Returns
- the number of desktops
const NETRootInfo & NETRootInfo::operator= | ( | const NETRootInfo & | rootinfo | ) |
const unsigned long * NETRootInfo::passedProperties | ( | ) | const |
|
inlineprotectedvirtual |
A Client should subclass NETRootInfo and reimplement this function when it wants to know when a window has been removed.
- Parameters
-
window the id of the window to remove
void NETRootInfo::restackRequest | ( | Window | window, |
RequestSource | source, | ||
Window | above, | ||
int | detail, | ||
Time | timestamp | ||
) |
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to restack a window.
See _NET_RESTACK_WINDOW for details.
- Parameters
-
window the id of the window to restack source the source of the request above other window in the restack request detail restack detail timestamp the timestamp of the request
Window NETRootInfo::rootWindow | ( | ) | const |
int NETRootInfo::screenNumber | ( | ) | const |
void NETRootInfo::sendPing | ( | Window | window, |
Time | timestamp | ||
) |
void NETRootInfo::setActiveWindow | ( | Window | window, |
NET::RequestSource | src, | ||
Time | timestamp, | ||
Window | active_window | ||
) |
Requests that the specified window becomes the active (focused) one.
- Parameters
-
window the id of the new active window src whether the request comes from normal application or from a pager or similar tool timestamp X server timestamp of the user action that caused the request active_window active window of the requesting application, if any
void NETRootInfo::setActiveWindow | ( | Window | window | ) |
void NETRootInfo::setClientList | ( | const Window * | windows, |
unsigned int | count | ||
) |
void NETRootInfo::setClientListStacking | ( | const Window * | windows, |
unsigned int | count | ||
) |
void NETRootInfo::setCurrentDesktop | ( | int | desktop, |
bool | ignore_viewport = false |
||
) |
Sets the current desktop to the specified desktop.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. It is however mapped to virtual desktops if needed.
- Parameters
-
desktop the number of the desktop ignore_viewport if false, viewport is mapped to virtual desktops
void NETRootInfo::setDesktopGeometry | ( | int | desktop, |
const NETSize & | geometry | ||
) |
Sets the desktop geometry to the specified geometry.
The desktop argument is ignored. Early drafts of the NET WM Specification were unclear about the semantics of this property.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. You should use calls for virtual desktops, viewport is mapped to them if needed.
- Parameters
-
desktop the number of the desktop geometry the new size of the desktop
void NETRootInfo::setDesktopLayout | ( | NET::Orientation | orientation, |
int | columns, | ||
int | rows, | ||
NET::DesktopLayoutCorner | corner | ||
) |
void NETRootInfo::setDesktopName | ( | int | desktop, |
const char * | desktopName | ||
) |
Sets the name of the specified desktop.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. Viewport is mapped to virtual desktops if needed, but not for this call.
- Parameters
-
desktop the number of the desktop desktopName the new name of the desktop
void NETRootInfo::setDesktopViewport | ( | int | desktop, |
const NETPoint & | viewport | ||
) |
Sets the viewport for the current desktop to the specified point.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. You should use calls for virtual desktops, viewport is mapped to them if needed.
- Parameters
-
desktop the number of the desktop viewport the new position of the desktop's viewport
void NETRootInfo::setNumberOfDesktops | ( | int | numberOfDesktops | ) |
Sets the number of desktops to the specified number.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. Viewport is mapped to virtual desktops if needed, but not for this call.
- Parameters
-
numberOfDesktops the number of desktops
void NETRootInfo::setShowingDesktop | ( | bool | showing | ) |
void NETRootInfo::setSupported | ( | NET::Property | property, |
bool | on = true |
||
) |
void NETRootInfo::setSupported | ( | NET::Property2 | property, |
bool | on = true |
||
) |
void NETRootInfo::setSupported | ( | NET::WindowType | property, |
bool | on = true |
||
) |
void NETRootInfo::setSupported | ( | NET::State | property, |
bool | on = true |
||
) |
void NETRootInfo::setSupported | ( | NET::Action | property, |
bool | on = true |
||
) |
void NETRootInfo::setVirtualRoots | ( | const Window * | windows, |
unsigned int | count | ||
) |
void NETRootInfo::setWorkArea | ( | int | desktop, |
const NETRect & | workArea | ||
) |
bool NETRootInfo::showingDesktop | ( | ) | const |
const unsigned long * NETRootInfo::supportedProperties | ( | ) | const |
In the Window Manager mode, this is equivalent to the properties argument passed to the constructor.
In the Client mode, if NET::Supported was passed in the properties argument, the returned value is array of all protocols and properties supported by the Window Manager. The elements of the array are the same as they would be passed to the Window Manager mode constructor, the size is the maximum array size the constructor accepts.
Window NETRootInfo::supportWindow | ( | ) | const |
void NETRootInfo::takeActivity | ( | Window | window, |
Time | timestamp, | ||
long | flags | ||
) |
Sends a take activity message with the given timestamp to the window, using the _NET_WM_TAKE_ACTIVITY protocol (see the WM spec for details).
- Parameters
-
window the window to which the message should be sent timestamp timestamp of the message flags arbitrary flags
|
protectedvirtual |
const Window * NETRootInfo::virtualRoots | ( | ) | const |
Returns an array of Window id's, which contain the virtual root windows.
- Returns
- the array of Window id's
- See also
- virtualRootsCount()
int NETRootInfo::virtualRootsCount | ( | ) | const |
Returns the number of window in the virtualRoots array.
- Returns
- the number of Window id's in the virtual root array
- See also
- virtualRoots()
const char * NETRootInfo::wmName | ( | ) | const |
NETRect NETRootInfo::workArea | ( | int | desktop | ) | const |
Display * NETRootInfo::x11Display | ( | ) | const |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.