KFile
#include <kurlnavigator.h>
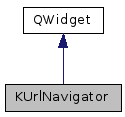
Public Slots | |
void | requestActivation () |
void | setLocationUrl (const KUrl &url) |
Signals | |
void | activated () |
void | editableStateChanged (bool editable) |
void | historyChanged () |
void | returnPressed () |
void | tabRequested (const KUrl &url) |
void | urlAboutToBeChanged (const KUrl &newUrl) |
void | urlChanged (const KUrl &url) |
void | urlsDropped (const KUrl &destination, QDropEvent *event) |
Public Member Functions | |
KUrlNavigator (QWidget *parent=0) | |
KUrlNavigator (KFilePlacesModel *placesModel, const KUrl &url, QWidget *parent) | |
virtual | ~KUrlNavigator () |
QStringList | customProtocols () const |
KUrlComboBox * | editor () const |
bool | goBack () |
bool | goForward () |
void | goHome () |
bool | goUp () |
int | historyIndex () const |
int | historySize () const |
KUrl | homeUrl () const |
bool | isActive () const |
bool | isPlacesSelectorVisible () const |
bool | isUrlEditable () const |
QByteArray | locationState (int historyIndex=-1) const |
KUrl | locationUrl (int historyIndex=-1) const |
void | saveLocationState (const QByteArray &state) |
void | setActive (bool active) |
void | setCustomProtocols (const QStringList &protocols) |
void | setHomeUrl (const KUrl &url) |
void | setPlacesSelectorVisible (bool visible) |
void | setShowFullPath (bool show) |
void | setUrlEditable (bool editable) |
bool | showFullPath () const |
KUrl | uncommittedUrl () const |
Detailed Description
Widget that allows to navigate through the paths of an URL.
The URL navigator offers two modes:
- Editable: The URL of the location is editable inside an editor. By pressing RETURN the URL will get activated.
- Non editable ("breadcrumb view"): The URL of the location is represented by a number of buttons, where each button represents a path of the URL. By clicking on a button the path will get activated. This mode also supports drag and drop of items.
The mode can be changed by clicking on the empty area of the URL navigator. It is recommended that the application remembers the setting or allows to configure the default mode (see KUrlNavigator::setUrlEditable()).
The URL navigator remembers the URL history during navigation and allows to go back and forward within this history.
In the non editable mode ("breadcrumb view") it can be configured whether the full path should be shown. It is recommended that the application remembers the setting or allows to configure the default mode (see KUrlNavigator::setShowFullPath()).
The typical usage of the KUrlNavigator is:
- Create an instance providing a places model and an URL.
- Create an instance of QAbstractItemView which shows the content of the URL given by the URL navigator.
- Connect to the signal KUrlNavigator::urlChanged() and synchronize the content of QAbstractItemView with the URL given by the URL navigator.
It is recommended, that the application remembers the state of the QAbstractItemView when the URL has been changed. This allows to restore the view state when going back in history. KUrlNavigator offers support for remembering the view state:
- The signal urlAboutToBeChanged() will be emitted before the URL change takes places. This allows the application to store the view state by KUrlNavigator::saveLocationState().
- The signal urlChanged() will be emitted after the URL change took place. This allows the application to restore the view state by getting the values from KUrlNavigator::locationState().
Definition at line 75 of file kurlnavigator.h.
Constructor & Destructor Documentation
KUrlNavigator::KUrlNavigator | ( | QWidget * | parent = 0 | ) |
- Since
- 4.5
Definition at line 810 of file kurlnavigator.cpp.
KUrlNavigator::KUrlNavigator | ( | KFilePlacesModel * | placesModel, |
const KUrl & | url, | ||
QWidget * | parent | ||
) |
- Parameters
-
placesModel Model for the places which are selectable inside a menu. A place can be a bookmark or a device. If it is 0, no places selector is displayed. url URL which is used for the navigation or editing. parent Parent widget.
Definition at line 817 of file kurlnavigator.cpp.
|
virtual |
Definition at line 826 of file kurlnavigator.cpp.
Member Function Documentation
|
signal |
Is emitted, if the URL navigator has been activated by an user interaction.
- See also
- KUrlNavigator::setActive()
QStringList KUrlNavigator::customProtocols | ( | ) | const |
- Returns
- The custom protocols if they are set, QStringList() otherwise.
Definition at line 1201 of file kurlnavigator.cpp.
|
signal |
Is emitted, if the editable state for the URL has been changed (see KUrlNavigator::setUrlEditable()).
KUrlComboBox * KUrlNavigator::editor | ( | ) | const |
- Returns
- The used editor when the navigator is in the edit mode
- See also
- KUrlNavigator::setUrlEditable()
Definition at line 1190 of file kurlnavigator.cpp.
bool KUrlNavigator::goBack | ( | ) |
Goes back one step in the URL history.
The signals KUrlNavigator::urlAboutToBeChanged(), KUrlNavigator::urlChanged() and KUrlNavigator::historyChanged() are emitted if true is returned. False is returned if the beginning of the history has already been reached and hence going back was not possible. The history index (see KUrlNavigator::historyIndex()) is increased by one if the operation was successful.
Definition at line 848 of file kurlnavigator.cpp.
bool KUrlNavigator::goForward | ( | ) |
Goes forward one step in the URL history.
The signals KUrlNavigator::urlAboutToBeChanged(), KUrlNavigator::urlChanged() and KUrlNavigator::historyChanged() are emitted if true is returned. False is returned if the end of the history has already been reached and hence going forward was not possible. The history index (see KUrlNavigator::historyIndex()) is decreased by one if the operation was successful.
Definition at line 866 of file kurlnavigator.cpp.
void KUrlNavigator::goHome | ( | ) |
Goes to the home URL and remembers the old URL in the history.
The signals KUrlNavigator::urlAboutToBeChanged(), KUrlNavigator::urlChanged() and KUrlNavigator::historyChanged() are emitted.
- See also
- KUrlNavigator::setHomeUrl()
Definition at line 895 of file kurlnavigator.cpp.
bool KUrlNavigator::goUp | ( | ) |
Goes up one step of the URL path and remembers the old path in the history.
The signals KUrlNavigator::urlAboutToBeChanged(), KUrlNavigator::urlChanged() and KUrlNavigator::historyChanged() are emitted if true is returned. False is returned if going up was not possible as the root has been reached.
Definition at line 883 of file kurlnavigator.cpp.
|
signal |
Is emitted, if the history has been changed.
Usually the history is changed if a new URL has been selected.
int KUrlNavigator::historyIndex | ( | ) | const |
- Returns
- The history index of the current location, where 0 <= history index < KUrlNavigator::historySize(). 0 is the most recent history entry.
Definition at line 1185 of file kurlnavigator.cpp.
int KUrlNavigator::historySize | ( | ) | const |
- Returns
- The amount of locations in the history. The data for each location can be retrieved by KUrlNavigator::locationUrl() and KUrlNavigator::locationState().
Definition at line 1180 of file kurlnavigator.cpp.
KUrl KUrlNavigator::homeUrl | ( | ) | const |
Definition at line 909 of file kurlnavigator.cpp.
bool KUrlNavigator::isActive | ( | ) | const |
- Returns
- True, if the URL navigator is in the active mode.
- See also
- KUrlNavigator::setActive()
Definition at line 957 of file kurlnavigator.cpp.
bool KUrlNavigator::isPlacesSelectorVisible | ( | ) | const |
- Returns
- True, if the places selector is visible.
Definition at line 978 of file kurlnavigator.cpp.
bool KUrlNavigator::isUrlEditable | ( | ) | const |
- Returns
- True, if the URL is editable within a line editor. If false is returned, each part of the URL is presented by a button for fast navigation ("breadcrumb view").
Definition at line 921 of file kurlnavigator.cpp.
QByteArray KUrlNavigator::locationState | ( | int | historyIndex = -1 | ) | const |
- Returns
- Location state given by historyIndex. If historyIndex is smaller than 0, the state of the current location is returned.
- Since
- 4.5
Definition at line 842 of file kurlnavigator.cpp.
KUrl KUrlNavigator::locationUrl | ( | int | historyIndex = -1 | ) | const |
- Returns
- URL of the location given by the historyIndex. If historyIndex is smaller than 0, the URL of the current location is returned.
- Since
- 4.5
Definition at line 831 of file kurlnavigator.cpp.
|
slot |
Activates the URL navigator (KUrlNavigator::isActive() will return true) and emits the signal KUrlNavigator::activated().
- See also
- KUrlNavigator::setActive()
Definition at line 1071 of file kurlnavigator.cpp.
|
signal |
This signal is emitted when the Return or Enter key is pressed.
void KUrlNavigator::saveLocationState | ( | const QByteArray & | state | ) |
Saves the location state described by state for the current location.
It is recommended that at least the scroll position of a view is remembered and restored when traversing through the history. Saving the location state should be done when the signal KUrlNavigator::urlAboutToBeChanged() has been emitted. Restoring the location state (see KUrlNavigator::locationState()) should be done when the signal KUrlNavigator::urlChanged() has been emitted.
Example:
- Since
- 4.5
Definition at line 837 of file kurlnavigator.cpp.
void KUrlNavigator::setActive | ( | bool | active | ) |
Set the URL navigator to the active mode, if active is true.
The active mode is default. The inactive mode only differs visually from the active mode, no change of the behavior is given.
Using the URL navigator in the inactive mode is useful when having split views, where the inactive view is indicated by an inactive URL navigator visually.
Definition at line 940 of file kurlnavigator.cpp.
void KUrlNavigator::setCustomProtocols | ( | const QStringList & | protocols | ) |
If an application supports only some special protocols, they can be set with protocols .
Definition at line 1195 of file kurlnavigator.cpp.
void KUrlNavigator::setHomeUrl | ( | const KUrl & | url | ) |
Sets the home URL used by KUrlNavigator::goHome().
If no home URL is set, the default home path of the user is used.
- Since
- 4.5
Definition at line 904 of file kurlnavigator.cpp.
|
slot |
Sets the location to url.
The old URL is added to the history. The signals KUrlNavigator::urlAboutToBeChanged(), KUrlNavigator::urlChanged() and KUrlNavigator::historyChanged() are emitted. Use KUrlNavigator::locationUrl() to read the location.
- Since
- 4.5
Definition at line 995 of file kurlnavigator.cpp.
void KUrlNavigator::setPlacesSelectorVisible | ( | bool | visible | ) |
Sets the places selector visible, if visible is true.
The places selector allows to select the places provided by the places model passed in the constructor. Per default the places selector is visible.
Definition at line 962 of file kurlnavigator.cpp.
void KUrlNavigator::setShowFullPath | ( | bool | show | ) |
Shows the full path of the URL even if a place represents a part of the URL.
Assuming that a place called "Pictures" uses the URL /home/user/Pictures. An URL like /home/user/Pictures/2008 is shown as [Pictures] > [2008] in the breadcrumb view, if showing the full path is turned off. If showing the full path is turned on, the URL is shown as [/] > [home] > [Pictures] > [2008].
- Since
- 4.2
Definition at line 926 of file kurlnavigator.cpp.
void KUrlNavigator::setUrlEditable | ( | bool | editable | ) |
Allows to edit the URL of the navigation bar if editable is true, and sets the focus accordingly.
If editable is false, each part of the URL is presented by a button for a fast navigation ("breadcrumb view").
Definition at line 914 of file kurlnavigator.cpp.
bool KUrlNavigator::showFullPath | ( | ) | const |
- Returns
- True, if the full path of the URL should be shown in the breadcrumb view.
- Since
- 4.2
Definition at line 934 of file kurlnavigator.cpp.
|
signal |
Is emitted if the URL url should be opened in a new tab because the user clicked on a breadcrumb with the middle mouse button.
- Since
- 4.5
KUrl KUrlNavigator::uncommittedUrl | ( | ) | const |
- Returns
- The currently entered, but not accepted URL. It is possible that the returned URL is not valid.
Definition at line 983 of file kurlnavigator.cpp.
|
signal |
Is emitted, before the location URL is going to be changed to newUrl.
The signal KUrlNavigator::urlChanged() will be emitted after the change has been done. Connecting to this signal is useful to save the state of a view with KUrlNavigator::saveLocationState().
- Since
- 4.5
|
signal |
Is emitted, if the location URL has been changed e.
g. by the user.
- See also
- KUrlNavigator::setUrl()
|
signal |
Is emitted if a dropping has been done above the destination destination.
The receiver must accept the drop event if the dropped data can be handled.
- Since
- 4.2
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.