KIO
#include <dataslave.h>
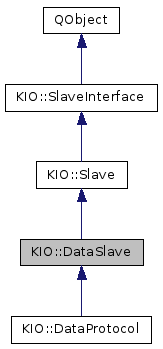
Classes | |
struct | QueueStruct |
Public Member Functions | |
DataSlave () | |
virtual | ~DataSlave () |
virtual void | get (const KUrl &url)=0 |
virtual void | hold (const KUrl &url) |
virtual void | mimetype (const KUrl &url)=0 |
virtual void | resume () |
virtual void | send (int cmd, const QByteArray &arr=QByteArray()) |
virtual void | setConfig (const MetaData &config) |
virtual void | setHost (const QString &host, quint16 port, const QString &user, const QString &passwd) |
virtual void | suspend () |
virtual bool | suspended () |
![]() | |
Slave (const QString &protocol, QObject *parent=0) | |
virtual | ~Slave () |
void | deref () |
QString | host () |
time_t | idleTime () |
bool | isAlive () |
bool | isConnected () |
KIO::SimpleJob * | job () const |
void | kill () |
QString | passwd () |
quint16 | port () |
QString | protocol () |
void | ref () |
void | resetHost () |
void | setConnected (bool c) |
void | setIdle () |
void | setJob (KIO::SimpleJob *job) |
void | setPID (pid_t) |
void | setProtocol (const QString &protocol) |
int | slave_pid () |
QString | slaveProtocol () |
QString | user () |
![]() | |
virtual | ~SlaveInterface () |
Connection * | connection () const |
KIO::filesize_t | offset () const |
void | sendMessageBoxAnswer (int result) |
void | sendResumeAnswer (bool resume) |
void | setConnection (Connection *connection) |
void | setOffset (KIO::filesize_t offset) |
void | setWindow (QWidget *window) |
QWidget * | window () const |
Protected Types | |
typedef QList< QueueStruct > | DispatchQueue |
enum | QueueType { Queue_mimeType = 1, Queue_totalSize, Queue_sendMetaData, Queue_data, Queue_finished } |
Protected Slots | |
void | dispatchNext () |
![]() | |
void | calcSpeed () |
Protected Member Functions | |
void | sendMetaData () |
void | setAllMetaData (const MetaData &) |
![]() | |
SlaveInterface (SlaveInterfacePrivate &dd, QObject *parent=0) | |
virtual bool | dispatch () |
virtual bool | dispatch (int _cmd, const QByteArray &data) |
void | dropNetwork (const QString &, const QString &) |
void | messageBox (int type, const QString &text, const QString &caption, const QString &buttonYes, const QString &buttonNo) |
void | messageBox (int type, const QString &text, const QString &caption, const QString &buttonYes, const QString &buttonNo, const QString &dontAskAgainName) |
void | requestNetwork (const QString &, const QString &) |
Protected Attributes | |
DispatchQueue | dispatchQueue |
![]() | |
SlaveInterfacePrivate *const | d_ptr |
Additional Inherited Members | |
![]() | |
void | accept () |
void | gotInput () |
void | timeout () |
![]() | |
void | slaveDied (KIO::Slave *slave) |
![]() | |
void | canResume (KIO::filesize_t) |
void | connected () |
void | data (const QByteArray &) |
void | dataReq () |
void | error (int, const QString &) |
void | errorPage () |
void | finished () |
void | infoMessage (const QString &) |
void | listEntries (const KIO::UDSEntryList &) |
void | metaData (const KIO::MetaData &) |
void | mimeType (const QString &) |
void | needSubUrlData () |
void | open () |
void | position (KIO::filesize_t) |
void | processedSize (KIO::filesize_t) |
void | redirection (const KUrl &) |
void | slaveStatus (pid_t, const QByteArray &, const QString &, bool) |
void | speed (unsigned long) |
void | statEntry (const KIO::UDSEntry &) |
void | totalSize (KIO::filesize_t) |
void | warning (const QString &) |
void | written (KIO::filesize_t) |
![]() | |
static bool | checkForHeldSlave (const KUrl &url) |
static Slave * | createSlave (const QString &protocol, const KUrl &url, int &error, QString &error_text) |
static Slave * | holdSlave (const QString &protocol, const KUrl &url) |
Detailed Description
This class provides a high performance implementation for the data url scheme (rfc2397).
Do not use this class in external applications. It is an implementation detail of KIO and subject to change without notice.
Definition at line 49 of file dataslave.h.
Member Typedef Documentation
|
protected |
Definition at line 99 of file dataslave.h.
Member Enumeration Documentation
|
protected |
identifiers of functions to be queued
Enumerator | |
---|---|
Queue_mimeType | |
Queue_totalSize | |
Queue_sendMetaData | |
Queue_data | |
Queue_finished |
Definition at line 85 of file dataslave.h.
Constructor & Destructor Documentation
DataSlave::DataSlave | ( | ) |
Definition at line 60 of file dataslave.cpp.
|
virtual |
Definition at line 69 of file dataslave.cpp.
Member Function Documentation
|
protectedslot |
dispatches next queued method.
Does nothing if there are no queued methods.
Definition at line 101 of file dataslave.cpp.
|
pure virtual |
Implemented in KIO::DataProtocol.
|
virtual |
Puts the kioslave associated with url
at halt, and return it to klauncher, in order to let another application connect to it and finish the job.
This is for the krunner case: type a URL in krunner, it will start downloading to find the mimetype (KRun), and then hold the slave, publish the held slave using, this method, and the final application can continue the same download by requesting the same URL.
Reimplemented from KIO::Slave.
Definition at line 73 of file dataslave.cpp.
|
pure virtual |
Implemented in KIO::DataProtocol.
|
virtual |
Resumes the operation of the attached kioslave.
Reimplemented from KIO::Slave.
Definition at line 83 of file dataslave.cpp.
|
virtual |
Sends the given command to the kioslave.
- Parameters
-
cmd command id arr byte array containing data
Reimplemented from KIO::Slave.
Definition at line 120 of file dataslave.cpp.
|
protected |
Sends metadata set with setAllMetaData.
Definition at line 170 of file dataslave.cpp.
|
protected |
Sets metadata.
Definition at line 166 of file dataslave.cpp.
|
virtual |
|
virtual |
Set host for url.
- Parameters
-
host to connect to. port to connect to. user to login as passwd to login with
Reimplemented from KIO::Slave.
Definition at line 151 of file dataslave.cpp.
|
virtual |
Suspends the operation of the attached kioslave.
Reimplemented from KIO::Slave.
Definition at line 77 of file dataslave.cpp.
|
virtual |
Tells whether the kioslave is suspended.
- Returns
- true if the kioslave is suspended.
Reimplemented from KIO::Slave.
Definition at line 147 of file dataslave.cpp.
Member Data Documentation
|
protected |
Definition at line 100 of file dataslave.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.