KIO
#include <fileundomanager.h>
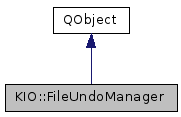
Classes | |
class | UiInterface |
Public Types | |
enum | CommandType { Copy, Move, Rename, Link, Mkdir, Trash, Put } |
Public Slots | |
void | undo () |
Signals | |
void | jobRecordingFinished (CommandType op) |
void | jobRecordingStarted (CommandType op) |
void | undoAvailable (bool avail) |
void | undoJobFinished () |
void | undoTextChanged (const QString &text) |
Public Member Functions | |
quint64 | currentCommandSerialNumber () const |
quint64 | newCommandSerialNumber () |
void | recordCopyJob (KIO::CopyJob *copyJob) |
void | recordJob (CommandType op, const KUrl::List &src, const KUrl &dst, KIO::Job *job) |
void | setUiInterface (UiInterface *ui) |
UiInterface * | uiInterface () const |
bool | undoAvailable () const |
QString | undoText () const |
Static Public Member Functions | |
static FileUndoManager * | self () |
Detailed Description
FileUndoManager: makes it possible to undo kio jobs.
This class is a singleton, use self() to access its only instance.
Definition at line 44 of file fileundomanager.h.
Member Enumeration Documentation
The type of job.
Put:
- Since
- 4.7, represents the creation of a file from data in memory. Used when pasting data from clipboard or drag-n-drop.
Enumerator | |
---|---|
Copy | |
Move | |
Rename | |
Link | |
Mkdir | |
Trash | |
Put |
Definition at line 135 of file fileundomanager.h.
Member Function Documentation
quint64 FileUndoManager::currentCommandSerialNumber | ( | ) | const |
Definition at line 314 of file fileundomanager.cpp.
|
signal |
Emitted when a job that has been recorded by FileUndoManager::recordJob() or FileUndoManager::recordCopyJob has been finished.
The command is now available for an undo-operation.
- Since
- 4.2
|
signal |
Emitted when a job recording has been started by FileUndoManager::recordJob() or FileUndoManager::recordCopyJob().
After the job recording has been finished, the signal jobRecordingFinished() will be emitted.
- Since
- 4.2
quint64 FileUndoManager::newCommandSerialNumber | ( | ) |
These two functions are useful when wrapping FileUndoManager and adding custom commands.
Each command has a unique ID. You can get a new serial number for a custom command with newCommandSerialNumber(), and then when you want to undo, check if the command FileUndoManager would undo is newer or older than your custom command.
Definition at line 309 of file fileundomanager.cpp.
void FileUndoManager::recordCopyJob | ( | KIO::CopyJob * | copyJob | ) |
Record this CopyJob while it's happening and add a command for it so that the user can undo it.
The signal jobRecordingStarted() is emitted.
Definition at line 254 of file fileundomanager.cpp.
void FileUndoManager::recordJob | ( | CommandType | op, |
const KUrl::List & | src, | ||
const KUrl & | dst, | ||
KIO::Job * | job | ||
) |
Record this job while it's happening and add a command for it so that the user can undo it.
The signal jobRecordingStarted() is emitted.
- Parameters
-
op the type of job - which is also the type of command that will be created for it src list of source urls dst destination url job the job to record
Definition at line 247 of file fileundomanager.cpp.
|
static |
- Returns
- the FileUndoManager instance
Definition at line 208 of file fileundomanager.cpp.
void FileUndoManager::setUiInterface | ( | UiInterface * | ui | ) |
Set a new UiInterface implementation.
This deletes the previous one.
- Parameters
-
ui the UiInterface instance, which becomes owned by the undo manager.
Definition at line 732 of file fileundomanager.cpp.
FileUndoManager::UiInterface * FileUndoManager::uiInterface | ( | ) | const |
- Returns
- the UiInterface instance passed to setUiInterface. This is useful for calling setParentWidget on it. Never delete it!
Definition at line 738 of file fileundomanager.cpp.
|
slot |
Undoes the last command Remember to call uiInterface()->setParentWidget(parentWidget) first, if you have multiple mainwindows.
Definition at line 325 of file fileundomanager.cpp.
bool FileUndoManager::undoAvailable | ( | ) | const |
- Returns
- true if undo is possible. Usually used for enabling/disabling the undo action.
Definition at line 278 of file fileundomanager.cpp.
|
signal |
Emitted when the value of undoAvailable() changes.
|
signal |
Emitted when an undo job finishes. Used for unit testing.
QString FileUndoManager::undoText | ( | ) | const |
- Returns
- the current text for the undo action.
Definition at line 283 of file fileundomanager.cpp.
|
signal |
Emitted when the value of undoText() changes.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.