KIO
#include <jobclasses.h>
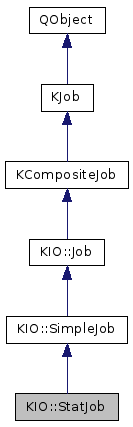
Public Types | |
enum | StatSide { SourceSide, DestinationSide } |
![]() | |
enum | Capability |
enum | KillVerbosity |
enum | Unit |
Signals | |
void | permanentRedirection (KIO::Job *job, const KUrl &fromUrl, const KUrl &toUrl) |
void | redirection (KIO::Job *job, const KUrl &url) |
![]() | |
void | canceled (KJob *job) |
void | connected (KIO::Job *job) |
![]() | |
void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1=qMakePair(QString(), QString()), const QPair< QString, QString > &field2=qMakePair(QString(), QString())) |
void | finished (KJob *job) |
void | infoMessage (KJob *job, const QString &plain, const QString &rich=QString()) |
void | percent (KJob *job, unsigned long percent) |
void | processedAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
void | processedSize (KJob *job, qulonglong size) |
void | result (KJob *job) |
void | resumed (KJob *job) |
void | speed (KJob *job, unsigned long speed) |
void | suspended (KJob *job) |
void | totalAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
void | totalSize (KJob *job, qulonglong size) |
void | warning (KJob *job, const QString &plain, const QString &rich=QString()) |
Public Member Functions | |
~StatJob () | |
KUrl | mostLocalUrl () const |
void | setDetails (short int details) |
void | setSide (StatSide side) |
void | setSide (bool source) |
const UDSEntry & | statResult () const |
![]() | |
~SimpleJob () | |
bool | isRedirectionHandlingEnabled () const |
virtual void | putOnHold () |
void | setRedirectionHandlingEnabled (bool handle) |
const KUrl & | url () const |
![]() | |
virtual | ~Job () |
void | addMetaData (const QString &key, const QString &value) |
void | addMetaData (const QMap< QString, QString > &values) |
QStringList | detailedErrorStrings (const KUrl *reqUrl=0L, int method=-1) const |
QString | errorString () const |
bool | isInteractive () const |
void | mergeMetaData (const QMap< QString, QString > &values) |
MetaData | metaData () const |
MetaData | outgoingMetaData () const |
Job * | parentJob () const |
QString | queryMetaData (const QString &key) |
void | setMetaData (const KIO::MetaData &metaData) |
void | setParentJob (Job *parentJob) |
void | showErrorDialog (QWidget *parent=0) |
void | start () |
JobUiDelegate * | ui () const |
![]() | |
KCompositeJob (QObject *parent=0) | |
virtual | ~KCompositeJob () |
virtual | ~KJob () |
Capabilities | capabilities () const |
int | error () const |
QString | errorText () const |
bool | exec () |
bool | isAutoDelete () const |
bool | isSuspended () const |
KJob (QObject *parent=0) | |
unsigned long | percent () const |
qulonglong | processedAmount (Unit unit) const |
void | setAutoDelete (bool autodelete) |
void | setUiDelegate (KJobUiDelegate *delegate) |
qulonglong | totalAmount (Unit unit) const |
KJobUiDelegate * | uiDelegate () const |
Protected Slots | |
virtual void | slotFinished () |
virtual void | slotMetaData (const KIO::MetaData &_metaData) |
![]() | |
virtual void | slotFinished () |
virtual void | slotMetaData (const KIO::MetaData &_metaData) |
virtual void | slotWarning (const QString &) |
![]() | |
virtual void | slotInfoMessage (KJob *job, const QString &plain, const QString &rich) |
virtual void | slotResult (KJob *job) |
Protected Member Functions | |
StatJob (StatJobPrivate &dd) | |
![]() | |
SimpleJob (SimpleJobPrivate &dd) | |
virtual bool | doKill () |
virtual bool | doResume () |
virtual bool | doSuspend () |
void | storeSSLSessionFromJob (const KUrl &m_redirectionURL) |
![]() | |
Job () | |
Job (JobPrivate &dd) | |
virtual bool | addSubjob (KJob *job) |
virtual bool | removeSubjob (KJob *job) |
![]() | |
KCompositeJob (KCompositeJobPrivate &dd, QObject *parent) | |
void | clearSubjobs () |
void | emitPercent (qulonglong processedAmount, qulonglong totalAmount) |
void | emitResult () |
void | emitSpeed (unsigned long speed) |
bool | hasSubjobs () |
KJob (KJobPrivate &dd, QObject *parent) | |
void | setCapabilities (Capabilities capabilities) |
void | setError (int errorCode) |
void | setErrorText (const QString &errorText) |
void | setPercent (unsigned long percentage) |
void | setProcessedAmount (Unit unit, qulonglong amount) |
void | setTotalAmount (Unit unit, qulonglong amount) |
const QList< KJob * > & | subjobs () const |
Additional Inherited Members | |
![]() | |
void | slotError (int, const QString &) |
![]() | |
bool | kill (KillVerbosity verbosity=Quietly) |
bool | resume () |
bool | suspend () |
![]() | |
static void | removeOnHold () |
![]() | |
KJobPrivate *const | d_ptr |
Detailed Description
A KIO job that retrieves information about a file or directory.
- See also
- KIO::stat()
Definition at line 440 of file jobclasses.h.
Member Enumeration Documentation
Enumerator | |
---|---|
SourceSide | |
DestinationSide |
Definition at line 445 of file jobclasses.h.
Constructor & Destructor Documentation
Member Function Documentation
KUrl StatJob::mostLocalUrl | ( | ) | const |
most local URL Call this in the slot connected to result, and only after making sure no error happened.
- Returns
- the most local URL for the URL we were stat'ing.
Sample usage: KIO::StatJob* job = KIO::mostLocalUrl("desktop:/foo"); job->ui()->setWindow(this); connect(job, SIGNAL(result(KJob*)), this, SLOT(slotMostLocalUrlResult(KJob*))); [...] // and in the slot if (job->error()) { [...] // doesn't exist } else { const KUrl localUrl = job->mostLocalUrl(); // localUrl = file:///$HOME/Desktop/foo [...] }
- Since
- 4.4
|
signal |
Signals a permanent redirection.
The redirection itself is handled internally.
- Parameters
-
job the job that is redirected fromUrl the original URL toUrl the new URL
Signals a redirection.
Use to update the URL shown to the user. The redirection itself is handled internally.
- Parameters
-
job the job that is redirected url the new url
void StatJob::setDetails | ( | short int | details | ) |
Selects the level of details
we want.
By default this is 2 (all details wanted, including modification time, size, etc.), setDetails(1) is used when deleting: we don't need all the information if it takes too much time, no need to follow symlinks etc. setDetails(0) is used for very simple probing: we'll only get the answer "it's a file or a directory, or it doesn't exist". This is used by KRun.
- Parameters
-
details 2 for all details, 1 for simple, 0 for very simple
void StatJob::setSide | ( | StatSide | side | ) |
void StatJob::setSide | ( | bool | source | ) |
A stat() can have two meanings.
Either we want to read from this URL, or to check if we can write to it. First case is "source", second is "dest". It is necessary to know what the StatJob is for, to tune the kioslave's behavior (e.g. with FTP).
- Parameters
-
source true for "source" mode, false for "dest" mode
|
protectedvirtualslot |
const UDSEntry & StatJob::statResult | ( | ) | const |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:05 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.