Plasma
#include <Plasma/GLApplet>
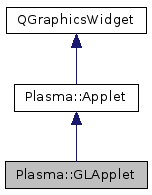
Public Member Functions | |
GLApplet (QGraphicsItem *parent, const QString &serviceId, int appletId) | |
GLApplet (QObject *parent, const QVariantList &args) | |
~GLApplet () | |
GLuint | bindTexture (const QImage &image, GLenum target=GL_TEXTURE_2D) |
void | deleteTexture (GLuint texture_id) |
void | makeCurrent () |
virtual void | paintGLInterface (QPainter *painter, const QStyleOptionGraphicsItem *option) |
![]() | |
Applet (QGraphicsItem *parent=0, const QString &serviceId=QString(), uint appletId=0) | |
Applet (const KPluginInfo &info, QGraphicsItem *parent=0, uint appletId=0) | |
Applet (QGraphicsItem *parent, const QString &serviceId, uint appletId, const QVariantList &args) | |
~Applet () | |
Q_INVOKABLE QAction * | action (QString name) const |
void | addAction (QString name, QAction *action) |
virtual void | addAssociatedWidget (QWidget *widget) |
Plasma::AspectRatioMode | aspectRatioMode () const |
QString | associatedApplication () const |
KUrl::List | associatedApplicationUrls () const |
BackgroundHints | backgroundHints () const |
QString | category () const |
KConfigGroup | config () const |
KConfigGroup | config (const QString &group) const |
ConfigLoader * | configScheme () const |
bool | configurationRequired () const |
Containment * | containment () const |
Context * | context () const |
virtual QList< QAction * > | contextualActions () |
virtual void | createConfigurationInterface (KConfigDialog *parent) |
QStringList | customCategories () |
Q_INVOKABLE DataEngine * | dataEngine (const QString &name) const |
bool | destroyed () const |
QFont | font () const |
virtual FormFactor | formFactor () const |
KConfigGroup | globalConfig () const |
KShortcut | globalShortcut () const |
bool | hasAuthorization (const QString &constraint) const |
bool | hasConfigurationInterface () const |
bool | hasFailedToLaunch () const |
bool | hasValidAssociatedApplication () const |
QString | icon () const |
uint | id () const |
ImmutabilityType | immutability () const |
virtual void | initExtenderItem (ExtenderItem *item) |
bool | isBusy () const |
bool | isContainment () const |
virtual bool | isPopupShowing () const |
virtual Location | location () const |
QRectF | mapFromView (const QGraphicsView *view, const QRect &rect) const |
QRect | mapToView (const QGraphicsView *view, const QRectF &rect) const |
QString | name () const |
const Package * | package () const |
void | paintWindowFrame (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) |
QString | pluginName () const |
QPoint | popupPosition (const QSize &s) const |
QPoint | popupPosition (const QSize &s, Qt::AlignmentFlag alignment) const |
virtual void | removeAssociatedWidget (QWidget *widget) |
virtual void | restore (KConfigGroup &group) |
virtual void | save (KConfigGroup &group) const |
QRect | screenRect () const |
void | setAspectRatioMode (Plasma::AspectRatioMode) |
void | setAssociatedApplication (const QString &string) |
void | setAssociatedApplicationUrls (const KUrl::List &urls) |
void | setBackgroundHints (const BackgroundHints hints) |
void | setCustomCategories (const QStringList &categories) |
void | setGlobalShortcut (const KShortcut &shortcut) |
bool | shouldConserveResources () const |
int | type () const |
void | updateConstraints (Plasma::Constraints constraints=Plasma::AllConstraints) |
QGraphicsView * | view () const |
Additional Inherited Members | |
![]() | |
enum | { Type = Plasma::AppletType } |
enum | BackgroundHint { NoBackground = 0, StandardBackground = 1, TranslucentBackground = 2, DefaultBackground = StandardBackground } |
typedef QHash< QString, Applet * > | Dict |
typedef QList< Applet * > | List |
![]() | |
virtual void | configChanged () |
virtual void | destroy () |
void | flushPendingConstraintsEvents () |
virtual void | init () |
bool | isPublished () const |
bool | isUserConfiguring () const |
void | lower () |
void | publish (Plasma::AnnouncementMethods methods, const QString &resourceName) |
void | raise () |
void | runAssociatedApplication () |
void | setBusy (bool busy) |
void | setImmutability (const ImmutabilityType immutable) |
void | setStatus (const ItemStatus stat) |
virtual void | showConfigurationInterface () |
void | showConfigurationInterface (QWidget *widget) |
QVariantList | startupArguments () const |
ItemStatus | status () const |
void | unpublish () |
![]() | |
void | activate () |
void | appletDestroyed (Plasma::Applet *applet) |
void | appletTransformedByUser () |
void | appletTransformedItself () |
void | configNeedsSaving () |
void | extenderItemRestored (Plasma::ExtenderItem *item) |
void | geometryChanged () |
void | immutabilityChanged (Plasma::ImmutabilityType immutable) |
void | messageButtonPressed (const Plasma::MessageButton button) |
void | newStatus (Plasma::ItemStatus status) |
void | releaseVisualFocus () |
void | sizeHintChanged (Qt::SizeHint which) |
![]() | |
static QString | category (const KPluginInfo &applet) |
static QString | category (const QString &appletName) |
static KPluginInfo::List | listAppletInfo (const QString &category=QString(), const QString &parentApp=QString()) |
static KPluginInfo::List | listAppletInfoForMimetype (const QString &mimetype) |
static KPluginInfo::List | listAppletInfoForUrl (const QUrl &url) |
static QStringList | listCategories (const QString &parentApp=QString(), bool visibleOnly=true) |
static Applet * | load (const QString &name, uint appletId=0, const QVariantList &args=QVariantList()) |
static Applet * | load (const KPluginInfo &info, uint appletId=0, const QVariantList &args=QVariantList()) |
static Applet * | loadPlasmoid (const QString &path, uint appletId=0, const QVariantList &args=QVariantList()) |
static PackageStructure::Ptr | packageStructure () |
![]() | |
Applet (QObject *parent, const QVariantList &args) | |
virtual void | constraintsEvent (Plasma::Constraints constraints) |
bool | eventFilter (QObject *o, QEvent *e) |
Extender * | extender () const |
void | focusInEvent (QFocusEvent *event) |
void | hoverEnterEvent (QGraphicsSceneHoverEvent *event) |
void | hoverLeaveEvent (QGraphicsSceneHoverEvent *event) |
bool | isRegisteredAsDragHandle (QGraphicsItem *item) |
QVariant | itemChange (GraphicsItemChange change, const QVariant &value) |
void | mouseMoveEvent (QGraphicsSceneMouseEvent *event) |
void | registerAsDragHandle (QGraphicsItem *item) |
void | resizeEvent (QGraphicsSceneResizeEvent *event) |
virtual void | saveState (KConfigGroup &config) const |
bool | sceneEventFilter (QGraphicsItem *watched, QEvent *event) |
void | setConfigurationRequired (bool needsConfiguring, const QString &reason=QString()) |
void | setFailedToLaunch (bool failed, const QString &reason=QString()) |
void | setHasConfigurationInterface (bool hasInterface) |
QPainterPath | shape () const |
void | showMessage (const QIcon &icon, const QString &message, const Plasma::MessageButtons buttons) |
QSizeF | sizeHint (Qt::SizeHint which, const QSizeF &constraint=QSizeF()) const |
void | timerEvent (QTimerEvent *event) |
void | unregisterAsDragHandle (QGraphicsItem *item) |
![]() | |
BackgroundHints | backgroundHints |
bool | busy |
QString | category |
bool | configurationRequired |
QRectF | geometry |
bool | hasConfigurationInterface |
bool | hasFailedToLaunch |
uint | id |
ImmutabilityType | immutability |
bool | isBusy |
QString | name |
QString | pluginName |
bool | shouldConserveResources |
bool | userConfiguring |
Detailed Description
Plasma Applet that is fully rendered using OpengGL.
Definition at line 37 of file glapplet.h.
Constructor & Destructor Documentation
Plasma::GLApplet::GLApplet | ( | QGraphicsItem * | parent, |
const QString & | serviceId, | ||
int | appletId | ||
) |
- Parameters
-
parent the QGraphicsItem this applet is parented to serviceId the name of the .desktop file containing the information about the widget appletId a unique id used to differentiate between multiple instances of the same Applet type
Definition at line 67 of file glapplet.cpp.
Plasma::GLApplet::GLApplet | ( | QObject * | parent, |
const QVariantList & | args | ||
) |
This constructor is to be used with the plugin loading systems found in KPluginInfo and KService.
The argument list is expected to have two elements: the KService service ID for the desktop entry and an applet ID which must be a base 10 number.
- Parameters
-
parent a QObject parent; you probably want to pass in 0 args a list of strings containing two entries: the service id and the applet id
Definition at line 80 of file glapplet.cpp.
Plasma::GLApplet::~GLApplet | ( | ) |
Definition at line 91 of file glapplet.cpp.
Member Function Documentation
GLuint Plasma::GLApplet::bindTexture | ( | const QImage & | image, |
GLenum | target = GL_TEXTURE_2D |
||
) |
Definition at line 96 of file glapplet.cpp.
void Plasma::GLApplet::deleteTexture | ( | GLuint | texture_id | ) |
Definition at line 105 of file glapplet.cpp.
void Plasma::GLApplet::makeCurrent | ( | ) |
Definition at line 204 of file glapplet.cpp.
|
virtual |
Reimplement this method to render using OpenGL.
QPainter passed to this method will always use OpenGL engine and rendering using OpenGL api directly is supported.
Definition at line 111 of file glapplet.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.