kopete/libkopete
#include <kopetepluginmanager.h>
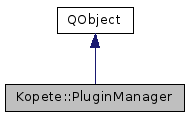
Public Types | |
enum | PluginLoadMode { LoadSync, LoadAsync } |
Public Slots | |
void | loadAllPlugins () |
Plugin * | loadPlugin (const QString &pluginId, PluginLoadMode mode=LoadSync) |
bool | unloadPlugin (const QString &pluginName) |
Signals | |
void | allPluginsLoaded () |
void | pluginLoaded (Kopete::Plugin *plugin) |
void | pluginUnloaded (const QString &pluginName) |
void | protocolLoaded (Kopete::Protocol *protocol) |
Public Member Functions | |
QList< KPluginInfo > | availablePlugins (const QString &category=QString()) const |
bool | isAllPluginsLoaded () const |
PluginList | loadedPlugins (const QString &category=QString()) const |
Plugin * | plugin (const QString &pluginName) const |
KPluginInfo | pluginInfo (const Kopete::Plugin *plugin) const |
bool | setPluginEnabled (const QString &name, bool enabled=true) |
void | shutdown () |
Static Public Member Functions | |
static PluginManager * | self () |
Detailed Description
Definition at line 40 of file kopetepluginmanager.h.
Member Enumeration Documentation
Plugin loading mode.
Used by loadPlugin(). Code that doesn't want to block the GUI and/or lot a lot of plugins at once should use asynchronous loading (LoadAsync
). The default is synchronous loading (LoadSync
).
Enumerator | |
---|---|
LoadSync | |
LoadAsync |
Definition at line 129 of file kopetepluginmanager.h.
Member Function Documentation
|
signal |
All plugins have been loaded by the plugin manager.
This signal is emitted exactly ONCE, when the plugin manager has emptied its plugin queue for the first time. This means that if you call an async loadPlugin() before loadAllPlugins() this signal is probably emitted after the initial call completes, unless you are quick enough to fill the queue before it completes, which is a dangerous race you shouldn't count upon :)
The signal is delayed one event loop iteration through a singleShot timer, but that is not guaranteed to be enough for account instantiation. You may need an additional timer for it in the code if you want to programmatically act on it.
If you use the signal for enabling/disabling GUI objects there is little chance a user is able to activate them in the short while that's remaining, the slow part of the code is over now and the remaining processing time is neglectable for the user.
QList< KPluginInfo > Kopete::PluginManager::availablePlugins | ( | const QString & | category = QString() | ) | const |
Returns a list of all available plugins for the given category.
Currently there are two categories, "Plugins" and "Protocols", but you can add your own categories if you want.
If you pass an empty string you get the complete list of ALL plugins.
You can query all information on the plugins through the KPluginInfo interface.
Definition at line 122 of file kopetepluginmanager.cpp.
bool Kopete::PluginManager::isAllPluginsLoaded | ( | ) | const |
This method check if all the plugins are loaded.
- Returns
- true if all the plugins are loaded.
Definition at line 500 of file kopetepluginmanager.cpp.
|
slot |
Loads all the enabled plugins.
Also used to reread the config file when the configuration has changed.
Definition at line 258 of file kopetepluginmanager.cpp.
PluginList Kopete::PluginManager::loadedPlugins | ( | const QString & | category = QString() | ) | const |
Returns a list of all plugins that are actually loaded.
If you omit the category you get all, otherwise it's a filtered list. See also availablePlugins().
Definition at line 138 of file kopetepluginmanager.cpp.
|
slot |
Load a single plugin by plugin name.
Returns an existing plugin if one is already loaded in memory.
If mode is set to Async, the plugin will be queued and loaded in the background. This method will return a null pointer. To get the loaded plugin you can track the pluginLoaded() signal.
See also plugin().
Definition at line 340 of file kopetepluginmanager.cpp.
Plugin * Kopete::PluginManager::plugin | ( | const QString & | pluginName | ) | const |
Search by plugin name.
This is the key used as X-KDE-PluginInfo-Name in the .desktop file, e.g. "kopete_jabber"
- Returns
- The Kopete::Plugin object found by the search, or a null pointer if the plugin is not loaded.
If you want to also load the plugin you can better use loadPlugin, which returns the pointer to the plugin if it's already loaded.
Definition at line 444 of file kopetepluginmanager.cpp.
KPluginInfo Kopete::PluginManager::pluginInfo | ( | const Kopete::Plugin * | plugin | ) | const |
- Returns
- the KPluginInfo for the specified plugin
Definition at line 153 of file kopetepluginmanager.cpp.
|
signal |
Signals a new plugin has just been loaded.
|
signal |
Signals a plugin has just been unloaded.
|
signal |
Signals a new protocol has just been loaded.
- Note
- pluginLoaded is also emitted before this signal
|
static |
Retrieve the plugin loader instance.
Definition at line 104 of file kopetepluginmanager.cpp.
bool Kopete::PluginManager::setPluginEnabled | ( | const QString & | name, |
bool | enabled = true |
||
) |
Enable a plugin.
This marks a plugin as enabled in the config file, so loadAll() can pick it up later.
This method does not actually load a plugin, it only edits the config file.
- Parameters
-
name is the name of the plugin as it is listed in the .desktop file in the X-KDE-Library field. enabled sets whether or not the plugin is enabled
Returns false when no appropriate plugin can be found.
Definition at line 481 of file kopetepluginmanager.cpp.
void Kopete::PluginManager::shutdown | ( | ) |
Shuts down the plugin manager on Kopete shutdown, but first unloads all plugins asynchronously.
After 3 seconds all plugins should be removed; what's still left by then is unloaded through a hard delete instead.
Note that this call also derefs the plugin manager from the event loop, so do NOT call this method when not terminating Kopete!
Definition at line 164 of file kopetepluginmanager.cpp.
|
slot |
Unload the plugin specified by pluginName
.
Definition at line 405 of file kopetepluginmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.