akregator
#include <folder.h>
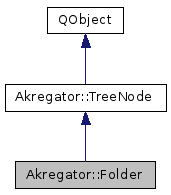
Public Slots | |
void | slotAddToFetchQueue (Akregator::FetchQueue *queue, bool intervalFetchesOnly=false) |
void | slotChildChanged (Akregator::TreeNode *node) |
void | slotChildDestroyed (Akregator::TreeNode *node) |
![]() | |
virtual void | slotAddToFetchQueue (Akregator::FetchQueue *queue, bool intervalFetchesOnly=false)=0 |
Signals | |
void | signalAboutToRemoveChild (Akregator::TreeNode *) |
void | signalChildAdded (Akregator::TreeNode *) |
void | signalChildRemoved (Akregator::Folder *, Akregator::TreeNode *) |
![]() | |
void | signalArticlesAdded (Akregator::TreeNode *, const QList< Akregator::Article > &guids) |
void | signalArticlesRemoved (Akregator::TreeNode *, const QList< Akregator::Article > &guids) |
void | signalArticlesUpdated (Akregator::TreeNode *, const QList< Akregator::Article > &guids) |
void | signalChanged (Akregator::TreeNode *) |
void | signalDestroyed (Akregator::TreeNode *) |
Public Member Functions | |
Folder (const QString &title=QString()) | |
~Folder () | |
bool | accept (TreeNodeVisitor *visitor) |
void | appendChild (TreeNode *node) |
TreeNode * | childAt (int pos) |
const TreeNode * | childAt (int pos) const |
QList< const TreeNode * > | children () const |
QList< TreeNode * > | children () |
KJob * | createMarkAsReadJob () |
QVector< const Feed * > | feeds () const |
QVector< Feed * > | feeds () |
TreeNode * | firstChild () |
const TreeNode * | firstChild () const |
QVector< const Folder * > | folders () const |
QVector< Folder * > | folders () |
QIcon | icon () const |
int | indexOf (const TreeNode *node) const |
void | insertChild (TreeNode *node, TreeNode *after) |
bool | isAggregation () const |
bool | isGroup () const |
bool | isOpen () const |
TreeNode * | lastChild () |
const TreeNode * | lastChild () const |
QList< const TreeNode * > | namedChildren (const QString &title) const |
QList< TreeNode * > | namedChildren (const QString &title) |
TreeNode * | next () |
const TreeNode * | next () const |
void | prependChild (TreeNode *node) |
void | removeChild (TreeNode *node) |
void | setOpen (bool open) |
bool | subtreeContains (const Akregator::TreeNode *node) const |
QDomElement | toOPML (QDomElement parent, QDomDocument document) const |
int | totalCount () const |
int | unread () const |
![]() | |
TreeNode () | |
virtual | ~TreeNode () |
ArticleListJob * | createListJob () |
virtual uint | id () const |
QPoint | listViewScrollBarPositions () const |
virtual const TreeNode * | nextSibling () const |
virtual TreeNode * | nextSibling () |
virtual const Folder * | parent () const |
virtual Folder * | parent () |
virtual const TreeNode * | prevSibling () const |
virtual TreeNode * | prevSibling () |
virtual void | setId (uint id) |
void | setListViewScrollBarPositions (const QPoint &pos) |
virtual void | setNotificationMode (bool doNotify) |
virtual void | setParent (Folder *parent) |
void | setTitle (const QString &title) |
QString | title () const |
Static Public Member Functions | |
static Folder * | fromOPML (const QDomElement &e) |
Protected Member Functions | |
void | doArticleNotification () |
void | insertChild (int index, TreeNode *node) |
![]() | |
virtual void | articlesModified () |
void | emitSignalDestroyed () |
virtual void | nodeModified () |
Detailed Description
Constructor & Destructor Documentation
|
explicit |
Creates a new folder with a given title.
- Parameters
-
title The title of the feed group
Definition at line 91 of file folder.cpp.
Folder::~Folder | ( | ) |
Definition at line 96 of file folder.cpp.
Member Function Documentation
|
virtual |
Implements Akregator::TreeNode.
Definition at line 75 of file folder.cpp.
void Folder::appendChild | ( | TreeNode * | node | ) |
inserts node
as last child
- Parameters
-
node the tree node to insert
Definition at line 215 of file folder.cpp.
|
virtual |
Reimplemented from Akregator::TreeNode.
Definition at line 379 of file folder.cpp.
|
virtual |
Reimplemented from Akregator::TreeNode.
Definition at line 386 of file folder.cpp.
returns the (direct) children of this node.
- Returns
- a list of pointers to the child nodes
Reimplemented from Akregator::TreeNode.
Definition at line 124 of file folder.cpp.
Reimplemented from Akregator::TreeNode.
Definition at line 132 of file folder.cpp.
|
virtual |
Implements Akregator::TreeNode.
Definition at line 317 of file folder.cpp.
|
protectedvirtual |
reimplement this in subclasses to do the actual notification called by articlesModified
Reimplemented from Akregator::TreeNode.
Definition at line 359 of file folder.cpp.
|
virtual |
Implements Akregator::TreeNode.
Definition at line 137 of file folder.cpp.
|
virtual |
Implements Akregator::TreeNode.
Definition at line 146 of file folder.cpp.
TreeNode * Folder::firstChild | ( | ) |
returns the first child of the group, 0 if none exist
Definition at line 266 of file folder.cpp.
const TreeNode * Folder::firstChild | ( | ) | const |
Definition at line 271 of file folder.cpp.
|
virtual |
Implements Akregator::TreeNode.
Definition at line 155 of file folder.cpp.
|
virtual |
Implements Akregator::TreeNode.
Definition at line 165 of file folder.cpp.
|
static |
creates a feed group parsed from a XML dom element.
Child nodes are not inserted or parsed.
- Parameters
-
e the element representing the feed group
- Returns
- a freshly created feed group
Definition at line 83 of file folder.cpp.
|
virtual |
Implements Akregator::TreeNode.
Definition at line 190 of file folder.cpp.
int Folder::indexOf | ( | const TreeNode * | node | ) | const |
Definition at line 175 of file folder.cpp.
inserts node
as child after child node after
.
if after
is not a child of this group, node
will be inserted as first child
- Parameters
-
node the tree node to insert after the node after which node
will be inserted
Definition at line 180 of file folder.cpp.
|
protected |
inserts node
as child on position index
- Parameters
-
index the position where to insert node the tree node to insert
Definition at line 195 of file folder.cpp.
|
inlinevirtual |
returns if the node represents an aggregation, i.e.
containing items from more than once source feed. Folders and virtual folders are aggregations, feeds are not.
Implements Akregator::TreeNode.
|
inlinevirtual |
Helps the rest of the app to decide if node should be handled as group or not.
Implements Akregator::TreeNode.
bool Folder::isOpen | ( | ) | const |
returns whether the feed group is opened or not.
Use only in FolderItem.
Definition at line 286 of file folder.cpp.
TreeNode * Folder::lastChild | ( | ) |
returns the last child of the group, 0 if none exist
Definition at line 276 of file folder.cpp.
const TreeNode * Folder::lastChild | ( | ) | const |
Definition at line 281 of file folder.cpp.
Definition at line 431 of file folder.cpp.
Definition at line 446 of file folder.cpp.
|
virtual |
returns the next node in the tree.
Calling next() unless it returns 0 iterates through the tree in pre-order
Implements Akregator::TreeNode.
Definition at line 393 of file folder.cpp.
|
virtual |
returns the next node in the tree.
Calling next() unless it returns 0 iterates through the tree in pre-order
Implements Akregator::TreeNode.
Definition at line 412 of file folder.cpp.
void Folder::prependChild | ( | TreeNode * | node | ) |
inserts node
as first child
- Parameters
-
node the tree node to insert
Definition at line 232 of file folder.cpp.
void Folder::removeChild | ( | TreeNode * | node | ) |
remove node
from children.
Note that node
will not be deleted
- Parameters
-
node the child node to remove
Definition at line 249 of file folder.cpp.
void Folder::setOpen | ( | bool | open | ) |
open/close the feed group (display it as expanded/collapsed in the tree view).
Use only in FolderItem.
Definition at line 291 of file folder.cpp.
|
signal |
|
signal |
emitted when a child was added
|
signal |
emitted when a child was removed
|
slot |
enqueues children recursively for fetching
- Parameters
-
queue a fetch queue
Definition at line 353 of file folder.cpp.
|
slot |
Called when a child was modified.
- Parameters
-
node the child that was changed
Definition at line 325 of file folder.cpp.
|
slot |
Called when a child was destroyed.
- Parameters
-
node the child that was destroyed
Definition at line 331 of file folder.cpp.
bool Folder::subtreeContains | ( | const Akregator::TreeNode * | node | ) | const |
Definition at line 338 of file folder.cpp.
|
virtual |
converts the feed group into OPML format for save and export and appends it to node parent
in document .
Children are processed and appended recursively.
- Parameters
-
parent The parent element document The DOM document
- Returns
- The newly created element representing this feed group
Implements Akregator::TreeNode.
Definition at line 110 of file folder.cpp.
|
virtual |
returns the number of articles in all children
- Returns
- number of articles
Implements Akregator::TreeNode.
Definition at line 301 of file folder.cpp.
|
virtual |
returns the number of unread articles in all children
- Returns
- number of unread articles
Implements Akregator::TreeNode.
Definition at line 296 of file folder.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:14 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.