akregator
#include <treenode.h>
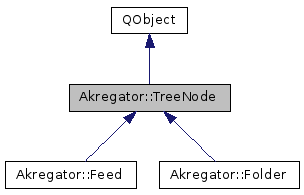
Public Slots | |
virtual void | slotAddToFetchQueue (Akregator::FetchQueue *queue, bool intervalFetchesOnly=false)=0 |
Signals | |
void | signalArticlesAdded (Akregator::TreeNode *, const QList< Akregator::Article > &guids) |
void | signalArticlesRemoved (Akregator::TreeNode *, const QList< Akregator::Article > &guids) |
void | signalArticlesUpdated (Akregator::TreeNode *, const QList< Akregator::Article > &guids) |
void | signalChanged (Akregator::TreeNode *) |
void | signalDestroyed (Akregator::TreeNode *) |
Public Member Functions | |
TreeNode () | |
virtual | ~TreeNode () |
virtual bool | accept (TreeNodeVisitor *visitor)=0 |
virtual TreeNode * | childAt (int pos) |
virtual const TreeNode * | childAt (int pos) const |
virtual QList< const TreeNode * > | children () const |
virtual QList< TreeNode * > | children () |
ArticleListJob * | createListJob () |
virtual KJob * | createMarkAsReadJob ()=0 |
virtual QVector< const Feed * > | feeds () const =0 |
virtual QVector< Feed * > | feeds ()=0 |
virtual QVector< const Folder * > | folders () const =0 |
virtual QVector< Folder * > | folders ()=0 |
virtual QIcon | icon () const =0 |
virtual uint | id () const |
virtual bool | isAggregation () const =0 |
virtual bool | isGroup () const =0 |
QPoint | listViewScrollBarPositions () const |
virtual const TreeNode * | next () const =0 |
virtual TreeNode * | next ()=0 |
virtual const TreeNode * | nextSibling () const |
virtual TreeNode * | nextSibling () |
virtual const Folder * | parent () const |
virtual Folder * | parent () |
virtual const TreeNode * | prevSibling () const |
virtual TreeNode * | prevSibling () |
virtual void | setId (uint id) |
void | setListViewScrollBarPositions (const QPoint &pos) |
virtual void | setNotificationMode (bool doNotify) |
virtual void | setParent (Folder *parent) |
void | setTitle (const QString &title) |
QString | title () const |
virtual QDomElement | toOPML (QDomElement parent, QDomDocument document) const =0 |
virtual int | totalCount () const =0 |
virtual int | unread () const =0 |
Protected Member Functions | |
virtual void | articlesModified () |
virtual void | doArticleNotification () |
void | emitSignalDestroyed () |
virtual void | nodeModified () |
Detailed Description
Abstract base class for all kind of elements in the feed tree, like feeds and feed groups (and search folders later).
TODO: detailed description goes here
Definition at line 59 of file treenode.h.
Constructor & Destructor Documentation
Akregator::TreeNode::TreeNode | ( | ) |
Standard constructor.
Definition at line 64 of file treenode.cpp.
|
virtual |
Standard destructor.
Definition at line 80 of file treenode.cpp.
Member Function Documentation
|
pure virtual |
Implemented in Akregator::Feed, and Akregator::Folder.
|
protectedvirtual |
call this if the articles in the node were changed.
Sends signalArticlesAdded/Updated/Removed signals Will do notification immediately or cache it, depending on m_doNotify
.
Definition at line 216 of file treenode.cpp.
|
virtual |
Reimplemented in Akregator::Folder.
Definition at line 167 of file treenode.cpp.
|
virtual |
Reimplemented in Akregator::Folder.
Definition at line 161 of file treenode.cpp.
returns the (direct) children of this node.
- Returns
- a list of pointers to the child nodes
Reimplemented in Akregator::Folder.
Definition at line 151 of file treenode.cpp.
Reimplemented in Akregator::Folder.
Definition at line 156 of file treenode.cpp.
ArticleListJob * Akregator::TreeNode::createListJob | ( | ) |
Definition at line 238 of file treenode.cpp.
|
pure virtual |
Implemented in Akregator::Feed, and Akregator::Folder.
|
protectedvirtual |
reimplement this in subclasses to do the actual notification called by articlesModified
Reimplemented in Akregator::Folder.
Definition at line 224 of file treenode.cpp.
|
protected |
Definition at line 69 of file treenode.cpp.
|
pure virtual |
Implemented in Akregator::Feed, and Akregator::Folder.
|
pure virtual |
Implemented in Akregator::Feed, and Akregator::Folder.
|
pure virtual |
Implemented in Akregator::Feed, and Akregator::Folder.
|
pure virtual |
Implemented in Akregator::Feed, and Akregator::Folder.
|
pure virtual |
Implemented in Akregator::Feed, and Akregator::Folder.
|
virtual |
returns the ID of this node.
IDs are managed by FeedList objects and must be unique within the list. Some IDs have a special meaning: 0
is the default value and indicates that no ID was set 1
is reserved for the "All Feeds" root node
Definition at line 198 of file treenode.cpp.
|
pure virtual |
returns if the node represents an aggregation, i.e.
containing items from more than once source feed. Folders and virtual folders are aggregations, feeds are not.
Implemented in Akregator::Feed, and Akregator::Folder.
|
pure virtual |
Helps the rest of the app to decide if node should be handled as group or not.
Only use where necessary, use polymorphism where possible.
- Returns
- whether the node is a feed group or not
Implemented in Akregator::Feed, and Akregator::Folder.
QPoint Akregator::TreeNode::listViewScrollBarPositions | ( | ) | const |
Definition at line 228 of file treenode.cpp.
|
pure virtual |
returns the next node in the tree.
Calling next() unless it returns 0 iterates through the tree in pre-order
Implemented in Akregator::Feed, and Akregator::Folder.
|
pure virtual |
Implemented in Akregator::Feed, and Akregator::Folder.
|
virtual |
Get the next sibling.
- Returns
- the next sibling, 0 if there is none
Definition at line 112 of file treenode.cpp.
|
virtual |
Definition at line 102 of file treenode.cpp.
|
protectedvirtual |
call this if you modified the actual node (title, unread count).
Call this only when the actual node has changed, i.e. title, unread count. Don't use for article changes! Will do notification immediately or cache it, depending on m_doNotify
.
Definition at line 208 of file treenode.cpp.
|
virtual |
Returns the parent node.
- Returns
- the parent feed group, 0 if there is none
Definition at line 141 of file treenode.cpp.
|
virtual |
Definition at line 146 of file treenode.cpp.
|
virtual |
Get the previous sibling.
- Returns
- the previous sibling, 0 if there is none
Definition at line 132 of file treenode.cpp.
|
virtual |
Definition at line 122 of file treenode.cpp.
|
virtual |
sets the ID
Definition at line 203 of file treenode.cpp.
void Akregator::TreeNode::setListViewScrollBarPositions | ( | const QPoint & | pos | ) |
Definition at line 233 of file treenode.cpp.
|
virtual |
- Parameters
-
doNotify notification on changes on/off flag notifyOccurredChanges notify changes occurred while turn off when set to true again
Definition at line 178 of file treenode.cpp.
|
virtual |
Sets parent node; Don't call this directly, is done automatically by insertChild-methods in Folder.
Definition at line 173 of file treenode.cpp.
void Akregator::TreeNode::setTitle | ( | const QString & | title | ) |
Sets the title of the node.
title
should not contain entities.
- Parameters
-
title the title string
Definition at line 92 of file treenode.cpp.
|
signal |
emitted when new articles were added to this node or any node in the subtree (for folders).
Note that this has nothing to do with fetching, the article might have been moved from somewhere else in the tree into this subtree, e.g. by moving the feed the article is in.
- Parameters
-
TreeNode* the node articles were added to QStringList the guids of the articles added
|
signal |
emitted when articles were removed from this subtree.
Note that this has nothing to do with actual article deletion! The article might have moved somewhere else in the tree, e.g. if the user moved the feed
|
signal |
emitted when articles were updated
|
signal |
Notification mechanism: emitted, when the node was modified and notification is enabled.
A node change is renamed title, icon, unread count. Added, updated or removed articles are not notified via this signal
|
signal |
Emitted when this object is deleted.
|
pure virtualslot |
adds node to a fetch queue
- Parameters
-
intervalFetchesOnly
QString Akregator::TreeNode::title | ( | ) | const |
|
pure virtual |
exports node and child nodes to OPML (with akregator settings)
- Parameters
-
parent the dom element the child node will be attached to document the opml document
Implemented in Akregator::Feed, and Akregator::Folder.
|
pure virtual |
returns the number of total articles in the node (for groups: the accumulated count of the subtree)
- Returns
- number of articles
Implemented in Akregator::Feed, and Akregator::Folder.
|
pure virtual |
The unread count, returns the number of new/unread articles in the node (for groups: the accumulated count of the subtree)
- Returns
- number of new/unread articles
Implemented in Akregator::Feed, and Akregator::Folder.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:15 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.