calendarsupport
#include <kcalprefs.h>
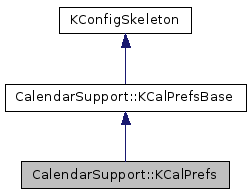
Public Member Functions | |
KCalPrefs () | |
virtual | ~KCalPrefs () |
QStringList | allEmails () |
QColor | categoryColor (const QString &cat) const |
Akonadi::Entity::Id | defaultCalendarId () const |
QString | email () |
QStringList | fullEmails () |
QString | fullName () |
bool | hasCategoryColor (const QString &cat) const |
QString | mailTransport () const |
void | setCategoryColor (const QString &cat, const QColor &color) |
void | setDefaultCalendarId (const Akonadi::Entity::Id) |
void | setEmail (const QString &) |
void | setFullName (const QString &) |
void | setTimeSpec (const KDateTime::Spec &spec) |
bool | thatIsMe (const QString &email) |
KDateTime::Spec | timeSpec () |
void | usrReadConfig () |
void | usrSetDefaults () |
void | usrWriteConfig () |
![]() | |
KCalPrefsBase () | |
~KCalPrefsBase () | |
QStringList | activeDesignerFields () const |
ItemStringList * | activeDesignerFieldsItem () |
QStringList | additionalMails () const |
ItemStringList * | additionalMailsItem () |
int | archiveAction () const |
ItemEnum * | archiveActionItem () |
bool | archiveEvents () const |
ItemBool * | archiveEventsItem () |
QString | archiveFile () const |
ItemString * | archiveFileItem () |
bool | archiveTodos () const |
ItemBool * | archiveTodosItem () |
QString | audioFilePath () const |
ItemPath * | audioFilePathItem () |
bool | autoArchive () const |
ItemBool * | autoArchiveItem () |
bool | defaultAudioFileReminders () const |
ItemBool * | defaultAudioFileRemindersItem () |
QDateTime | defaultDuration () const |
ItemDateTime * | defaultDurationItem () |
bool | defaultEventReminders () const |
ItemBool * | defaultEventRemindersItem () |
bool | defaultTodoReminders () const |
ItemBool * | defaultTodoRemindersItem () |
bool | emailControlCenter () const |
ItemBool * | emailControlCenterItem () |
QStringList | eventTemplates () const |
ItemStringList * | eventTemplatesItem () |
bool | excludeHolidays () const |
ItemBool * | excludeHolidaysItem () |
int | expiryTime () const |
ItemInt * | expiryTimeItem () |
int | expiryUnit () const |
ItemEnum * | expiryUnitItem () |
QString | holidays () const |
ItemString * | holidaysItem () |
QStringList | journalTemplates () const |
ItemStringList * | journalTemplatesItem () |
int | reminderTime () const |
ItemInt * | reminderTimeItem () |
int | reminderTimeUnits () const |
ItemInt * | reminderTimeUnitsItem () |
void | setActiveDesignerFields (const QStringList &v) |
void | setAdditionalMails (const QStringList &v) |
void | setArchiveAction (int v) |
void | setArchiveEvents (bool v) |
void | setArchiveFile (const QString &v) |
void | setArchiveTodos (bool v) |
void | setAudioFilePath (const QString &v) |
void | setAutoArchive (bool v) |
void | setDefaultAudioFileReminders (bool v) |
void | setDefaultDuration (const QDateTime &v) |
void | setDefaultEventReminders (bool v) |
void | setDefaultTodoReminders (bool v) |
void | setEmailControlCenter (bool v) |
void | setEventTemplates (const QStringList &v) |
void | setExcludeHolidays (bool v) |
void | setExpiryTime (int v) |
void | setExpiryUnit (int v) |
void | setHolidays (const QString &v) |
void | setJournalTemplates (const QStringList &v) |
void | setReminderTime (int v) |
void | setReminderTimeUnits (int v) |
void | setShowTimeZoneSelectorInIncidenceEditor (bool v) |
void | setStartTime (const QDateTime &v) |
void | setTodoTemplates (const QStringList &v) |
void | setUnsetCategoryColor (const QColor &v) |
void | setUseGroupwareCommunication (bool v) |
void | setUserEmail (const QString &v) |
void | setUserName (const QString &v) |
void | setWorkWeekMask (int v) |
bool | showTimeZoneSelectorInIncidenceEditor () const |
ItemBool * | showTimeZoneSelectorInIncidenceEditorItem () |
QDateTime | startTime () const |
ItemDateTime * | startTimeItem () |
QStringList | todoTemplates () const |
ItemStringList * | todoTemplatesItem () |
QColor | unsetCategoryColor () const |
ItemColor * | unsetCategoryColorItem () |
bool | useGroupwareCommunication () const |
ItemBool * | useGroupwareCommunicationItem () |
QString | userEmail () const |
ItemString * | userEmailItem () |
QString | userName () const |
ItemString * | userNameItem () |
int | workWeekMask () const |
ItemInt * | workWeekMaskItem () |
Static Public Member Functions | |
static KCalPrefs * | instance () |
Protected Member Functions | |
void | fillMailDefaults () |
void | setTimeZoneDefault () |
Additional Inherited Members | |
![]() | |
enum | EnumArchiveAction { actionDelete, actionArchive } |
enum | EnumExpiryUnit { UnitDays, UnitWeeks, UnitMonths } |
![]() | |
QStringList | mActiveDesignerFields |
QStringList | mAdditionalMails |
int | mArchiveAction |
bool | mArchiveEvents |
QString | mArchiveFile |
bool | mArchiveTodos |
QString | mAudioFilePath |
bool | mAutoArchive |
bool | mDefaultAudioFileReminders |
QDateTime | mDefaultDuration |
bool | mDefaultEventReminders |
bool | mDefaultTodoReminders |
bool | mEmailControlCenter |
QStringList | mEventTemplates |
bool | mExcludeHolidays |
int | mExpiryTime |
int | mExpiryUnit |
QString | mHolidays |
QStringList | mJournalTemplates |
int | mReminderTime |
int | mReminderTimeUnits |
bool | mShowTimeZoneSelectorInIncidenceEditor |
QDateTime | mStartTime |
QStringList | mTodoTemplates |
QColor | mUnsetCategoryColor |
bool | mUseGroupwareCommunication |
QString | mUserEmail |
QString | mUserName |
int | mWorkWeekMask |
Detailed Description
Definition at line 34 of file kcalprefs.h.
Constructor & Destructor Documentation
KCalPrefs::KCalPrefs | ( | ) |
Constructor disabled for public.
Use instance() to create a KCalPrefs object.
Definition at line 66 of file kcalprefs.cpp.
|
virtual |
Definition at line 70 of file kcalprefs.cpp.
Member Function Documentation
QStringList KCalPrefs::allEmails | ( | ) |
Returns all email addresses for the user.
Definition at line 231 of file kcalprefs.cpp.
QColor KCalPrefs::categoryColor | ( | const QString & | cat | ) | const |
Definition at line 316 of file kcalprefs.cpp.
Akonadi::Entity::Id KCalPrefs::defaultCalendarId | ( | ) | const |
Definition at line 118 of file kcalprefs.cpp.
QString KCalPrefs::email | ( | ) |
Definition at line 221 of file kcalprefs.cpp.
|
protected |
Fill empty mail fields with default values.
Definition at line 141 of file kcalprefs.cpp.
QStringList KCalPrefs::fullEmails | ( | ) |
Returns all email addresses together with the full username for the user.
Definition at line 244 of file kcalprefs.cpp.
QString KCalPrefs::fullName | ( | ) |
Definition at line 201 of file kcalprefs.cpp.
bool KCalPrefs::hasCategoryColor | ( | const QString & | cat | ) | const |
Definition at line 327 of file kcalprefs.cpp.
|
static |
Get instance of KCalPrefs.
It is made sure that there is only one instance.
Definition at line 75 of file kcalprefs.cpp.
QString CalendarSupport::KCalPrefs::mailTransport | ( | ) | const |
void KCalPrefs::setCategoryColor | ( | const QString & | cat, |
const QColor & | color | ||
) |
Definition at line 311 of file kcalprefs.cpp.
void KCalPrefs::setDefaultCalendarId | ( | const Akonadi::Entity::Id | id | ) |
Definition at line 123 of file kcalprefs.cpp.
void CalendarSupport::KCalPrefs::setEmail | ( | const QString & | ) |
void CalendarSupport::KCalPrefs::setFullName | ( | const QString & | ) |
void KCalPrefs::setTimeSpec | ( | const KDateTime::Spec & | spec | ) |
Definition at line 113 of file kcalprefs.cpp.
|
protected |
Definition at line 128 of file kcalprefs.cpp.
bool KCalPrefs::thatIsMe | ( | const QString & | ) |
Return true if the given email belongs to the user.
Definition at line 268 of file kcalprefs.cpp.
KDateTime::Spec KCalPrefs::timeSpec | ( | ) |
Definition at line 108 of file kcalprefs.cpp.
void KCalPrefs::usrReadConfig | ( | ) |
Read preferences from config file.
Definition at line 156 of file kcalprefs.cpp.
void KCalPrefs::usrSetDefaults | ( | ) |
Set preferences to default values.
Definition at line 87 of file kcalprefs.cpp.
void KCalPrefs::usrWriteConfig | ( | ) |
Write preferences to config file.
Definition at line 181 of file kcalprefs.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:54:59 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.