kalarm
#include <preferences.h>
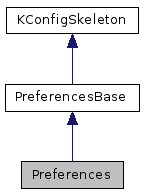
Public Types | |
enum | MailFrom { MAIL_FROM_KMAIL, MAIL_FROM_SYS_SETTINGS, MAIL_FROM_ADDR } |
![]() | |
enum | { signalShowInSystemTrayChanged = 0x1, signalAutoHideSystemTrayChanged = 0x2, signalAskResourceChanged = 0x4, signalBase_TimeZoneChanged = 0x8, signalBase_HolidayRegionChanged = 0x10, signalBase_StartOfDayChanged = 0x20, signalBase_WorkTimeChanged = 0x40, signalMessageFontChanged = 0x80, signalDisabledColourChanged = 0x100, signalArchivedColourChanged = 0x200, signalArchivedKeepDaysChanged = 0x400, signalFeb29TypeChanged = 0x800, signalTooltipPreferencesChanged = 0x1000 } |
enum | Backend { Kresources, Akonadi } |
enum | CmdLogType { Log_Discard, Log_File, Log_Terminal } |
enum | Feb29Type { Feb29_Feb28, Feb29_Mar1, Feb29_None } |
enum | MailClient { sendmail, kmail } |
enum | RecurType { Recur_None, Recur_Login, Recur_SubDaily, Recur_Daily, Recur_Weekly, Recur_Monthly, Recur_Yearly } |
enum | SoundType { Sound_None, Sound_Beep, Sound_File, Sound_Speak } |
Signals | |
void | holidaysChanged (const KHolidays::HolidayRegion &newHolidays) |
void | startOfDayChanged (const QTime &newStartOfDay) |
void | timeZoneChanged (const KTimeZone &newTz) |
void | workTimeChanged (const QTime &startTime, const QTime &endTime, const QBitArray &workDays) |
![]() | |
void | archivedColourChanged (const QColor &ArchivedColour) |
void | archivedKeepDaysChanged (int ArchivedKeepDays) |
void | askResourceChanged (bool AskResource) |
void | autoHideSystemTrayChanged (int AutoHideSystemTray) |
void | base_HolidayRegionChanged (const QString &Base_HolidayRegion) |
void | base_StartOfDayChanged (const QDateTime &Base_StartOfDay) |
void | base_TimeZoneChanged (const QString &Base_TimeZone) |
void | base_WorkTimeChanged (const QDateTime &Base_WorkDayStart, const QDateTime &Base_WorkDayEnd, int Base_WorkDays) |
void | disabledColourChanged (const QColor &DisabledColour) |
void | feb29TypeChanged (Feb29Type DefaultFeb29Type) |
void | messageFontChanged (const QFont &MessageFont) |
void | showInSystemTrayChanged (bool ShowInSystemTray) |
void | tooltipPreferencesChanged () |
Static Public Member Functions | |
static bool | autoStartChangedByUser () |
static QString | cmdXTermCommand () |
static bool | confirmAlarmDeletion () |
static void | connect (const char *signal, const QObject *receiver, const char *member) |
static float | defaultSoundVolume () |
static QString | emailAddress () |
static QString | emailBccAddress () |
static MailFrom | emailBccFrom () |
static bool | emailBccUseSystemSettings () |
static bool | emailCopyToKMail () |
static MailFrom | emailFrom () |
static bool | emailQueuedNotify () |
static const KHolidays::HolidayRegion & | holidays () |
static Backend | previousBackend () |
static QString | previousVersion () |
static bool | quitWarn () |
static Preferences * | self () |
static void | setAskAutoStart (bool yes) |
static void | setAutoStartChangedByUser (bool c) |
static void | setCmdXTermCommand (const QString &cmd) |
static void | setConfirmAlarmDeletion (bool yes) |
static void | setDefaultSoundVolume (float v) |
static void | setEmailAddress (MailFrom, const QString &address) |
static void | setEmailBccAddress (bool useSystemSettings, const QString &address) |
static void | setEmailCopyToKMail (bool yes) |
static void | setEmailQueuedNotify (bool yes) |
static void | setHolidayRegion (const QString ®ionCode) |
static void | setQuitWarn (bool yes) |
static void | setStartOfDay (const QTime &) |
static void | setTimeZone (const KTimeZone &) |
static void | setWorkDayEnd (const QTime &t) |
static void | setWorkDays (const QBitArray &) |
static void | setWorkDayStart (const QTime &t) |
static QTime | startOfDay () |
static KTimeZone | timeZone (bool reload=false) |
static QTime | workDayEnd () |
static QBitArray | workDays () |
static QTime | workDayStart () |
![]() | |
static QColor | archivedColour () |
static int | archivedKeepDays () |
static bool | askResource () |
static int | autoHideSystemTray () |
static bool | autoStart () |
static Backend | backend () |
static QString | base_CmdXTermCommand () |
static bool | base_ConfirmAlarmDeletion () |
static int | base_DefaultSoundVolume () |
static QString | base_EmailBccAddress () |
static bool | base_EmailCopyToKMail () |
static QString | base_EmailFrom () |
static bool | base_EmailQueuedNotify () |
static QString | base_HolidayRegion () |
static bool | base_QuitWarn () |
static QDateTime | base_StartOfDay () |
static QString | base_TimeZone () |
static QDateTime | base_WorkDayEnd () |
static uint | base_WorkDays () |
static QDateTime | base_WorkDayStart () |
static bool | defaultAutoClose () |
static QColor | defaultBgColour () |
static bool | defaultCancelOnPreActionError () |
static QString | defaultCmdLogFile () |
static CmdLogType | defaultCmdLogType () |
static bool | defaultCmdScript () |
static bool | defaultConfirmAck () |
static bool | defaultCopyToKOrganizer () |
static int | defaultDeferTime () |
static bool | defaultDontShowPreActionError () |
static bool | defaultEmailBcc () |
static bool | defaultExecPreActionOnDeferral () |
static Feb29Type | defaultFeb29Type () |
static QColor | defaultFgColour () |
static int | defaultLateCancel () |
static QString | defaultPostAction () |
static QString | defaultPreAction () |
static RecurType | defaultRecurPeriod () |
static TimePeriod::Units | defaultReminderUnits () |
static QString | defaultSoundFile () |
static bool | defaultSoundRepeat () |
static SoundType | defaultSoundType () |
static QColor | disabledColour () |
static MailClient | emailClient () |
static int | kOrgEventDuration () |
static int | messageButtonDelay () |
static QFont | messageFont () |
static bool | modalMessages () |
static bool | noAutoStart () |
static PreferencesBase * | self () |
static void | setArchivedColour (const QColor &v) |
static void | setArchivedKeepDays (int v) |
static void | setAskResource (bool v) |
static void | setAutoHideSystemTray (int v) |
static void | setAutoStart (bool v) |
static void | setBackend (Backend v) |
static void | setBase_CmdXTermCommand (const QString &v) |
static void | setBase_ConfirmAlarmDeletion (bool v) |
static void | setBase_DefaultSoundVolume (int v) |
static void | setBase_EmailBccAddress (const QString &v) |
static void | setBase_EmailCopyToKMail (bool v) |
static void | setBase_EmailFrom (const QString &v) |
static void | setBase_EmailQueuedNotify (bool v) |
static void | setBase_HolidayRegion (const QString &v) |
static void | setBase_QuitWarn (bool v) |
static void | setBase_StartOfDay (const QDateTime &v) |
static void | setBase_TimeZone (const QString &v) |
static void | setBase_WorkDayEnd (const QDateTime &v) |
static void | setBase_WorkDays (uint v) |
static void | setBase_WorkDayStart (const QDateTime &v) |
static void | setDefaultAutoClose (bool v) |
static void | setDefaultBgColour (const QColor &v) |
static void | setDefaultCancelOnPreActionError (bool v) |
static void | setDefaultCmdLogFile (const QString &v) |
static void | setDefaultCmdLogType (CmdLogType v) |
static void | setDefaultCmdScript (bool v) |
static void | setDefaultConfirmAck (bool v) |
static void | setDefaultCopyToKOrganizer (bool v) |
static void | setDefaultDeferTime (int v) |
static void | setDefaultDontShowPreActionError (bool v) |
static void | setDefaultEmailBcc (bool v) |
static void | setDefaultExecPreActionOnDeferral (bool v) |
static void | setDefaultFeb29Type (Feb29Type v) |
static void | setDefaultFgColour (const QColor &v) |
static void | setDefaultLateCancel (int v) |
static void | setDefaultPostAction (const QString &v) |
static void | setDefaultPreAction (const QString &v) |
static void | setDefaultRecurPeriod (RecurType v) |
static void | setDefaultReminderUnits (TimePeriod::Units v) |
static void | setDefaultSoundFile (const QString &v) |
static void | setDefaultSoundRepeat (bool v) |
static void | setDefaultSoundType (SoundType v) |
static void | setDisabledColour (const QColor &v) |
static void | setEmailClient (MailClient v) |
static void | setKOrgEventDuration (int v) |
static void | setMessageButtonDelay (int v) |
static void | setMessageFont (const QFont &v) |
static void | setModalMessages (bool v) |
static void | setNoAutoStart (bool v) |
static void | setShowInSystemTray (bool v) |
static void | setShowTooltipAlarmTime (bool v) |
static void | setShowTooltipTimeToAlarm (bool v) |
static void | setTooltipAlarmCount (int v) |
static void | setTooltipTimeToPrefix (const QString &v) |
static void | setVersion (const QString &v) |
static void | setWakeFromSuspendAdvance (int v) |
static bool | showInSystemTray () |
static bool | showTooltipAlarmTime () |
static bool | showTooltipTimeToAlarm () |
static int | tooltipAlarmCount () |
static QString | tooltipTimeToPrefix () |
static QString | version () |
static int | wakeFromSuspendAdvance () |
Static Public Attributes | |
static const QLatin1String | ASK_AUTO_START |
static const QLatin1String | CONFIRM_ALARM_DELETION |
static const QLatin1String | EMAIL_QUEUED_NOTIFY |
static const QLatin1String | QUIT_WARN |
Detailed Description
Definition at line 36 of file preferences.h.
Member Enumeration Documentation
Enumerator | |
---|---|
MAIL_FROM_KMAIL | |
MAIL_FROM_SYS_SETTINGS | |
MAIL_FROM_ADDR |
Definition at line 40 of file preferences.h.
Member Function Documentation
|
inlinestatic |
Definition at line 44 of file preferences.h.
|
static |
Definition at line 281 of file preferences.cpp.
|
inlinestatic |
Definition at line 65 of file preferences.h.
|
static |
Definition at line 292 of file preferences.cpp.
|
inlinestatic |
Definition at line 80 of file preferences.h.
|
static |
Definition at line 227 of file preferences.cpp.
|
static |
Definition at line 258 of file preferences.cpp.
|
static |
Definition at line 250 of file preferences.cpp.
|
static |
Definition at line 266 of file preferences.cpp.
|
inlinestatic |
Definition at line 67 of file preferences.h.
|
static |
Definition at line 214 of file preferences.cpp.
|
inlinestatic |
Definition at line 69 of file preferences.h.
|
static |
Definition at line 150 of file preferences.cpp.
|
signal |
|
inlinestatic |
Definition at line 49 of file preferences.h.
|
inlinestatic |
Definition at line 48 of file preferences.h.
|
inlinestatic |
Definition at line 63 of file preferences.h.
|
static |
Definition at line 80 of file preferences.cpp.
|
static |
Definition at line 112 of file preferences.cpp.
|
inlinestatic |
Definition at line 45 of file preferences.h.
|
static |
Definition at line 286 of file preferences.cpp.
|
inlinestatic |
Definition at line 66 of file preferences.h.
|
inlinestatic |
Definition at line 81 of file preferences.h.
|
static |
Definition at line 237 of file preferences.cpp.
|
static |
Definition at line 271 of file preferences.cpp.
|
inlinestatic |
Definition at line 68 of file preferences.h.
|
inlinestatic |
Definition at line 70 of file preferences.h.
|
static |
Definition at line 161 of file preferences.cpp.
|
inlinestatic |
Definition at line 64 of file preferences.h.
|
static |
Definition at line 172 of file preferences.cpp.
|
static |
Definition at line 139 of file preferences.cpp.
|
inlinestatic |
Definition at line 61 of file preferences.h.
|
static |
Definition at line 196 of file preferences.cpp.
|
inlinestatic |
Definition at line 60 of file preferences.h.
|
inlinestatic |
Definition at line 55 of file preferences.h.
|
signal |
|
static |
Definition at line 122 of file preferences.cpp.
|
signal |
|
inlinevirtual |
Definition at line 89 of file preferences.h.
|
inlinestatic |
Definition at line 58 of file preferences.h.
|
static |
Definition at line 187 of file preferences.cpp.
|
inlinestatic |
Definition at line 57 of file preferences.h.
|
signal |
Member Data Documentation
|
static |
Definition at line 85 of file preferences.h.
|
static |
Definition at line 86 of file preferences.h.
|
static |
Definition at line 87 of file preferences.h.
|
static |
Definition at line 84 of file preferences.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:59:11 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.