kalarm/lib
#include <timeedit.h>
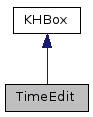
Public Slots | |
virtual void | setValue (int minutes) |
void | setValue (const QTime &t) |
Signals | |
void | valueChanged (int minutes) |
Public Member Functions | |
TimeEdit (QWidget *parent=0) | |
bool | isReadOnly () const |
bool | isValid () const |
int | maximum () const |
QTime | maxTime () const |
int | minimum () const |
void | setMaximum (int minutes) |
void | setMaximum (const QTime &time) |
void | setMinimum (int minutes) |
virtual void | setReadOnly (bool readOnly) |
void | setValid (bool valid) |
void | setWrapping (bool on) |
QTime | time () const |
int | value () const |
bool | wrapping () const |
Detailed Description
Widget to enter a time of day.
The TimeEdit class provides a widget to enter a time of day in hours and minutes, using a 12- or 24-hour clock according to the user's locale settings.
It displays a TimeSpinBox widget to enter hours and minutes. If a 12-hour clock is being used, it also displays a combo box to select am or pm.
TimeSpinBox displays a spin box with two pairs of spin buttons, one for hours and one for minutes. It provides accelerated stepping using the spin buttons, when the shift key is held down (inherited from SpinBox2). The default shift steps are 5 minutes and 6 hours.
The widget may be set as read-only. This has the same effect as disabling it, except that its appearance is unchanged.
Definition at line 50 of file timeedit.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
- Parameters
-
parent The parent object of this widget.
Definition at line 31 of file timeedit.cpp.
Member Function Documentation
|
inline |
Returns true if the widget is read only.
Definition at line 59 of file timeedit.h.
bool TimeEdit::isValid | ( | ) | const |
Returns true if the widget contains a valid value.
Definition at line 67 of file timeedit.cpp.
int TimeEdit::maximum | ( | ) | const |
Returns the maximum value of the widget in minutes.
Definition at line 117 of file timeedit.cpp.
|
inline |
Returns the maximum value of the widget as a QTime value.
Definition at line 89 of file timeedit.h.
int TimeEdit::minimum | ( | ) | const |
Returns the minimum value of the widget in minutes.
Definition at line 112 of file timeedit.cpp.
void TimeEdit::setMaximum | ( | int | minutes | ) |
Sets the maximum value of the widget.
Definition at line 129 of file timeedit.cpp.
|
inline |
Sets the maximum value of the widget.
Definition at line 95 of file timeedit.h.
void TimeEdit::setMinimum | ( | int | minutes | ) |
Sets the minimum value of the widget.
Definition at line 122 of file timeedit.cpp.
|
virtual |
Sets whether the widget is read-only for the user.
If read-only, the time cannot be edited and the spin buttons and am/pm combo box are inactive.
- Parameters
-
readOnly True to set the widget read-only, false to set it read-write.
Definition at line 51 of file timeedit.cpp.
void TimeEdit::setValid | ( | bool | valid | ) |
Sets whether the edit value is valid.
If newly invalid, the value is displayed as asterisks. If newly valid, the value is set to the minimum value.
- Parameters
-
valid True to set the value valid, false to set it invalid.
Definition at line 77 of file timeedit.cpp.
|
virtualslot |
Sets the value of the widget.
Definition at line 92 of file timeedit.cpp.
|
inlineslot |
Sets the value of the widget.
Definition at line 101 of file timeedit.h.
void TimeEdit::setWrapping | ( | bool | on | ) |
Sets whether it is possible to step the value from the highest value to the lowest value and vice versa.
- Parameters
-
on True to enable wrapping, else false.
Definition at line 107 of file timeedit.cpp.
|
inline |
Returns the entered time as a QTime value.
Definition at line 77 of file timeedit.h.
int TimeEdit::value | ( | ) | const |
Returns the entered time as a value in minutes.
Definition at line 62 of file timeedit.cpp.
|
signal |
This signal is emitted every time the value of the widget changes (for whatever reason).
- Parameters
-
minutes The new value.
bool TimeEdit::wrapping | ( | ) | const |
Returns true if it is possible to step the value from the highest value to the lowest value and vice versa.
Definition at line 102 of file timeedit.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:59:21 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.