kleopatra
#include <qwizard.h>
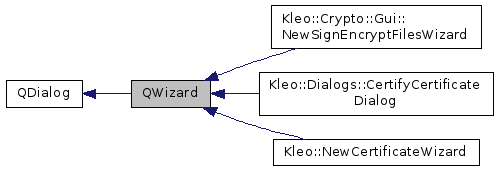
Public Types | |
enum | WizardButton { BackButton, NextButton, CommitButton, FinishButton, CancelButton, HelpButton, CustomButton1, CustomButton2, CustomButton3, Stretch, NoButton = -1, NStandardButtons = 6, NButtons = 9 } |
enum | WizardOption { IndependentPages = 0x00000001, IgnoreSubTitles = 0x00000002, ExtendedWatermarkPixmap = 0x00000004, NoDefaultButton = 0x00000008, NoBackButtonOnStartPage = 0x00000010, NoBackButtonOnLastPage = 0x00000020, DisabledBackButtonOnLastPage = 0x00000040, HaveNextButtonOnLastPage = 0x00000080, HaveFinishButtonOnEarlyPages = 0x00000100, NoCancelButton = 0x00000200, CancelButtonOnLeft = 0x00000400, HaveHelpButton = 0x00000800, HelpButtonOnRight = 0x00001000, HaveCustomButton1 = 0x00002000, HaveCustomButton2 = 0x00004000, HaveCustomButton3 = 0x00008000 } |
enum | WizardPixmap { WatermarkPixmap, LogoPixmap, BannerPixmap, BackgroundPixmap, NPixmaps } |
enum | WizardStyle { ClassicStyle, ModernStyle, MacStyle, AeroStyle, NStyles } |
Public Slots | |
void | back () |
void | next () |
void | restart () |
Signals | |
void | currentIdChanged (int id) |
void | customButtonClicked (int which) |
void | helpRequested () |
void | pageAdded (int id) |
void | pageRemoved (int id) |
Public Member Functions | |
QWizard (QWidget *parent=0, Qt::WindowFlags flags=0) | |
~QWizard () | |
int | addPage (QWizardPage *page) |
QAbstractButton * | button (WizardButton which) const |
QString | buttonText (WizardButton which) const |
int | currentId () const |
QWizardPage * | currentPage () const |
QVariant | field (const QString &name) const |
bool | hasVisitedPage (int id) const |
virtual int | nextId () const |
WizardOptions | options () const |
QWizardPage * | page (int id) const |
QList< int > | pageIds () const |
QPixmap | pixmap (WizardPixmap which) const |
void | removePage (int id) |
void | setButton (WizardButton which, QAbstractButton *button) |
void | setButtonLayout (const QList< WizardButton > &layout) |
void | setButtonText (WizardButton which, const QString &text) |
void | setDefaultProperty (const char *className, const char *property, const char *changedSignal) |
void | setField (const QString &name, const QVariant &value) |
void | setOption (WizardOption option, bool on=true) |
void | setOptions (WizardOptions options) |
void | setPage (int id, QWizardPage *page) |
void | setPixmap (WizardPixmap which, const QPixmap &pixmap) |
void | setSideWidget (QWidget *widget) |
void | setStartId (int id) |
void | setSubTitleFormat (Qt::TextFormat format) |
void | setTitleFormat (Qt::TextFormat format) |
void | setVisible (bool visible) |
void | setWizardStyle (WizardStyle style) |
QWidget * | sideWidget () const |
QSize | sizeHint () const |
int | startId () const |
Qt::TextFormat | subTitleFormat () const |
bool | testOption (WizardOption option) const |
Qt::TextFormat | titleFormat () const |
virtual bool | validateCurrentPage () |
QList< int > | visitedPages () const |
WizardStyle | wizardStyle () const |
Protected Member Functions | |
virtual void | cleanupPage (int id) |
void | done (int result) |
bool | event (QEvent *event) |
virtual void | initializePage (int id) |
void | paintEvent (QPaintEvent *event) |
void | resizeEvent (QResizeEvent *event) |
Properties | |
int | currentId |
WizardOptions | options |
int | startId |
Qt::TextFormat | subTitleFormat |
Qt::TextFormat | titleFormat |
WizardStyle | wizardStyle |
Detailed Description
The QWizard class provides a framework for wizards.
- Since
- 4.3 A wizard (also called an assistant on Mac OS X) is a special type of input dialog that consists of a sequence of pages. A wizard's purpose is to guide the user through a process step by step. Wizards are useful for complex or infrequent tasks that users may find difficult to learn.
QWizard inherits QDialog and represents a wizard. Each page is a QWizardPage (a QWidget subclass). To create your own wizards, you can use these classes directly, or you can subclass them for more control.
Topics:
Member Enumeration Documentation
This enum specifies the buttons in a wizard.
BackButton The Back button ( {Go Back} on Mac OS X) NextButton The Next button ( Continue on Mac OS X) CommitButton The Commit button FinishButton The Finish button ( Done on Mac OS X) CancelButton The Cancel button (see also NoCancelButton) HelpButton The Help button (see also HaveHelpButton) CustomButton1 The first user-defined button (see also HaveCustomButton1) CustomButton2 The second user-defined button (see also HaveCustomButton2) CustomButton3 The third user-defined button (see also HaveCustomButton3)
The following value is only useful when calling setButtonLayout():
Stretch A horizontal stretch in the button layout
NoButton NStandardButtons NButtons
Enumerator | |
---|---|
BackButton | |
NextButton | |
CommitButton | |
FinishButton | |
CancelButton | |
HelpButton | |
CustomButton1 | |
CustomButton2 | |
CustomButton3 | |
Stretch | |
NoButton | |
NStandardButtons | |
NButtons |
This enum specifies various options that affect the look and feel of a wizard.
IndependentPages The pages are independent of each other (i.e., they don't derive values from each other). IgnoreSubTitles Don't show any subtitles, even if they are set. ExtendedWatermarkPixmap Extend any WatermarkPixmap all the way down to the window's edge. NoDefaultButton Don't make the Next or Finish button the dialog's {QPushButton::setDefault()}{default button}. NoBackButtonOnStartPage Don't show the Back button on the start page. NoBackButtonOnLastPage Don't show the Back button on the last page. DisabledBackButtonOnLastPage Disable the Back button on the last page. HaveNextButtonOnLastPage Show the (disabled) Next button on the last page. HaveFinishButtonOnEarlyPages Show the (disabled) Finish button on non-final pages. NoCancelButton Don't show the Cancel button. CancelButtonOnLeft Put the Cancel button on the left of Back (rather than on the right of Finish or Next). HaveHelpButton Show the Help button. HelpButtonOnRight Put the Help button on the far right of the button layout (rather than on the far left). HaveCustomButton1 Show the first user-defined button (CustomButton1). HaveCustomButton2 Show the second user-defined button (CustomButton2). HaveCustomButton3 Show the third user-defined button (CustomButton3).
- See also
- setOptions(), setOption(), testOption()
This enum specifies the pixmaps that can be associated with a page.
WatermarkPixmap The tall pixmap on the left side of a ClassicStyle or ModernStyle page LogoPixmap The small pixmap on the right side of a ClassicStyle or ModernStyle page header BannerPixmap The pixmap that occupies the background of a ModernStyle page header BackgroundPixmap The pixmap that occupies the background of a MacStyle wizard
NPixmaps
- See also
- setPixmap(), QWizardPage::setPixmap(), {Elements of a Wizard Page}
Enumerator | |
---|---|
WatermarkPixmap | |
LogoPixmap | |
BannerPixmap | |
BackgroundPixmap | |
NPixmaps |
enum QWizard::WizardStyle |
This enum specifies the different looks supported by QWizard.
ClassicStyle Classic Windows look ModernStyle Modern Windows look MacStyle Mac OS X look AeroStyle Windows Aero look
NStyles
- See also
- setWizardStyle(), WizardOption, {Wizard Look and Feel}
Enumerator | |
---|---|
ClassicStyle | |
ModernStyle | |
MacStyle | |
AeroStyle | |
NStyles |
Constructor & Destructor Documentation
|
explicit |
Constructs a wizard with the given parent and window flags.
- See also
- parent(), windowFlags()
Definition at line 2175 of file qwizard.cpp.
QWizard::~QWizard | ( | ) |
Destroys the wizard and its pages, releasing any allocated resources.
Definition at line 2185 of file qwizard.cpp.
Member Function Documentation
int QWizard::addPage | ( | QWizardPage * | page | ) |
Adds the given page to the wizard, and returns the page's ID.
The ID is guaranteed to be larger than any other ID in the QWizard so far.
- See also
- setPage(), page(), pageAdded()
Definition at line 2199 of file qwizard.cpp.
|
slot |
Goes back to the previous page.
This is equivalent to pressing the Back button.
Definition at line 3076 of file qwizard.cpp.
QAbstractButton * QWizard::button | ( | WizardButton | which | ) | const |
Returns the button corresponding to role which.
- See also
- setButton(), setButtonText()
Definition at line 2773 of file qwizard.cpp.
QString QWizard::buttonText | ( | WizardButton | which | ) | const |
Returns the text on button which.
If a text has ben set using setButtonText(), this text is returned.
By default, the text on buttons depends on the wizardStyle. For example, on Mac OS X, the Next button is called Continue.
- See also
- button(), setButton(), setButtonText(), QWizardPage::buttonText(), QWizardPage::setButtonText()
Definition at line 2671 of file qwizard.cpp.
|
protectedvirtual |
This virtual function is called by QWizard to clean up page id just before the user leaves it by clicking Back (unless the QWizard::IndependentPages option is set).
The default implementation calls QWizardPage::cleanupPage() on page(id).
Definition at line 3280 of file qwizard.cpp.
int QWizard::currentId | ( | ) | const |
|
signal |
This signal is emitted when the current page changes, with the new current id.
- See also
- currentId(), currentPage()
QWizardPage * QWizard::currentPage | ( | ) | const |
Returns a pointer to the current page, or 0 if there is no current page (e.g., before the wizard is shown).
This is equivalent to calling page(currentId()).
- See also
- page(), currentId(), restart()
Definition at line 2439 of file qwizard.cpp.
|
signal |
This signal is emitted when the user clicks a custom button. which can be CustomButton1, CustomButton2, or CustomButton3.
By default, no custom button is shown. Call setOption() with HaveCustomButton1, HaveCustomButton2, or HaveCustomButton3 to have one, and use setButtonText() or setButton() to configure it.
- See also
- helpRequested()
|
protected |
- Reimplemented from superclass.
Definition at line 3231 of file qwizard.cpp.
|
protected |
- Reimplemented from superclass.
Definition at line 3133 of file qwizard.cpp.
QVariant QWizard::field | ( | const QString & | name | ) | const |
Returns the value of the field called name.
This function can be used to access fields on any page of the wizard.
Definition at line 2493 of file qwizard.cpp.
bool QWizard::hasVisitedPage | ( | int | id | ) | const |
Returns true if the page history contains page id; otherwise, returns false.
Pressing Back marks the current page as "unvisited" again.
- See also
- visitedPages()
Definition at line 2365 of file qwizard.cpp.
|
signal |
This signal is emitted when the user clicks the Help button.
By default, no Help button is shown. Call setOption(HaveHelpButton, true) to have one.
Example:
- See also
- customButtonClicked()
|
protectedvirtual |
This virtual function is called by QWizard to prepare page id just before it is shown either as a result of QWizard::restart() being called, or as a result of the user clicking Next. (However, if the QWizard::IndependentPages option is set, this function is only called the first time the page is shown.)
By reimplementing this function, you can ensure that the page's fields are properly initialized based on fields from previous pages.
The default implementation calls QWizardPage::initializePage() on page(id).
Definition at line 3262 of file qwizard.cpp.
|
slot |
Advances to the next page.
This is equivalent to pressing the Next or Commit button.
Definition at line 3092 of file qwizard.cpp.
|
virtual |
This virtual function is called by QWizard to find out which page to show when the user clicks the Next button.
The return value is the ID of the next page, or -1 if no page follows.
The default implementation calls QWizardPage::nextId() on the currentPage().
By reimplementing this function, you can specify a dynamic page order.
- See also
- QWizardPage::nextId(), currentPage()
Definition at line 3326 of file qwizard.cpp.
WizardOptions QWizard::options | ( | ) | const |
QWizardPage * QWizard::page | ( | int | id | ) | const |
Returns the page with the given id, or 0 if there is no such page.
Definition at line 2349 of file qwizard.cpp.
|
signal |
QList< int > QWizard::pageIds | ( | ) | const |
|
signal |
- Since
- 4.7
This signal is emitted whenever a page is removed from the wizard. The page's id is passed as parameter.
- See also
- removePage(), startId()
|
protected |
- Reimplemented from superclass.
Definition at line 3178 of file qwizard.cpp.
QPixmap QWizard::pixmap | ( | WizardPixmap | which | ) | const |
Returns the pixmap set for role which.
By default, the only pixmap that is set is the BackgroundPixmap on Mac OS X.
- See also
- QWizardPage::pixmap(), {Elements of a Wizard Page}
Definition at line 2855 of file qwizard.cpp.
void QWizard::removePage | ( | int | id | ) |
Removes the page with the given id. cleanupPage() will be called if necessary.
- Note
- Removing a page may influence the value of the startId property.
- Since
- 4.5
- See also
- addPage(), setPage(), pageRemoved(), startId()
Definition at line 2275 of file qwizard.cpp.
|
protected |
- Reimplemented from superclass.
Definition at line 3156 of file qwizard.cpp.
|
slot |
Restarts the wizard at the start page. This function is called automatically when the wizard is shown.
- See also
- startId()
Definition at line 3121 of file qwizard.cpp.
void QWizard::setButton | ( | WizardButton | which, |
QAbstractButton * | button | ||
) |
Sets the button corresponding to role which to button.
To add extra buttons to the wizard (e.g., a Print button), one way is to call setButton() with CustomButton1 to CustomButton3, and make the buttons visible using the HaveCustomButton1 to HaveCustomButton3 options.
- See also
- setButtonText(), setButtonLayout(), options
Definition at line 2743 of file qwizard.cpp.
void QWizard::setButtonLayout | ( | const QList< WizardButton > & | layout | ) |
Sets the order in which buttons are displayed to layout, where layout is a list of {WizardButton}s.
The default layout depends on the options (e.g., whether HelpButtonOnRight) that are set. You can call this function if you need more control over the buttons' layout than what options already provides.
You can specify horizontal stretches in the layout using Stretch.
Example:
- See also
- setButton(), setButtonText(), setOptions()
Definition at line 2706 of file qwizard.cpp.
void QWizard::setButtonText | ( | WizardButton | which, |
const QString & | text | ||
) |
Sets the text on button which to be text.
By default, the text on buttons depends on the wizardStyle. For example, on Mac OS X, the Next button is called Continue.
To add extra buttons to the wizard (e.g., a Print button), one way is to call setButtonText() with CustomButton1, CustomButton2, or CustomButton3 to set their text, and make the buttons visible using the HaveCustomButton1, HaveCustomButton2, and/or HaveCustomButton3 options.
Button texts may also be set on a per-page basis using QWizardPage::setButtonText().
Definition at line 2646 of file qwizard.cpp.
void QWizard::setDefaultProperty | ( | const char * | className, |
const char * | property, | ||
const char * | changedSignal | ||
) |
Sets the default property for className to be property, and the associated change signal to be changedSignal.
The default property is used when an instance of className (or of one of its subclasses) is passed to QWizardPage::registerField() and no property is specified.
QWizard knows the most common Qt widgets. For these (or their subclasses), you don't need to specify a property or a changedSignal. The table below lists these widgets:
Widget Property Change Notification Signal QAbstractButton bool {QAbstractButton::}{checked} {QAbstractButton::}{toggled()} QAbstractSlider int {QAbstractSlider::}{value} {QAbstractSlider::}{valueChanged()} QComboBox int {QComboBox::}{currentIndex} {QComboBox::}{currentIndexChanged()} QDateTimeEdit QDateTime {QDateTimeEdit::}{dateTime} {QDateTimeEdit::}{dateTimeChanged()} QLineEdit QString {QLineEdit::}{text} {QLineEdit::}{textChanged()} QListWidget int {QListWidget::}{currentRow} {QListWidget::}{currentRowChanged()} QSpinBox int {QSpinBox::}{value} {QSpinBox::}{valueChanged()}
- See also
- QWizardPage::registerField()
Definition at line 2891 of file qwizard.cpp.
void QWizard::setField | ( | const QString & | name, |
const QVariant & | value | ||
) |
Sets the value of the field called name to value.
This function can be used to set fields on any page of the wizard.
Definition at line 2470 of file qwizard.cpp.
void QWizard::setOption | ( | WizardOption | option, |
bool | on = true |
||
) |
Sets the given option to be enabled if on is true; otherwise, clears the given option.
- See also
- options, testOption(), setWizardStyle()
Definition at line 2562 of file qwizard.cpp.
void QWizard::setOptions | ( | WizardOptions | options | ) |
Definition at line 2595 of file qwizard.cpp.
void QWizard::setPage | ( | int | id, |
QWizardPage * | page | ||
) |
Adds the given page to the wizard with the given id.
- Note
- Adding a page may influence the value of the startId property in case it was not set explicitly.
- See also
- addPage(), page(), pageAdded()
Definition at line 2219 of file qwizard.cpp.
void QWizard::setPixmap | ( | WizardPixmap | which, |
const QPixmap & | pixmap | ||
) |
Sets the pixmap for role which to pixmap.
The pixmaps are used by QWizard when displaying a page. Which pixmaps are actually used depend on the {Wizard Look and Feel}{wizard style}.
Pixmaps can also be set for a specific page using QWizardPage::setPixmap().
- See also
- QWizardPage::setPixmap(), {Elements of a Wizard Page}
Definition at line 2839 of file qwizard.cpp.
void QWizard::setSideWidget | ( | QWidget * | widget | ) |
- Since
- 4.7
Sets the given widget to be shown on the left side of the wizard. For styles which use the WatermarkPixmap (ClassicStyle and ModernStyle) the side widget is displayed on top of the watermark, for other styles or when the watermark is not provided the side widget is displayed on the left side of the wizard.
Passing 0 shows no side widget.
When the widget is not 0 the wizard reparents it.
Any previous side widget is hidden.
You may call setSideWidget() with the same widget at different times.
All widgets set here will be deleted by the wizard when it is destroyed unless you separately reparent the widget after setting some other side widget (or 0).
By default, no side widget is present.
Definition at line 2928 of file qwizard.cpp.
void QWizard::setStartId | ( | int | id | ) |
Definition at line 2405 of file qwizard.cpp.
void QWizard::setSubTitleFormat | ( | Qt::TextFormat | format | ) |
Definition at line 2814 of file qwizard.cpp.
void QWizard::setTitleFormat | ( | Qt::TextFormat | format | ) |
Definition at line 2793 of file qwizard.cpp.
void QWizard::setVisible | ( | bool | visible | ) |
- Reimplemented from superclass.
Definition at line 2956 of file qwizard.cpp.
void QWizard::setWizardStyle | ( | WizardStyle | style | ) |
Definition at line 2519 of file qwizard.cpp.
QWidget * QWizard::sideWidget | ( | ) | const |
- Since
- 4.7
Returns the widget on the left side of the wizard or 0.
By default, no side widget is present.
Definition at line 2946 of file qwizard.cpp.
QSize QWizard::sizeHint | ( | ) | const |
- Reimplemented from superclass.
Definition at line 2969 of file qwizard.cpp.
int QWizard::startId | ( | ) | const |
Qt::TextFormat QWizard::subTitleFormat | ( | ) | const |
bool QWizard::testOption | ( | WizardOption | option | ) | const |
Returns true if the given option is enabled; otherwise, returns false.
- See also
- options, setOption(), setWizardStyle()
Definition at line 2575 of file qwizard.cpp.
Qt::TextFormat QWizard::titleFormat | ( | ) | const |
|
virtual |
This virtual function is called by QWizard when the user clicks Next or Finish to perform some last-minute validation. If it returns true, the next page is shown (or the wizard finishes); otherwise, the current page stays up.
The default implementation calls QWizardPage::validatePage() on the currentPage().
When possible, it is usually better style to disable the Next or Finish button (by specifying {mandatory fields} or by reimplementing QWizardPage::isComplete()) than to reimplement validateCurrentPage().
- See also
- QWizardPage::validatePage(), currentPage()
Definition at line 3303 of file qwizard.cpp.
QList< int > QWizard::visitedPages | ( | ) | const |
Returns the list of IDs of visited pages, in the order in which the pages were visited.
Pressing Back marks the current page as "unvisited" again.
- See also
- hasVisitedPage()
Definition at line 2379 of file qwizard.cpp.
WizardStyle QWizard::wizardStyle | ( | ) | const |
Property Documentation
|
read |
the ID of the current page
This property cannot be set directly. To change the current page, call next(), back(), or restart().
By default, this property has a value of -1, indicating that no page is currently shown.
- See also
- currentIdChanged(), currentPage()
|
readwrite |
the various options that affect the look and feel of the wizard
By default, the following options are set (depending on the platform):
Windows: HelpButtonOnRight. Mac OS X: NoDefaultButton and NoCancelButton. X11 and QWS (Qt for Embedded Linux): none.
- See also
- wizardStyle
|
readwrite |
|
readwrite |
the text format used by page subtitles
The default format is Qt::AutoText.
- See also
- QWizardPage::title, titleFormat
|
readwrite |
the text format used by page titles
The default format is Qt::AutoText.
- See also
- QWizardPage::title, subTitleFormat
|
readwrite |
the look and feel of the wizard
By default, QWizard uses the AeroStyle on a Windows Vista system with alpha compositing enabled, regardless of the current widget style. If this is not the case, the default wizard style depends on the current widget style as follows: MacStyle is the default if the current widget style is QMacStyle, ModernStyle is the default if the current widget style is QWindowsStyle, and ClassicStyle is the default in all other cases.
- See also
- {Wizard Look and Feel}, options
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:56:42 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.