knode
#include <foldertreewidget.h>
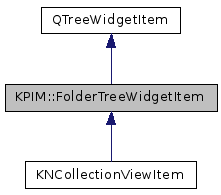
Public Types | |
enum | FolderType { Inbox, Outbox, SentMail, Trash, Drafts, Templates, Root, Calendar, Tasks, Journals, Contacts, Notes, Other } |
enum | Protocol { Local, Imap, CachedImap, News, Search, NONE } |
Protected Member Functions | |
virtual QString | elidedLabelText (const QFontMetrics &metrics, unsigned int width) const |
void | setLabelTextElided (bool labelTextElided) |
Detailed Description
A folder tree node to be used with FolderTreeWidget.
Definition at line 225 of file foldertreewidget.h.
Member Enumeration Documentation
Folder type information Please note that this list should be kept in the order of items that one wants to be shown in the FolderTreeWidget, but better keep it in sync with the type in KFolderTree.
Enumerator | |
---|---|
Inbox | |
Outbox | |
SentMail | |
Trash | |
Drafts | |
Templates | |
Root | |
Calendar | |
Tasks | |
Journals | |
Contacts | |
Notes | |
Other |
Definition at line 245 of file foldertreewidget.h.
Protocol information associated to the item.
Please note that this list should be kept in the order of items that one wants to be shown in the foldertreewidget.
Enumerator | |
---|---|
Local | |
Imap | |
CachedImap | |
News | |
Search | |
NONE |
Definition at line 234 of file foldertreewidget.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a root-item.
Definition at line 334 of file foldertreewidget.cpp.
|
explicit |
Constructs a child-item.
Definition at line 348 of file foldertreewidget.cpp.
Member Function Documentation
|
inline |
Returns true if this item should bypass the size, total and unread display logic.
Normally when this item is a toplevel one and has no children it doesn't display the counts. With this flag set it displays the counts regardless of this. FIXME: Why this logic is actually hardwired here ?
Definition at line 438 of file foldertreewidget.h.
|
inline |
Returns the size in bytes of the children folders displayed in the special "DataSize" column.
Definition at line 364 of file foldertreewidget.h.
|
inline |
Returns the total message count for the children.
Displayed in the special "Total" column by FolderTreeWidget.
Definition at line 357 of file foldertreewidget.h.
|
inline |
Returns the unread message count for the children.
Displayed in the special "Unread" column by FolderTreeWidget.
Definition at line 350 of file foldertreewidget.h.
|
inline |
Returns the size in bytes of the folder, displayed in the special "DataSize" column.
If the size is meaningless (for folders that don't have storage, for example) then this function returns -1.
Definition at line 337 of file foldertreewidget.h.
|
protectedvirtual |
Returns the label text that has been elided if necessary to fit into the width
for the given font metrics
.
The default implementation elides the right of the label.
Reimplemented in KNCollectionViewItem.
Definition at line 619 of file foldertreewidget.cpp.
|
inline |
Returns the type of the folder.
Definition at line 396 of file foldertreewidget.h.
|
inline |
Returns the sate of the "Close to quota" warning for this folder.
Definition at line 289 of file foldertreewidget.h.
|
inline |
Returns the textual data for the "Label" column of the parent FolderTreeWidget.
Definition at line 300 of file foldertreewidget.h.
|
inline |
Returns true if the last painting operation had to elide the label text thus making it partially invisible.
This flag is meaningful only for currently visible items.
Definition at line 428 of file foldertreewidget.h.
|
virtual |
Operator used for item sorting.
Reimplemented in KNCollectionViewItem.
Definition at line 569 of file foldertreewidget.cpp.
|
inline |
Returns the protocol associated to the folder item.
Definition at line 379 of file foldertreewidget.h.
QString KPIM::FolderTreeWidgetItem::protocolDescription | ( | ) | const |
Returns a descriptive string of the folder's protocol.
Definition at line 364 of file foldertreewidget.cpp.
|
inline |
Sets whether this item should bypass the size, total and unread display logic.
Normally when this item is a toplevel one and has no children it doesn't display the counts. With this flag set it displays the counts regardless of this. FIXME: Why this logic is actually hardwired here ?
Definition at line 448 of file foldertreewidget.h.
void KPIM::FolderTreeWidgetItem::setDataSize | ( | qint64 | s | ) |
Sets the size in bytes of the folder to be displayed in the special "DataSize" column.
If s is -1 then the size becomes meaningless and is displayed as a dash in the view.
Definition at line 499 of file foldertreewidget.cpp.
|
inline |
Sets the type of the folder.
Definition at line 402 of file foldertreewidget.h.
void KPIM::FolderTreeWidgetItem::setIsCloseToQuota | ( | bool | closeToQuota | ) |
Sets the status of the close to quota warning.
Definition at line 557 of file foldertreewidget.cpp.
void KPIM::FolderTreeWidgetItem::setLabelText | ( | const QString & | label | ) |
Sets the textual data for the "Label" column of the parent FolderTreeWidget.
Definition at line 439 of file foldertreewidget.cpp.
|
inlineprotected |
This is called by FolderTreeWidgetItemLabelColumnDelegate to update the mLabelTextElided flag.
Definition at line 456 of file foldertreewidget.h.
|
inline |
Sets the protocol associated to the folder item.
Definition at line 385 of file foldertreewidget.h.
void KPIM::FolderTreeWidgetItem::setTotalCount | ( | int | totalCount | ) |
Sets the total message count to be displayed in the special "Total" column.
Definition at line 476 of file foldertreewidget.cpp.
void KPIM::FolderTreeWidgetItem::setUnreadCount | ( | int | unreadCount | ) |
Sets the unread message count to be displayed in the special "Unread" column.
Definition at line 453 of file foldertreewidget.cpp.
|
inline |
Returns the total message count.
Displayed in the special "Total" column by FolderTreeWidget.
Definition at line 324 of file foldertreewidget.h.
|
inline |
Returns the unread message count.
Displayed in the special "Unread" column by FolderTreeWidget.
Definition at line 312 of file foldertreewidget.h.
bool KPIM::FolderTreeWidgetItem::updateChildrenCounts | ( | ) |
Gathers the counts for the children.
Please note that this function is NOT recursive. It will simply scan the children and sum up their own+children counts. It will also NOT update any text. Returns true if any of the counts has actually changed.
Definition at line 393 of file foldertreewidget.cpp.
void KPIM::FolderTreeWidgetItem::updateColumn | ( | int | columnIndex | ) |
Triggers an update for the specified column of this item.
Definition at line 546 of file foldertreewidget.cpp.
void KPIM::FolderTreeWidgetItem::updateExpandedState | ( | ) |
This should be called whenever this item is expanded/collapsed.
It updates the text for the total, unread and size column. If the item is collapsed, that numbers will include the sum of the child numbers, otherwise it will just be the numbers of this item.
Definition at line 538 of file foldertreewidget.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:37 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.