knode
#include <foldertreewidget.h>
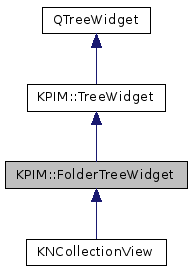
Signals | |
void | renamed (QTreeWidgetItem *item) |
![]() | |
void | columnVisibilityChanged (int logicalIndex) |
Public Member Functions | |
FolderTreeWidget (QWidget *parent, const char *name=0) | |
int | addDataSizeColumn (const QString &headerLabel) |
int | addLabelColumn (const QString &headerLabel) |
int | addTotalColumn (const QString &headerLabel) |
int | addUnreadColumn (const QString &headerLabel) |
const QColor & | closeToQuotaWarningColor () const |
int | dataSizeColumnIndex () const |
bool | dataSizeColumnVisible () const |
int | labelColumnIndex () const |
bool | labelColumnVisible () const |
void | setCloseToQuotaWarningColor (const QColor &clr) |
void | setUnreadCountColor (const QColor &clr) |
int | totalColumnIndex () const |
bool | totalColumnVisible () const |
int | unreadColumnIndex () const |
bool | unreadColumnVisible () const |
const QColor & | unreadCountColor () const |
void | updateColumnForItem (FolderTreeWidgetItem *item, int columnIndex) |
![]() | |
TreeWidget (QWidget *parent, const char *name=0) | |
int | addColumn (const QString &label, int headerAlignment=Qt::AlignLeft|Qt::AlignVCenter) |
virtual bool | fillHeaderContextMenu (KMenu *menu, const QPoint &clickPoint) |
QTreeWidgetItem * | firstItem () const |
bool | isColumnHidden (int logicalIndex) const |
QTreeWidgetItem * | lastItem () const |
bool | manualColumnHidingEnabled () const |
bool | restoreLayout (KConfigGroup &group, const QString &keyName=QString()) |
bool | restoreLayout (KConfig *config, const QString &groupName, const QString &keyName=QString()) |
bool | saveLayout (KConfigGroup &group, const QString &keyName=QString()) const |
bool | saveLayout (KConfig *config, const QString &groupName, const QString &keyName=QString()) const |
void | setColumnHidden (int logicalIndex, bool hide) |
bool | setColumnText (int columnIndex, const QString &label) |
void | setManualColumnHidingEnabled (bool enable) |
void | toggleColumn (int logicalIndex) |
Additional Inherited Members | |
![]() | |
virtual void | changeEvent (QEvent *e) |
Detailed Description
A tree widget useful for displaying a tree of folders containing messages.
This widget provides some facilities commonly used to display a tree of folders containing messages.
Besides the standard functionality of a KPIM::TreeWidget this implementation will handle four special columns: the "Label", the "Unread", the "Total" and the "DataSize" column.
You add a Label column with addLabelColumn(). It will fetch the text data from FolderTreeWidgetItem::labelText() instead of QTreeWidgetItem::text(). It will also display the unread message count if the "Unread" column is not visible.
You add a "Unread" column with addUnreadColumn(). It will fetch the numeric count of unread messages from FolderTreeWidgetItem::unreadCount() and it will automatically display it aligned to the right.
You add a "Total" column with addTotalColumn(). It will fetch the numeric total count of messages from FolderTreeWidgetItem::totalCount() and it will automatically display it aligned to the right.
You add a "DataSize" column with addSizeColumn(). It will fetch the size in bytes of the message folder from FolderTreeWidgetItem::size() and it will display it aligned to the right and with a suitable textual rappresentation.
You can set the color of the unread message count with setUnreadCountColor(). When the items are marked with the closeToQuota() warning then the text in the "Label" column is displayed with the color set by setCloseToQuotaWarningColor().
Definition at line 73 of file foldertreewidget.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a FolderTreeWidget instance.
Definition at line 216 of file foldertreewidget.cpp.
Member Function Documentation
int KPIM::FolderTreeWidget::addDataSizeColumn | ( | const QString & | headerLabel | ) |
Adds a special "DataSize" column to this view and returns its logical index.
If a "DataSize" column was already present in the view then nothing is added and the logical index of the existing column is returned.
Definition at line 289 of file foldertreewidget.cpp.
int KPIM::FolderTreeWidget::addLabelColumn | ( | const QString & | headerLabel | ) |
Adds a special "Label" column to this view and returns its logical index.
If a "Label" column was already present in the view then nothing is added and the logical index of the existing column is returned.
Definition at line 244 of file foldertreewidget.cpp.
int KPIM::FolderTreeWidget::addTotalColumn | ( | const QString & | headerLabel | ) |
Adds a special "Total" column to this view and returns its logical index.
If a "Total" column was already present in the view then nothing is added and the logical index of the existing column is returned.
Definition at line 261 of file foldertreewidget.cpp.
int KPIM::FolderTreeWidget::addUnreadColumn | ( | const QString & | headerLabel | ) |
Adds a special "Unread" column to this view and returns its logical index.
If a "Unread" column was already present in the view then nothing is added and the logical index of the existing column is returned.
Definition at line 275 of file foldertreewidget.cpp.
|
inline |
Returns the color used to display the "Label" column text when the item is marked as close to quota.
Definition at line 177 of file foldertreewidget.h.
|
inline |
Returns the logical index of the "DataSize" column or -1 if such a column has not been added (yet).
Definition at line 165 of file foldertreewidget.h.
bool KPIM::FolderTreeWidget::dataSizeColumnVisible | ( | ) | const |
Returns true if the widget contains a "DataSize" column and it's currently visible.
Definition at line 296 of file foldertreewidget.cpp.
|
inline |
Returns the logical index of the "Label" column or -1 if such a column has not been added (yet).
Definition at line 108 of file foldertreewidget.h.
bool KPIM::FolderTreeWidget::labelColumnVisible | ( | ) | const |
Returns true if the widget contains a "Label" column and it's currently visible.
Definition at line 254 of file foldertreewidget.cpp.
|
signal |
This signal is emitted when the label of item
has changed after an edition.
void KPIM::FolderTreeWidget::setCloseToQuotaWarningColor | ( | const QColor & | clr | ) |
Sets the color used to display the "Label" column text when the item is marked as close to quota.
Definition at line 316 of file foldertreewidget.cpp.
void KPIM::FolderTreeWidget::setUnreadCountColor | ( | const QColor & | clr | ) |
Sets the color used to display the unread message count in the "Label" column.
Definition at line 322 of file foldertreewidget.cpp.
|
inline |
Returns the logical index of the "Total" column or -1 if such a column has not been added (yet).
Definition at line 146 of file foldertreewidget.h.
bool KPIM::FolderTreeWidget::totalColumnVisible | ( | ) | const |
Returns true if the widget contains a "Total" column and it's currently visible.
Definition at line 268 of file foldertreewidget.cpp.
|
inline |
Returns the logical index of the "Unread" column or -1 if such a column has not been added (yet).
Definition at line 127 of file foldertreewidget.h.
bool KPIM::FolderTreeWidget::unreadColumnVisible | ( | ) | const |
Returns true if the widget contains an "Unread" column and it's currently visible.
Definition at line 282 of file foldertreewidget.cpp.
|
inline |
Returns the color used to display the unread message count in the "Label" column.
Definition at line 189 of file foldertreewidget.h.
void KPIM::FolderTreeWidget::updateColumnForItem | ( | FolderTreeWidgetItem * | item, |
int | columnIndex | ||
) |
Triggers an update for the specified column of the specified item.
Definition at line 303 of file foldertreewidget.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:37 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.