korganizer
#include <koeventview.h>
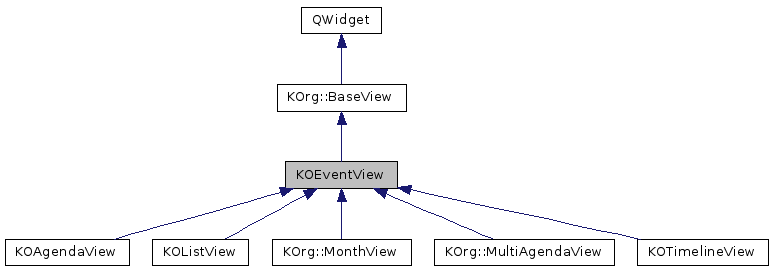
Public Types | |
enum | { BRIGHTNESS_FACTOR = 125 } |
Public Slots | |
void | defaultAction (const Akonadi::Item &incidence) |
void | focusChanged (QWidget *, QWidget *) |
![]() | |
virtual void | changeIncidenceDisplay (const Akonadi::Item &, Akonadi::IncidenceChanger::ChangeType)=0 |
virtual void | clearSelection () |
virtual void | dayPassed (const QDate &) |
virtual bool | eventDurationHint (QDateTime &startDt, QDateTime &endDt, bool &allDay) |
virtual void | flushView () |
virtual void | setIncidenceChanger (Akonadi::IncidenceChanger *changer) |
virtual void | showIncidences (const Akonadi::Item::List &incidenceList, const QDate &date)=0 |
virtual void | updateConfig () |
virtual void | updateView ()=0 |
Signals | |
void | datesSelected (const KCalCore::DateList datelist) |
void | shiftedEvent (const QDate &olddate, const QDate &ewdate) |
![]() | |
void | copyIncidenceSignal (const Akonadi::Item &) |
void | copyIncidenceToResourceSignal (const Akonadi::Item &, const QString &) |
void | cutIncidenceSignal (const Akonadi::Item &) |
void | deleteIncidenceSignal (const Akonadi::Item &) |
void | dissociateOccurrencesSignal (const Akonadi::Item &, const QDate &) |
void | editIncidenceSignal (const Akonadi::Item &) |
void | endMultiModify () |
void | incidenceSelected (const Akonadi::Item &, const QDate) |
void | moveIncidenceToResourceSignal (const Akonadi::Item &, const QString &) |
void | newEventSignal () |
void | newEventSignal (const QDate &) |
void | newEventSignal (const QDateTime &) |
void | newEventSignal (const QDateTime &, const QDateTime &) |
void | newJournalSignal (const QDate &) |
void | newSubTodoSignal (const Akonadi::Item &) |
void | newTodoSignal (const QDate &) |
void | pasteIncidenceSignal () |
void | showIncidenceSignal (const Akonadi::Item &) |
void | startMultiModify (const QString &) |
void | toggleAlarmSignal (const Akonadi::Item &) |
void | toggleTodoCompletedSignal (const Akonadi::Item &) |
Public Member Functions | |
KOEventView (QWidget *parent=0) | |
virtual | ~KOEventView () |
KOEventPopupMenu * | eventPopup () |
bool | isEventView () |
virtual int | maxDatesHint () const =0 |
QMenu * | newEventPopup () |
virtual void | setTypeAheadReceiver (QObject *o) |
int | showMoveRecurDialog (const Akonadi::Item &inc, const QDate &date) |
bool | supportsDateNavigation () const |
![]() | |
BaseView (QWidget *parent=0) | |
virtual | ~BaseView () |
KDateTime | actualEndDateTime () const |
KDateTime | actualStartDateTime () const |
virtual Akonadi::ETMCalendar::Ptr | calendar () |
EventViews::EventView::Changes | changes () const |
virtual Akonadi::Collection::Id | collectionId () const |
virtual int | currentDateCount () const =0 |
KDateTime | endDateTime () const |
virtual void | getHighlightMode (bool &highlightEvents, bool &highlightTodos, bool &highlightJournals) |
virtual bool | hasConfigurationDialog () const |
QByteArray | identifier () const |
virtual CalPrinterBase::PrintType | printType () const |
virtual void | restoreConfig (const KConfigGroup &configGroup) |
virtual void | saveConfig (KConfigGroup &configGroup) |
virtual KCalCore::DateList | selectedIncidenceDates ()=0 |
virtual Akonadi::Item::List | selectedIncidences ()=0 |
virtual QDateTime | selectionEnd () |
virtual QDateTime | selectionStart () |
virtual void | setCalendar (const Akonadi::ETMCalendar::Ptr &cal) |
virtual void | setChanges (EventViews::EventView::Changes changes) |
virtual void | setDateRange (const KDateTime &start, const KDateTime &end, const QDate &preferredMonth=QDate()) |
virtual void | setIdentifier (const QByteArray &identifier) |
virtual void | showConfigurationDialog (QWidget *parent) |
KDateTime | startDateTime () const |
virtual bool | supportsDateRangeSelection () |
virtual bool | supportsZoom () |
virtual bool | usesFullWindow () |
virtual BaseView * | viewAt (const QPoint &p) |
Static Public Member Functions | |
static bool | usesCompletedTodoPixmap (const Akonadi::Item &todo, const QDate &date) |
Protected Slots | |
void | popupCopy () |
void | popupCut () |
void | popupDelete () |
void | popupEdit () |
void | popupShow () |
virtual void | showNewEventPopup () |
![]() | |
virtual void | calendarReset () |
Protected Attributes | |
Akonadi::Item | mCurrentIncidence |
![]() | |
Akonadi::IncidenceChanger * | mChanger |
Additional Inherited Members | |
![]() | |
virtual QPair< KDateTime, KDateTime > | actualDateRange (const KDateTime &start, const KDateTime &end, const QDate &preferredMonth=QDate()) const |
virtual void | doRestoreConfig (const KConfigGroup &configGroup) |
virtual void | doSaveConfig (KConfigGroup &configGroup) |
virtual void | showDates (const QDate &start, const QDate &end, const QDate &preferredMonth=QDate())=0 |
Detailed Description
KOEventView is the abstract base class from which all other calendar views for event data are derived.
It provides methods for displaying appointments and events on one or more days. The actual number of days that a view actually supports is not defined by this abstract class; that is up to the classes that inherit from it. It also provides methods for updating the display, retrieving the currently selected event (or events), and the like.
Abstract class from which all event views are derived.
- See also
- KOListView, KOAgendaView, KOMonthView
Definition at line 54 of file koeventview.h.
Member Enumeration Documentation
anonymous enum |
Enumerator | |
---|---|
BRIGHTNESS_FACTOR |
Definition at line 58 of file koeventview.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a view.
- Parameters
-
cal is a pointer to the calendar object from which events will be retrieved for display. parent is the parent QWidget.
Definition at line 43 of file koeventview.cpp.
|
virtual |
Destructor.
Views will do view-specific cleanups here.
Definition at line 63 of file koeventview.cpp.
Member Function Documentation
|
signal |
When the view changes the dates that are selected in one way or another, this signal is emitted.
It should be connected back to the KDateNavigator object so that it changes appropriately, and any other objects that need to be aware that the list of selected dates has changed.
- Parameters
-
datelist the new list of selected dates
|
slot |
Performs the default action for an incidence, e.g.
open the event editor, when double-clicking an event in the agenda view.
Definition at line 164 of file koeventview.cpp.
KOEventPopupMenu * KOEventView::eventPopup | ( | ) |
Construct a standard context menu for an event.
Definition at line 69 of file koeventview.cpp.
Definition at line 224 of file koeventview.cpp.
|
inlinevirtual |
This view is a view for displaying events.
Reimplemented from KOrg::BaseView.
Definition at line 93 of file koeventview.h.
|
pure virtual |
provides a hint back to the caller on the maximum number of dates that the view supports.
A return value of 0 means no maximum.
Implemented in KOrg::MonthView, KOTimelineView, KOListView, KOrg::MultiAgendaView, and KOAgendaView.
QMenu * KOEventView::newEventPopup | ( | ) |
Construct a standard context that allows to create a new event.
Definition at line 99 of file koeventview.cpp.
|
protectedslot |
Definition at line 144 of file koeventview.cpp.
|
protectedslot |
Definition at line 137 of file koeventview.cpp.
|
protectedslot |
Definition at line 130 of file koeventview.cpp.
|
protectedslot |
Definition at line 123 of file koeventview.cpp.
|
protectedslot |
Definition at line 116 of file koeventview.cpp.
|
virtual |
Reimplemented in KOAgendaView, and KOrg::MonthView.
Definition at line 219 of file koeventview.cpp.
|
signal |
Emitted when an event is moved using the mouse in an agenda view (week / month).
int KOEventView::showMoveRecurDialog | ( | const Akonadi::Item & | inc, |
const QDate & | date | ||
) |
Definition at line 181 of file koeventview.cpp.
|
protectedvirtualslot |
Definition at line 151 of file koeventview.cpp.
|
inlinevirtual |
Returns true if the view supports navigation through the date navigator ( selecting a date range, changing month, changing year, etc.
)
Reimplemented from KOrg::BaseView.
Definition at line 119 of file koeventview.h.
|
static |
Definition at line 243 of file koeventview.cpp.
Member Data Documentation
|
protected |
Definition at line 156 of file koeventview.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:56:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.