korganizer
#include <baseview.h>
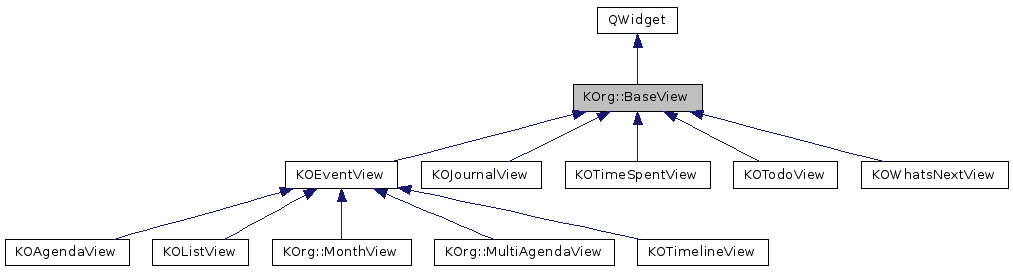
Public Slots | |
virtual void | changeIncidenceDisplay (const Akonadi::Item &, Akonadi::IncidenceChanger::ChangeType)=0 |
virtual void | clearSelection () |
virtual void | dayPassed (const QDate &) |
virtual bool | eventDurationHint (QDateTime &startDt, QDateTime &endDt, bool &allDay) |
virtual void | flushView () |
virtual void | setIncidenceChanger (Akonadi::IncidenceChanger *changer) |
virtual void | showIncidences (const Akonadi::Item::List &incidenceList, const QDate &date)=0 |
virtual void | updateConfig () |
virtual void | updateView ()=0 |
Signals | |
void | copyIncidenceSignal (const Akonadi::Item &) |
void | copyIncidenceToResourceSignal (const Akonadi::Item &, const QString &) |
void | cutIncidenceSignal (const Akonadi::Item &) |
void | deleteIncidenceSignal (const Akonadi::Item &) |
void | dissociateOccurrencesSignal (const Akonadi::Item &, const QDate &) |
void | editIncidenceSignal (const Akonadi::Item &) |
void | endMultiModify () |
void | incidenceSelected (const Akonadi::Item &, const QDate) |
void | moveIncidenceToResourceSignal (const Akonadi::Item &, const QString &) |
void | newEventSignal () |
void | newEventSignal (const QDate &) |
void | newEventSignal (const QDateTime &) |
void | newEventSignal (const QDateTime &, const QDateTime &) |
void | newJournalSignal (const QDate &) |
void | newSubTodoSignal (const Akonadi::Item &) |
void | newTodoSignal (const QDate &) |
void | pasteIncidenceSignal () |
void | showIncidenceSignal (const Akonadi::Item &) |
void | startMultiModify (const QString &) |
void | toggleAlarmSignal (const Akonadi::Item &) |
void | toggleTodoCompletedSignal (const Akonadi::Item &) |
Public Member Functions | |
BaseView (QWidget *parent=0) | |
virtual | ~BaseView () |
KDateTime | actualEndDateTime () const |
KDateTime | actualStartDateTime () const |
virtual Akonadi::ETMCalendar::Ptr | calendar () |
EventViews::EventView::Changes | changes () const |
virtual Akonadi::Collection::Id | collectionId () const |
virtual int | currentDateCount () const =0 |
KDateTime | endDateTime () const |
virtual void | getHighlightMode (bool &highlightEvents, bool &highlightTodos, bool &highlightJournals) |
virtual bool | hasConfigurationDialog () const |
QByteArray | identifier () const |
virtual bool | isEventView () |
virtual CalPrinterBase::PrintType | printType () const |
virtual void | restoreConfig (const KConfigGroup &configGroup) |
virtual void | saveConfig (KConfigGroup &configGroup) |
virtual KCalCore::DateList | selectedIncidenceDates ()=0 |
virtual Akonadi::Item::List | selectedIncidences ()=0 |
virtual QDateTime | selectionEnd () |
virtual QDateTime | selectionStart () |
virtual void | setCalendar (const Akonadi::ETMCalendar::Ptr &cal) |
virtual void | setChanges (EventViews::EventView::Changes changes) |
virtual void | setDateRange (const KDateTime &start, const KDateTime &end, const QDate &preferredMonth=QDate()) |
virtual void | setIdentifier (const QByteArray &identifier) |
virtual void | showConfigurationDialog (QWidget *parent) |
KDateTime | startDateTime () const |
virtual bool | supportsDateNavigation () const |
virtual bool | supportsDateRangeSelection () |
virtual bool | supportsZoom () |
virtual bool | usesFullWindow () |
virtual BaseView * | viewAt (const QPoint &p) |
Protected Slots | |
virtual void | calendarReset () |
Protected Member Functions | |
virtual QPair< KDateTime, KDateTime > | actualDateRange (const KDateTime &start, const KDateTime &end, const QDate &preferredMonth=QDate()) const |
virtual void | doRestoreConfig (const KConfigGroup &configGroup) |
virtual void | doSaveConfig (KConfigGroup &configGroup) |
virtual void | showDates (const QDate &start, const QDate &end, const QDate &preferredMonth=QDate())=0 |
Protected Attributes | |
Akonadi::IncidenceChanger * | mChanger |
Detailed Description
This class provides an interface for all views being displayed within the main calendar view.
It has functions to update the view, to specify date range and other display parameter and to return selected objects. An important class, which inherits KOBaseView is KOEventView, which provides the interface for all views of event data like the agenda or the month view.
Base class for calendar views
- See also
- KOTodoView, KOEventView, KOListView, KOAgendaView, KOMonthView
Definition at line 51 of file baseview.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a view.
- Parameters
-
cal Pointer to the calendar object from which events will be retrieved for display. parent parent widget.
Definition at line 58 of file baseview.cpp.
|
virtual |
Member Function Documentation
|
protectedvirtual |
From the requested date range (passed via setDateRange()), calculates the adjusted date range actually displayed by the view, depending on the view's supported range (e.g., a month view always displays one month).
The default implementation returns the range unmodified
Definition at line 221 of file baseview.cpp.
KDateTime BaseView::actualEndDateTime | ( | ) | const |
Definition at line 144 of file baseview.cpp.
KDateTime BaseView::actualStartDateTime | ( | ) | const |
Definition at line 139 of file baseview.cpp.
|
virtual |
Return calendar object of this view.
Definition at line 80 of file baseview.cpp.
|
protectedvirtualslot |
Definition at line 217 of file baseview.cpp.
|
pure virtualslot |
Updates the current display to reflect the changes to one particular incidence.
Implemented in KOTimelineView.
EventViews::EventView::Changes BaseView::changes | ( | ) | const |
Returns if there are pending changes and a redraw is needed.
Definition at line 234 of file baseview.cpp.
|
virtualslot |
Clear selection.
The incidenceSelected signal is not emitted.
Definition at line 181 of file baseview.cpp.
|
inlinevirtual |
Reimplemented in KOrg::MultiAgendaView.
Definition at line 202 of file baseview.h.
|
signal |
instructs the receiver to copy the incidence
|
signal |
Copy the incidence to the specified resource.
|
pure virtual |
Returns the number of currently shown dates.
A return value of 0 means no idea.
Implemented in KOListView, KOTimelineView, KOTodoView, KOJournalView, KOAgendaView, KOrg::MultiAgendaView, KOTimeSpentView, KOrg::MonthView, and KOWhatsNextView.
|
signal |
instructs the receiver to cut the Incidence
|
virtualslot |
Definition at line 90 of file baseview.cpp.
|
signal |
instructs the receiver to delete the Incidence in some manner; some possibilities include automatically, with a confirmation dialog box, etc.
Doesn't make sense to connect to more than one receiver.
|
signal |
Dissociate from a recurring incidence the occurrence on the given date to a new incidence or dissociate all occurrences from the given date onwards.
|
protectedvirtual |
reimplement to read view-specific settings
Definition at line 173 of file baseview.cpp.
|
protectedvirtual |
reimplement to write view specific settings
Definition at line 177 of file baseview.cpp.
|
signal |
instructs the receiver to begin editing the incidence specified in some manner.
Doesn't make sense to connect to more than one receiver.
KDateTime BaseView::endDateTime | ( | ) | const |
Definition at line 134 of file baseview.cpp.
|
signal |
|
virtualslot |
Sets the default start/end date/time for new events.
Return true if anything was changed
Reimplemented in KOTimelineView, KOAgendaView, KOrg::MonthView, and KOrg::MultiAgendaView.
Definition at line 185 of file baseview.cpp.
|
virtualslot |
Write all unsaved data back to calendar store.
Definition at line 100 of file baseview.cpp.
|
virtual |
Returns which incidence types should used to embolden day numbers in the date navigator when this view is selected.
BaseView provides a default implementation that only highlights events because that's how the behaviour has always been, and most views are event orientated, even one or two which don't inherit KOEventView are about events (timespent).
This function writes to these 3 parameters the result, the original value is ignored
Reimplemented in KOJournalView, and KOTodoView.
Definition at line 193 of file baseview.cpp.
|
virtual |
Reimplemented in KOrg::MultiAgendaView.
Definition at line 113 of file baseview.cpp.
QByteArray BaseView::identifier | ( | ) | const |
Definition at line 153 of file baseview.cpp.
|
signal |
|
virtual |
Returns if this view is a view for displaying events.
Reimplemented in KOEventView.
Definition at line 85 of file baseview.cpp.
|
signal |
Move the incidence to the specified resource.
|
signal |
instructs the receiver to create a new event in given collection.
Doesn't make sense to connect to more than one receiver.
|
signal |
instructs the receiver to create a new event with the specified beginning time.
Doesn't make sense to connect to more than one receiver.
|
signal |
instructs the receiver to create a new event with the specified beginning time.
Doesn't make sense to connect to more than one receiver.
|
signal |
instructs the receiver to create a new event, with the specified beginning end ending times.
Doesn't make sense to connect to more than one receiver.
|
signal |
|
signal |
|
signal |
|
signal |
instructs the receiver to paste the incidence
|
virtual |
Reimplemented in KOListView, KOTodoView, KOJournalView, KOrg::MonthView, KOTimelineView, KOAgendaView, and KOWhatsNextView.
Definition at line 75 of file baseview.cpp.
|
virtual |
reads the view configuration.
View-specific configuration can be restored via doRestoreConfig()
- Parameters
-
configGroup the group to read settings from
- See also
- doRestoreConfig()
Reimplemented in KOrg::MultiAgendaView.
Definition at line 163 of file baseview.cpp.
|
virtual |
writes out the view configuration.
View-specific configuration can be saved via doSaveConfig()
- Parameters
-
configGroup the group to store settings in
- See also
- doSaveConfig()
Reimplemented in KOrg::MultiAgendaView.
Definition at line 168 of file baseview.cpp.
|
pure virtual |
Returns a list of the dates of selected events.
Most views can probably only select a single event at a time, but some may be able to select more than one.
Implemented in KOListView, KOAgendaView, KOJournalView, KOTimeSpentView, KOTimelineView, KOTodoView, KOrg::MonthView, KOrg::MultiAgendaView, and KOWhatsNextView.
|
pure virtual |
- Returns
- a list of selected events. Most views can probably only select a single event at a time, but some may be able to select more than one.
Implemented in KOListView, KOAgendaView, KOJournalView, KOTimelineView, KOTodoView, KOTimeSpentView, KOrg::MultiAgendaView, KOrg::MonthView, and KOWhatsNextView.
|
inlinevirtual |
Returns the end of the selection, or an invalid QDateTime if there is no selection or the view doesn't support selecting cells.
Reimplemented in KOAgendaView, and KOrg::MonthView.
Definition at line 99 of file baseview.h.
|
inlinevirtual |
Returns the start of the selection, or an invalid QDateTime if there is no selection or the view doesn't support selecting cells.
Reimplemented in KOAgendaView, and KOrg::MonthView.
Definition at line 93 of file baseview.h.
|
virtual |
Reimplemented in KOAgendaView, KOrg::MonthView, KOListView, KOTimelineView, KOJournalView, KOTimeSpentView, KOrg::MultiAgendaView, KOTodoView, and KOWhatsNextView.
Definition at line 68 of file baseview.cpp.
|
virtual |
Notifies the view that there are pending changes so a redraw is needed.
- Parameters
-
changes Types of changes that were made
Reimplemented in KOAgendaView, and KOrg::MultiAgendaView.
Definition at line 229 of file baseview.cpp.
|
virtual |
Show incidences for the given date range.
The date range actually shown may be different from the requested range, depending on the particular requirements of the view.
- Parameters
-
start Start of date range. end End of date range.
Reimplemented in KOAgendaView, KOrg::MultiAgendaView, and KOrg::MonthView.
Definition at line 118 of file baseview.cpp.
|
virtual |
Definition at line 158 of file baseview.cpp.
|
virtualslot |
Assign a new incidence change helper object.
Reimplemented in KOrg::MonthView, KOListView, and KOTimelineView.
Definition at line 95 of file baseview.cpp.
|
virtual |
Reimplemented in KOrg::MultiAgendaView.
Definition at line 149 of file baseview.cpp.
|
protectedpure virtual |
Implemented in KOTimelineView.
|
pure virtualslot |
Shows given incidences.
Depending on the actual view it might not be possible to show all given events.
- Parameters
-
incidenceList a list of incidences to show. date is the QDate on which the incidences are being shown.
Implemented in KOTimelineView.
|
signal |
instructs the receiver to show the incidence in read-only mode.
KDateTime BaseView::startDateTime | ( | ) | const |
Definition at line 129 of file baseview.cpp.
|
signal |
|
inlinevirtual |
Returns true if the view supports navigation through the date navigator ( selecting a date range, changing month, changing year, etc.
)
Reimplemented in KOEventView, and KOWhatsNextView.
Definition at line 200 of file baseview.h.
|
virtual |
returns whether this view supports date range selection Base implementation returns true.
Reimplemented in KOTodoView, and KOrg::MonthView.
Definition at line 212 of file baseview.cpp.
|
virtual |
returns whether this view supports zoom.
Base implementation returns false.
Definition at line 207 of file baseview.cpp.
|
signal |
instructs the receiver to toggle the alarms of the Incidence.
|
signal |
instructs the receiver to toggle the completion state of the Incidence (which must be a Todo type).
|
virtualslot |
Re-reads the KOrganizer configuration and picks up relevant changes which are applicable to the view.
Definition at line 109 of file baseview.cpp.
|
pure virtualslot |
Updates the current display to reflect changes that may have happened in the calendar since the last display refresh.
Implemented in KOTimelineView.
|
virtual |
returns whether this view should be displayed full window.
Base implementation returns false.
Reimplemented in KOTodoView, and KOrg::MonthView.
Definition at line 202 of file baseview.cpp.
|
virtual |
returns the view at the given widget coordinate.
This is usually the view itself, except for composite views, where a subview will be returned. The default implementation returns this
.
Definition at line 104 of file baseview.cpp.
Member Data Documentation
|
protected |
Definition at line 397 of file baseview.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:56:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.