messageviewer
#include <viewer.h>
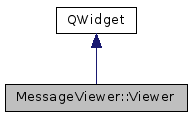
Public Types | |
enum | AttachmentAction { Open = 1, OpenWith = 2, View = 3, Save = 4, Properties = 5, ChiasmusEncrypt = 6, Delete = 7, Edit = 8, Copy = 9, ScrollTo = 10 } |
enum | ForceDisplayTo { Unknown = 0, Text = 1, Html = 2 } |
enum | ResourceOnlineMode { AllResources = 0, SelectedResource = 1 } |
enum | UpdateMode { Force = 0, Delayed } |
Public Slots | |
void | slotAttachmentSaveAll () |
void | slotAttachmentSaveAs () |
void | slotChangeDisplayMail (Viewer::ForceDisplayTo, bool) |
void | slotFind () |
void | slotJumpDown () |
void | slotSaveMessage () |
void | slotScrollDown (int pixels=10) |
void | slotScrollNext () |
void | slotScrollPrior () |
void | slotScrollUp (int pixels=10) |
void | slotShowMessageSource () |
void | slotTranslate () |
void | slotZoomIn () |
void | slotZoomOut () |
void | slotZoomReset () |
Signals | |
void | deleteMessage (const Akonadi::Item &) |
void | itemRemoved () |
void | makeResourceOnline (MessageViewer::Viewer::ResourceOnlineMode mode) |
void | moveMessageToTrash () |
void | popupMenu (const Akonadi::Item &msg, const KUrl &url, const KUrl &imageUrl, const QPoint &mousePos) |
void | replaceMsgByUnencryptedVersion () |
void | requestConfigSync () |
void | showMessage (KMime::Message::Ptr message, const QString &encoding) |
void | showReader (KMime::Content *aMsgPart, bool aHTML, const QString &encoding) |
void | showStatusBarMessage (const QString &message) |
void | urlClicked (const Akonadi::Item &, const KUrl &) |
Public Member Functions | |
Viewer (QWidget *parent, QWidget *mainWindow=0, KActionCollection *actionCollection=0, Qt::WindowFlags f=0) | |
virtual | ~Viewer () |
bool | adblockEnabled () const |
void | addMessageLoadedHandler (AbstractMessageLoadedHandler *handler) |
bool | atBottom () const |
const AttachmentStrategy * | attachmentStrategy () const |
KAction * | blockImage () |
void | clear (UpdateMode updateMode=Delayed) |
void | clearSelection () |
QWidget * | configWidget () |
KAction * | copyAction () |
KAction * | copyImageLocation () |
void | copySelectionToClipboard () |
KAction * | copyURLAction () |
CSSHelper * | cssHelper () const |
void | deleteMessage () |
void | displaySplashPage (const QString &info) |
void | enableMessageDisplay () |
KAction * | expandShortUrlAction () |
KAction * | findInMessageAction () |
HeaderStrategy * | headerStrategy () const |
HeaderStyle * | headerStyle () const |
bool | htmlLoadExternal () const |
bool | htmlLoadExtOverride () const |
bool | htmlMail () const |
bool | htmlOverride () const |
KUrl | imageUrlClicked () const |
bool | isAShortUrl (const KUrl &url) const |
bool | isFixedFont () const |
QWidget * | mainWindow () |
KMime::Message::Ptr | message () const |
Akonadi::Item | messageItem () const |
QString | messagePath () const |
QAbstractItemModel * | messageTreeModel () const |
KAction * | openBlockableItems () |
QString | overrideEncoding () const |
void | print () |
void | printMessage (const Akonadi::Item &msg) |
void | printPreview () |
void | printPreviewMessage (const Akonadi::Item &message) |
void | readConfig () |
void | removeMessageLoadedHandler (AbstractMessageLoadedHandler *handler) |
KAction * | resetMessageDisplayFormatAction () |
KAction * | saveAsAction () |
void | saveMainFrameScreenshotInFile (const QString &filename) |
KAction * | saveMessageDisplayFormatAction () |
Qt::ScrollBarPolicy | scrollBarPolicy (Qt::Orientation orientation) const |
void | selectAll () |
KAction * | selectAllAction () |
QString | selectedText () const |
void | setAppName (const QString &appName) |
void | setAttachmentStrategy (const AttachmentStrategy *strategy) |
void | setDecryptMessageOverwrite (bool overwrite=true) |
void | setExternalWindow (bool b) |
void | setHeaderStyleAndStrategy (HeaderStyle *style, HeaderStrategy *strategy) |
void | setHtmlLoadExtOverride (bool override) |
void | setHtmlOverride (bool override) |
void | setMessage (KMime::Message::Ptr message, UpdateMode updateMode=Delayed) |
void | setMessageItem (const Akonadi::Item &item, UpdateMode updateMode=Delayed) |
void | setMessagePart (KMime::Content *aMsgPart) |
void | setMessagePath (const QString &path) |
void | setOverrideEncoding (const QString &encoding) |
void | setPrinting (bool enable) |
void | setScrollBarPolicy (Qt::Orientation orientation, Qt::ScrollBarPolicy policy) |
void | setUseFixedFont (bool useFixedFont) |
void | setZoomFactor (qreal zoomFactor) |
void | setZoomTextOnly (bool textOnly) |
KAction * | speakTextAction () |
KToggleAction * | toggleFixFontAction () |
KToggleAction * | toggleMimePartTreeAction () |
KAction * | translateAction () |
void | update (UpdateMode updateMode=Delayed) |
KUrl | urlClicked () const |
KAction * | urlOpenAction () |
KAction * | viewSourceAction () |
void | writeConfig (bool withSync=true) |
bool | zoomTextOnly () const |
Static Public Member Functions | |
static Akonadi::ItemFetchJob * | createFetchJob (const Akonadi::Item &item) |
Protected Member Functions | |
virtual void | closeEvent (QCloseEvent *) |
virtual bool | event (QEvent *e) |
virtual void | resizeEvent (QResizeEvent *) |
Protected Attributes | |
ViewerPrivate *const | d_ptr |
Detailed Description
This is the main widget for the viewer.
See the documentation of ViewerPrivate for implementation details. See Mainpage.dox for an overview of the classes in the messageviewer library.
Member Enumeration Documentation
Constructor & Destructor Documentation
|
explicit |
Create a mail viewer widget.
- Parameters
-
parent parent widget mainWindow the application's main window actionCollection the action collection where the widget's actions will belong to f window flags
Definition at line 50 of file viewer.cpp.
|
virtual |
Definition at line 58 of file viewer.cpp.
Member Function Documentation
bool MessageViewer::Viewer::adblockEnabled | ( | ) | const |
Definition at line 665 of file viewer.cpp.
void MessageViewer::Viewer::addMessageLoadedHandler | ( | AbstractMessageLoadedHandler * | handler | ) |
Adds a handler
for actions that will be executed when the message has been loaded into the view.
Definition at line 541 of file viewer.cpp.
bool MessageViewer::Viewer::atBottom | ( | ) | const |
Returns true if the message view is scrolled to the bottom.
Definition at line 207 of file viewer.cpp.
const AttachmentStrategy * MessageViewer::Viewer::attachmentStrategy | ( | ) | const |
Definition at line 349 of file viewer.cpp.
KAction * MessageViewer::Viewer::blockImage | ( | ) |
Definition at line 659 of file viewer.cpp.
|
inline |
void MessageViewer::Viewer::clearSelection | ( | ) |
Definition at line 570 of file viewer.cpp.
|
protectedvirtual |
Some necessary event handling.
Definition at line 170 of file viewer.cpp.
QWidget * MessageViewer::Viewer::configWidget | ( | ) |
Get an instance for the configuration widget.
The caller has the ownership and must delete the widget. See also configObject(); The caller should also call the widget's slotSettingsChanged() if the configuration has changed.
Definition at line 304 of file viewer.cpp.
KAction * MessageViewer::Viewer::copyAction | ( | ) |
Definition at line 436 of file viewer.cpp.
KAction * MessageViewer::Viewer::copyImageLocation | ( | ) |
Definition at line 448 of file viewer.cpp.
void MessageViewer::Viewer::copySelectionToClipboard | ( | ) |
Definition at line 577 of file viewer.cpp.
KAction * MessageViewer::Viewer::copyURLAction | ( | ) |
Definition at line 430 of file viewer.cpp.
|
static |
Create an item fetch job that is suitable for using to fetch the message item that will be displayed on this viewer.
It will set the correct fetch scope. You still need to connect to the job's result signal.
Definition at line 525 of file viewer.cpp.
CSSHelper * MessageViewer::Viewer::cssHelper | ( | ) | const |
Definition at line 374 of file viewer.cpp.
void MessageViewer::Viewer::deleteMessage | ( | ) |
Initiates a delete, by sending a signal to delete the message item.
Definition at line 558 of file viewer.cpp.
|
signal |
void MessageViewer::Viewer::displaySplashPage | ( | const QString & | info | ) |
Display a generic HTML splash page instead of a message.
- Parameters
-
info - the text to be displayed in HTML format
Definition at line 121 of file viewer.cpp.
void MessageViewer::Viewer::enableMessageDisplay | ( | ) |
Enable the displaying of messages again after an splash (or other) page was displayed.
Definition at line 127 of file viewer.cpp.
|
protectedvirtual |
Watch for palette changes.
Definition at line 324 of file viewer.cpp.
KAction * MessageViewer::Viewer::expandShortUrlAction | ( | ) |
Definition at line 686 of file viewer.cpp.
KAction * MessageViewer::Viewer::findInMessageAction | ( | ) |
Definition at line 619 of file viewer.cpp.
HeaderStrategy * MessageViewer::Viewer::headerStrategy | ( | ) | const |
Definition at line 399 of file viewer.cpp.
HeaderStyle * MessageViewer::Viewer::headerStyle | ( | ) | const |
Definition at line 405 of file viewer.cpp.
bool MessageViewer::Viewer::htmlLoadExternal | ( | ) | const |
Is loading ext.
references to be supported? Takes into account override
Definition at line 274 of file viewer.cpp.
bool MessageViewer::Viewer::htmlLoadExtOverride | ( | ) | const |
Get the load external references override setting.
Definition at line 262 of file viewer.cpp.
bool MessageViewer::Viewer::htmlMail | ( | ) | const |
Is html mail to be supported? Takes into account override.
Definition at line 268 of file viewer.cpp.
bool MessageViewer::Viewer::htmlOverride | ( | ) | const |
Get the html override setting.
Definition at line 244 of file viewer.cpp.
KUrl MessageViewer::Viewer::imageUrlClicked | ( | ) | const |
Definition at line 490 of file viewer.cpp.
bool MessageViewer::Viewer::isAShortUrl | ( | const KUrl & | url | ) | const |
Definition at line 680 of file viewer.cpp.
bool MessageViewer::Viewer::isFixedFont | ( | ) | const |
Definition at line 280 of file viewer.cpp.
|
signal |
Emitted when the item, previously set with setMessageItem, has been removed.
QWidget * MessageViewer::Viewer::mainWindow | ( | ) |
Definition at line 292 of file viewer.cpp.
|
signal |
KMime::Message::Ptr MessageViewer::Viewer::message | ( | ) | const |
Returns the current message displayed in the viewer.
Definition at line 312 of file viewer.cpp.
Akonadi::Item MessageViewer::Viewer::messageItem | ( | ) | const |
Returns the current message item displayed in the viewer.
Definition at line 318 of file viewer.cpp.
QString MessageViewer::Viewer::messagePath | ( | ) | const |
The path to the message in terms of Akonadi collection hierarchy.
Definition at line 109 of file viewer.cpp.
QAbstractItemModel * MessageViewer::Viewer::messageTreeModel | ( | ) | const |
A QAIM tree model of the message structure.
Definition at line 520 of file viewer.cpp.
|
signal |
KAction * MessageViewer::Viewer::openBlockableItems | ( | ) |
Definition at line 674 of file viewer.cpp.
QString MessageViewer::Viewer::overrideEncoding | ( | ) | const |
Definition at line 361 of file viewer.cpp.
|
signal |
The user presses the right mouse button.
'url' may be 0.
void MessageViewer::Viewer::print | ( | ) |
Print the currently displayed message.
Definition at line 151 of file viewer.cpp.
void MessageViewer::Viewer::printMessage | ( | const Akonadi::Item & | msg | ) |
Sets a message as the current one and print it immediately.
- Parameters
-
message the message to display and print
Definition at line 133 of file viewer.cpp.
void MessageViewer::Viewer::printPreview | ( | ) |
Definition at line 145 of file viewer.cpp.
void MessageViewer::Viewer::printPreviewMessage | ( | const Akonadi::Item & | message | ) |
Definition at line 139 of file viewer.cpp.
void MessageViewer::Viewer::readConfig | ( | ) |
Definition at line 514 of file viewer.cpp.
void MessageViewer::Viewer::removeMessageLoadedHandler | ( | AbstractMessageLoadedHandler * | handler | ) |
Removes the handler
for actions that will be executed when the message has been loaded into the view.
Definition at line 551 of file viewer.cpp.
|
signal |
Emitted after parsing of a message to have it stored in unencrypted state in it's folder.
|
signal |
KAction * MessageViewer::Viewer::resetMessageDisplayFormatAction | ( | ) |
Definition at line 647 of file viewer.cpp.
|
protectedvirtual |
Definition at line 157 of file viewer.cpp.
KAction * MessageViewer::Viewer::saveAsAction | ( | ) |
Definition at line 460 of file viewer.cpp.
void MessageViewer::Viewer::saveMainFrameScreenshotInFile | ( | const QString & | filename | ) |
Definition at line 653 of file viewer.cpp.
KAction * MessageViewer::Viewer::saveMessageDisplayFormatAction | ( | ) |
Definition at line 641 of file viewer.cpp.
Qt::ScrollBarPolicy MessageViewer::Viewer::scrollBarPolicy | ( | Qt::Orientation | orientation | ) | const |
Returns the scrollbar policy for the scrollbar defined by orientation.
- See also
- setScrollBarPolicy()
void MessageViewer::Viewer::selectAll | ( | ) |
Definition at line 564 of file viewer.cpp.
KAction * MessageViewer::Viewer::selectAllAction | ( | ) |
Definition at line 393 of file viewer.cpp.
QString MessageViewer::Viewer::selectedText | ( | ) | const |
Definition at line 231 of file viewer.cpp.
void MessageViewer::Viewer::setAppName | ( | const QString & | appName | ) |
Set the application name that is shown when the splash screen is active.
- Parameters
-
appName - A QString that is set to the calling application name.
Definition at line 256 of file viewer.cpp.
void MessageViewer::Viewer::setAttachmentStrategy | ( | const AttachmentStrategy * | strategy | ) |
Definition at line 355 of file viewer.cpp.
void MessageViewer::Viewer::setDecryptMessageOverwrite | ( | bool | overwrite = true | ) |
Enforce message decryption.
Definition at line 298 of file viewer.cpp.
void MessageViewer::Viewer::setExternalWindow | ( | bool | b | ) |
Definition at line 418 of file viewer.cpp.
void MessageViewer::Viewer::setHeaderStyleAndStrategy | ( | HeaderStyle * | style, |
HeaderStrategy * | strategy | ||
) |
Definition at line 411 of file viewer.cpp.
void MessageViewer::Viewer::setHtmlLoadExtOverride | ( | bool | override | ) |
Override default load external references setting.
Definition at line 250 of file viewer.cpp.
void MessageViewer::Viewer::setHtmlOverride | ( | bool | override | ) |
Override default html mail setting.
Definition at line 238 of file viewer.cpp.
void MessageViewer::Viewer::setMessage | ( | KMime::Message::Ptr | message, |
UpdateMode | updateMode = Delayed |
||
) |
Set the message that shall be shown.
- Parameters
-
msg - the message to be shown. If 0, an empty page is displayed. updateMode - update the display immediately or not. See UpdateMode.
Definition at line 86 of file viewer.cpp.
void MessageViewer::Viewer::setMessageItem | ( | const Akonadi::Item & | item, |
UpdateMode | updateMode = Delayed |
||
) |
Set the Akonadi item that will be displayed.
- Parameters
-
item - the Akonadi item to be displayed. If it doesn't hold a mail (KMime::Message::Ptr as payload data), an empty page is shown. updateMode - update the display immediately or not. See UpdateMode.
Definition at line 95 of file viewer.cpp.
void MessageViewer::Viewer::setMessagePart | ( | KMime::Content * | aMsgPart | ) |
Instead of settings a message to be shown sets a message part to be shown.
Definition at line 502 of file viewer.cpp.
void MessageViewer::Viewer::setMessagePath | ( | const QString & | path | ) |
Set the path to the message in terms of Akonadi collection hierarchy.
Definition at line 115 of file viewer.cpp.
void MessageViewer::Viewer::setOverrideEncoding | ( | const QString & | encoding | ) |
Definition at line 367 of file viewer.cpp.
void MessageViewer::Viewer::setPrinting | ( | bool | enable | ) |
Definition at line 472 of file viewer.cpp.
void MessageViewer::Viewer::setScrollBarPolicy | ( | Qt::Orientation | orientation, |
Qt::ScrollBarPolicy | policy | ||
) |
Sets the scrollbar policy for the scrollbar defined by orientation to policy.
- See also
- scrollBarPolicy()
Definition at line 535 of file viewer.cpp.
void MessageViewer::Viewer::setUseFixedFont | ( | bool | useFixedFont | ) |
Definition at line 286 of file viewer.cpp.
void MessageViewer::Viewer::setZoomFactor | ( | qreal | zoomFactor | ) |
Definition at line 583 of file viewer.cpp.
void MessageViewer::Viewer::setZoomTextOnly | ( | bool | textOnly | ) |
Definition at line 607 of file viewer.cpp.
|
signal |
Emitted when the message should be shown in a separate window.
|
signal |
Emitted when the content should be shown in a separate window.
|
signal |
Emitted when a status bar message is shown.
Note that the status bar message is also set to KPIM::BroadcastStatus in addition.
|
slot |
Definition at line 183 of file viewer.cpp.
|
slot |
Definition at line 177 of file viewer.cpp.
|
slot |
Definition at line 625 of file viewer.cpp.
|
slot |
Definition at line 336 of file viewer.cpp.
|
slot |
Definition at line 213 of file viewer.cpp.
|
slot |
Definition at line 189 of file viewer.cpp.
|
slot |
Definition at line 201 of file viewer.cpp.
|
slot |
Definition at line 225 of file viewer.cpp.
|
slot |
Definition at line 219 of file viewer.cpp.
|
slot |
HTML Widget scrollbar and layout handling.
Scrolling always happens in the direction of the slot that is called. I.e. the methods take the absolute value of
Definition at line 195 of file viewer.cpp.
|
slot |
Definition at line 508 of file viewer.cpp.
|
slot |
Definition at line 342 of file viewer.cpp.
|
slot |
Definition at line 595 of file viewer.cpp.
|
slot |
Definition at line 601 of file viewer.cpp.
|
slot |
Definition at line 589 of file viewer.cpp.
KAction * MessageViewer::Viewer::speakTextAction | ( | ) |
Definition at line 442 of file viewer.cpp.
KToggleAction * MessageViewer::Viewer::toggleFixFontAction | ( | ) |
Definition at line 381 of file viewer.cpp.
KToggleAction * MessageViewer::Viewer::toggleMimePartTreeAction | ( | ) |
Definition at line 387 of file viewer.cpp.
KAction * MessageViewer::Viewer::translateAction | ( | ) |
Definition at line 454 of file viewer.cpp.
void MessageViewer::Viewer::update | ( | UpdateMode | updateMode = Delayed | ) |
Definition at line 496 of file viewer.cpp.
KUrl MessageViewer::Viewer::urlClicked | ( | ) | const |
Definition at line 484 of file viewer.cpp.
|
signal |
The message viewer handles some types of urls itself, most notably http(s) and ftp(s).
When it can't handle the url it will emit this signal.
KAction * MessageViewer::Viewer::urlOpenAction | ( | ) |
Definition at line 466 of file viewer.cpp.
KAction * MessageViewer::Viewer::viewSourceAction | ( | ) |
Definition at line 424 of file viewer.cpp.
void MessageViewer::Viewer::writeConfig | ( | bool | withSync = true | ) |
Definition at line 478 of file viewer.cpp.
bool MessageViewer::Viewer::zoomTextOnly | ( | ) | const |
Definition at line 613 of file viewer.cpp.
Member Data Documentation
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:55:58 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.