messageviewer
#include <viewer_p.h>
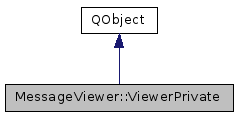
Signals | |
void | changeDisplayMail (Viewer::ForceDisplayTo, bool) |
void | itemRemoved () |
void | makeResourceOnline (MessageViewer::Viewer::ResourceOnlineMode mode) |
void | moveMessageToTrash () |
void | popupMenu (const Akonadi::Item &msg, const KUrl &url, const KUrl &imageUrl, const QPoint &mousePos) |
void | replaceMsgByUnencryptedVersion () |
void | requestConfigSync () |
void | showMessage (KMime::Message::Ptr message, const QString &encoding) |
void | showReader (KMime::Content *aMsgPart, bool aHTML, const QString &encoding) |
void | showStatusBarMessage (const QString &message) |
void | urlClicked (const Akonadi::Item &msg, const KUrl &url) |
Public Member Functions | |
ViewerPrivate (Viewer *aParent, QWidget *mainWindow, KActionCollection *actionCollection) | |
virtual | ~ViewerPrivate () |
KToggleAction * | actionForAttachmentStrategy (const AttachmentStrategy *) |
KToggleAction * | actionForHeaderStyle (const HeaderStyle *, const HeaderStrategy *) |
void | adjustLayout () |
void | atmViewMsg (KMime::Message::Ptr message) |
void | attachmentCopy (const KMime::Content::List &contents) |
void | attachmentEncryptWithChiasmus (KMime::Content *content) |
void | attachmentOpen (KMime::Content *node) |
void | attachmentOpenWith (KMime::Content *node, KService::Ptr offer=KService::Ptr()) |
void | attachmentProperties (KMime::Content *node) |
const AttachmentStrategy * | attachmentStrategy () const |
void | attachmentView (KMime::Content *atmNode) |
void | createActions () |
QString | createAtmFileLink (const QString &atmFileName) const |
void | createWidgets () |
CSSHelper * | cssHelper () const |
bool | decryptMessage () const |
bool | deleteAttachment (KMime::Content *node, bool showWarning=true) |
void | displayMessage () |
void | displaySplashPage (const QString &info) |
bool | editAttachment (KMime::Content *node, bool showWarning=true) |
void | enableMessageDisplay () |
bool | eventFilter (QObject *obj, QEvent *ev) |
KMime::Content * | findContentByType (KMime::Content *content, const QByteArray &type) |
KService::Ptr | getServiceOffer (KMime::Content *content) |
void | goOnline () |
void | goResourceOnline () |
HeaderStrategy * | headerStrategy () const |
HeaderStyle * | headerStyle () const |
bool | htmlLoadExternal () const |
bool | htmlLoadExtOverride () const |
bool | htmlMail () const |
bool | htmlOverride () const |
MailWebView * | htmlPart () const |
HtmlWriter * | htmlWriter () const |
bool | isAShortUrl (const KUrl &url) const |
KMime::Message::Ptr | message () const |
Akonadi::Item | messageItem () const |
KMime::Content * | nodeFromUrl (const KUrl &url) |
NodeHelper * | nodeHelper () const |
void | openAttachment (KMime::Content *node, const QString &fileName) |
const QTextCodec * | overrideCodec () const |
QString | overrideEncoding () const |
void | parseContent (KMime::Content *content) |
int | pointsToPixel (int pointSize) const |
void | postProcessMessage (ObjectTreeParser *otp, KMMsgEncryptionState encryptionState) |
void | prepareHandleAttachment (KMime::Content *node, const QString &fileName) |
void | printMessage (const Akonadi::Item &msg) |
void | printPreviewMessage (const Akonadi::Item &message) |
void | readConfig () |
void | readGlobalOverrideCodec () |
QString | renderAttachments (KMime::Content *node, const QColor &bgColor) const |
void | resetStateForNewMessage () |
void | saveMainFrameScreenshotInFile (const QString &filename) |
void | saveRelativePosition () |
void | scrollToAttachment (KMime::Content *node) |
KMime::Content::List | selectedContents () |
void | setAttachmentStrategy (const AttachmentStrategy *strategy) |
void | setDecryptMessageOverwrite (bool overwrite=true) |
void | setExternalWindow (bool b) |
void | setHeaderStyleAndStrategy (HeaderStyle *style, HeaderStrategy *strategy, bool writeInConfigFile=false) |
void | setHtmlLoadExtOverride (bool override) |
void | setHtmlOverride (bool override) |
void | setMessage (const KMime::Message::Ptr &msg, Viewer::UpdateMode updateMode=Viewer::Delayed) |
void | setMessageInternal (const KMime::Message::Ptr message, Viewer::UpdateMode updateMode) |
void | setMessageItem (const Akonadi::Item &item, Viewer::UpdateMode updateMode=Viewer::Delayed) |
void | setMessagePart (KMime::Content *node) |
void | setOverrideEncoding (const QString &encoding) |
virtual void | setPrinting (bool enable) |
void | setShowAttachmentQuicklist (bool showAttachmentQuicklist=true) |
void | setShowFullCcAddressList (bool showFullCcAddressList=true) |
void | setShowFullToAddressList (bool showFullToAddressList=true) |
void | setShowRawToltecMail (bool showRawToltecMail) |
void | setShowSignatureDetails (bool showDetails=true) |
void | setUseFixedFont (bool useFixedFont) |
void | setZoomFactor (qreal zoomFactor) |
void | setZoomTextOnly (bool textOnly) |
void | showAttachmentPopup (KMime::Content *node, const QString &name, const QPoint &p) |
bool | showAttachmentQuicklist () const |
void | showContextMenu (KMime::Content *content, const QPoint &point) |
bool | showFullCcAddressList () const |
bool | showFullToAddressList () const |
void | showHideMimeTree () |
bool | showRawToltecMail () const |
bool | showSignatureDetails () const |
void | showVCard (KMime::Content *msgPart) |
void | toggleFullAddressList (const QString &field) |
Viewer * | viewer () const |
void | writeConfig (bool withSync=true) |
QString | writeMsgHeader (KMime::Message *aMsg, KMime::Content *vCardNode=0, bool topLevel=false) |
Static Public Member Functions | |
static const QTextCodec * | codecForName (const QByteArray &_str) |
Static Public Attributes | |
static const int | delay |
static const qreal | zoomBy |
Detailed Description
Private class for the Viewer, the main widget in the messageviewer library.
This class creates all subwidgets, like the MailWebView, the HtmlStatusBar and the FindBarMailWebView.
Also, ViewerPrivate creates and exposes all actions.
- Displaying a message
Before displaying a message, a message needs to be set. This can be done in two ways, with setMessageItem() and with setMessage(). setMessageItem() is the preferred way, as the viewer can then remember the Akonadi::Item belonging to the message. The Akonadi::Item is needed when modifying the message, for example when editing or deleting an attachment. Sometimes passing an Akonadi::Item to the viewer is not possible, for example when double-clicking an attached message, in which case a new KMime::Message is constructed out of the attachment, and a separate window is opened for it. In this case, the KMime::Message has no associated Akonadi::Item. If there is an Akonadi::Item available, it will be monitored for changes and the viewer automatically updated on external changes.
Once a message is set, update() is called. update() can also be called after the message has already been displayed. As an example, this is the case when the user decides to decrypt the message. The decryption can happen async, and once the decryption is finished, update() is called to display the now decrypted content. See the documentation of ObjectTreeParser on how exactly decryption is handled. update() is just a thin wrapper that calls updateReaderWin(). The only difference is that update() has a timer that prevents too many slow calls to updateReaderWin() in a short time frame. updateReaderWin() again is only a thin wrapper that resets some state and then calls displayMessage(). displayMessage() itself is again a thin wrapper, which starts the HtmlWriter and then calls parseMsg(). Finally, parseMsg() does the real work. It uses ObjectTreeParser::parseObjectTree() to let the ObjectTreeParser parse the message and generate the HTML code for it. As mentioned before, it can happen that the ObjectTreeParser needs to do some operation that happens async, for example decrypting. In this case, the ObjectTreeParser will create a BodyPartMemento, which basically is a wrapper around the job that does the async operation. Once the async operation is finished. the BodyPartMemento will trigger an update() of ViewerPrivate, so that ObjectTreeParser::parseObjectTree() gets called again and the ObjectTreeParser then can generate HTML which has the decrypted content of the message. Again, see the documentation of ObjectTreeParser for the details. Additionally, parseMsg() does some evil hack for saving unencrypted messages should the config option for that be set.
- Displaying a MIME part of the message
The viewer can show only a part of the message, for example by clicking on a MIME part in the message structure viewer or by double-clicking an attached message. In this case, setMessagePart() is called. There are two of these functions. One even has special handling for images, special handling for binary attachments and special handling of attached messages. In the last case, a new KMime::Message is constructed and set as the main message with setMessage().
- Attachment Handling
Some of those actions are actions that operate on a single attachment. For those, there is usually a slot, like slotAttachmentCopy(). These actions are triggered from the attachment context menu, which is shown in showAttachmentPopup(). The actions are connected to slotHandleAttachment() when they are activated. The action to edit an attachment uses the EditorWatcher to detect when editing with an external editor is finished. Upon finishing, slotAttachmentEditDone() is called, which then creates an ItemModifyJob to store the changes of the attachment. A map of currently active EditorWatcher and their KMime::Content is available in mEditorWatchers. For most attachment actions, the attachment is first written to a temp file. The action is then executed on this temp file. Writing the attachment to a temp file is done with NodeHelper::writeNodeToTempFile(). This method is called before opening or copying an attachment or when rendering the attachment list. The ObjectTreeParser also calls NodeHelper::writeNodeToTempFile() in some places. Once the temp file is written, NodeHelper::tempFileUrlFromNode() can be used to get the file name of the temp file for a specific MIME part. This is for example used by the handler for 'attachment:' URLs, AttachmentURLHandler.
Since URLs for attachments are in the "attachment:" scheme, dragging them as-is to outside applications wouldn't work, since other applications don't understand this scheme. Therefore, the viewer has special handling for dragging URLs: In eventFilter(), drags are detected, and the URL handler is called to deal with the drag. The attachment URL handler then starts a drag with the file:// URL of the temp file of the attachment, which it gets with NodeHelper::tempFileUrlFromNode().
TODO: How are attachment handled that are loaded on demand? How does prepareHandleAttachment() work? TODO: This temp file handling is a big mess and could use a rewrite, especially in the face of load on demand. There shouldn't be the need to write out tempfiles until really needed.
Some header styles display an attachment list in the header. The HTML code for the attachment list cannot be generated by the HeaderStyle itself, since that does not know about all attachments. Therefore, the attachment list needs to be created by ViewerPrivate. For this, the HeaderStyle writes out a placeholder for the attachment list when it creates the HTML for the header. Once the ObjectTreeParser is finished with the message, injectAttachments() is called. injectAttachments() searches for the placeholder and replaces that with the real HTML code for the attachments.
One of the attachment actions is to scoll to the attachment. That action is only available when right-clicking the header. The action scrolls to the attachment in the body and draws a yellow frame around the attachment. This is done in scrollToAttachment(). The attachment in the body and the div which is used for the colored frame are both created by the ObjectTreeParser.
- Misc
ViewerPrivate holds the NodeHelper, which is passed on to the ObjectTreeParser when it needs it. It also holds the HeaderStyle, HeaderStrategy, AttachmentStrategy, CSSHelper, HtmlWriter and more, some of them again passed to the ObjectTreeParser when it needs it.
Definition at line 182 of file viewer_p.h.
Constructor & Destructor Documentation
MessageViewer::ViewerPrivate::ViewerPrivate | ( | Viewer * | aParent, |
QWidget * | mainWindow, | ||
KActionCollection * | actionCollection | ||
) |
|
virtual |
Member Function Documentation
KToggleAction* MessageViewer::ViewerPrivate::actionForAttachmentStrategy | ( | const AttachmentStrategy * | ) |
KToggleAction* MessageViewer::ViewerPrivate::actionForHeaderStyle | ( | const HeaderStyle * | , |
const HeaderStrategy * | |||
) |
void MessageViewer::ViewerPrivate::adjustLayout | ( | ) |
void MessageViewer::ViewerPrivate::atmViewMsg | ( | KMime::Message::Ptr | message | ) |
View message part of type message/RFC822 in extra viewer window.
void MessageViewer::ViewerPrivate::attachmentCopy | ( | const KMime::Content::List & | contents | ) |
void MessageViewer::ViewerPrivate::attachmentEncryptWithChiasmus | ( | KMime::Content * | content | ) |
void MessageViewer::ViewerPrivate::attachmentOpen | ( | KMime::Content * | node | ) |
void MessageViewer::ViewerPrivate::attachmentOpenWith | ( | KMime::Content * | node, |
KService::Ptr | offer = KService::Ptr() |
||
) |
void MessageViewer::ViewerPrivate::attachmentProperties | ( | KMime::Content * | node | ) |
|
inline |
Get/set the message attachment strategy.
Definition at line 313 of file viewer_p.h.
void MessageViewer::ViewerPrivate::attachmentView | ( | KMime::Content * | atmNode | ) |
|
signal |
|
slot |
|
static |
Return a QTextCodec for the specified charset.
This function is a bit more tolerant, than QTextCodec::codecForName
void MessageViewer::ViewerPrivate::createActions | ( | ) |
QString MessageViewer::ViewerPrivate::createAtmFileLink | ( | const QString & | atmFileName | ) | const |
void MessageViewer::ViewerPrivate::createWidgets | ( | ) |
CSSHelper* MessageViewer::ViewerPrivate::cssHelper | ( | ) | const |
bool MessageViewer::ViewerPrivate::decryptMessage | ( | ) | const |
Returns whether the message should be decryted.
bool MessageViewer::ViewerPrivate::deleteAttachment | ( | KMime::Content * | node, |
bool | showWarning = true |
||
) |
Delete the attachment the.
- Parameters
-
node points to. Returns false if the user cancelled the deletion, true in all other cases (including failure to delete the attachment!)
void MessageViewer::ViewerPrivate::displayMessage | ( | ) |
Feeds the HTML viewer with the contents of the given message.
HTML begin/end parts are written around the message.
void MessageViewer::ViewerPrivate::displaySplashPage | ( | const QString & | info | ) |
Display a generic HTML splash page instead of a message.
- Parameters
-
info - the text to be displayed in HTML format
bool MessageViewer::ViewerPrivate::editAttachment | ( | KMime::Content * | node, |
bool | showWarning = true |
||
) |
Edit the attachment the.
- Parameters
-
node points to. Returns false if the user cancelled the editing, true in all other cases!
void MessageViewer::ViewerPrivate::enableMessageDisplay | ( | ) |
Enable the displaying of messages again after an splash (or other) page was displayed.
bool MessageViewer::ViewerPrivate::eventFilter | ( | QObject * | obj, |
QEvent * | ev | ||
) |
Event filter.
KMime::Content* MessageViewer::ViewerPrivate::findContentByType | ( | KMime::Content * | content, |
const QByteArray & | type | ||
) |
KService::Ptr MessageViewer::ViewerPrivate::getServiceOffer | ( | KMime::Content * | content | ) |
void MessageViewer::ViewerPrivate::goOnline | ( | ) |
void MessageViewer::ViewerPrivate::goResourceOnline | ( | ) |
|
inline |
Get the message header strategy.
Definition at line 308 of file viewer_p.h.
|
inline |
Get the message header style.
Definition at line 298 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::htmlLoadExternal | ( | ) | const |
bool MessageViewer::ViewerPrivate::htmlLoadExtOverride | ( | ) | const |
Get the load external references override setting.
bool MessageViewer::ViewerPrivate::htmlMail | ( | ) | const |
bool MessageViewer::ViewerPrivate::htmlOverride | ( | ) | const |
Get the html override setting.
|
inline |
Access to the MailWebView used for the viewer.
Use with care!
Definition at line 215 of file viewer_p.h.
|
inline |
Return the HtmlWriter connected to the MailWebView we use.
Definition at line 236 of file viewer_p.h.
|
slot |
bool MessageViewer::ViewerPrivate::isAShortUrl | ( | const KUrl & | url | ) | const |
|
signal |
|
signal |
|
inline |
Definition at line 246 of file viewer_p.h.
|
inline |
Definition at line 244 of file viewer_p.h.
|
signal |
KMime::Content* MessageViewer::ViewerPrivate::nodeFromUrl | ( | const KUrl & | url | ) |
Returns message part from given URL or null if invalid.
The URL's path is a KMime::ContentIndex path, or an index for the extra nodes, followed by : and the ContentIndex path.
|
inline |
Definition at line 240 of file viewer_p.h.
void MessageViewer::ViewerPrivate::openAttachment | ( | KMime::Content * | node, |
const QString & | fileName | ||
) |
Open the attachment pointed to the node.
- Parameters
-
fileName - if not empty, use this file to load the attachment content
const QTextCodec* MessageViewer::ViewerPrivate::overrideCodec | ( | ) | const |
Get codec corresponding to the currently selected override character encoding.
- Returns
- The override codec or 0 if auto-detection is selected.
|
inline |
Get selected override character encoding.
- Returns
- The encoding selected by the user or an empty string if auto-detection is selected.
Definition at line 321 of file viewer_p.h.
void MessageViewer::ViewerPrivate::parseContent | ( | KMime::Content * | content | ) |
Parse the given content and generate HTML out of it for display.
int MessageViewer::ViewerPrivate::pointsToPixel | ( | int | pointSize | ) | const |
Calculate the pixel size.
|
signal |
void MessageViewer::ViewerPrivate::postProcessMessage | ( | ObjectTreeParser * | otp, |
KMMsgEncryptionState | encryptionState | ||
) |
void MessageViewer::ViewerPrivate::prepareHandleAttachment | ( | KMime::Content * | node, |
const QString & | fileName | ||
) |
Sets the current attachment ID and the current attachment temporary filename to the given values.
Call this so that slotHandleAttachment() knows which attachment to handle.
void MessageViewer::ViewerPrivate::printMessage | ( | const Akonadi::Item & | msg | ) |
Print message.
void MessageViewer::ViewerPrivate::printPreviewMessage | ( | const Akonadi::Item & | message | ) |
void MessageViewer::ViewerPrivate::readConfig | ( | ) |
Read settings from app's config file.
void MessageViewer::ViewerPrivate::readGlobalOverrideCodec | ( | ) |
Read override codec from configuration.
QString MessageViewer::ViewerPrivate::renderAttachments | ( | KMime::Content * | node, |
const QColor & | bgColor | ||
) | const |
|
signal |
|
signal |
void MessageViewer::ViewerPrivate::resetStateForNewMessage | ( | ) |
void MessageViewer::ViewerPrivate::saveMainFrameScreenshotInFile | ( | const QString & | filename | ) |
void MessageViewer::ViewerPrivate::saveRelativePosition | ( | ) |
Saves the relative position of the scroll view.
Call this before calling update() if you want to preserve the current view.
void MessageViewer::ViewerPrivate::scrollToAttachment | ( | KMime::Content * | node | ) |
|
slot |
Select message body.
KMime::Content::List MessageViewer::ViewerPrivate::selectedContents | ( | ) |
void MessageViewer::ViewerPrivate::setAttachmentStrategy | ( | const AttachmentStrategy * | strategy | ) |
void MessageViewer::ViewerPrivate::setDecryptMessageOverwrite | ( | bool | overwrite = true | ) |
Enforce message decryption.
void MessageViewer::ViewerPrivate::setExternalWindow | ( | bool | b | ) |
void MessageViewer::ViewerPrivate::setHeaderStyleAndStrategy | ( | HeaderStyle * | style, |
HeaderStrategy * | strategy, | ||
bool | writeInConfigFile = false |
||
) |
Set the header style and strategy.
We only want them to be set together.
void MessageViewer::ViewerPrivate::setHtmlLoadExtOverride | ( | bool | override | ) |
Override default load external references setting.
void MessageViewer::ViewerPrivate::setHtmlOverride | ( | bool | override | ) |
Override default html mail setting.
void MessageViewer::ViewerPrivate::setMessage | ( | const KMime::Message::Ptr & | msg, |
Viewer::UpdateMode | updateMode = Viewer::Delayed |
||
) |
Set the message that shall be shown.
- Parameters
-
msg - the message to be shown. If 0, an empty page is displayed. updateMode - update the display immediately or not. See MailViewer::UpdateMode.
void MessageViewer::ViewerPrivate::setMessageInternal | ( | const KMime::Message::Ptr | message, |
Viewer::UpdateMode | updateMode | ||
) |
void MessageViewer::ViewerPrivate::setMessageItem | ( | const Akonadi::Item & | item, |
Viewer::UpdateMode | updateMode = Viewer::Delayed |
||
) |
void MessageViewer::ViewerPrivate::setMessagePart | ( | KMime::Content * | node | ) |
Instead of settings a message to be shown sets a message part to be shown.
void MessageViewer::ViewerPrivate::setOverrideEncoding | ( | const QString & | encoding | ) |
Set the override character encoding.
|
inlinevirtual |
Set printing mode.
Definition at line 327 of file viewer_p.h.
void MessageViewer::ViewerPrivate::setShowAttachmentQuicklist | ( | bool | showAttachmentQuicklist = true | ) |
void MessageViewer::ViewerPrivate::setShowFullCcAddressList | ( | bool | showFullCcAddressList = true | ) |
Show or hide the full list of "To" addresses.
void MessageViewer::ViewerPrivate::setShowFullToAddressList | ( | bool | showFullToAddressList = true | ) |
Show or hide the full list of "To" addresses.
|
inline |
Definition at line 427 of file viewer_p.h.
void MessageViewer::ViewerPrivate::setShowSignatureDetails | ( | bool | showDetails = true | ) |
Show signature details.
void MessageViewer::ViewerPrivate::setUseFixedFont | ( | bool | useFixedFont | ) |
void MessageViewer::ViewerPrivate::setZoomFactor | ( | qreal | zoomFactor | ) |
void MessageViewer::ViewerPrivate::setZoomTextOnly | ( | bool | textOnly | ) |
void MessageViewer::ViewerPrivate::showAttachmentPopup | ( | KMime::Content * | node, |
const QString & | name, | ||
const QPoint & | p | ||
) |
bool MessageViewer::ViewerPrivate::showAttachmentQuicklist | ( | ) | const |
void MessageViewer::ViewerPrivate::showContextMenu | ( | KMime::Content * | content, |
const QPoint & | point | ||
) |
bool MessageViewer::ViewerPrivate::showFullCcAddressList | ( | ) | const |
Return weather to show or hide the full list of "To" addresses.
bool MessageViewer::ViewerPrivate::showFullToAddressList | ( | ) | const |
Return weather to show or hide the full list of "To" addresses.
void MessageViewer::ViewerPrivate::showHideMimeTree | ( | ) |
Show or hide the Mime Tree Viewer if configuration is set to smart mode.
|
signal |
|
inline |
Definition at line 426 of file viewer_p.h.
|
signal |
bool MessageViewer::ViewerPrivate::showSignatureDetails | ( | ) | const |
Show signature details.
|
signal |
void MessageViewer::ViewerPrivate::showVCard | ( | KMime::Content * | msgPart | ) |
show window containing information about a vCard.
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
Copy the selected text to the clipboard.
|
slot |
|
slot |
Some attachment operations.
|
slot |
|
slot |
|
slot |
|
slot |
The user selected "Find" from the menu.
|
slot |
|
slot |
Does an action for the current attachment.
The action is defined by the KMHandleAttachmentCommand::AttachmentAction enum. prepareHandleAttachment() needs to be called before calling this to set the correct attachment ID.
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
Print message.
Called on as a response of finished() signal of mPartHtmlWriter after rendering is finished. In the very end it deletes the KMReaderWin window that was created for the purpose of rendering.
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
|
slot |
Show the message source.
|
slot |
|
slot |
|
slot |
|
slot |
The user toggled the "Fixed Font" flag from the view menu.
|
slot |
Toggle display mode between HTML and plain text.
|
slot |
|
slot |
|
slot |
Copy URL in mUrlCurrent to clipboard.
Removes "mailto:" at beginning of URL before copying.
|
slot |
The mouse has moved on or off an URL.
|
slot |
An URL has been activate with a click.
|
slot |
The user presses the right mouse button on an URL.
|
slot |
|
slot |
|
slot |
|
slot |
void MessageViewer::ViewerPrivate::toggleFullAddressList | ( | const QString & | field | ) |
Show/Hide the field with id "field".
|
slot |
Re-parse the current message.
|
slot |
Refresh the reader window.
|
signal |
|
inline |
Definition at line 242 of file viewer_p.h.
|
slot |
void MessageViewer::ViewerPrivate::writeConfig | ( | bool | withSync = true | ) |
Write settings to app's config file.
Calls sync() if withSync is true.
QString MessageViewer::ViewerPrivate::writeMsgHeader | ( | KMime::Message * | aMsg, |
KMime::Content * | vCardNode = 0 , |
||
bool | topLevel = false |
||
) |
Creates a nice mail header depending on the current selected header style.
Member Data Documentation
|
static |
Definition at line 646 of file viewer_p.h.
KActionCollection* MessageViewer::ViewerPrivate::mActionCollection |
Definition at line 661 of file viewer_p.h.
QString MessageViewer::ViewerPrivate::mAppName |
Definition at line 711 of file viewer_p.h.
const AttachmentStrategy* MessageViewer::ViewerPrivate::mAttachmentStrategy |
Definition at line 643 of file viewer_p.h.
KAction* MessageViewer::ViewerPrivate::mBlockableItems |
Definition at line 680 of file viewer_p.h.
KAction* MessageViewer::ViewerPrivate::mBlockImage |
Definition at line 679 of file viewer_p.h.
KHBox* MessageViewer::ViewerPrivate::mBox |
Definition at line 633 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mCanStartDrag |
Definition at line 686 of file viewer_p.h.
KToggleAction* MessageViewer::ViewerPrivate::mCaretBrowsing |
Definition at line 671 of file viewer_p.h.
KUrl MessageViewer::ViewerPrivate::mClickedUrl |
Definition at line 683 of file viewer_p.h.
HtmlStatusBar* MessageViewer::ViewerPrivate::mColorBar |
Definition at line 634 of file viewer_p.h.
KAction* MessageViewer::ViewerPrivate::mCopyAction |
Definition at line 662 of file viewer_p.h.
KAction* MessageViewer::ViewerPrivate::mCopyImageLocation |
Definition at line 677 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mCopyURLAction |
Definition at line 662 of file viewer_p.h.
CSSHelper* MessageViewer::ViewerPrivate::mCSSHelper |
Definition at line 656 of file viewer_p.h.
KMime::Content* MessageViewer::ViewerPrivate::mCurrentContent |
Definition at line 701 of file viewer_p.h.
QString MessageViewer::ViewerPrivate::mCurrentFileName |
Definition at line 703 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mDecrytMessageOverwrite |
Definition at line 694 of file viewer_p.h.
QMap<EditorWatcher*, KMime::Content*> MessageViewer::ViewerPrivate::mEditorWatchers |
Definition at line 705 of file viewer_p.h.
KAction* MessageViewer::ViewerPrivate::mExpandUrlAction |
Definition at line 681 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mExternalWindow |
Definition at line 698 of file viewer_p.h.
FindBarMailWebView* MessageViewer::ViewerPrivate::mFindBar |
Definition at line 640 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mFindInMessageAction |
Definition at line 662 of file viewer_p.h.
KToggleAction* MessageViewer::ViewerPrivate::mHeaderOnlyAttachmentsAction |
Definition at line 666 of file viewer_p.h.
HeaderStrategy* MessageViewer::ViewerPrivate::mHeaderStrategy |
Definition at line 644 of file viewer_p.h.
HeaderStyle* MessageViewer::ViewerPrivate::mHeaderStyle |
Definition at line 645 of file viewer_p.h.
KUrl MessageViewer::ViewerPrivate::mHoveredUrl |
Definition at line 682 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mHtmlLoadExternal |
Definition at line 628 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mHtmlLoadExtOverride |
Definition at line 628 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mHtmlMail |
Definition at line 628 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mHtmlOverride |
Definition at line 628 of file viewer_p.h.
HtmlWriter* MessageViewer::ViewerPrivate::mHtmlWriter |
Definition at line 687 of file viewer_p.h.
QString MessageViewer::ViewerPrivate::mIdOfLastViewedMessage |
Definition at line 659 of file viewer_p.h.
KUrl MessageViewer::ViewerPrivate::mImageUrl |
Definition at line 684 of file viewer_p.h.
Kleo::SpecialJob* MessageViewer::ViewerPrivate::mJob |
Definition at line 706 of file viewer_p.h.
QPoint MessageViewer::ViewerPrivate::mLastClickPosition |
Definition at line 685 of file viewer_p.h.
int MessageViewer::ViewerPrivate::mLevelQuote |
Definition at line 693 of file viewer_p.h.
QWidget* MessageViewer::ViewerPrivate::mMainWindow |
Definition at line 660 of file viewer_p.h.
KMime::Message::Ptr MessageViewer::ViewerPrivate::mMessage |
Definition at line 629 of file viewer_p.h.
Akonadi::Item MessageViewer::ViewerPrivate::mMessageItem |
Definition at line 630 of file viewer_p.h.
QSet<AbstractMessageLoadedHandler*> MessageViewer::ViewerPrivate::mMessageLoadedHandlers |
Definition at line 712 of file viewer_p.h.
KMime::Content* MessageViewer::ViewerPrivate::mMessagePartNode |
Definition at line 702 of file viewer_p.h.
QString MessageViewer::ViewerPrivate::mMessagePath |
Definition at line 704 of file viewer_p.h.
MimeTreeModel* MessageViewer::ViewerPrivate::mMimePartModel |
Definition at line 638 of file viewer_p.h.
QTreeView* MessageViewer::ViewerPrivate::mMimePartTree |
Definition at line 636 of file viewer_p.h.
Akonadi::Monitor MessageViewer::ViewerPrivate::mMonitor |
Definition at line 710 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mMsgDisplay |
This is true if the viewer currently is displaying a message.
Can be false, for example when the splash/busy page is displayed.
Definition at line 654 of file viewer_p.h.
NodeHelper* MessageViewer::ViewerPrivate::mNodeHelper |
Definition at line 627 of file viewer_p.h.
QString MessageViewer::ViewerPrivate::mOldGlobalOverrideEncoding |
Definition at line 650 of file viewer_p.h.
QString MessageViewer::ViewerPrivate::mOverrideEncoding |
Definition at line 649 of file viewer_p.h.
QPointer<WebKitPartHtmlWriter> MessageViewer::ViewerPrivate::mPartHtmlWriter |
Used only to be able to connect and disconnect finished() signal in printMsg() and slotPrintMsg() since mHtmlWriter points only to abstract non-QObject class.
Definition at line 690 of file viewer_p.h.
Akonadi::Item::Id MessageViewer::ViewerPrivate::mPreviouslyViewedItem |
Definition at line 713 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mPrinting |
Definition at line 658 of file viewer_p.h.
int MessageViewer::ViewerPrivate::mRecursionCountForDisplayMessage |
Definition at line 700 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mResetMessageDisplayFormat |
Definition at line 662 of file viewer_p.h.
QTimer MessageViewer::ViewerPrivate::mResizeTimer |
Definition at line 648 of file viewer_p.h.
float MessageViewer::ViewerPrivate::mSavedRelativePosition |
Definition at line 692 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mSaveMessageAction |
Definition at line 662 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mSaveMessageDisplayFormat |
Definition at line 662 of file viewer_p.h.
ScamDetectionWarningWidget* MessageViewer::ViewerPrivate::mScamDetectionWarning |
Definition at line 716 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mScrollDownAction |
Definition at line 662 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mScrollDownMoreAction |
Definition at line 662 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mScrollUpAction |
Definition at line 662 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mScrollUpMoreAction |
Definition at line 662 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mSelectAllAction |
Definition at line 662 of file viewer_p.h.
KSelectAction* MessageViewer::ViewerPrivate::mSelectEncodingAction |
Definition at line 667 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mShowAttachmentQuicklist |
Definition at line 696 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mShowFullCcAddressList |
Definition at line 709 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mShowFullToAddressList |
Definition at line 708 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mShowRawToltecMail |
Definition at line 697 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mShowSignatureDetails |
Definition at line 695 of file viewer_p.h.
KAction* MessageViewer::ViewerPrivate::mSpeakTextAction |
Definition at line 676 of file viewer_p.h.
QSplitter* MessageViewer::ViewerPrivate::mSplitter |
Definition at line 632 of file viewer_p.h.
GrantleeTheme::GrantleeThemeManager* MessageViewer::ViewerPrivate::mThemeManager |
Definition at line 715 of file viewer_p.h.
KToggleAction * MessageViewer::ViewerPrivate::mToggleDisplayModeAction |
Definition at line 668 of file viewer_p.h.
KToggleAction* MessageViewer::ViewerPrivate::mToggleFixFontAction |
Definition at line 668 of file viewer_p.h.
KToggleAction* MessageViewer::ViewerPrivate::mToggleMimePartTreeAction |
Definition at line 675 of file viewer_p.h.
KAction* MessageViewer::ViewerPrivate::mTranslateAction |
Definition at line 678 of file viewer_p.h.
PimCommon::TranslatorWidget* MessageViewer::ViewerPrivate::mTranslatorWidget |
Definition at line 641 of file viewer_p.h.
QTimer MessageViewer::ViewerPrivate::mUpdateReaderWinTimer |
Definition at line 647 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mUrlOpenAction |
Definition at line 662 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mUseFixedFont |
Definition at line 657 of file viewer_p.h.
MailWebView* MessageViewer::ViewerPrivate::mViewer |
Definition at line 639 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mViewSourceAction |
Definition at line 662 of file viewer_p.h.
qreal MessageViewer::ViewerPrivate::mZoomFactor |
Definition at line 719 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mZoomInAction |
Definition at line 674 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mZoomOutAction |
Definition at line 674 of file viewer_p.h.
KAction * MessageViewer::ViewerPrivate::mZoomResetAction |
Definition at line 674 of file viewer_p.h.
bool MessageViewer::ViewerPrivate::mZoomTextOnly |
Definition at line 699 of file viewer_p.h.
KAction* MessageViewer::ViewerPrivate::mZoomTextOnlyAction |
Definition at line 674 of file viewer_p.h.
Viewer* const MessageViewer::ViewerPrivate::q |
Definition at line 707 of file viewer_p.h.
|
static |
Definition at line 718 of file viewer_p.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:55:58 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.