KCal Library
#include <resourcecached.h>
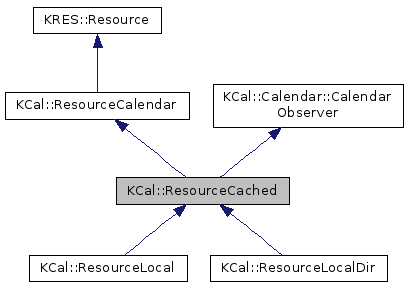
Public Types | |
enum | { ReloadNever, ReloadOnStartup, ReloadInterval } |
enum | { SaveNever, SaveOnExit, SaveInterval, SaveDelayed, SaveAlways } |
enum | CacheAction { DefaultCache, NoSyncCache, SyncCache } |
Public Member Functions | |
ResourceCached (const KConfigGroup &group) | |
Incidence::List | addedIncidences () const |
bool | addEvent (Event *event) |
virtual KCAL_DEPRECATED bool | addJournal (Journal *journal) |
bool | addTodo (Todo *todo) |
Alarm::List | alarms (const KDateTime &from, const KDateTime &to) |
Alarm::List | alarmsTo (const KDateTime &to) |
Incidence::List | allChanges () const |
Incidence::List | changedIncidences () const |
void | cleanUpEventCache (const KCal::Event::List &eventList) |
void | cleanUpTodoCache (const KCal::Todo::List &todoList) |
void | clearCache () |
void | clearChange (Incidence *incidence) |
void | clearChange (const QString &uid) |
void | clearChanges () |
bool | defaultReloadInhibited () const |
void | deleteAllEvents () |
virtual void | deleteAllJournals () |
void | deleteAllTodos () |
Incidence::List | deletedIncidences () const |
bool | deleteEvent (Event *event) |
virtual bool | deleteJournal (Journal *) |
bool | deleteTodo (Todo *) |
void | disableChangeNotification () |
void | enableChangeNotification () |
Event * | event (const QString &UniqueStr) |
Event::List | events () |
bool | hasChanges () const |
KRES::IdMapper & | idMapper () |
bool | inhibitDefaultReload (bool inhibit) |
virtual Journal * | journal (const QString &uid) |
KDateTime | lastLoad () const |
KDateTime | lastSave () const |
bool | load (CacheAction action) |
virtual bool | load () |
bool | loadFromCache () |
Person | owner () const |
Event::List | rawEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | rawEvents (const QDate &start, const QDate &end, const KDateTime::Spec &timeSpec=KDateTime::Spec(), bool inclusive=false) |
Event::List | rawEventsForDate (const QDate &date, const KDateTime::Spec &timeSpec=KDateTime::Spec(), EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | rawEventsForDate (const KDateTime &dt) |
Journal::List | rawJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Journal::List | rawJournalsForDate (const QDate &date) |
Todo::List | rawTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Todo::List | rawTodosForDate (const QDate &date) |
void | readConfig (const KConfigGroup &group) |
bool | reloaded () const |
int | reloadInterval () const |
int | reloadPolicy () const |
bool | save (CacheAction action, Incidence *incidence=0) |
virtual bool | save (Incidence *incidence=0) |
int | saveInterval () const |
int | savePolicy () const |
void | saveToCache () |
void | setOwner (const Person &owner) |
void | setReloadInterval (int minutes) |
void | setReloadPolicy (int policy) |
void | setSaveInterval (int minutes) |
void | setSavePolicy (int policy) |
void | setTimeSpec (const KDateTime::Spec &timeSpec) |
void | setTimeZoneId (const QString &timeZoneId) |
virtual void | shiftTimes (const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) |
KDateTime::Spec | timeSpec () const |
QString | timeZoneId () const |
Todo * | todo (const QString &uid) |
void | writeConfig (KConfigGroup &group) |
![]() | |
ResourceCalendar (const KConfigGroup &group) | |
virtual bool | addIncidence (Incidence *) |
virtual bool | canHaveSubresources () const |
virtual bool | deleteIncidence (Incidence *) |
Incidence * | incidence (const QString &uid) |
virtual QString | infoText () const |
bool | isResolveConflictSet () const |
virtual bool | isSaving () |
virtual QString | labelForSubresource (const QString &resource) const |
virtual KABC::Lock * | lock ()=0 |
Incidence::List | rawIncidences () |
bool | save (Incidence *incidence=0) |
bool | save (QString &err, Incidence *incidence=0) |
bool | saveInhibited () const |
void | setInhibitSave (bool inhibit) |
void | setResolveConflict (bool b) |
virtual bool | setValue (const QString &key, const QString &value) |
virtual bool | subresourceActive (const QString &resource) const |
virtual QString | subresourceIdentifier (Incidence *incidence) |
virtual QStringList | subresources () const |
![]() | |
Resource (const KConfigGroup &group) | |
void | close () |
virtual void | dump () const |
QString | identifier () const |
bool | isActive () const |
bool | isOpen () const |
bool | open () |
virtual bool | readOnly () const |
virtual QString | resourceName () const |
void | setActive (bool active) |
void | setIdentifier (const QString &identifier) |
virtual void | setReadOnly (bool value) |
virtual void | setResourceName (const QString &name) |
void | setType (const QString &type) |
QString | type () const |
![]() | |
virtual | ~CalendarObserver () |
virtual void | calendarModified (bool modified, Calendar *calendar) |
Protected Slots | |
void | setIdMapperIdentifier () |
void | slotReload () |
void | slotSave () |
Protected Member Functions | |
void | addInfoText (QString &) const |
virtual QString | cacheFile () const |
CalendarLocal * | calendar () const |
void | calendarIncidenceAdded (KCal::Incidence *incidence) |
void | calendarIncidenceChanged (KCal::Incidence *incidence) |
void | calendarIncidenceDeleted (KCal::Incidence *incidence) |
virtual QString | changesCacheFile (const QString &type) const |
void | checkForAutomaticSave () |
bool | checkForReload () |
bool | checkForSave () |
virtual void | doClose () |
virtual bool | doLoad (bool syncCache)=0 |
virtual bool | doOpen () |
virtual bool | doSave (bool syncCache)=0 |
virtual bool | doSave (bool syncCache, Incidence *incidence) |
void | loadChangesCache (QMap< Incidence *, bool > &map, const QString &type) |
void | loadChangesCache () |
void | saveChangesCache (const QMap< Incidence *, bool > &map, const QString &type) |
void | saveChangesCache () |
void | setReloaded (bool done) |
void | setupReloadTimer () |
void | setupSaveTimer () |
![]() | |
void | loadError (const QString &errorMessage=QString()) |
bool | noReadOnlyOnLoad () const |
bool | receivedLoadError () const |
bool | receivedSaveError () const |
void | saveError (const QString &errorMessage=QString()) |
void | setNoReadOnlyOnLoad (bool noReadOnly) |
void | setReceivedLoadError (bool b) |
void | setReceivedSaveError (bool b) |
Additional Inherited Members | |
![]() | |
virtual bool | addSubresource (const QString &resource, const QString &parent) |
virtual bool | removeSubresource (const QString &resource) |
virtual void | setSubresourceActive (const QString &resource, bool active) |
virtual QString | subresourceType (const QString &resource) |
![]() | |
void | resourceChanged (ResourceCalendar *) |
void | resourceLoaded (ResourceCalendar *) |
void | resourceLoadError (ResourceCalendar *, const QString &error) |
void | resourceSaved (ResourceCalendar *) |
void | resourceSaveError (ResourceCalendar *, const QString &error) |
void | signalSubresourceAdded (ResourceCalendar *, const QString &type, const QString &subresource, const QString &label) |
void | signalSubresourceRemoved (ResourceCalendar *, const QString &, const QString &) |
Detailed Description
This class provides a calendar resource using a local CalendarLocal object to cache the calendar data.
Definition at line 43 of file resourcecached.h.
Member Enumeration Documentation
anonymous enum |
Reload policy.
Whether and when to automatically reload the resource.
- See also
- setReloadPolicy(), reloadPolicy()
Enumerator | |
---|---|
ReloadNever |
never reload the resource automatically |
ReloadOnStartup |
reload when the resource is opened |
ReloadInterval |
reload at regular intervals set by setReloadInterval() |
Definition at line 52 of file resourcecached.h.
anonymous enum |
Save policy.
Whether and when to automatically save the resource.
- See also
- setSavePolicy(), savePolicy()
Enumerator | |
---|---|
SaveNever |
never save the resource automatically |
SaveOnExit |
save when the resource is closed |
SaveInterval |
save at regular intervals set by setSaveInterval() |
SaveDelayed |
save after every change, after a 15 second delay |
SaveAlways |
save after every change, after a 1 second delay |
Definition at line 62 of file resourcecached.h.
Whether to update the cache file when loading a resource, or whether to upload the cache file after saving the resource.
Only applicable to genuinely cached resources.
Enumerator | |
---|---|
DefaultCache |
use the default action set by setReloadPolicy() or setSavePolicy() |
NoSyncCache |
perform a cache-only operation, without downloading or uploading |
SyncCache |
update the cache file before loading, or upload cache after saving |
Definition at line 75 of file resourcecached.h.
Member Function Documentation
|
virtual |
Add event to calendar.
- Parameters
-
event is a pointer to the Event to insert.
Implements KCal::ResourceCalendar.
Definition at line 232 of file resourcecached.cpp.
|
protectedvirtual |
Add info text for concrete resources.
Called by infoText().
Reimplemented from KCal::ResourceCalendar.
Definition at line 853 of file resourcecached.cpp.
|
virtual |
Add a Journal entry to calendar.
- Parameters
-
journal is a pointer to the Journal to insert.
Implements KCal::ResourceCalendar.
Definition at line 320 of file resourcecached.cpp.
|
virtual |
Add a todo to the todolist.
- Parameters
-
todo is a pointer to the Todo to insert.
Implements KCal::ResourceCalendar.
Definition at line 280 of file resourcecached.cpp.
|
virtual |
Return all alarms, which occur in the given time interval.
Implements KCal::ResourceCalendar.
Definition at line 345 of file resourcecached.cpp.
|
virtual |
Return all alarms, which occur before given date.
Implements KCal::ResourceCalendar.
Definition at line 340 of file resourcecached.cpp.
|
protectedvirtual |
This method is used by loadFromCache() and saveToCache(), reimplement it to change the location of the cache.
Definition at line 600 of file resourcecached.cpp.
|
protectedvirtual |
Notify the Observer that an Incidence has been inserted.
- Parameters
-
incidence is a pointer to the Incidence that was inserted.
Reimplemented from KCal::Calendar::CalendarObserver.
Definition at line 664 of file resourcecached.cpp.
|
protectedvirtual |
Notify the Observer that an Incidence has been modified.
- Parameters
-
incidence is a pointer to the Incidence that was modified.
Reimplemented from KCal::Calendar::CalendarObserver.
Definition at line 677 of file resourcecached.cpp.
|
protectedvirtual |
Notify the Observer that an Incidence has been removed.
- Parameters
-
incidence is a pointer to the Incidence that was removed.
Reimplemented from KCal::Calendar::CalendarObserver.
Definition at line 691 of file resourcecached.cpp.
|
protectedvirtual |
Functions for keeping the changes persistent.
Definition at line 605 of file resourcecached.cpp.
|
protected |
Check if reload required according to reload policy.
Definition at line 834 of file resourcecached.cpp.
|
protected |
Check if save required according to save policy.
Definition at line 845 of file resourcecached.cpp.
void ResourceCached::clearCache | ( | ) |
Clear cache.
Definition at line 524 of file resourcecached.cpp.
|
virtual |
Removes all Events from this calendar.
Implements KCal::ResourceCalendar.
Reimplemented in KCal::ResourceLocalDir.
Definition at line 245 of file resourcecached.cpp.
|
virtual |
Removes all Journals from this calendar.
Implements KCal::ResourceCalendar.
Reimplemented in KCal::ResourceLocalDir.
Definition at line 300 of file resourcecached.cpp.
|
virtual |
Removes all todos from this calendar.
Implements KCal::ResourceCalendar.
Reimplemented in KCal::ResourceLocalDir.
Definition at line 290 of file resourcecached.cpp.
|
virtual |
Deletes an event from this calendar.
Implements KCal::ResourceCalendar.
Reimplemented in KCal::ResourceLocalDir.
Definition at line 238 of file resourcecached.cpp.
|
virtual |
Remove a Journal from the calendar.
Implements KCal::ResourceCalendar.
Reimplemented in KCal::ResourceLocalDir.
Definition at line 295 of file resourcecached.cpp.
|
virtual |
Remove a todo from the todolist.
Implements KCal::ResourceCalendar.
Reimplemented in KCal::ResourceLocalDir.
Definition at line 285 of file resourcecached.cpp.
|
protectedvirtual |
Virtual method from KRES::Resource, called when the last instace of the resource is closed.
Reimplemented from KRES::Resource.
Definition at line 867 of file resourcecached.cpp.
|
protectedpure virtual |
Do the actual loading of the resource data.
Called by load(CacheAction).
Implements KCal::ResourceCalendar.
Implemented in KCal::ResourceLocal, and KCal::ResourceLocalDir.
|
protectedvirtual |
Opens the resource.
Dummy implementation, so child classes don't have to reimplement this method. By default, this does not do anything, but can be reimplemented in child classes
Reimplemented from KRES::Resource.
Reimplemented in KCal::ResourceLocalDir.
Definition at line 878 of file resourcecached.cpp.
|
protectedpure virtual |
Do the actual saving of the resource data.
Called by save(CacheAction). Saves the resource data to the cache and optionally uploads (if a remote resource).
- Parameters
-
syncCache if true, the cache will be uploaded to the remote resource. If false, only the cache will be updated.
Implements KCal::ResourceCalendar.
Implemented in KCal::ResourceLocal, and KCal::ResourceLocalDir.
|
protectedvirtual |
Do the actual saving of the resource data.
Called by save(CacheAction). Save one Incidence. The default implementation calls doSave(bool) to save everything.
- Parameters
-
syncCache if true
, the cache will be uploaded to the remote resource. Iffalse
, only the cache will be updatedincidence The incidence to be saved.
Reimplemented from KCal::ResourceCalendar.
Reimplemented in KCal::ResourceLocal, and KCal::ResourceLocalDir.
Definition at line 503 of file resourcecached.cpp.
|
virtual |
Retrieves an event on the basis of the unique string ID.
Implements KCal::ResourceCalendar.
Definition at line 250 of file resourcecached.cpp.
Event::List KCal::ResourceCached::events | ( | ) |
Return filtered list of all events in calendar.
KRES::IdMapper & ResourceCached::idMapper | ( | ) |
Returns a reference to the id mapper.
Definition at line 595 of file resourcecached.cpp.
bool ResourceCached::inhibitDefaultReload | ( | bool | inhibit | ) |
Inhibit or allow cache reloads when using load(DefaultCache).
If inhibited, this overrides the policy set by setReloadPolicy(), preventing any non-explicit reloads from being performed. If not inhibited, reloads take place according to the policy set by setReloadPolicy().
- Parameters
-
inhibit true to inhibit reloads, false to allow them
Definition at line 151 of file resourcecached.cpp.
|
virtual |
Return Journal with given unique id.
Implements KCal::ResourceCalendar.
Definition at line 325 of file resourcecached.cpp.
KDateTime KCal::ResourceCached::lastLoad | ( | ) | const |
Return time of last load.
KDateTime KCal::ResourceCached::lastSave | ( | ) | const |
Return time of last save.
bool ResourceCached::load | ( | CacheAction | action | ) |
Load resource data, specifying whether to refresh the cache file first.
For a non-cached resource, this method has the same effect as load().
- Parameters
-
action is the type of CacheAction for this data loading.
Definition at line 382 of file resourcecached.cpp.
|
virtual |
Load resource data.
Reimplemented from KCal::ResourceCalendar.
Definition at line 432 of file resourcecached.cpp.
bool ResourceCached::loadFromCache | ( | ) |
Load the resource from the cache.
- Returns
- true if the cache file exists, false if not
Definition at line 437 of file resourcecached.cpp.
Person KCal::ResourceCached::owner | ( | ) | const |
Return the owner of the calendar's full name.
Definition at line 889 of file resourcecached.cpp.
|
virtual |
Return unfiltered list of all events in calendar.
Implements KCal::ResourceCalendar.
Definition at line 275 of file resourcecached.cpp.
|
virtual |
Get unfiltered events in a range of dates.
If inclusive is set to true, only events are returned, which are completely included in the range.
- Parameters
-
start date at the begin of the searching range end date at the end of the searching range timeSpec timeSpec of the searching range inclusive if true
, only match events which are completely within the specified range
Implements KCal::ResourceCalendar.
Definition at line 264 of file resourcecached.cpp.
|
virtual |
Builds and then returns a list of all events that match for the date specified.
useful for dayView, etc. etc.
- Parameters
-
date date for which to get the events timeSpec the time specification of the date sortField field used as the sort key for the result list sortDirection direction of sorting according to sortField
Implements KCal::ResourceCalendar.
Definition at line 255 of file resourcecached.cpp.
|
virtual |
Get unfiltered events for date dt
.
Implements KCal::ResourceCalendar.
Definition at line 270 of file resourcecached.cpp.
|
virtual |
Return list of all journals.
Implements KCal::ResourceCalendar.
Definition at line 330 of file resourcecached.cpp.
|
virtual |
Return list of journals for the given date.
Implements KCal::ResourceCalendar.
Definition at line 335 of file resourcecached.cpp.
|
virtual |
Return list of all todos.
Implements KCal::ResourceCalendar.
Definition at line 305 of file resourcecached.cpp.
|
virtual |
Returns list of todos due on the specified date.
Implements KCal::ResourceCalendar.
Definition at line 315 of file resourcecached.cpp.
bool ResourceCached::reloaded | ( | ) | const |
Return whether the resource cache has been reloaded since startup.
Definition at line 119 of file resourcecached.cpp.
int ResourceCached::reloadInterval | ( | ) | const |
Return reload interval in minutes.
Definition at line 146 of file resourcecached.cpp.
int ResourceCached::reloadPolicy | ( | ) | const |
bool ResourceCached::save | ( | CacheAction | action, |
Incidence * | incidence = 0 |
||
) |
Save the resource data to cache, and optionally upload the cache file afterwards.
For a non-cached resource, this method has the same effect as save().
- Parameters
-
action is the type of CacheAction for this data saving. incidence if given as 0, doSave(bool) is called to save all incidences, else doSave(bool, incidence) is called to save only the given one.
Definition at line 456 of file resourcecached.cpp.
|
virtual |
Save resource data.
Definition at line 498 of file resourcecached.cpp.
int ResourceCached::saveInterval | ( | ) | const |
Return save interval in minutes.
Definition at line 177 of file resourcecached.cpp.
int ResourceCached::savePolicy | ( | ) | const |
void ResourceCached::saveToCache | ( | ) |
Save the resource back to the cache.
Definition at line 509 of file resourcecached.cpp.
void KCal::ResourceCached::setOwner | ( | const Person & | owner | ) |
Set the owner of the calendar.
Should be owner's full name.
- Parameters
-
owner the person who owns this calendar resource
Definition at line 884 of file resourcecached.cpp.
|
protected |
Set the cache-reloaded status.
Non-local resources must set this true once the cache has been downloaded successfully.
- Parameters
-
done the new cache-reloaded status
Definition at line 124 of file resourcecached.cpp.
void ResourceCached::setReloadInterval | ( | int | minutes | ) |
Set reload interval in minutes which is used when reload policy is ReloadInterval.
Definition at line 141 of file resourcecached.cpp.
void ResourceCached::setReloadPolicy | ( | int | policy | ) |
Set reload policy.
This controls when the cache is refreshed.
ReloadNever never reload ReloadOnStartup reload when resource is started ReloadInterval reload regularly after given interval
Definition at line 129 of file resourcecached.cpp.
void ResourceCached::setSaveInterval | ( | int | minutes | ) |
Set save interval in minutes which is used when save policy is SaveInterval.
Definition at line 172 of file resourcecached.cpp.
void ResourceCached::setSavePolicy | ( | int | policy | ) |
Set save policy.
This controls when the cache is refreshed.
SaveNever never save SaveOnExit save when resource is exited SaveInterval save regularly after given interval SaveDelayed save on every change, after small delay SaveAlways save on every change
Definition at line 160 of file resourcecached.cpp.
|
virtual |
Set the time specification (time zone, etc.).
- Parameters
-
timeSpec the time specification to set
- See also
- timeSpec()
Implements KCal::ResourceCalendar.
Definition at line 350 of file resourcecached.cpp.
|
virtual |
Set id of timezone, e.g.
"Europe/Berlin"
- Parameters
-
timeZoneId the identifier for the timezone
- See also
- timeZoneId()
Implements KCal::ResourceCalendar.
Definition at line 360 of file resourcecached.cpp.
|
virtual |
ResourceCalendar::shiftTimes()
Implements KCal::ResourceCalendar.
Definition at line 370 of file resourcecached.cpp.
|
virtual |
Get the viewing time specification (time zone etc.) for the calendar.
- Returns
- time specification
Implements KCal::ResourceCalendar.
Definition at line 355 of file resourcecached.cpp.
|
virtual |
Returns the viewing time zone ID for the resource.
- Returns
- the string containing the time zone ID, or empty string if the viewing time specification is not a time zone.
Implements KCal::ResourceCalendar.
Definition at line 365 of file resourcecached.cpp.
|
virtual |
Searches todolist for an event with this unique string identifier, returns a pointer or null.
Implements KCal::ResourceCalendar.
Definition at line 310 of file resourcecached.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:58 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.