KCalCore Library
#include <incidencebase.h>
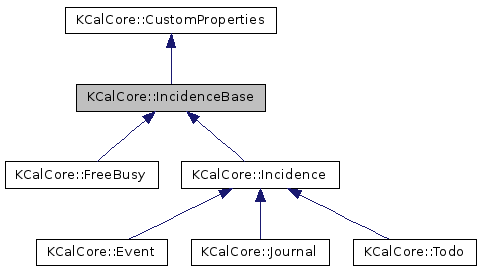
Classes | |
class | IncidenceObserver |
Public Types | |
enum | DateTimeRole { RoleAlarmStartOffset = 0, RoleAlarmEndOffset, RoleSort, RoleCalendarHashing, RoleStartTimeZone, RoleEndTimeZone, RoleEndRecurrenceBase, RoleEnd, RoleDisplayEnd, RoleAlarm, RoleRecurrenceStart, RoleDisplayStart, RoleDnD } |
enum | Field { FieldDtStart, FieldDtEnd, FieldLastModified, FieldDescription, FieldSummary, FieldLocation, FieldCompleted, FieldPercentComplete, FieldDtDue, FieldCategories, FieldRelatedTo, FieldRecurrence, FieldAttachment, FieldSecrecy, FieldStatus, FieldTransparency, FieldResources, FieldPriority, FieldGeoLatitude, FieldGeoLongitude, FieldRecurrenceId, FieldAlarms, FieldSchedulingId, FieldAttendees, FieldOrganizer, FieldCreated, FieldRevision, FieldDuration, FieldContact, FieldComment, FieldUid, FieldUnknown, FieldUrl } |
enum | IncidenceType { TypeEvent = 0, TypeTodo, TypeJournal, TypeFreeBusy, TypeUnknown } |
typedef QSharedPointer < IncidenceBase > | Ptr |
Public Member Functions | |
IncidenceBase () | |
virtual | ~IncidenceBase () |
virtual bool | accept (Visitor &v, IncidenceBase::Ptr incidence) |
void | addAttendee (const Attendee::Ptr &attendee, bool doUpdate=true) |
void | addComment (const QString &comment) |
void | addContact (const QString &contact) |
bool | allDay () const |
Attendee::Ptr | attendeeByMail (const QString &email) const |
Attendee::Ptr | attendeeByMails (const QStringList &emails, const QString &email=QString()) const |
Attendee::Ptr | attendeeByUid (const QString &uid) const |
int | attendeeCount () const |
Attendee::List | attendees () const |
void | clearAttendees () |
void | clearComments () |
void | clearContacts () |
QStringList | comments () const |
QStringList | contacts () const |
virtual KDateTime | dateTime (DateTimeRole role) const =0 |
void | deleteAttendee (const Attendee::Ptr &attendee, bool doUpdate=true) |
QSet< IncidenceBase::Field > | dirtyFields () const |
virtual KDateTime | dtStart () const |
Duration | duration () const |
void | endUpdates () |
bool | hasDuration () const |
bool | isReadOnly () const |
KDateTime | lastModified () const |
virtual QLatin1String | mimeType () const =0 |
bool | operator!= (const IncidenceBase &ib) const |
IncidenceBase & | operator= (const IncidenceBase &other) |
bool | operator== (const IncidenceBase &ib) const |
Person::Ptr | organizer () const |
virtual KDateTime | recurrenceId () const |
void | registerObserver (IncidenceObserver *observer) |
bool | removeComment (const QString &comment) |
bool | removeContact (const QString &contact) |
void | resetDirtyFields () |
void | setAllDay (bool allDay) |
virtual void | setDateTime (const KDateTime &dateTime, DateTimeRole role)=0 |
void | setDirtyFields (const QSet< IncidenceBase::Field > &) |
virtual void | setDtStart (const KDateTime &dtStart) |
virtual void | setDuration (const Duration &duration) |
void | setHasDuration (bool hasDuration) |
virtual void | setLastModified (const KDateTime &lm) |
void | setOrganizer (const Person::Ptr &organizer) |
void | setOrganizer (const QString &organizer) |
virtual void | setReadOnly (bool readOnly) |
void | setUid (const QString &uid) |
void | setUrl (const QUrl &url) |
virtual void | shiftTimes (const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) |
void | startUpdates () |
virtual IncidenceType | type () const =0 |
virtual QByteArray | typeStr () const =0 |
QString | uid () const |
void | unRegisterObserver (IncidenceObserver *observer) |
void | update () |
void | updated () |
KUrl | uri () const |
QUrl | url () const |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &other) | |
virtual | ~CustomProperties () |
QMap< QByteArray, QString > | customProperties () const |
QString | customProperty (const QByteArray &app, const QByteArray &key) const |
QString | nonKDECustomProperty (const QByteArray &name) const |
QString | nonKDECustomPropertyParameters (const QByteArray &name) const |
CustomProperties & | operator= (const CustomProperties &other) |
bool | operator== (const CustomProperties &properties) const |
void | removeCustomProperty (const QByteArray &app, const QByteArray &key) |
void | removeNonKDECustomProperty (const QByteArray &name) |
void | setCustomProperties (const QMap< QByteArray, QString > &properties) |
void | setCustomProperty (const QByteArray &app, const QByteArray &key, const QString &value) |
void | setNonKDECustomProperty (const QByteArray &name, const QString &value, const QString ¶meters=QString()) |
Static Public Member Functions | |
static quint32 | magicSerializationIdentifier () |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
Protected Types | |
enum | VirtualHook { SerializerHook, DeserializerHook } |
Protected Member Functions | |
IncidenceBase (const IncidenceBase &ib) | |
virtual IncidenceBase & | assign (const IncidenceBase &other) |
virtual void | customPropertyUpdate () |
virtual void | customPropertyUpdated () |
virtual bool | equals (const IncidenceBase &incidenceBase) const |
void | setFieldDirty (IncidenceBase::Field field) |
virtual void | virtual_hook (int id, void *data)=0 |
Protected Attributes | |
bool | mReadOnly |
Friends | |
KCALCORE_EXPORT QDataStream & | operator<< (QDataStream &stream, const KCalCore::IncidenceBase::Ptr &) |
KCALCORE_EXPORT QDataStream & | operator>> (QDataStream &stream, const KCalCore::IncidenceBase::Ptr &) |
Detailed Description
An abstract class that provides a common base for all calendar incidence classes.
define: organizer (person) define: uid (same as the attendee uid?)
Several properties are not allowed for VFREEBUSY objects (see rfc:2445), so they are not in IncidenceBase. The hierarchy is:
So IncidenceBase contains all properties that are common to all classes, and Incidence contains all additional properties that are common to Events, Todos and Journals, but are not allowed for FreeBusy entries.
Definition at line 109 of file incidencebase.h.
Member Typedef Documentation
typedef QSharedPointer<IncidenceBase> KCalCore::IncidenceBase::Ptr |
A shared pointer to an IncidenceBase.
Definition at line 115 of file incidencebase.h.
Member Enumeration Documentation
The different types of incidence date/times roles.
- See also
- dateTime()
Enumerator | |
---|---|
RoleAlarmStartOffset |
Role for an incidence alarm's starting offset date/time. |
RoleAlarmEndOffset |
Role for an incidence alarm's ending offset date/time. |
RoleSort |
Role for an incidence's date/time used when sorting. |
RoleCalendarHashing |
Role for looking up an incidence in a Calendar. |
RoleStartTimeZone |
Role for determining an incidence's starting timezone. |
RoleEndTimeZone |
Role for determining an incidence's ending timezone. |
RoleEnd |
Role for determining an incidence's dtEnd, will return an invalid KDateTime if the incidence does not support dtEnd. |
RoleDisplayEnd |
Role used for display purposes, represents the end boundary if an incidence supports dtEnd. |
RoleAlarm |
Role for determining the date/time of the first alarm. Returns invalid time if the incidence doesn't have any alarm |
RoleRecurrenceStart |
Role for determining the start of the recurrence. Currently that's DTSTART for an event and DTDUE for a to-do. (NOTE: If the incidence is a to-do, recurrence should be calculated having DTSTART for a reference, not DT-DUE. This is one place KCalCore isn't compliant with RFC2445) |
RoleDisplayStart |
Role for display purposes, represents the start boundary of an incidence. To-dos return dtDue here, for historical reasons |
RoleDnD |
Role for determining new start and end dates after a DnD. |
Definition at line 133 of file incidencebase.h.
The different types of incidence fields.
Definition at line 160 of file incidencebase.h.
The different types of incidences, per RFC2445.
Enumerator | |
---|---|
TypeEvent |
Type is an event. |
TypeTodo |
Type is a to-do. |
TypeJournal |
Type is a journal. |
TypeFreeBusy |
Type is a free/busy. |
TypeUnknown |
Type unknown. |
Definition at line 121 of file incidencebase.h.
Constructor & Destructor Documentation
IncidenceBase::IncidenceBase | ( | ) |
Constructs an empty IncidenceBase.
Private class that helps to provide binary compatibility between releases.
Definition at line 123 of file incidencebase.cpp.
|
virtual |
Destroys the IncidenceBase.
Definition at line 137 of file incidencebase.cpp.
|
protected |
Constructs an IncidenceBase as a copy of another IncidenceBase object.
- Parameters
-
ib is the IncidenceBase to copy.
Definition at line 130 of file incidencebase.cpp.
Member Function Documentation
|
virtual |
Accept IncidenceVisitor.
A class taking part in the visitor mechanism has to provide this implementation:
bool accept(Visitor &v) { return v.visit(this); }
- Parameters
-
v is a reference to a Visitor object. incidence is a valid IncidenceBase object for visting.
Definition at line 220 of file incidencebase.cpp.
void IncidenceBase::addAttendee | ( | const Attendee::Ptr & | attendee, |
bool | doUpdate = true |
||
) |
Add Attendee to this incidence.
IncidenceBase takes ownership of the Attendee object.
- Parameters
-
attendee a pointer to the attendee to add doUpdate If true the Observers are notified, if false they are not.
Definition at line 428 of file incidencebase.cpp.
void IncidenceBase::addComment | ( | const QString & | comment | ) |
Adds a comment to the incidence.
Does not add a linefeed character; simply appends the text as specified.
- Parameters
-
comment is the QString containing the comment to add.
- See also
- removeComment().
Definition at line 355 of file incidencebase.cpp.
void IncidenceBase::addContact | ( | const QString & | contact | ) |
Adds a contact to thieincidence.
Does not add a linefeed character; simply appends the text as specified.
- Parameters
-
contact is the QString containing the contact to add.
- See also
- removeContact().
Definition at line 390 of file incidencebase.cpp.
bool IncidenceBase::allDay | ( | ) | const |
Returns true or false depending on whether the incidence is all-day.
i.e. has a date but no time attached to it.
- See also
- setAllDay()
Definition at line 326 of file incidencebase.cpp.
|
protectedvirtual |
Provides polymorfic assignment.
- Parameters
-
other is the IncidenceBase to assign.
Reimplemented in KCalCore::Incidence, KCalCore::Todo, KCalCore::FreeBusy, KCalCore::Event, and KCalCore::Journal.
Definition at line 154 of file incidencebase.cpp.
Attendee::Ptr IncidenceBase::attendeeByMail | ( | const QString & | ) | const |
Returns the attendee with the specified email address.
- Parameters
-
email is a QString containing an email address of the form "FirstName LastName <emailaddress>".
- See also
- attendeeByMails(), attendeesByUid().
Definition at line 502 of file incidencebase.cpp.
Attendee::Ptr IncidenceBase::attendeeByMails | ( | const QStringList & | emails, |
const QString & | email = QString() |
||
) | const |
Returns the first incidence attendee with one of the specified email addresses.
- Parameters
-
emails is a list of QStrings containing email addresses of the form "FirstName LastName <emailaddress>". email is a QString containing a single email address to search in addition to the list specified in emails
.
- See also
- attendeeByMail(), attendeesByUid().
Definition at line 514 of file incidencebase.cpp.
Attendee::Ptr IncidenceBase::attendeeByUid | ( | const QString & | uid | ) | const |
Returns the incidence attendee with the specified attendee UID.
- Parameters
-
uid is a QString containing an attendee UID.
- See also
- attendeeByMail(), attendeeByMails().
Definition at line 534 of file incidencebase.cpp.
int IncidenceBase::attendeeCount | ( | ) | const |
Returns the number of incidence attendees.
Definition at line 488 of file incidencebase.cpp.
Attendee::List IncidenceBase::attendees | ( | ) | const |
Returns a list of incidence attendees.
All pointers in the list are valid.
Definition at line 483 of file incidencebase.cpp.
void IncidenceBase::clearAttendees | ( | ) |
Removes all attendees from the incidence.
Definition at line 493 of file incidencebase.cpp.
void IncidenceBase::clearComments | ( | ) |
Deletes all incidence comments.
Definition at line 379 of file incidencebase.cpp.
void IncidenceBase::clearContacts | ( | ) |
Deletes all incidence contacts.
Definition at line 417 of file incidencebase.cpp.
QStringList IncidenceBase::comments | ( | ) | const |
Returns all incidence comments as a list of strings.
Definition at line 385 of file incidencebase.cpp.
QStringList IncidenceBase::contacts | ( | ) | const |
Returns all incidence contacts as a list of strings.
Definition at line 423 of file incidencebase.cpp.
|
protectedvirtual |
CustomProperties::customPropertyUpdate()
Reimplemented from KCalCore::CustomProperties.
Definition at line 632 of file incidencebase.cpp.
|
protectedvirtual |
CustomProperties::customPropertyUpdated()
Reimplemented from KCalCore::CustomProperties.
Definition at line 637 of file incidencebase.cpp.
|
pure virtual |
Returns a date/time corresponding to the specified DateTimeRole.
- Parameters
-
role is a DateTimeRole.
Implemented in KCalCore::Todo, KCalCore::FreeBusy, KCalCore::Event, and KCalCore::Journal.
void IncidenceBase::deleteAttendee | ( | const Attendee::Ptr & | attendee, |
bool | doUpdate = true |
||
) |
Delete single attendee from the incidence.
The given attendee will be delete()d at the end of this call.
- Parameters
-
attendee The attendee to be removeComment doUpdate If true the Observers are notified, if false they are not.
Definition at line 462 of file incidencebase.cpp.
QSet< IncidenceBase::Field > IncidenceBase::dirtyFields | ( | ) | const |
Returns a QSet with all Fields that were changed since the incidence was created or resetDirtyFields() was called.
- See also
- resetDirtyFields()
Definition at line 652 of file incidencebase.cpp.
|
virtual |
Returns an incidence's starting date/time as a KDateTime.
- See also
- setDtStart().
Reimplemented in KCalCore::Todo.
Definition at line 321 of file incidencebase.cpp.
Duration IncidenceBase::duration | ( | ) | const |
Returns the length of the incidence duration.
- See also
- setDuration()
Definition at line 555 of file incidencebase.cpp.
void IncidenceBase::endUpdates | ( | ) |
Call this when a group of updates is complete, to notify observers that the instance has changed.
This should be called in conjunction with startUpdates().
Definition at line 622 of file incidencebase.cpp.
|
protectedvirtual |
Provides polymorfic comparison for equality.
Only called by IncidenceBase::operator==() which guarantees that incidenceBase
is of the right type.
- Parameters
-
incidenceBase is the IncidenceBase to compare against.
- Returns
- true if the incidences are equal; false otherwise.
Reimplemented in KCalCore::Incidence, KCalCore::Todo, KCalCore::FreeBusy, KCalCore::Event, and KCalCore::Journal.
Definition at line 179 of file incidencebase.cpp.
bool IncidenceBase::hasDuration | ( | ) | const |
Returns true if the incidence has a duration; false otherwise.
- See also
- setHasDuration()
Definition at line 565 of file incidencebase.cpp.
bool IncidenceBase::isReadOnly | ( | ) | const |
Returns true the object is read-only; false otherwise.
- See also
- setReadOnly()
Definition at line 301 of file incidencebase.cpp.
KDateTime IncidenceBase::lastModified | ( | ) | const |
Returns the time the incidence was last modified.
- See also
- setLastModified()
Definition at line 256 of file incidencebase.cpp.
|
static |
Constant that identifies KCalCore data in a binary stream.
static
- Since
- 4.12
Definition at line 673 of file incidencebase.cpp.
|
pure virtual |
Returns the Akonadi specific sub MIME type of a KCalCore::IncidenceBase item, e.g.
getting "application/x-vnd.akonadi.calendar.event" for a KCalCore::Event.
Implemented in KCalCore::Todo, KCalCore::FreeBusy, KCalCore::Event, and KCalCore::Journal.
bool IncidenceBase::operator!= | ( | const IncidenceBase & | ib | ) | const |
Compares this with IncidenceBase ib
for inequality.
- Parameters
-
ib is the IncidenceBase to compare against.
- Returns
- true if the incidences are /not/ equal; false otherwise.
Definition at line 174 of file incidencebase.cpp.
IncidenceBase & IncidenceBase::operator= | ( | const IncidenceBase & | other | ) |
Assignment operator.
All data belonging to derived classes are also copied.
- See also
- assign(). The caller guarantees that both types match.
Dirty field FieldUnknown will be set.
- Parameters
-
other is the IncidenceBase to assign.
Definition at line 142 of file incidencebase.cpp.
bool IncidenceBase::operator== | ( | const IncidenceBase & | ib | ) | const |
Compares this with IncidenceBase ib
for equality.
All data belonging to derived classes are also compared.
- See also
- equals().
- Parameters
-
ib is the IncidenceBase to compare against.
- Returns
- true if the incidences are equal; false otherwise.
Definition at line 164 of file incidencebase.cpp.
Person::Ptr IncidenceBase::organizer | ( | ) | const |
Returns the Person associated with this incidence.
If no Person was set through setOrganizer(), a default Person() is returned.
- See also
- setOrganizer(const QString &), setOrganizer(const Person &)
Definition at line 288 of file incidencebase.cpp.
|
virtual |
Returns the incidence recurrenceId.
- Returns
- incidences recurrenceId value
- See also
- setRecurrenceId().
Reimplemented in KCalCore::Incidence.
Definition at line 642 of file incidencebase.cpp.
void IncidenceBase::registerObserver | ( | IncidenceBase::IncidenceObserver * | observer | ) |
Register observer.
The observer is notified when the observed object changes.
- Parameters
-
observer is a pointer to an IncidenceObserver object that will be watching this incidence.
- See also
- unRegisterObserver()
Definition at line 581 of file incidencebase.cpp.
bool IncidenceBase::removeComment | ( | const QString & | comment | ) |
Removes a comment from the incidence.
Removes the first comment whose string is an exact match for the specified string in comment
.
- Parameters
-
comment is the QString containing the comment to remove.
- Returns
- true if match found, false otherwise.
- See also
- addComment().
Definition at line 360 of file incidencebase.cpp.
bool IncidenceBase::removeContact | ( | const QString & | contact | ) |
Removes a contact from the incidence.
Removes the first contact whose string is an exact match for the specified string in contact
.
- Parameters
-
contact is the QString containing the contact to remove.
- Returns
- true if match found, false otherwise.
- See also
- addContact().
Definition at line 398 of file incidencebase.cpp.
void IncidenceBase::resetDirtyFields | ( | ) |
void IncidenceBase::setAllDay | ( | bool | allDay | ) |
Sets whether the incidence is all-day, i.e.
has a date but no time attached to it.
- Parameters
-
allDay sets whether the incidence is all-day.
- See also
- allDay()
Definition at line 331 of file incidencebase.cpp.
|
pure virtual |
Sets the date/time corresponding to the specified DateTimeRole.
- Parameters
-
dateTime is KDateTime value to set. role is a DateTimeRole.
Implemented in KCalCore::Todo, KCalCore::FreeBusy, KCalCore::Event, and KCalCore::Journal.
void IncidenceBase::setDirtyFields | ( | const QSet< IncidenceBase::Field > & | dirtyFields | ) |
Sets which fields are dirty.
- See also
- dirtyFields()
- Since
- 4.8
Definition at line 667 of file incidencebase.cpp.
|
virtual |
Sets the incidence's starting date/time with a KDateTime.
The incidence's all-day status is set according to whether dtStart
is a date/time (not all-day) or date-only (all-day).
- Parameters
-
dtStart is the incidence start date/time.
- See also
- dtStart().
Reimplemented in KCalCore::Incidence, KCalCore::Todo, KCalCore::FreeBusy, and KCalCore::Event.
Definition at line 306 of file incidencebase.cpp.
|
virtual |
Sets the incidence duration.
- Parameters
-
duration the incidence duration
- See also
- duration()
Reimplemented in KCalCore::Event.
Definition at line 546 of file incidencebase.cpp.
|
protected |
Marks Field field
as dirty.
- Parameters
-
field is the Field type to mark as dirty.
- See also
- dirtyFields()
Definition at line 657 of file incidencebase.cpp.
void IncidenceBase::setHasDuration | ( | bool | hasDuration | ) |
Sets if the incidence has a duration.
- Parameters
-
hasDuration true if the incidence has a duration; false otherwise.
- See also
- hasDuration()
Definition at line 560 of file incidencebase.cpp.
|
virtual |
Sets the time the incidence was last modified to lm
.
It is stored as a UTC date/time.
- Parameters
-
lm is the KDateTime when the incidence was last modified.
- See also
- lastModified()
Reimplemented in KCalCore::Incidence.
Definition at line 240 of file incidencebase.cpp.
void IncidenceBase::setOrganizer | ( | const Person::Ptr & | organizer | ) |
Sets the organizer for the incidence.
- See also
- organizer(), setOrganizer(const QString &)
Definition at line 261 of file incidencebase.cpp.
void IncidenceBase::setOrganizer | ( | const QString & | organizer | ) |
Sets the incidence organizer to any string organizer
.
- Parameters
-
organizer is a string to use as the incidence organizer.
- See also
- organizer(), setOrganizer(const Person &)
Definition at line 276 of file incidencebase.cpp.
|
virtual |
Sets readonly status.
- Parameters
-
readOnly if set, the incidence is read-only; else the incidence can be modified.
- See also
- isReadOnly().
Reimplemented in KCalCore::Incidence.
Definition at line 296 of file incidencebase.cpp.
void IncidenceBase::setUid | ( | const QString & | uid | ) |
Sets the unique id for the incidence to uid
.
- Parameters
-
uid is the string containing the incidence uid.
- See also
- uid()
Definition at line 227 of file incidencebase.cpp.
void IncidenceBase::setUrl | ( | const QUrl & | url | ) |
Sets the incidences url.
This property can be used to point to a more dynamic rendition of the incidence. I.e. a website related to the incidence.
- Parameters
-
url of the incience.
- See also
- url()
- Since
- 4.12
Definition at line 570 of file incidencebase.cpp.
|
virtual |
Shift the times of the incidence so that they appear at the same clock time as before but in a new time zone.
The shift is done from a viewing time zone rather than from the actual incidence time zone.
For example, shifting an incidence whose start time is 09:00 America/New York, using an old viewing time zone (oldSpec
) of Europe/London, to a new time zone (newSpec
) of Europe/Paris, will result in the time being shifted from 14:00 (which is the London time of the incidence start) to 14:00 Paris time.
- Parameters
-
oldSpec the time specification which provides the clock times newSpec the new time specification
Reimplemented in KCalCore::Todo, KCalCore::Incidence, KCalCore::Event, and KCalCore::FreeBusy.
Definition at line 344 of file incidencebase.cpp.
void IncidenceBase::startUpdates | ( | ) |
Call this when a group of updates is going to be made.
This suppresses change notifications until endUpdates() is called, at which point updated() will automatically be called.
Definition at line 616 of file incidencebase.cpp.
|
pure virtual |
Returns the incidence type.
Implemented in KCalCore::FreeBusy, KCalCore::Event, KCalCore::Todo, and KCalCore::Journal.
|
pure virtual |
Prints the type of incidence as a string.
Implemented in KCalCore::FreeBusy, KCalCore::Event, KCalCore::Todo, and KCalCore::Journal.
QString IncidenceBase::uid | ( | ) | const |
Returns the unique id (uid) for the incidence.
- See also
- setUid()
Definition at line 235 of file incidencebase.cpp.
void IncidenceBase::unRegisterObserver | ( | IncidenceBase::IncidenceObserver * | observer | ) |
Unregister observer.
It isn't notified anymore about changes.
- Parameters
-
observer is a pointer to an IncidenceObserver object that will be watching this incidence.
- See also
- registerObserver().
Definition at line 588 of file incidencebase.cpp.
void IncidenceBase::update | ( | ) |
Call this to notify the observers after the IncidenceBase object will be changed.
Definition at line 593 of file incidencebase.cpp.
void IncidenceBase::updated | ( | ) |
Call this to notify the observers after the IncidenceBase object has changed.
Definition at line 604 of file incidencebase.cpp.
KUrl IncidenceBase::uri | ( | ) | const |
Returns the uri for the incidence, of form urn:x-ical:<uid>
Definition at line 662 of file incidencebase.cpp.
QUrl IncidenceBase::url | ( | ) | const |
Returns the url.
- Returns
- incidences url value
- See also
- setUrl()
- Since
- 4.12
Definition at line 576 of file incidencebase.cpp.
|
protectedpure virtual |
Standard trick to add virtuals later.
- Parameters
-
id is any integer unique to this class which we will use to identify the method to be called. data is a pointer to some glob of data, typically a struct. TODO_KDE5: change from int to VirtualHook type.
Reimplemented from KCalCore::CustomProperties.
Implemented in KCalCore::Todo, KCalCore::FreeBusy, KCalCore::Event, and KCalCore::Journal.
Friends And Related Function Documentation
|
friend |
|
friend |
Member Data Documentation
|
protected |
Identifies a read-only incidence.
Definition at line 746 of file incidencebase.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:59:58 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.