KCalCore Library
#include <todo.h>
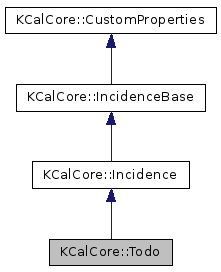
Public Member Functions | |
Todo () | |
Todo (const Todo &other) | |
~Todo () | |
Todo * | clone () const |
KDateTime | completed () const |
KDateTime | dateTime (DateTimeRole role) const |
KDateTime | dtDue (bool first=false) const |
KDateTime | dtRecurrence () const |
virtual KDateTime | dtStart () const |
KDateTime | dtStart (bool first) const |
bool | hasCompletedDate () const |
bool | hasDueDate () const |
bool | hasStartDate () const |
QLatin1String | iconName (const KDateTime &recurrenceId=KDateTime()) const |
bool | isCompleted () const |
bool | isInProgress (bool first) const |
bool | isNotStarted (bool first) const |
bool | isOpenEnded () const |
bool | isOverdue () const |
QLatin1String | mimeType () const |
int | percentComplete () const |
virtual bool | recursOn (const QDate &date, const KDateTime::Spec &timeSpec) const |
void | setAllDay (bool allDay) |
void | setCompleted (bool completed) |
void | setCompleted (const KDateTime &completeDate) |
void | setDateTime (const KDateTime &dateTime, DateTimeRole role) |
void | setDtDue (const KDateTime &dtDue, bool first=false) |
void | setDtRecurrence (const KDateTime &dt) |
void | setDtStart (const KDateTime &dtStart) |
KCALCORE_DEPRECATED void | setHasDueDate (bool hasDueDate) |
KCALCORE_DEPRECATED void | setHasStartDate (bool hasStartDate) |
void | setPercentComplete (int percent) |
virtual void | shiftTimes (const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) |
IncidenceType | type () const |
QByteArray | typeStr () const |
![]() | |
Incidence () | |
virtual | ~Incidence () |
void | addAlarm (const Alarm::Ptr &alarm) |
void | addAttachment (const Attachment::Ptr &attachment) |
Alarm::List | alarms () const |
QString | altDescription () const |
Attachment::List | attachments () const |
Attachment::List | attachments (const QString &mime) const |
QStringList | categories () const |
QString | categoriesStr () const |
void | clearAlarms () |
void | clearAttachments () |
void | clearRecurrence () |
void | clearTempFiles () |
KDateTime | created () const |
QString | customStatus () const |
void | deleteAttachment (const Attachment::Ptr &attachment) |
void | deleteAttachments (const QString &mime) |
QString | description () const |
bool | descriptionIsRich () const |
virtual KDateTime | endDateForStart (const KDateTime &startDt) const |
float | geoLatitude () const |
float | geoLongitude () const |
bool | hasAltDescription () const |
bool | hasEnabledAlarms () const |
bool | hasGeo () const |
bool | hasRecurrenceId () const |
QString | instanceIdentifier () const |
bool | localOnly () const |
QString | location () const |
bool | locationIsRich () const |
Alarm::Ptr | newAlarm () |
int | priority () const |
void | recreate () |
Recurrence * | recurrence () const |
KDateTime | recurrenceId () const |
ushort | recurrenceType () const |
virtual void | recurrenceUpdated (Recurrence *recurrence) |
bool | recurs () const |
bool | recursAt (const KDateTime &dt) const |
QString | relatedTo (RelType relType=RelTypeParent) const |
void | removeAlarm (const Alarm::Ptr &alarm) |
QStringList | resources () const |
int | revision () const |
QString | richDescription () const |
QString | richLocation () const |
QString | richSummary () const |
QString | schedulingID () const |
Secrecy | secrecy () const |
void | setAllDay (bool allDay) |
void | setAltDescription (const QString &altdescription) |
void | setCategories (const QStringList &categories) |
void | setCategories (const QString &catStr) |
void | setCreated (const KDateTime &dt) |
void | setCustomStatus (const QString &status) |
void | setDescription (const QString &description, bool isRich) |
void | setDescription (const QString &description) |
void | setGeoLatitude (float geolatitude) |
void | setGeoLongitude (float geolongitude) |
void | setHasGeo (bool hasGeo) |
void | setLastModified (const KDateTime &lm) |
void | setLocalOnly (bool localonly) |
void | setLocation (const QString &location, bool isRich) |
void | setLocation (const QString &location) |
void | setPriority (int priority) |
void | setReadOnly (bool readonly) |
void | setRecurrenceId (const KDateTime &recurrenceId) |
void | setRelatedTo (const QString &uid, RelType relType=RelTypeParent) |
void | setResources (const QStringList &resources) |
void | setRevision (int rev) |
void | setSchedulingID (const QString &sid, const QString &uid=QString()) |
void | setSecrecy (Secrecy secrecy) |
void | setStatus (Status status) |
void | setSummary (const QString &summary, bool isRich) |
void | setSummary (const QString &summary) |
void | setThisAndFuture (bool thisAndFuture) |
virtual QList< KDateTime > | startDateTimesForDate (const QDate &date, const KDateTime::Spec &timeSpec=KDateTime::LocalZone) const |
virtual QList< KDateTime > | startDateTimesForDateTime (const KDateTime &datetime) const |
Status | status () const |
QString | summary () const |
bool | summaryIsRich () const |
bool | supportsGroupwareCommunication () const |
bool | thisAndFuture () const |
QString | writeAttachmentToTempFile (const Attachment::Ptr &attachment) const |
![]() | |
IncidenceBase () | |
virtual | ~IncidenceBase () |
void | addAttendee (const Attendee::Ptr &attendee, bool doUpdate=true) |
void | addComment (const QString &comment) |
void | addContact (const QString &contact) |
bool | allDay () const |
Attendee::Ptr | attendeeByMail (const QString &email) const |
Attendee::Ptr | attendeeByMails (const QStringList &emails, const QString &email=QString()) const |
Attendee::Ptr | attendeeByUid (const QString &uid) const |
int | attendeeCount () const |
Attendee::List | attendees () const |
void | clearAttendees () |
void | clearComments () |
void | clearContacts () |
QStringList | comments () const |
QStringList | contacts () const |
void | deleteAttendee (const Attendee::Ptr &attendee, bool doUpdate=true) |
QSet< IncidenceBase::Field > | dirtyFields () const |
Duration | duration () const |
void | endUpdates () |
bool | hasDuration () const |
bool | isReadOnly () const |
KDateTime | lastModified () const |
bool | operator!= (const IncidenceBase &ib) const |
IncidenceBase & | operator= (const IncidenceBase &other) |
bool | operator== (const IncidenceBase &ib) const |
Person::Ptr | organizer () const |
void | registerObserver (IncidenceObserver *observer) |
bool | removeComment (const QString &comment) |
bool | removeContact (const QString &contact) |
void | resetDirtyFields () |
void | setAllDay (bool allDay) |
void | setDirtyFields (const QSet< IncidenceBase::Field > &) |
virtual void | setDuration (const Duration &duration) |
void | setHasDuration (bool hasDuration) |
void | setOrganizer (const Person::Ptr &organizer) |
void | setOrganizer (const QString &organizer) |
void | setUid (const QString &uid) |
void | setUrl (const QUrl &url) |
void | startUpdates () |
QString | uid () const |
void | unRegisterObserver (IncidenceObserver *observer) |
void | update () |
void | updated () |
KUrl | uri () const |
QUrl | url () const |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &other) | |
virtual | ~CustomProperties () |
QMap< QByteArray, QString > | customProperties () const |
QString | customProperty (const QByteArray &app, const QByteArray &key) const |
QString | nonKDECustomProperty (const QByteArray &name) const |
QString | nonKDECustomPropertyParameters (const QByteArray &name) const |
CustomProperties & | operator= (const CustomProperties &other) |
bool | operator== (const CustomProperties &properties) const |
void | removeCustomProperty (const QByteArray &app, const QByteArray &key) |
void | removeNonKDECustomProperty (const QByteArray &name) |
void | setCustomProperties (const QMap< QByteArray, QString > &properties) |
void | setCustomProperty (const QByteArray &app, const QByteArray &key, const QString &value) |
void | setNonKDECustomProperty (const QByteArray &name, const QString &value, const QString ¶meters=QString()) |
Static Public Member Functions | |
static QLatin1String | todoMimeType () |
![]() | |
static QStringList | mimeTypes () |
![]() | |
static quint32 | magicSerializationIdentifier () |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
Protected Member Functions | |
virtual IncidenceBase & | assign (const IncidenceBase &other) |
virtual bool | equals (const IncidenceBase &todo) const |
virtual void | virtual_hook (int id, void *data) |
![]() | |
Incidence (const Incidence &other) | |
void | deserialize (QDataStream &in) |
void | serialize (QDataStream &out) |
![]() | |
IncidenceBase (const IncidenceBase &ib) | |
virtual void | customPropertyUpdate () |
virtual void | customPropertyUpdated () |
void | setFieldDirty (IncidenceBase::Field field) |
Additional Inherited Members | |
![]() | |
enum | VirtualHook { SerializerHook, DeserializerHook } |
![]() | |
bool | mReadOnly |
Detailed Description
Member Typedef Documentation
typedef QVector<Ptr> KCalCore::Todo::List |
typedef QSharedPointer<Todo> KCalCore::Todo::Ptr |
Constructor & Destructor Documentation
KCalCore::Todo::Todo | ( | ) |
Constructs an empty to-do.
KCalCore::Todo::Todo | ( | const Todo & | other | ) |
Copy constructor.
- Parameters
-
other is the to-do to copy.
KCalCore::Todo::~Todo | ( | ) |
Destroys a to-do.
Member Function Documentation
|
protectedvirtual |
Provides polymorfic assignment.
- Parameters
-
other is the IncidenceBase to assign.
Reimplemented from KCalCore::Incidence.
|
virtual |
Returns an exact copy of this todo.
The returned object is owned by the caller.
- Returns
- A pointer to a Todo containing an exact copy of this object.
Implements KCalCore::Incidence.
KDateTime Todo::completed | ( | ) | const |
Returns the to-do was completion datetime.
- Returns
- A KDateTime for the completeion datetime of the to-do.
- See also
- hasCompletedDate()
|
virtual |
Returns a date/time corresponding to the specified DateTimeRole.
- Parameters
-
role is a DateTimeRole.
Implements KCalCore::IncidenceBase.
KDateTime Todo::dtDue | ( | bool | first = false | ) | const |
Returns the todo due datetime.
- Parameters
-
first If true and the todo recurs, the due datetime of the first occurrence will be returned. If false and recurrent, the datetime of the current occurrence will be returned. If non-recurrent, the normal due datetime will be returned.
- Returns
- A KDateTime containing the todo due datetime.
KDateTime Todo::dtRecurrence | ( | ) | const |
|
virtual |
Returns an incidence's starting date/time as a KDateTime.
- See also
- setDtStart().
Reimplemented from KCalCore::IncidenceBase.
KDateTime Todo::dtStart | ( | bool | first | ) | const |
Returns the start datetime of the todo.
- Parameters
-
first If true, the start datetime of the todo will be returned; also, if the todo recurs, the start datetime of the first occurrence will be returned. If false and the todo recurs, the relative start datetime will be returned, based on the datetime returned by dtRecurrence().
- Returns
- A KDateTime for the start datetime of the todo.
|
protectedvirtual |
Compare this with todo
for equality.
- Parameters
-
todo is the to-do to compare.
Reimplemented from KCalCore::Incidence.
bool Todo::hasCompletedDate | ( | ) | const |
Returns if the to-do has a completion datetime.
- Returns
- true if the to-do has a date associated with completion; false otherwise.
- See also
- setCompleted(), completed()
bool Todo::hasDueDate | ( | ) | const |
bool Todo::hasStartDate | ( | ) | const |
|
virtual |
Returns the name of the icon that best represents this incidence.
- Parameters
-
recurrenceId Some recurring incidences might use a different icon, for example, completed to-do occurrences. Use this parameter to identify the specific occurrence in a recurring serie.
Implements KCalCore::Incidence.
bool Todo::isCompleted | ( | ) | const |
Returns if the todo is 100% completed.
- Returns
- true if the todo is 100% completed; false otherwise.
- See also
- isOverdue, isInProgress(), isOpenEnded(), isNotStarted(bool), setCompleted(), percentComplete()
bool Todo::isInProgress | ( | bool | first | ) | const |
Returns true, if the to-do is in-progress (started, or >0% completed); otherwise return false.
If the to-do is overdue, then it is not considered to be in-progress.
- Parameters
-
first If true, the start and due dates of the todo will be used; also, if the todo recurs, the start date and due date of the first occurrence will be used. If false and the todo recurs, the relative start and due dates will be used, based on the date returned by dtRecurrence().
- See also
- isOverdue(), isCompleted(), isOpenEnded(), isNotStarted(bool)
bool Todo::isNotStarted | ( | bool | first | ) | const |
Returns true, if the to-do has yet to be started (no start date and 0% completed); otherwise return false.
- Parameters
-
first If true, the start date of the todo will be used; also, if the todo recurs, the start date of the first occurrence will be used. If false and the todo recurs, the relative start date will be used, based on the date returned by dtRecurrence().
- See also
- isOverdue(), isCompleted(), isInProgress(), isOpenEnded()
bool Todo::isOpenEnded | ( | ) | const |
Returns true, if the to-do is open-ended (no due date); false otherwise.
- See also
- isOverdue(), isCompleted(), isInProgress(), isNotStarted(bool)
bool Todo::isOverdue | ( | ) | const |
Returns true if this todo is overdue (e.g.
due date is lower than today and not completed), else false.
- See also
- isCompleted(), isInProgress(), isOpenEnded(), isNotStarted(bool)
|
virtual |
Returns the Akonadi specific sub MIME type of a KCalCore::IncidenceBase item, e.g.
getting "application/x-vnd.akonadi.calendar.event" for a KCalCore::Event.
Implements KCalCore::IncidenceBase.
int Todo::percentComplete | ( | ) | const |
Returns what percentage of the to-do is completed.
- Returns
- The percentage complete of the to-do as an integer between 0 and 100, inclusive.
- See also
- setPercentComplete(), isCompleted()
|
virtual |
Returns true if the date
specified is one on which the to-do will recur.
Todos are a special case, hence the overload. It adds an extra check, which make it return false if there's an occurrence between the recur start and today.
- Parameters
-
date is the date to check. timeSpec is the
Reimplemented from KCalCore::Incidence.
void Todo::setCompleted | ( | bool | completed | ) |
Sets completed state.
- Parameters
-
completed If true set completed state to 100%, if false set completed state to 0%.
- See also
- isCompleted(), percentComplete()
void Todo::setCompleted | ( | const KDateTime & | completeDate | ) |
Sets date and time of completion.
- Parameters
-
completeDate is the to-do completion date.
- See also
- completed(), hasCompletedDate()
|
virtual |
Sets the date/time corresponding to the specified DateTimeRole.
- Parameters
-
dateTime is KDateTime value to set. role is a DateTimeRole.
Implements KCalCore::IncidenceBase.
void KCalCore::Todo::setDtDue | ( | const KDateTime & | dtDue, |
bool | first = false |
||
) |
Sets due date and time.
- Parameters
-
dtDue The due date/time. first If true and the todo recurs, the due date of the first occurrence will be returned. If false and recurrent, the date of the current occurrence will be returned. If non-recurrent, the normal due date will be returned.
void Todo::setDtRecurrence | ( | const KDateTime & | dt | ) |
|
virtual |
Sets the start datetime of the todo.
- Parameters
-
dtStart is the to-do start datetime.
Reimplemented from KCalCore::Incidence.
void Todo::setHasDueDate | ( | bool | hasDueDate | ) |
Sets if the todo has a due datetime.
- Parameters
-
hasDueDate true if todo has a due datetime, otherwise false
- Deprecated:
- Use setDtDue( KDateTime() )
void Todo::setHasStartDate | ( | bool | hasStartDate | ) |
Sets if the todo has a start datetime.
- Parameters
-
hasStartDate true if todo has a start datetime, otherwise false.
- Deprecated:
- Use setDtStart( KDateTime() )
void Todo::setPercentComplete | ( | int | percent | ) |
Sets what percentage of the to-do is completed.
Valid values are in the range from 0 to 100.
- Parameters
-
percent is the completion percentage, which as integer value between 0 and 100, inclusive.
- See also
- isCompleted(), setCompleted()
|
virtual |
Shift the times of the incidence so that they appear at the same clock time as before but in a new time zone.
The shift is done from a viewing time zone rather than from the actual incidence time zone.
For example, shifting an incidence whose start time is 09:00 America/New York, using an old viewing time zone (oldSpec
) of Europe/London, to a new time zone (newSpec
) of Europe/Paris, will result in the time being shifted from 14:00 (which is the London time of the incidence start) to 14:00 Paris time.
- Parameters
-
oldSpec the time specification which provides the clock times newSpec the new time specification
Reimplemented from KCalCore::Incidence.
|
static |
Returns the Akonadi specific sub MIME type of a KCalCore::Todo.
|
virtual |
Returns the incidence type.
Implements KCalCore::IncidenceBase.
|
virtual |
Prints the type of incidence as a string.
Implements KCalCore::IncidenceBase.
|
protectedvirtual |
Standard trick to add virtuals later.
- Parameters
-
id is any integer unique to this class which we will use to identify the method to be called. data is a pointer to some glob of data, typically a struct. TODO_KDE5: change from int to VirtualHook type.
Implements KCalCore::IncidenceBase.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:59:58 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.