okteta
#include <bytearrayrowcolumnrenderer.h>
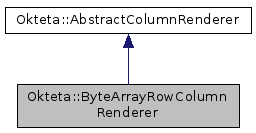
Public Types | |
enum | FrameStyle { Frame, Left, Right } |
Protected Member Functions | |
bool | getNextMarkedAddressRange (AddressRange *markedRange, unsigned int *flag, const AddressRange &range) const |
bool | getNextSelectedAddressRange (AddressRange *selectedRange, unsigned int *flag, const AddressRange &range) const |
void | recalcByteWidth () |
void | recalcX () |
void | renderBookmark (QPainter *painter, const QBrush &brush) |
void | renderByteText (QPainter *painter, Byte byte, Character charByte, int codings, const QColor &color) const |
void | renderCode (QPainter *painter, const QString &code, const QColor &color) const |
void | renderMarking (QPainter *painter, const LinePositionRange &linePositions, Address byteIndex, int flag) |
void | renderPlain (QPainter *painter, const LinePositionRange &linePositions, Address byteIndex) |
void | renderRange (QPainter *painter, const QBrush &brush, const LinePositionRange &linePositions, int flag) |
void | renderSelection (QPainter *painter, const LinePositionRange &linePositions, Address byteIndex, int flag) |
void | renderSelectionSpaceBehind (QPainter *painter, LinePosition linePosition) |
void | renderSpaceBehind (QPainter *painter, const QBrush &brush, LinePosition linePosition) |
![]() | |
void | renderBlankLine (QPainter *painter) const |
void | restrictToXSpan (PixelXRange *xSpan) const |
void | setWidth (PixelX width) |
Detailed Description
base class of all buffer column displayers holds all information about the vertical layout of a buffer column knows how to paint the data and the editing things (focus, cursor, selection) but does not offer
Definition at line 62 of file bytearrayrowcolumnrenderer.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Frame | |
Left | |
Right |
Definition at line 65 of file bytearrayrowcolumnrenderer.h.
Constructor & Destructor Documentation
Okteta::ByteArrayRowColumnRenderer::ByteArrayRowColumnRenderer | ( | AbstractColumnStylist * | stylist, |
AbstractByteArrayModel * | byteArrayModel, | ||
ByteArrayTableLayout * | layout, | ||
ByteArrayTableRanges * | ranges | ||
) |
Definition at line 52 of file bytearrayrowcolumnrenderer.cpp.
|
virtual |
Definition at line 950 of file bytearrayrowcolumnrenderer.cpp.
Member Function Documentation
|
inline |
Definition at line 347 of file bytearrayrowcolumnrenderer.h.
QRect Okteta::ByteArrayRowColumnRenderer::byteRect | ( | const Coord & | coord | ) | const |
Definition at line 493 of file bytearrayrowcolumnrenderer.cpp.
QRect Okteta::ByteArrayRowColumnRenderer::byteRect | ( | const Coord & | coord, |
AbstractByteArrayView::CodingTypeId | codingId | ||
) | const |
Definition at line 507 of file bytearrayrowcolumnrenderer.cpp.
|
inline |
Definition at line 325 of file bytearrayrowcolumnrenderer.h.
|
inline |
Definition at line 323 of file bytearrayrowcolumnrenderer.h.
AbstractByteArrayView::CodingTypeId Okteta::ByteArrayRowColumnRenderer::codingIdofY | ( | PixelY | y | ) | const |
Definition at line 80 of file bytearrayrowcolumnrenderer.cpp.
PixelX Okteta::ByteArrayRowColumnRenderer::columnRightXOfLinePosition | ( | LinePosition | posInLine | ) | const |
returns right relative x-coord of byte at position posInLine
Definition at line 467 of file bytearrayrowcolumnrenderer.cpp.
PixelX Okteta::ByteArrayRowColumnRenderer::columnXOfLinePosition | ( | LinePosition | posInLine | ) | const |
returns relative x-coord of byte at position posInLine
Definition at line 462 of file bytearrayrowcolumnrenderer.cpp.
PixelXRange Okteta::ByteArrayRowColumnRenderer::columnXsOfLinePositionsInclSpaces | ( | const LinePositionRange & | linePositions | ) | const |
Definition at line 483 of file bytearrayrowcolumnrenderer.cpp.
|
inline |
Definition at line 327 of file bytearrayrowcolumnrenderer.h.
|
inline |
Definition at line 324 of file bytearrayrowcolumnrenderer.h.
|
inline |
Definition at line 330 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 922 of file bytearrayrowcolumnrenderer.cpp.
|
protected |
Definition at line 892 of file bytearrayrowcolumnrenderer.cpp.
|
inline |
Definition at line 326 of file bytearrayrowcolumnrenderer.h.
|
inline |
Definition at line 345 of file bytearrayrowcolumnrenderer.h.
|
inline |
returns true if "unprintable" chars (>32) are displayed in the char column with their corresponding character, default is false
Definition at line 349 of file bytearrayrowcolumnrenderer.h.
|
inline |
Definition at line 331 of file bytearrayrowcolumnrenderer.h.
|
inline |
Definition at line 334 of file bytearrayrowcolumnrenderer.h.
LinePosition Okteta::ByteArrayRowColumnRenderer::linePositionOfColumnX | ( | PixelX | x | ) | const |
returns byte pos at pixel with relative x-coord x
Definition at line 421 of file bytearrayrowcolumnrenderer.cpp.
LinePosition Okteta::ByteArrayRowColumnRenderer::linePositionOfX | ( | PixelX | x | ) | const |
returns byte pos at pixel with absolute x-coord x
Definition at line 345 of file bytearrayrowcolumnrenderer.cpp.
LinePositionRange Okteta::ByteArrayRowColumnRenderer::linePositionsOfColumnXs | ( | PixelX | x, |
PixelX | width | ||
) | const |
returns byte linePositions covered by pixels with relative x-coord x
Definition at line 435 of file bytearrayrowcolumnrenderer.cpp.
LinePositionRange Okteta::ByteArrayRowColumnRenderer::linePositionsOfX | ( | PixelX | x, |
PixelX | width | ||
) | const |
returns byte linePositions covered by pixels with absolute x-coord x
Definition at line 382 of file bytearrayrowcolumnrenderer.cpp.
LinePosition Okteta::ByteArrayRowColumnRenderer::magneticLinePositionOfX | ( | PixelX | x | ) | const |
returns byte pos at pixel with absolute x-coord x, and sets the flag to true if we are closer to the right
Definition at line 361 of file bytearrayrowcolumnrenderer.cpp.
|
inline |
Definition at line 328 of file bytearrayrowcolumnrenderer.h.
void Okteta::ByteArrayRowColumnRenderer::prepareRendering | ( | const PixelXRange & | Xs | ) |
Definition at line 521 of file bytearrayrowcolumnrenderer.cpp.
|
protected |
default implementation sets byte width to one digit width
Definition at line 243 of file bytearrayrowcolumnrenderer.cpp.
|
protected |
Definition at line 315 of file bytearrayrowcolumnrenderer.cpp.
|
protected |
Definition at line 775 of file bytearrayrowcolumnrenderer.cpp.
void Okteta::ByteArrayRowColumnRenderer::renderByte | ( | QPainter * | painter, |
Address | byteIndex, | ||
AbstractByteArrayView::CodingTypeId | codingId | ||
) |
paints the byte with background.
- Parameters
-
byteIndex Index of the byte to paint. If -1 only the background is painted.
Definition at line 804 of file bytearrayrowcolumnrenderer.cpp.
|
protected |
Definition at line 275 of file bytearrayrowcolumnrenderer.cpp.
|
protected |
Definition at line 301 of file bytearrayrowcolumnrenderer.cpp.
void Okteta::ByteArrayRowColumnRenderer::renderCursor | ( | QPainter * | painter, |
Address | byteIndex, | ||
AbstractByteArrayView::CodingTypeId | codingId | ||
) |
paints a cursor based on the type of the byte.
- Parameters
-
byteIndex Index of the byte to paint the cursor for. If -1 a space is used as char.
Definition at line 874 of file bytearrayrowcolumnrenderer.cpp.
void Okteta::ByteArrayRowColumnRenderer::renderEditedByte | ( | QPainter * | painter, |
Byte | byte, | ||
const QString & | editBuffer | ||
) |
Definition at line 258 of file bytearrayrowcolumnrenderer.cpp.
|
virtual |
Definition at line 537 of file bytearrayrowcolumnrenderer.cpp.
void Okteta::ByteArrayRowColumnRenderer::renderFramedByte | ( | QPainter * | painter, |
Address | byteIndex, | ||
AbstractByteArrayView::CodingTypeId | codingId, | ||
FrameStyle | style | ||
) |
paints the byte with background and a frame around.
- Parameters
-
byteIndex Index of the byte to paint the frame for. If -1 a space is used as char. style the style of the framing
Definition at line 846 of file bytearrayrowcolumnrenderer.cpp.
void Okteta::ByteArrayRowColumnRenderer::renderLinePositions | ( | QPainter * | painter, |
Line | lineIndex, | ||
const LinePositionRange & | linePositions | ||
) |
Definition at line 553 of file bytearrayrowcolumnrenderer.cpp.
|
protected |
Definition at line 752 of file bytearrayrowcolumnrenderer.cpp.
|
virtual |
the actual painting call for a column's line.
The default implementation simply paints the background
Reimplemented from Okteta::AbstractColumnRenderer.
Definition at line 547 of file bytearrayrowcolumnrenderer.cpp.
|
protected |
Definition at line 652 of file bytearrayrowcolumnrenderer.cpp.
|
protected |
Definition at line 782 of file bytearrayrowcolumnrenderer.cpp.
|
protected |
Definition at line 697 of file bytearrayrowcolumnrenderer.cpp.
|
protected |
Definition at line 743 of file bytearrayrowcolumnrenderer.cpp.
|
protected |
Definition at line 795 of file bytearrayrowcolumnrenderer.cpp.
void Okteta::ByteArrayRowColumnRenderer::resetXBuffer | ( | ) |
creates new buffer for x-values; to be called on any change of NoOfBytesPerLine or metrics
Definition at line 113 of file bytearrayrowcolumnrenderer.cpp.
PixelX Okteta::ByteArrayRowColumnRenderer::rightXOfLinePosition | ( | LinePosition | posInLine | ) | const |
returns right absolute x-coord of byte at position posInLine
Definition at line 415 of file bytearrayrowcolumnrenderer.cpp.
PixelY Okteta::ByteArrayRowColumnRenderer::rowHeight | ( | ) | const |
Definition at line 90 of file bytearrayrowcolumnrenderer.cpp.
void Okteta::ByteArrayRowColumnRenderer::set | ( | AbstractByteArrayModel * | byteArrayModel | ) |
Definition at line 106 of file bytearrayrowcolumnrenderer.cpp.
bool Okteta::ByteArrayRowColumnRenderer::setBinaryGapWidth | ( | PixelX | binaryGapWidth | ) |
sets the spacing in the middle of a binary byte in the value column
- Parameters
-
binaryGapWidth spacing in the middle of a binary in pixels returns true if there was a change
Definition at line 226 of file bytearrayrowcolumnrenderer.cpp.
bool Okteta::ByteArrayRowColumnRenderer::setByteSpacingWidth | ( | PixelX | byteSpacingWidth | ) |
sets the spacing between the bytes in the hex column
- Parameters
-
byteSpacingWidth spacing between the bytes in pixels returns true if there was a change
Definition at line 166 of file bytearrayrowcolumnrenderer.cpp.
|
inline |
Definition at line 341 of file bytearrayrowcolumnrenderer.h.
|
inline |
sets the codec to be used by the char column.
Definition at line 336 of file bytearrayrowcolumnrenderer.h.
void Okteta::ByteArrayRowColumnRenderer::setFontMetrics | ( | const QFontMetrics & | fontMetrics | ) |
sets the metrics of the used font
Definition at line 132 of file bytearrayrowcolumnrenderer.cpp.
bool Okteta::ByteArrayRowColumnRenderer::setGroupSpacingWidth | ( | PixelX | groupSpacingWidth | ) |
sets the spacing between the groups of bytes in the hex column
- Parameters
-
groupSpacingWidth spacing between the groups in pixels returns true if there was a change
Definition at line 196 of file bytearrayrowcolumnrenderer.cpp.
bool Okteta::ByteArrayRowColumnRenderer::setNoOfGroupedBytes | ( | Size | noOfGroupedBytes | ) |
sets the number of grouped bytes in the hex column
- Parameters
-
noOfGroupedBytes numbers of grouped bytes, 0 means no grouping returns true if there was a change
Definition at line 182 of file bytearrayrowcolumnrenderer.cpp.
|
inline |
sets whether "unprintable" chars (>32) should be displayed in the char column with their corresponding character.
- Parameters
-
showingNonprinting returns true if there was a change
Definition at line 371 of file bytearrayrowcolumnrenderer.h.
bool Okteta::ByteArrayRowColumnRenderer::setSpacing | ( | PixelX | byteSpacingWidth, |
Size | noOfGroupedBytes = 0 , |
||
PixelX | groupSpacingWidth = 0 |
||
) |
sets the spacing in the hex column
- Parameters
-
byteSpacingWidth spacing between the bytes in pixels noOfGroupedBytes numbers of grouped bytes, 0 means no grouping groupSpacingWidth spacing between the groups in pixels returns true if there was a change
Definition at line 148 of file bytearrayrowcolumnrenderer.cpp.
|
inline |
sets the substitute character for "unprintable" chars returns true if there was a change
Definition at line 353 of file bytearrayrowcolumnrenderer.h.
|
inline |
sets the undefined character for "undefined" chars returns true if there was a change
Definition at line 362 of file bytearrayrowcolumnrenderer.h.
void Okteta::ByteArrayRowColumnRenderer::setValueCodec | ( | ValueCoding | valueCoding, |
const ValueCodec * | valueCodec | ||
) |
Definition at line 212 of file bytearrayrowcolumnrenderer.cpp.
void Okteta::ByteArrayRowColumnRenderer::setVisibleCodings | ( | int | visibleCodings | ) |
Definition at line 126 of file bytearrayrowcolumnrenderer.cpp.
|
inline |
returns the actually used substitute character for "unprintable" chars, default is '.
'
Definition at line 350 of file bytearrayrowcolumnrenderer.h.
|
inline |
returns the actually used undefined character for "undefined" chars, default is '?'
Definition at line 351 of file bytearrayrowcolumnrenderer.h.
|
inline |
Definition at line 320 of file bytearrayrowcolumnrenderer.h.
LinePositionRange Okteta::ByteArrayRowColumnRenderer::visibleLinePositions | ( | PixelX | x, |
PixelX | width | ||
) | const |
returns the linePositions that overlap with the x-coords relative to the view
|
inline |
Definition at line 332 of file bytearrayrowcolumnrenderer.h.
PixelX Okteta::ByteArrayRowColumnRenderer::xOfLinePosition | ( | LinePosition | posInLine | ) | const |
returns absolute x-coord of byte at position posInLine
Definition at line 410 of file bytearrayrowcolumnrenderer.cpp.
PixelXRange Okteta::ByteArrayRowColumnRenderer::xsOfLinePositionsInclSpaces | ( | const LinePositionRange & | linePositions | ) | const |
returns the
Definition at line 473 of file bytearrayrowcolumnrenderer.cpp.
PixelY Okteta::ByteArrayRowColumnRenderer::yOfCodingId | ( | AbstractByteArrayView::CodingTypeId | codingId | ) | const |
Definition at line 96 of file bytearrayrowcolumnrenderer.cpp.
Member Data Documentation
|
protected |
Definition at line 296 of file bytearrayrowcolumnrenderer.h.
|
protected |
calculated: Offset in pixels of the second half of the binary
Definition at line 302 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 251 of file bytearrayrowcolumnrenderer.h.
|
protected |
pointer to the buffer
Definition at line 245 of file bytearrayrowcolumnrenderer.h.
|
protected |
width of inserting cursor in pixel
size of the line margin
Definition at line 272 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 288 of file bytearrayrowcolumnrenderer.h.
|
protected |
total width of byte display in pixel
Definition at line 268 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 253 of file bytearrayrowcolumnrenderer.h.
|
mutableprotected |
buffer to hold the formatted valueCoding
Definition at line 300 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 259 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 261 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 257 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 263 of file bytearrayrowcolumnrenderer.h.
|
protected |
width of spacing in pixel
Definition at line 274 of file bytearrayrowcolumnrenderer.h.
|
protected |
index of right position
Definition at line 285 of file bytearrayrowcolumnrenderer.h.
|
protected |
pointer to the layout
Definition at line 247 of file bytearrayrowcolumnrenderer.h.
|
protected |
pointer to array with buffered linePositions (relative to column position) any spacing gets assigned to the byte left to it -> ...|c|c|c |c|c...
Definition at line 282 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 283 of file bytearrayrowcolumnrenderer.h.
|
protected |
number of grouped bytes
Definition at line 277 of file bytearrayrowcolumnrenderer.h.
|
protected |
pointer to the ranges
Definition at line 249 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 314 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 313 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 316 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 315 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 306 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 317 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 308 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 310 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 294 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 292 of file bytearrayrowcolumnrenderer.h.
|
protected |
Definition at line 255 of file bytearrayrowcolumnrenderer.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:04:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.