superkaramba
#include <karambainterface.h>
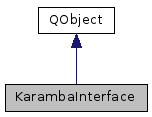
Public Slots | |
bool | acceptDrops (Karamba *k) const |
bool | addImageTooltip (const Karamba *k, ImageLabel *image, const QString &text) const |
bool | addMenuConfigOption (Karamba *k, const QString &key, const QString &name) const |
QObject * | addMenuItem (Karamba *k, KMenu *menu, const QString &text, const QString &icon) const |
QObject * | addMenuSeparator (Karamba *k, KMenu *menu) const |
bool | attachClickArea (const Karamba *k, Meter *m, const QString &leftButton=QString(), const QString &middleButton=QString(), const QString &rightButton=QString()) const |
bool | callTheme (Karamba *k, const QString &theme, const QString &info) const |
bool | changeImageAlpha (const Karamba *k, ImageLabel *image, int a, int r=-1, int g=-1, int b=-1, int ms=0) const |
bool | changeImageAnimation (Karamba *k, ImageLabel *image, bool enable) const |
bool | changeImageChannelIntensity (const Karamba *k, ImageLabel *image, double ratio, const QString &channel, int ms=0) const |
bool | changeImageIntensity (const Karamba *k, ImageLabel *image, double ratio, int ms=0) const |
bool | changeImageToGray (const Karamba *k, ImageLabel *image, int ms=0) const |
QObject * | changeInputBox (const Karamba *k, Input *input, const QString &text) const |
bool | changeInputBoxBackgroundColor (const Karamba *k, Input *input, int red, int green, int blue, int alpha=255) const |
bool | changeInputBoxFont (const Karamba *k, Input *input, const QString &font) const |
bool | changeInputBoxFontColor (const Karamba *k, Input *input, int red, int green, int blue, int alpha=255) const |
bool | changeInputBoxFontSize (const Karamba *k, Input *input, int size) const |
bool | changeInputBoxFrameColor (const Karamba *k, Input *input, int red, int green, int blue, int alpha=255) const |
bool | changeInputBoxSelectedTextColor (const Karamba *k, Input *input, int red, int green, int blue, int alpha=255) const |
bool | changeInputBoxSelection (const Karamba *k, Input *input, int start, int length) const |
bool | changeInputBoxSelectionColor (const Karamba *k, Input *input, int red, int green, int blue, int alpha=255) const |
bool | changeInterval (Karamba *k, int interval) const |
QObject * | changeRichText (const Karamba *k, RichTextLabel *label, const QString &text) const |
bool | changeRichTextFont (const Karamba *k, RichTextLabel *label, const QString &font) const |
bool | changeRichTextSize (const Karamba *k, RichTextLabel *label, int size) const |
QObject * | changeText (const Karamba *k, TextLabel *label, const QString &text) const |
bool | changeTextColor (const Karamba *k, TextLabel *text, int red, int green, int blue, int alpha=255) const |
bool | changeTextFont (const Karamba *k, TextLabel *text, const QString &font) const |
bool | changeTextShadow (const Karamba *k, TextLabel *label, int shadow) const |
bool | changeTextSize (const Karamba *k, TextLabel *text, int size) const |
bool | clearInputBoxSelection (const Karamba *k, Input *input) const |
bool | clearInputFocus (const Karamba *k, Input *input) const |
QObject * | createBackgroundImage (Karamba *k, int x, int y, const QString &imagePath) const |
QObject * | createBar (Karamba *k, int x, int y, int w, int h, const QString &path="") const |
QObject * | createCanvasWidget (Karamba *k, QWidget *widget) |
QObject * | createClickArea (Karamba *k, int x, int y, int width, int height, const QString &onClick) const |
QObject * | createEmptyImage (Karamba *k, int x, int y, int w, int h) const |
QObject * | createGraph (Karamba *k, int x, int y, int w, int h, int points) const |
QObject * | createImage (Karamba *k, int x, int y, const QString &image) const |
QObject * | createInputBox (Karamba *k, int x, int y, int w, int h, const QString &text) const |
QObject * | createMenu (Karamba *k) const |
QObject * | createRichText (Karamba *k, const QString &text, bool underline=false) const |
QObject * | createServiceClickArea (Karamba *k, int x, int y, int width, int height, const QString &name, const QString &exec, const QString &icon) const |
QObject * | createSystray (const Karamba *k, int x, int y, int w, int h) const |
QObject * | createTaskIcon (Karamba *k, int x, int y, int ctask) const |
QObject * | createText (Karamba *k, int x, int y, int width, int height, const QString &text="") const |
bool | createWidgetMask (const Karamba *k, const QString &mask) const |
bool | deleteBar (Karamba *k, Bar *bar) const |
bool | deleteGraph (Karamba *k, Graph *graph) const |
bool | deleteImage (Karamba *k, ImageLabel *image) const |
bool | deleteInputBox (Karamba *k, Input *input) const |
bool | deleteMenu (Karamba *k, KMenu *menu) const |
bool | deleteRichText (Karamba *k, RichTextLabel *label) const |
bool | deleteText (Karamba *k, TextLabel *text) const |
QVariantList | desktopSize () const |
int | execute (const QString &command) const |
int | executeInteractive (Karamba *k, const QStringList &command) |
QString | getBarImage (const Karamba *k, const Bar *bar) const |
QVariantList | getBarMinMax (const Karamba *k, const Bar *bar) const |
QVariantList | getBarPos (const Karamba *k, const Bar *bar) const |
QString | getBarSensor (const Karamba *k, const Bar *bar) const |
QVariantList | getBarSize (const Karamba *k, const Bar *bar) const |
int | getBarValue (const Karamba *k, const Bar *bar) const |
bool | getBarVertical (const Karamba *k, const Bar *bar) const |
int | getCurrentWindowCount (const Karamba *k) const |
QString | getExecutingApplication () const |
QVariantList | getGraphColor (const Karamba *k, const Graph *graph) const |
QVariantList | getGraphFillColor (const Karamba *k, const Graph *graph) const |
QVariantList | getGraphMinMax (const Karamba *k, const Graph *graph) const |
QString | getGraphPlot (const Karamba *k, const Graph *graph) const |
QVariantList | getGraphPos (const Karamba *k, const Graph *graph) const |
QString | getGraphScroll (const Karamba *k, const Graph *graph) const |
QString | getGraphSensor (const Karamba *k, const Graph *graph) const |
bool | getGraphShouldFill (const Karamba *k, const Graph *graph) const |
QVariantList | getGraphSize (const Karamba *k, const Graph *graph) const |
int | getGraphValue (const Karamba *k, const Graph *graph) const |
QString | getIconByName (const QString &icon, int size) const |
bool | getImageAnimation (Karamba *k, ImageLabel *image) const |
QString | getImageElement (Karamba *k, ImageLabel *image) const |
int | getImageHeight (const Karamba *k, const ImageLabel *image) const |
QString | getImagePath (const Karamba *k, const ImageLabel *image) const |
QVariantList | getImagePos (const Karamba *k, const ImageLabel *image) const |
QString | getImageSensor (const Karamba *k, const ImageLabel *image) const |
QVariantList | getImageSize (const Karamba *k, const ImageLabel *image) const |
int | getImageWidth (const Karamba *k, const ImageLabel *image) const |
QString | getIncomingData (const Karamba *k) const |
QVariantList | getInputBoxBackgroundColor (const Karamba *k, const Input *input) const |
QString | getInputBoxFont (const Karamba *k, const Input *input) const |
QVariantList | getInputBoxFontColor (const Karamba *k, const Input *input) const |
int | getInputBoxFontSize (const Karamba *k, const Input *input) const |
QVariantList | getInputBoxFrameColor (const Karamba *k, const Input *input) const |
QVariantList | getInputBoxPos (const Karamba *k, const Input *input) const |
QVariantList | getInputBoxSelectedTextColor (const Karamba *k, const Input *input) const |
QVariantList | getInputBoxSelection (const Karamba *k, Input *input) const |
QVariantList | getInputBoxSelectionColor (const Karamba *k, const Input *input) const |
QVariantList | getInputBoxSize (const Karamba *k, const Input *input) const |
int | getInputBoxTextWidth (const Karamba *k, Input *input) const |
QString | getInputBoxValue (const Karamba *k, const Input *input) const |
QObject * | getInputFocus (const Karamba *k) const |
QString | getIp (const Karamba *k, const QString &interface) const |
int | getNumberOfDesktops (const Karamba *k) const |
QObject * | getPlasmaSensor (Karamba *k, const QString &engine, const QString &source=QString()) |
QString | getPrettyThemeName (const Karamba *k) const |
QString | getRichTextFont (const Karamba *k, const RichTextLabel *label) const |
int | getRichTextFontSize (const Karamba *k, const RichTextLabel *label) const |
QVariantList | getRichTextPos (const Karamba *k, const RichTextLabel *label) const |
QString | getRichTextSensor (const Karamba *k, const RichTextLabel *label) const |
QVariantList | getRichTextSize (const Karamba *k, const RichTextLabel *label) const |
QString | getRichTextValue (const Karamba *k, const RichTextLabel *label) const |
QVariantList | getServiceGroups (const QString &path) const |
QVariantList | getStartupInfo (const Karamba *k, const Startup *startup) const |
QVariantList | getStartupList (const Karamba *k) const |
bool | getSystraySize (const Karamba *k) const |
QVariantList | getTaskInfo (const Karamba *k, Task *task) const |
QVariantList | getTaskList (const Karamba *k) const |
QStringList | getTaskNames (const Karamba *k) const |
QString | getTextAlign (const Karamba *k, const TextLabel *text) const |
QVariantList | getTextColor (const Karamba *k, const TextLabel *text) const |
QString | getTextFont (const Karamba *k, const TextLabel *text) const |
int | getTextFontSize (const Karamba *k, const TextLabel *text) const |
QVariantList | getTextPos (const Karamba *k, const TextLabel *text) const |
QString | getTextSensor (const Karamba *k, const TextLabel *text) const |
int | getTextShadow (const Karamba *k, const TextLabel *text) const |
QVariantList | getTextSize (const Karamba *k, const TextLabel *text) const |
int | getTextTextWidth (const Karamba *k, const TextLabel *text) const |
QString | getTextValue (const Karamba *k, const TextLabel *label) const |
QObject * | getThemeBar (const Karamba *k, const QString &meter) const |
QObject * | getThemeGraph (const Karamba *k, const QString &meter) const |
QObject * | getThemeImage (const Karamba *k, const QString &meter) const |
QObject * | getThemeInputBox (const Karamba *k, const QString &meter) const |
QString | getThemePath (const Karamba *k=0) const |
QObject * | getThemeRichText (const Karamba *k, const QString &meter) const |
QObject * | getThemeText (const Karamba *k, const QString &meter) const |
double | getUpdateTime (const Karamba *k) const |
QVariantList | getWidgetPosition (const Karamba *k) const |
bool | hide (Karamba *k) const |
bool | hideBar (const Karamba *k, Bar *bar) const |
bool | hideGraph (const Karamba *k, Graph *graph) const |
bool | hideImage (const Karamba *k, ImageLabel *image) const |
bool | hideInputBox (const Karamba *k, Input *input) const |
bool | hideRichText (const Karamba *k, RichTextLabel *label) const |
bool | hideSystray (const Karamba *k) const |
bool | hideText (const Karamba *k, TextLabel *text) const |
QString | language (const Karamba *k) const |
bool | managementPopup (const Karamba *k) const |
bool | moveBar (const Karamba *k, Bar *bar, int x, int y) const |
bool | moveCanvasWidget (Karamba *k, QObject *canvaswidget, int x, int y, int w, int h) |
bool | moveGraph (const Karamba *k, Graph *graph, int x, int y) const |
bool | moveImage (Karamba *k, ImageLabel *image, int x, int y) const |
bool | moveInputBox (Karamba *k, Input *input, int x, int y) const |
bool | moveRichText (Karamba *k, RichTextLabel *label, int x, int y) const |
bool | moveSystray (const Karamba *k, int x, int y, int w, int h) const |
bool | moveText (Karamba *k, TextLabel *text, int x, int y) const |
bool | moveWidget (Karamba *k, int x, int y) const |
Karamba * | openNamedTheme (const QString &themePath, const QString &themeName, bool startAsSubTheme) const |
Karamba * | openTheme (const QString &themePath) const |
bool | performTaskAction (const Karamba *k, Task *task, int action) const |
bool | popupMenu (const Karamba *k, KMenu *menu, int x, int y) const |
QVariant | readConfigEntry (const Karamba *k, const QString &key) const |
bool | readMenuConfigOption (const Karamba *k, const QString &key) const |
QString | readThemeFile (const Karamba *k, const QString &file) const |
bool | redrawWidget (Karamba *k) const |
bool | redrawWidgetBackground (const Karamba *k) const |
bool | reloadTheme (Karamba *k) const |
bool | removeClickArea (Karamba *k, ClickArea *area) const |
bool | removeImageEffects (const Karamba *k, ImageLabel *image) const |
bool | removeImageTransformations (const Karamba *k, ImageLabel *image) const |
bool | removeMenuItem (Karamba *k, KMenu *menu, QAction *action) const |
bool | resizeBar (const Karamba *k, Bar *bar, int width, int height) const |
bool | resizeGraph (const Karamba *k, Graph *graph, int width, int height) const |
bool | resizeImage (const Karamba *k, ImageLabel *image, int width, int height) const |
bool | resizeImageSmooth (Karamba *k, ImageLabel *image, int width, int height) const |
bool | resizeInputBox (const Karamba *k, Input *input, int width, int height) const |
bool | resizeRichText (const Karamba *k, RichTextLabel *label, int width, int height) const |
bool | resizeText (const Karamba *k, TextLabel *text, int width, int height) const |
bool | resizeWidget (Karamba *k, int width, int height) const |
bool | rotateImage (const Karamba *k, ImageLabel *image, int deg) const |
bool | run (const QString &appName, const QString &command, const QString &icon, const QStringList &arguments) |
bool | setBarImage (const Karamba *k, Bar *bar, const QString &image) const |
bool | setBarMinMax (const Karamba *k, Bar *bar, int min, int max) const |
bool | setBarSensor (Karamba *k, Bar *bar, const QString &sensor) const |
QObject * | setBarValue (const Karamba *k, Bar *bar, int value) const |
bool | setBarVertical (const Karamba *k, Bar *bar, bool vert) const |
bool | setGraphColor (const Karamba *k, Graph *graph, int red, int green, int blue, int alpha=255) const |
bool | setGraphFillColor (const Karamba *k, Graph *graph, int red, int green, int blue, int alpha=255) const |
bool | setGraphMinMax (const Karamba *k, Graph *bar, int min, int max) const |
bool | setGraphPlot (const Karamba *k, Graph *graph, const QString &plot) const |
bool | setGraphScroll (const Karamba *k, Graph *graph, const QString &scroll) const |
bool | setGraphSensor (Karamba *k, Graph *graph, const QString &sensor) const |
bool | setGraphShouldFill (const Karamba *k, Graph *graph, bool shouldFill) const |
QObject * | setGraphValue (const Karamba *k, Graph *graph, int value) const |
bool | setImageElement (Karamba *k, ImageLabel *image, const QString &element) const |
bool | setImageElementAll (Karamba *k, ImageLabel *image) const |
QObject * | setImagePath (const Karamba *k, ImageLabel *image, const QString &path) const |
bool | setImageSensor (Karamba *k, ImageLabel *image, const QString &sensor) const |
bool | setIncomingData (Karamba *k, const QString &prettyThemeName, const QString &data) const |
bool | setInputFocus (const Karamba *k, Input *input) const |
bool | setMenuConfigOption (Karamba *k, const QString &key, bool value) const |
bool | setPixel (Karamba *k, ImageLabel *image, int x, int y, int r, int g, int b, int a=255) |
bool | setRichTextSensor (Karamba *k, RichTextLabel *label, const QString &sensor) const |
bool | setRichTextWidth (const Karamba *k, RichTextLabel *label, int width) const |
bool | setTextAlign (const Karamba *k, TextLabel *text, const QString &alignment) const |
bool | setTextScroll (const Karamba *k, TextLabel *text, const QString &type, int x=0, int y=0, int gap=0, int pause=0) const |
bool | setTextSensor (Karamba *k, TextLabel *text, const QString &sensor) const |
bool | setUpdateTime (Karamba *k, double updateTime) const |
bool | setWantRightButton (Karamba *k, bool enable) const |
bool | show (Karamba *k) const |
bool | showBar (const Karamba *k, Bar *bar) const |
bool | showGraph (const Karamba *k, Graph *graph) const |
bool | showImage (const Karamba *k, ImageLabel *image) const |
bool | showInputBox (const Karamba *k, Input *input) const |
bool | showRichText (const Karamba *k, RichTextLabel *label) const |
bool | showSystray (const Karamba *k) const |
bool | showText (const Karamba *k, TextLabel *text) const |
bool | toggleShowDesktop (const Karamba *k) const |
bool | toggleWidgetRedraw (const Karamba *k, bool enable) const |
bool | translateAll (const Karamba *k, int x, int y) const |
bool | updateSystrayLayout (const Karamba *k) const |
QString | userLanguage (const Karamba *k) const |
QStringList | userLanguages (const Karamba *k) const |
QStringList | version () const |
bool | writeConfigEntry (const Karamba *k, const QString &key, const QVariant &value) const |
Signals | |
void | activeTaskChanged (QObject *, QObject *) |
void | commandFinished (QObject *, int) |
void | commandOutput (QObject *, int, QString) |
void | desktopChanged (QObject *, int) |
void | initWidget (QObject *) |
void | itemDropped (QObject *, QString, int, int) |
void | keyPressed (QObject *, QObject *, QString) |
void | menuItemClicked (QObject *, QObject *, QObject *) |
void | menuOptionChanged (QObject *, QString, bool) |
void | meterClicked (QObject *, QObject *, int) |
void | meterClicked (QObject *, QString, int) |
void | startupAdded (QObject *, QObject *) |
void | startupRemoved (QObject *, QObject *) |
void | taskAdded (QObject *, QObject *) |
void | taskRemoved (QObject *, QObject *) |
void | themeNotify (QObject *, QString, QString) |
void | wallpaperChanged (QObject *, int) |
void | widgetClicked (QObject *, int, int, int) |
void | widgetClosed (QObject *) |
void | widgetMouseMoved (QObject *, int, int, int) |
void | widgetUpdated (QObject *) |
Public Member Functions | |
KarambaInterface (Karamba *k) | |
virtual | ~KarambaInterface () |
void | callActiveTaskChanged (Karamba *k, Task *t) |
void | callCommandFinished (Karamba *k, int pid) |
void | callCommandOutput (Karamba *k, int pid, char *buffer) |
void | callDesktopChanged (Karamba *k, int desktop) |
void | callInitWidget (Karamba *k) |
void | callItemDropped (Karamba *k, const QString &text, int x, int y) |
void | callKeyPressed (Karamba *k, Meter *meter, const QString &key) |
void | callMenuItemClicked (Karamba *k, KMenu *menu, QAction *id) |
void | callMenuOptionChanged (Karamba *k, const QString &key, bool value) |
void | callMeterClicked (Karamba *k, Meter *m, int button) |
void | callMeterClicked (Karamba *k, const QString &str, int button) |
void | callStartupAdded (Karamba *k, Startup *t) |
void | callStartupRemoved (Karamba *k, Startup *t) |
void | callTaskAdded (Karamba *k, Task *t) |
void | callTaskRemoved (Karamba *k, Task *t) |
void | callThemeNotify (Karamba *k, const QString &sender, const QString &data) |
void | callWallpaperChanged (Karamba *k, int desktop) |
void | callWidgetClicked (Karamba *k, int x, int y, int button) |
void | callWidgetClosed (Karamba *k) |
void | callWidgetMouseMoved (Karamba *k, int x, int y, int button) |
void | callWidgetUpdated (Karamba *k) |
bool | initInterpreter () |
void | startInterpreter () |
Detailed Description
Definition at line 42 of file karambainterface.h.
Constructor & Destructor Documentation
KarambaInterface::KarambaInterface | ( | Karamba * | k | ) |
Definition at line 71 of file karambainterface.cpp.
|
virtual |
Definition at line 78 of file karambainterface.cpp.
Member Function Documentation
|
slot |
Misc/acceptDrops.
SYNOPSIS boolean acceptDrops(widget) DESCRIPTION Calling this enables your widget to receive Drop events. In other words, the user will be able to drag icons from his/her desktop and drop them on your widget. The "itemDropped" callback is called as a result with the data about the icon that was dropped on your widget. This allows, for example, icon bars where items are added to the icon bar by Drag and Drop. ARGUMENTS
- reference to widget – karamba RETURN VALUE true if successful
Definition at line 3411 of file karambainterface.cpp.
|
slot |
Image/addImageTooltip.
SYNOPSIS boolean addImageTooltip(widget, image, text) DESCRIPTION This creats a tooltip for image with text.
Note:
- If you move the image, the tooltip does not move! It stays! Do not create a tooltip if the image is off-screen because you will not be able to ever see it. ARGUMENTS
- reference to widget – karamba
- reference to image – image
- string text – tooltip text RETURN VALUE true if successful
Definition at line 1749 of file karambainterface.cpp.
|
slot |
Config/addMenuConfigOption.
SYNOPSIS boolean addMenuConfigOption(widget, key, name) DESCRIPTION SuperKaramba supports a simplistic configuration pop-up menu. This menu appears when you right-click on a widget and choose Configure Theme. Basically, it allows you to have check-able entries in the menu to allow the user to enable or disable features in your theme.
Before you use any configuration menu stuff, you NEED to add a new callback to your script:
def menuOptionChanged(widget, key, value):
This will get called whenever a config menu option is changed. Now you can add items to the config menu:
addMenuConfigOption(widget, String key, String name)
Key is the name of a key value where the value will be saved automatically into the widget's config file. Name is the actual text that will show up in the config menu.
For example, I could allow the user to enable or disable a clock showing up in my theme:
karamba.addMenuConfigOption(widget, "showclock", "Display a clock") ARGUMENTS
- reference to widget – karamba
- string key – key for menu item
- string name – name of the graph to get RETURN VALUE true if successful
Definition at line 2306 of file karambainterface.cpp.
|
slot |
Menu/addMenuItem.
SYNOPSIS reference addMenuItem(widget, menu, text, icon) DESCRIPTION This adds an entry to the given menu with label text and with given icon. Icon can be just an application name in which case the user's current icon set is used, or can be a path to a 16x16 png file.
The function returns the reference to the menu item, which identifies that popup menu item uniquely among popupmenu items application-wide or returns 0 if the given menu doesn't exist. ARGUMENTS
- reference to widget – karamba
- reference to menu – menu
- string text – text for menu item
- string icon – icon name or path RETURN VALUE reference to the menu item
Definition at line 3245 of file karambainterface.cpp.
Menu/addMenuSeparator.
SYNOPSIS reference addMenuSeparator(widget, menu) DESCRIPTION This adds an menu separator to the given menu. ARGUMENTS
- reference to widget – karamba
- reference to menu – menu RETURN VALUE reference to the separator
Definition at line 3272 of file karambainterface.cpp.
|
slot |
Misc/attachClickArea.
SYNOPSIS boolean attachClickArea(widget, meter, lB, mB, rB) DESCRIPTION It is possible to attach a clickarea to a meter (image or text field), which is moved and resized correctly if the meter is moved or resized.
There is also a callback meterClicked(widget, meter, button) which is called whenever a meter is clicked (if something is attached to it). Given an Image or a TextLabel, this call makes it clickable. When a mouse click is detected, the callback meterClicked is called.
lB, mB, and rB are strings that specify what command is executed when this meter is clicked with the left mouse button, middle mouse button, and right mouse button respectively. If given, the appropriate command is executed when the mouse click is received.
The keyword arguments are all optional. If command is an empty string nothing is executed.
For now the command given to RightButton has obviosly no effect (because that brings up the SuperKaramba menu).
ARGUMENTS
- reference to widget – karamba
- reference to meter – meter
- string lB – command to left mouse button
- string mB – command to middle mouse button
- string rB – command to right mouse button
RETURN VALUE true if successful
Definition at line 3456 of file karambainterface.cpp.
Definition at line 447 of file karambainterface.cpp.
void KarambaInterface::callCommandFinished | ( | Karamba * | k, |
int | pid | ||
) |
Definition at line 472 of file karambainterface.cpp.
void KarambaInterface::callCommandOutput | ( | Karamba * | k, |
int | pid, | ||
char * | buffer | ||
) |
Definition at line 477 of file karambainterface.cpp.
void KarambaInterface::callDesktopChanged | ( | Karamba * | k, |
int | desktop | ||
) |
Definition at line 502 of file karambainterface.cpp.
void KarambaInterface::callInitWidget | ( | Karamba * | k | ) |
Definition at line 422 of file karambainterface.cpp.
void KarambaInterface::callItemDropped | ( | Karamba * | k, |
const QString & | text, | ||
int | x, | ||
int | y | ||
) |
Definition at line 482 of file karambainterface.cpp.
Definition at line 512 of file karambainterface.cpp.
void KarambaInterface::callMenuItemClicked | ( | Karamba * | k, |
KMenu * | menu, | ||
QAction * | id | ||
) |
Definition at line 442 of file karambainterface.cpp.
void KarambaInterface::callMenuOptionChanged | ( | Karamba * | k, |
const QString & | key, | ||
bool | value | ||
) |
Definition at line 437 of file karambainterface.cpp.
Definition at line 487 of file karambainterface.cpp.
void KarambaInterface::callMeterClicked | ( | Karamba * | k, |
const QString & | str, | ||
int | button | ||
) |
Definition at line 492 of file karambainterface.cpp.
Definition at line 462 of file karambainterface.cpp.
Definition at line 467 of file karambainterface.cpp.
Definition at line 452 of file karambainterface.cpp.
Definition at line 457 of file karambainterface.cpp.
|
slot |
Misc/callTheme.
SYNOPSIS boolean callTheme(widget, theme, info) DESCRIPTION Calls a theme - identified by the pretty name - and passes it a string to it. This will work, despite superkaramba being multithreaded, because it uses the DBus interface to contact the other theme. If you need to pass complex arguments (dictionaries, lists etc.) then use the python "repr" and "eval" functions to marshall and unmarshall the data structure. ARGUMENTS
- reference to widget – karamba
- string theme – pretty theme name
- string info – a string containing the info to be passed to the theme RETURN VALUE true if successful
Definition at line 3493 of file karambainterface.cpp.
void KarambaInterface::callThemeNotify | ( | Karamba * | k, |
const QString & | sender, | ||
const QString & | data | ||
) |
Definition at line 517 of file karambainterface.cpp.
void KarambaInterface::callWallpaperChanged | ( | Karamba * | k, |
int | desktop | ||
) |
Definition at line 522 of file karambainterface.cpp.
void KarambaInterface::callWidgetClicked | ( | Karamba * | k, |
int | x, | ||
int | y, | ||
int | button | ||
) |
Definition at line 497 of file karambainterface.cpp.
void KarambaInterface::callWidgetClosed | ( | Karamba * | k | ) |
Definition at line 432 of file karambainterface.cpp.
void KarambaInterface::callWidgetMouseMoved | ( | Karamba * | k, |
int | x, | ||
int | y, | ||
int | button | ||
) |
Definition at line 507 of file karambainterface.cpp.
void KarambaInterface::callWidgetUpdated | ( | Karamba * | k | ) |
Definition at line 427 of file karambainterface.cpp.
|
slot |
Image/changeImageAlpha.
SYNOPSIS boolean changeImageAlpha(widget, image, alpha, red=-1, green=-1, blue=-1, millisec=0) DESCRIPTION This will change the image transparency to the given alpha value. The R,G, or B value of -1 indicates that pixels of all intensities in this channel will be assigned the given alpha. If a color (R,G, or B) intensity is specified in the range of 0-255, the alpha value is applied only to the pixels with that intensity. This allows to make, for example, only black (R=0,G=0,B=0) pixels transparent, while keeping all other pixel's alpha unchanged. The value of millisec instructs how long the transparency effect should stay. The value of 0 makes the alpha effect permanent. ARGUMENTS
- reference to widget – karamba
- reference to image – image
- integer alpha – alpha value to use
- integer red – specific intensity of red component (optional) (defaults to "all intensities")
- integer green – specific intensity of green component (optional) (defaults to "all intensities")
- integer blue – blue component of color
- integer millisec – specific intensity of blue component (optional) (defaults to "all intensities") RETURN VALUE true if successful
Definition at line 1873 of file karambainterface.cpp.
|
slot |
Image/changeImageAnimation.
SYNOPSIS boolean changeImageAnimation(widget, image, enable) DESCRIPTION This function can be used to enable or disable animations in an SVG image. The animation is on by default. ARGUMENTS
- reference to widget – karamba
- reference to image – image
- boolean enable – enable or disable animations RETURN VALUE true if successful
Definition at line 2164 of file karambainterface.cpp.
|
slot |
Image/changeImageChannelIntensity.
SYNOPSIS boolean changeImageChannelIntensity(widget, image, ratio, channel, millisec) DESCRIPTION Changes the "intensity" of the image color channel, which is similar to it's brightness. ARGUMENTS
- reference to widget – karamba
- reference to image – image
- decimal ratio – -1.0 to 1.0 (dark to bright)
- string channel – color channel (red|green|blue)
- integer millisec – milliseconds before the image is restored (optional) RETURN VALUE true if successful
Definition at line 1777 of file karambainterface.cpp.
|
slot |
Image/changeImageIntensity.
SYNOPSIS boolean changeImageIntensity(widget, image, ratio, millisec) DESCRIPTION Changes the "intensity" of the image, which is similar to it's brightness. ratio is a floating point number from -1.0 to 1.0 that determines how much to brighten or darken the image. Millisec specifies how long in milliseconds before the image is restored to it's original form. This is useful for "mouse over" type animations. Using 0 for millisec disables this feature and leaves the image permanently affected. ARGUMENTS
- reference to widget – karamba
- reference to image – image
- decimal ratio – -1.0 to 1.0 (dark to bright)
- integer millisec – milliseconds before the image is restored (optional) RETURN VALUE true if successful
Definition at line 1809 of file karambainterface.cpp.
|
slot |
Image/changeImageToGray.
SYNOPSIS boolean changeImageToGray(widget, image, millisec) DESCRIPTION Turns the given image into a grayscale image. Millisec specifies how long in milliseconds before the image is restored to it's original form. This is useful for "mouse over" type animations. Using 0 for millisec disables this feature and leaves the image permanently affected. ARGUMENTS
- reference to widget – karamba
- reference to image – image
- integer millisec – milliseconds before the image is restored (optional) RETURN VALUE true if successful
Definition at line 1837 of file karambainterface.cpp.
|
slot |
InputBox/changeInputBox.
SYNOPSIS reference changeInputBox(widget, inputBox, value) DESCRIPTION This function will change the contents of a input box widget. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box
- string value – new text of the input box RETURN VALUE reference to input box
Definition at line 2584 of file karambainterface.cpp.
|
slot |
InputBox/changeInputBoxBackgroundColor.
SYNOPSIS reference changeInputBoxBackgroundColor(widget, inputBox, r, g, b, a=255) DESCRIPTION This will change the background color of a Input Box widget. InputBox is the reference to the text object to change r, g, b are ints from 0 to 255 that represent red, green, blue and the alpha channel. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box
- integer red – red component of color
- integer green – green component of color
- integer blue – blue component of color
- integer alpha – alpha component of color RETURN VALUE true if successful
Definition at line 2843 of file karambainterface.cpp.
|
slot |
InputBox/changeInputBoxFont.
SYNOPSIS boolean changeInputBoxFont(widget, inputBox, font) DESCRIPTION This will change the font of a Input Box widget. InputBox is the reference to the Input Box object to change. Font is a string with the name of the font to use. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – inputBox
- string font – font name RETURN VALUE true if successful
Definition at line 2677 of file karambainterface.cpp.
|
slot |
InputBox/changeInputBoxFontColor.
SYNOPSIS boolean changeInputBoxFontColor(widget, inputBox, r, g, b, a=255) DESCRIPTION This will change the color of a text of a Input Box widget. InputBox is the reference to the text object to change r, g, b, a are ints from 0 to 255 that represent red, green, blue and the alpha channel. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box
- integer red – red component of color
- integer green – green component of color
- integer blue – blue component of color
- integer alpha – alpha component of color (optional) RETURN VALUE true if successful
Definition at line 2727 of file karambainterface.cpp.
|
slot |
InputBox/changeInputBoxFontSize.
SYNOPSIS boolean changeInputBoxFontSize(widget, text, size) DESCRIPTION This will change the font size of a Input Box widget. InputBox is the reference to the text object to change. Size is the new font point size. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box
- integer size – new font size for text RETURN VALUE true if successful
Definition at line 3017 of file karambainterface.cpp.
|
slot |
InputBox/changeInputBoxFrameColor.
SYNOPSIS boolean changeInputBoxFrameColor(widget, inputBox, r, g, b, a=255) DESCRIPTION This will change the frame color of a Input Box widget. InputBox is the reference to the text object to change r, g, b are ints from 0 to 255 that represent red, green, blue and the alpha channel. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box
- integer red – red component of color
- integer green – green component of color
- integer blue – blue component of color
- integer alpha – alpha component of color (optional) RETURN VALUE true if successful
Definition at line 2903 of file karambainterface.cpp.
|
slot |
InputBox/changeInputBoxSelectedTextColor.
SYNOPSIS boolean changeInputBoxSelectedTextColor(widget, inputBox, r, g, b, a=255) DESCRIPTION This will change the selected text color of a Input Box widget. InputBox is the reference to the text object to change r, g, b are ints from 0 to 255 that represent red, green, blue and alpha channel. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box
- integer red – red component of color
- integer green – green component of color
- integer blue – blue component of color
- integer alpha – alpha component of color (optional) RETURN VALUE true if successful
Definition at line 2960 of file karambainterface.cpp.
|
slot |
InputBox/changeInputBoxSelection.
SYNOPSIS boolean changeInputBoxSelection(widget, inputBox, start, length) DESCRIPTION This will set the selection of the input box. The range can be set by the start cursor position and the length of the selection. Both arguments are counted in characters and not pixel. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – inputBox
- integer start – start of the selection
- integer length – length of the selection RETURN VALUE true if successful
Definition at line 3149 of file karambainterface.cpp.
|
slot |
InputBox/changeInputBoxSelectionColor.
SYNOPSIS boolean changeInputBoxSelectionColor(widget, inputBox, r, g, b, a=255) DESCRIPTION This will change the color of the selection of a Input Box widget. InputBox is the reference to the text object to change r, g, b, a are ints from 0 to 255 that represent red, green, blue and the alpha channel. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box
- integer red – red component of color
- integer green – green component of color
- integer blue – blue component of color
- integer alpha – alpha component of color (optional) RETURN VALUE true if successful
Definition at line 2785 of file karambainterface.cpp.
|
slot |
Misc/changeInterval.
SYNOPSIS boolean changeInterval(widget, interval) DESCRIPTION This function changes your widget's refresh rate (ms) ARGUMENTS
- reference to widget – karamba
- integer interval – interval, in ms RETURN VALUE true if successful
Definition at line 3514 of file karambainterface.cpp.
|
slot |
RichText/changeRichText.
SYNOPSIS reference changeRichText(widget, richtext, value) DESCRIPTION This will change the contents of a rich text meter. richText is the reference to the text object to change that you saved from the createRichText() call. text is a string containing the new value for the rich text object.
The differance between Rich Text and a regular text field is that rich text fields can display HTML code embedded in your text.
In a ... tag command is executed if the link is click with the left mouse button.
Except if command starts with an '#' (ie: href="#value" ) the callback meterClicked is called with value (without the #) as the meter argument.
Also inline images work. Unfortunately currently only when using absolute paths. ARGUMENTS
- reference to widget – karamba
- reference to richtext – richtext
- string value – new text RETURN VALUE true if successful
Definition at line 4620 of file karambainterface.cpp.
|
slot |
RichText/changeRichTextFont.
SYNOPSIS long changeRichTextFont(widget, richtext, font) DESCRIPTION This will change the font of a richtext meter. ARGUMENTS
- long widget – karamba
- long richtext – richtext
- string font – name of the new font RETURN VALUE 1 if successful
Definition at line 4715 of file karambainterface.cpp.
|
slot |
RichText/changeRichTextSize.
SYNOPSIS boolean changeRichTextSize(widget, richtext, size) DESCRIPTION Sets the font size of a richtext meter. ARGUMENTS
- reference to widget – karamba
- reference to richtext – richtext
- long size – new font point size RETURN VALUE true if successful
Definition at line 4760 of file karambainterface.cpp.
|
slot |
Text/changeText.
SYNOPSIS reference changeText(widget, text, value) DESCRIPTION This will change the contents of a text widget. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter
- long value – new text to display RETURN VALUE true if successful
Definition at line 5455 of file karambainterface.cpp.
|
slot |
Text/changeTextColor.
SYNOPSIS boolean changeTextColor(widget, text, r, g, b, a=255) DESCRIPTION This will change the color of a text widget (only ones you created through python currently). textToChange is the reference to the text object to change that you saved from the createText() call. r, g, b are ints from 0 to 255 that represent red, green, blue and the alpha channel. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter
- integer red – red component of color
- integer green – green component of color
- integer blue – blue component of color
- integer alpha – alpha component of color (optional) RETURN VALUE true if successful
Definition at line 5592 of file karambainterface.cpp.
|
slot |
Text/changeTextFont.
SYNOPSIS boolean changeTextFont(widget, text, font) DESCRIPTION This will change the font of a text widget (only ones you created through python currently). Text is the reference to the text object to change that you saved from the createText() call. Font is a string with the name of the font to use. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter
- string font – font name RETURN VALUE true if successful
Definition at line 5541 of file karambainterface.cpp.
|
slot |
Text/changeTextShadow.
SYNOPSIS boolean changeTextShadow(widget, text, shadow) DESCRIPTION This will change the shadow size of a text widget (only ones you created through python currently). textToChange is the reference to the text object to change that you saved from the createText() call. size is the offset of the shadow in pixels. 1 or 2 is a good value in most cases. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter
- integer shadow – shadow offset RETURN VALUE true if successful
Definition at line 5494 of file karambainterface.cpp.
Text/changeTextSize.
SYNOPSIS boolean changeTextSize(widget, text, size) DESCRIPTION This will change the font size of a text widget (only ones you created through python currently). text is the reference to the text object to change that you saved from the createText() call. size is the new font point size. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter
- integer size – new size for text RETURN VALUE true if successful
Definition at line 5631 of file karambainterface.cpp.
InputBox/clearInputBoxSelection.
SYNOPSIS boolean clearInputBoxSelection(widget, inputBox) DESCRIPTION This will clear the selection of the input box. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – inputBox RETURN VALUE true if successful
Definition at line 3201 of file karambainterface.cpp.
InputBox/clearInputFocus.
SYNOPSIS boolean clearInputFocus(widget, inputBox) DESCRIPTION Releases the input focus from the Input Box. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – pointer to Input Box RETURN VALUE true if successful
Definition at line 3082 of file karambainterface.cpp.
|
signal |
|
signal |
|
slot |
Image/createBackgroundImage.
SYNOPSIS reference createBackgroundImage(widget, x, y, w, h, image) DESCRIPTION This creates an background image on your widget at x, y. The filename should be given as the path parameter. In theory the image could be local or could be a url. It works just like adding an image in your theme file. You will need to save the return value to be able to call other functions on your image, such as moveImage() ARGUMENTS
- reference to widget – karamba
- integer x – x coordinate
- integer y – y coordinate
- string image – path to the image for the background RETURN VALUE reference to new image meter
Definition at line 1902 of file karambainterface.cpp.
|
slot |
Bar/createBar.
SYNOPSIS reference createBar(widget, x, y, w, h, image) DESCRIPTION This creates a bar at x, y with width and height w, h. ARGUMENTS
- reference to widget – karamba
- integer x – x coordinate
- integer y – y coordinate
- integer w – width
- integer h – height
- string image – Path to image RETURN VALUE Reference to new bar meter
Definition at line 546 of file karambainterface.cpp.
CanvasWidget/createCanvasWidget.
SYNOPSIS canvaswidget createCanvasWidget(widget, widget) DESCRIPTION Adds a QWidget to the scene and returns a canvaswidget object. ARGUMENTS
- reference to widget – karamba
- reference to widget – the QWidget which should be added to the QGraphicsScene. RETURN VALUE the reference to an instance of a QGraphicsProxyWidget canvaswidget object.
Definition at line 5943 of file karambainterface.cpp.
|
slot |
Misc/createClickArea.
SYNOPSIS reference createClickArea(widget, x, y, w, h, cmdToRun) DESCRIPTION This creates a clickable area at x, y with width and height w, h. When this area is clicked, cmd_to_run will be executed. The mouse will change over this area. ARGUMENTS
- reference to widget – karamba
- integer x – x coordinate
- integer y – y coordinate
- integer w – width
- integer h – height
- string cmdToRun – command to be run RETURN VALUE reference to the new click area
Definition at line 3561 of file karambainterface.cpp.
Image/createEmptyImage.
SYNOPSIS reference createEmptyImage(widget, x, y, w, h) DESCRIPTION This creates an empty image on your widget at x, y. The empy image allows you to draw the contents with the setPixel() function without loading an image first. ARGUMENTS
- reference to widget – karamba
- integer x – x coordinate
- integer y – y coordinate
- integer w – width of the image
- integer h – height of the image RETURN VALUE reference to new image meter
Definition at line 1477 of file karambainterface.cpp.
|
slot |
Graph/createGraph.
SYNOPSIS reference createGraph(widget, x, y, width, height, points) DESCRIPTION This creates a graph at x, y with width and height. ARGUMENTS
- reference to widget – karamba
- integer x – x coordinate
- integer y – y coordinate
- integer w – width
- integer h – height
- integer points – Number of points in graph RETURN VALUE reference to new graph meter
Definition at line 927 of file karambainterface.cpp.
|
slot |
Image/createImage.
SYNOPSIS reference createImage(widget, x, y, image) DESCRIPTION This creates an image on your widget at x, y. The filename should be given as the path parameter. In theory the image could be local or could be a url. It works just like adding an image in your theme file. You will need to save the return value to be able to call other functions on your image, such as moveImage() ARGUMENTS
- reference to widget – karamba
- integer x – x coordinate
- integer y – y coordinate
- string image – image for the imagelabel RETURN VALUE reference to new image meter
Definition at line 1444 of file karambainterface.cpp.
|
slot |
InputBox/createInputBox.
SYNOPSIS reference createInputBox(widget, x, y, w, h, text) DESCRIPTION This creates a new Input Box at x, y with width and height w, h. You need to save the return value of this function to call other functions on your Input Box field, such as changeInputBox(). The karamba widget is automatically set active, to allow user interactions. ARGUMENTS
- reference to widget – karamba
- integer x – x coordinate
- integer y – y coordinate
- integer w – width
- integer h – height
- string text – text for the Input Box RETURN VALUE reference to new Input Box
Definition at line 2451 of file karambainterface.cpp.
Menu/createMenu.
SYNOPSIS reference createMenu(widget) DESCRIPTION This creates an empty popup menu and returns a reference to the menu. ARGUMENTS
- reference to widget – karamba RETURN VALUE reference to menu
Definition at line 3297 of file karambainterface.cpp.
|
slot |
RichText/createRichText.
SYNOPSIS reference createRichText(widget, text, underlineLinks) DESCRIPTION This creates creates a new rich text meter. underlineLinks is a boolean that determines if html links will be automatically underlined so that the user knows that the links can be clicked on. You need to save the return value of this function to call other functions on your rich text field, such as changeRichText().
The differance between Rich Text and a regular text field is that rich text fields can display HTML code embedded in your text.
In a ... tag command is executed if the link is click with the left mouse button.
Except if command starts with an '#' (ie: href="#value" ) the callback meterClicked is called with value (without the #) as the meter argument.
Also inline images work. Unfortunately currently only when using absolute paths. ARGUMENTS
- reference to widget – karamba
- string text – text for richtext
- boolean underlineLinks – should the links be underlined RETURN VALUE reference to new richtext meter
Definition at line 4450 of file karambainterface.cpp.
|
slot |
Misc/createServiceClickArea.
SYNOPSIS reference createServiceClickArea(widget, x, y, w, h, name_of_command, cmd_to_run, icon_to_display) DESCRIPTION This creates a clickable area at x, y with width and height w, h. When this area is clicked, cmd_to_run will be executed. The mouse will change to the clickable icon when over this area. For more information on the difference between createClickArea and createServiceClickArea, see the KDE documentation about KService, and the difference between KRun::run and KRun::runCommand. ARGUMENTS
- reference to widget – karamba
- integer x – x coordinate
- integer y – y coordinate
- integer w – width
- integer h – height
- string name_of_command – name to be displayed
- string cmd_to_run – command to be run
- string icon_to_display – name of icon to be displayed RETURN VALUE reference to the new click area
Definition at line 3622 of file karambainterface.cpp.
|
slot |
Definition at line 4831 of file karambainterface.cpp.
Image/createTaskIcon.
SYNOPSIS reference createTaskIcon(widget, x, y, ctask) DESCRIPTION This creates a task image at x, y. ARGUMENTS
- reference to widget – karamba
- integer x – x coordinate
- integer y – y coordinate
- integer task – task RETURN VALUE reference to new image meter
Definition at line 1935 of file karambainterface.cpp.
|
slot |
Text/createText.
SYNOPSIS reference createText(widget, x, y, w, h, text) DESCRIPTION This creates a new text at x, y with width and height w, h. You need to save the return value of this function to call other functions on your text field, such as changeText() ARGUMENTS
- reference to widget – karamba
- integer x – x coordinate
- integer y – y coordinate
- integer w – width
- integer h – height
- string text – text for the textlabel RETURN VALUE Pointer to new text meter
Definition at line 5235 of file karambainterface.cpp.
|
slot |
Widget/createWidgetMask.
SYNOPSIS long createWidgetMask(widget, mask) DESCRIPTION This function doesn't work currently due to a bug in KDE. Please use MASK= in your .theme file for the time being. WARNING This function does nothing in SuperKaramba 0.50 and later ARGUMENTS
- reference to widget – karamba
- string mask – The path to the widget mask file. RETURN VALUE true if successful
Definition at line 5761 of file karambainterface.cpp.
Bar/deleteBar.
SYNOPSIS boolean deleteBar(widget, bar) DESCRIPTION This deletes the bar. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar RETURN VALUE true if successful
Definition at line 573 of file karambainterface.cpp.
Graph/deleteGraph.
SYNOPSIS boolean deleteGraph(widget, graph) DESCRIPTION This deletes graph. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE true if successful
Definition at line 951 of file karambainterface.cpp.
|
slot |
Image/deleteImage.
SYNOPSIS boolean deleteImage(widget, image) DESCRIPTION This removes the image from the widhet. Please do not call functions on "image" after calling deleteImage, as it does not exist anymore and that could cause crashes in some cases. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE true if successful
Definition at line 1504 of file karambainterface.cpp.
InputBox/deleteInputBox.
SYNOPSIS boolean deleteInputBox(widget, inputBox) DESCRIPTION This removes the Input Box object from the widget. Please do not call functions of the Input Box after calling deleteInputBox, as it does not exist anymore and that could cause crashes in some cases. The karamba widget is automatically set passive, when no more Input Boxes are on the karamba widget. ARGUMENTS
- reference to widget – karamba
- reference widget – InputBox RETURN VALUE true if successful
Definition at line 2485 of file karambainterface.cpp.
|
slot |
Menu/deleteMenu.
SYNOPSIS boolean deleteMenu(widget, menu) DESCRIPTION This deletes the referenced menu if that menu exists. ARGUMENTS
- reference to widget – karamba
- reference to menu – pointer to menu RETURN VALUE true if menu existed and was deleted, returns false otherwise.
Definition at line 3318 of file karambainterface.cpp.
|
slot |
RichText/deleteRichText.
SYNOPSIS boolean deleteRichText(widget, richtext) DESCRIPTION This removes the rich text from the widget. Please do not call functions on "text" after calling deleteRichText, as it does not exist anymore and that could cause crashes in some cases. ARGUMENTS
- reference to widget – karamba
- reference to widget – richtext RETURN VALUE true if successful
Definition at line 4479 of file karambainterface.cpp.
Text/deleteText.
SYNOPSIS boolean deleteText(widget, text) DESCRIPTION This removes a text object from the widget. Please do not call functions on "text" after calling deleteText, as it does not exist anymore and that could cause crashes in some cases. ARGUMENTS
- reference to widget – karamba
- reference to widget – text meter RETURN VALUE true if successful
Definition at line 5265 of file karambainterface.cpp.
|
signal |
|
slot |
Misc/desktopSize.
SYNOPSIS array desktopSize() DESCRIPTION Returns the screen size of the display. RETURN VALUE array with width and height of the screen
Definition at line 4370 of file karambainterface.cpp.
|
slot |
Misc/execute.
SYNOPSIS integer execute(command) DESCRIPTION This command simply executes a program or command on the system. This is just for convience (IE you could accomplish this directly through python, but sometimes threading problems crop up that way). The only option is a string containing the command to execute. ARGUMENTS
- string command – command to execute RETURN VALUE process id of the executed command
Definition at line 3539 of file karambainterface.cpp.
|
slot |
Misc/executeInteractive.
SYNOPSIS integer executeInteractive(widget, command) DESCRIPTION This command executes a program or command on the system. Additionally it allows you to get any text that the program outputs. Futhermore, it won't freeze up your widget while the command executes.
To use it, call executeInteractive with the reference to your widget and a list of command options. The array is simply a list that contains the command as the first entry, and each option as a separate list entry. Output from the command is returned via the commandOutput callback.
The command returns the process number of the command. This is useful if you want to run more than one program at a time. The number will allow you to figure out which program is outputting in the commandOutput callback.
Example: Run the command "ls -la *.zip"
myCommand = ["ls", "-la", "*.zip"] karamba.executeInteractive(widget, myCommand) ARGUMENTS
- reference to widget – karamba
- list command – command to execute RETURN VALUE process id of the executed command
Definition at line 3666 of file karambainterface.cpp.
Bar/getBarImage.
SYNOPSIS string getBarImage(widget, bar) DESCRIPTION Get the path to the bar image. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar RETURN VALUE path to bar image
Definition at line 899 of file karambainterface.cpp.
Bar/getBarMinMax.
SYNOPSIS array getBarMinMax(widget, bar) DESCRIPTION Returns possible min and max values of the bar. ARGUMENTS
- reference to widget – karamba
- reference bar – bar RETURN VALUE array with max & min values
Definition at line 613 of file karambainterface.cpp.
Bar/getBarPos.
SYNOPSIS array getBarPos(widget, bar) DESCRIPTION Given a reference to a bar object, this will return a array containing the x and y coordinate of a bar object. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar RETURN VALUE array with x and y coordinates
Definition at line 650 of file karambainterface.cpp.
Bar/getBarSensor.
SYNOPSIS string getBarSensor(widget, bar) DESCRIPTION Gets the current sensor string. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar RETURN VALUE sensor string
Definition at line 685 of file karambainterface.cpp.
Bar/getBarSize.
SYNOPSIS array getBarSize(widget, bar) DESCRIPTION Given a reference to a bar object, this will return a array containing the height and width of the bar object. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar RETURN VALUE array with width and height
Definition at line 722 of file karambainterface.cpp.
Bar/getBarValue.
SYNOPSIS integer getBarValue(widget, bar) DESCRIPTION Returns current bar value. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar RETURN VALUE value
Definition at line 757 of file karambainterface.cpp.
Bar/getBarVertical.
SYNOPSIS boolean getBarVertical(widget, bar) DESCRIPTION Check if bar is a vertical bar. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar RETURN VALUE true if vertical
Definition at line 856 of file karambainterface.cpp.
|
slot |
Definition at line 4852 of file karambainterface.cpp.
|
slot |
Misc/getExecutingApplication.
SYNOPSIS strint getExecutingApplication() DESCRIPTION Returns the name of the application that runs the theme. It will return "superkaramba" if it runs in SuperKaramba or "plasma" if the theme runs in the Plasma desktop. RETURN VALUE string with the application name
Definition at line 4412 of file karambainterface.cpp.
Graph/getGraphColor.
SYNOPSIS array getGraphColor(widget, graph) DESCRIPTION Gets the current graph color components. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE (red, green, blue, alpha)
Definition at line 1233 of file karambainterface.cpp.
|
slot |
Graph/getGraphFillColor.
SYNOPSIS array getGraphFillColor(widget, graph) DESCRIPTION Gets the current graph fill color components. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE (red, green, blue, alpha)
Definition at line 1277 of file karambainterface.cpp.
Graph/getGraphMinMax.
SYNOPSIS array getGraphMinMax(widget, graph) DESCRIPTION Returns current graph minimal and maximal values. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE max and min values
Definition at line 991 of file karambainterface.cpp.
Graph/getGraphPlot.
SYNOPSIS QString getGraphPlot(widget, graph) DESCRIPTION get string description of the plot direction ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE QString "up" or "down"
Definition at line 1350 of file karambainterface.cpp.
Graph/getGraphPos.
SYNOPSIS array getGraphPos(widget, graph) DESCRIPTION Given a reference to a graph object, this will return an array containing the x and y coordinates of the graph object. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE x and y coordinates
Definition at line 1028 of file karambainterface.cpp.
Graph/getGraphScroll.
SYNOPSIS QString getGraphScroll(widget, graph) DESCRIPTION get string description of the scroll direction ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE QString "left" or "right"
Definition at line 1306 of file karambainterface.cpp.
Graph/getGraphSensor.
SYNOPSIS string getGraphSensor(widget, graph) DESCRIPTION Gets the current sensor string of the graph. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE sensor string
Definition at line 1063 of file karambainterface.cpp.
Graph/getGraphShouldFill.
SYNOPSIS QString getGraphShouldFill(widget, graph) DESCRIPTION get bool describing whether or not the graph will draw itself filled ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE bool true if graph should draw itself filled
Definition at line 1394 of file karambainterface.cpp.
Graph/getGraphSize.
SYNOPSIS array getGraphSize(widget, graph) DESCRIPTION Given a reference to a graph object, this will return an array containing the height and width of a graph object. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE width and height of the graph
Definition at line 1100 of file karambainterface.cpp.
Graph/getGraphValue.
SYNOPSIS integer getGraphValue(widget, graph) DESCRIPTION Returns current graph value. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE value
Definition at line 1135 of file karambainterface.cpp.
|
slot |
Misc/getIconByName.
SYNOPSIS strint getIconByName(name, size) DESCRIPTION Returns the absolute path to the icon. This function respects the current used icon set. ARGUMENTS
- string name – name of the icon
- integer size – Size of the icon (16, 22, 32, 48, 64, 128 available) RETURN VALUE string with the absolute path to the icon.
Definition at line 4395 of file karambainterface.cpp.
|
slot |
Image/getImageAnimation.
SYNOPSIS boolean getImageAnimation(widget, image) DESCRIPTION This function returns if the animation of an SVG image is currently activated. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE true if animation is activated or false if it is deactivated or not an SVG image.
Definition at line 2187 of file karambainterface.cpp.
|
slot |
Image/getImageElement.
SYNOPSIS string getImageElement(widget, image) DESCRIPTION This function returns the ID of the currently drawn element of an SVG image. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE ID of the element or empty string if the image is not an SVG image or the complete SVG image is drawn.
Definition at line 2257 of file karambainterface.cpp.
|
slot |
Image/getImageHeight.
SYNOPSIS integer getImageHeight(widget, image) DESCRIPTION This returns the height of an image. This is useful if you have rotated an image and its size changed, so you do not know how big it is anymore. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE height of the image
Definition at line 1980 of file karambainterface.cpp.
|
slot |
Image/getImagePath.
SYNOPSIS string getImagePath(widget, image) DESCRIPTION Returns the current image path. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE path
Definition at line 1667 of file karambainterface.cpp.
|
slot |
Image/getImagePos.
SYNOPSIS array getImagePos(widget, image) DESCRIPTION Given a reference to a image object, this will return a tuple containing the x and y coordinate of a image object. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE x and y coordinates of the image
Definition at line 1548 of file karambainterface.cpp.
|
slot |
Image/getImageSensor.
SYNOPSIS string getImageSensor(widget, image) DESCRIPTION Gets the current sensor string. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE sensor string
Definition at line 1584 of file karambainterface.cpp.
|
slot |
Image/getImageSize.
SYNOPSIS array getImageSize(widget, image) DESCRIPTION Given a reference to a image object, this will return an array containing the height and width of a image object. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE height and width of the image
Definition at line 1629 of file karambainterface.cpp.
|
slot |
Image/getImageWidth.
SYNOPSIS integer getImageWidth(widget, image) DESCRIPTION This returns the width of an image. This is useful if you have rotated an image and its size changed, so you do not know how big it is anymore. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE width of the image
Definition at line 2002 of file karambainterface.cpp.
|
slot |
Misc/getIncomingData.
SYNOPSIS string getIncomingData(widget) DESCRIPTION Obtains the last data received by any other theme that set the "incoming data" of this theme. This isn't particularly sophisticated and could benefit from the data being placed in an FIFO queue instead. ARGUMENTS
- reference to widget – karamba RETURN VALUE string containing the last information received from setIncomingData
Definition at line 4168 of file karambainterface.cpp.
|
slot |
InputBox/getInputBoxBackgroundColor.
SYNOPSIS array getInputBoxBackgroundColor(widget, inputBox) DESCRIPTION Gets the current background color of a Input Box ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box RETURN VALUE (red, green, blue, alpha)
Definition at line 2867 of file karambainterface.cpp.
InputBox/getInputBoxFont.
SYNOPSIS string getInputBoxFont(widget, inputBox) DESCRIPTION Gets the current Input Box font name ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box RETURN VALUE font name
Definition at line 2700 of file karambainterface.cpp.
|
slot |
InputBox/getInputBoxFontColor.
SYNOPSIS array getInputBoxFontColor(widget, inputBox) DESCRIPTION Gets the current text color of a Input Box ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box RETURN VALUE (red, green, blue, alpha)
Definition at line 2750 of file karambainterface.cpp.
InputBox/getInputBoxFontSize.
SYNOPSIS integer getInputBoxFontSize(widget, inputBox) DESCRIPTION Gets the current text font size. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box RETURN VALUE text font size
Definition at line 3039 of file karambainterface.cpp.
|
slot |
InputBox/getInputBoxFrameColor.
SYNOPSIS array getInputBoxFrameColor(widget, inputBox) DESCRIPTION Gets the current frame color of a Input Box ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box RETURN VALUE (red, green, blue, alpha)
Definition at line 2925 of file karambainterface.cpp.
InputBox/getInputBoxPos.
SYNOPSIS array getInputBoxPos(widget, inputBox) DESCRIPTION Given a reference to a Input Box object, this will return an array containing the x and y coordinate of an Input Box. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box RETURN VALUE x and y coordinates of the inputBox
Definition at line 2529 of file karambainterface.cpp.
|
slot |
InputBox/getInputBoxSelectedTextColor.
SYNOPSIS array getInputBoxSelectedTextColor(widget, inputBox) DESCRIPTION Gets the current selected text color of a Input Box. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – pointer to Input Box RETURN VALUE (red, green, blue, alpha)
Definition at line 2984 of file karambainterface.cpp.
InputBox/getInputBoxSelection.
SYNOPSIS array getInputBoxSelection(widget, inputBox) DESCRIPTION This will return the selection of the input box. If no selection was made in the input box (-1, 0) will be returned for the start and length of the selection. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – inputBox RETURN VALUE array with the selection start and length in characters
Definition at line 3174 of file karambainterface.cpp.
|
slot |
InputBox/getInputBoxSelectionColor.
SYNOPSIS array getInputBoxSelectionColor(widget, inputBox) DESCRIPTION Gets the current selection color of a Input Box ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box RETURN VALUE (red, green, blue, alpha)
Definition at line 2808 of file karambainterface.cpp.
|
slot |
InputBox/getInputBoxSize.
SYNOPSIS array getInputBoxSize(widget, inputBox) DESCRIPTION Given a reference to a Input Box object, this will return an array containing the height and width of a Input Box object. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box RETURN VALUE width and hight of the input box
Definition at line 2566 of file karambainterface.cpp.
InputBox/getInputBoxTextWidth.
SYNOPSIS integer getInputBoxTextWidth(widget, input) DESCRIPTION Gets the width of the complete text of the Input Box. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – pointer to InputBox RETURN VALUE the width or -1
Definition at line 3124 of file karambainterface.cpp.
InputBox/getInputBoxValue.
SYNOPSIS string getInputBoxValue(widget, inputBox) DESCRIPTION Returns current Input Box text. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box RETURN VALUE input box text
Definition at line 2601 of file karambainterface.cpp.
InputBox/getInputFocus.
SYNOPSIS boolean getInputFocus(widget) DESCRIPTION Gets the Input Box that is currently focused. ARGUMENTS
- reference to widget – karamba RETURN VALUE the input box or 0
Definition at line 3102 of file karambainterface.cpp.
|
slot |
Misc/getIp.
SYNOPSIS string getIp(widget, interface_name) DESCRIPTION Gets the current IP address of the interface_name interface. ARGUMENTS
- reference to widget – karamba
- string interface_name – name of the interface RETURN VALUE ip address as string
Definition at line 3703 of file karambainterface.cpp.
|
slot |
Misc/getNumberOfDesktop.
SYNOPSIS integer getNumberOfDesktop(widget) DESCRIPTION Returns number of desktops ARGUMENTS
- reference to widget – karamba RETURN VALUE number of desktops
Definition at line 3738 of file karambainterface.cpp.
|
slot |
Sensor/getPlasmaSensor.
SYNOPSIS dataengine getPlasmaSensor(widget, engine, source) DESCRIPTION Returns a sensor for Plasma::DataEngine objects. ARGUMENTS
- reference to widget – karamba
- string engine – The name of the dataengine. This could be for example "time" for the Plasma TimeEngine.
- string source – The source. E.g. "Local" for the Plasma TimeEngine. RETURN VALUE the reference to an instance of the SuperKaramba PlasmaSensor class. See also http://websvn.kde.org/trunk/kdeutils/superkaramba/src/sensors/plasmaengine.h
Definition at line 5923 of file karambainterface.cpp.
|
slot |
Misc/getPrettyThemeName.
SYNOPSIS string getPrettyName(theme) DESCRIPTION When a theme is created (with openNamedTheme), there is an option to give the theme an alternative name. This is useful if you open several widgets from the same theme: you need to give them unique names in order to contact them (for example, with callTheme or with setIncomingData) ARGUMENTS
- string theme – path to new theme RETURN VALUE the pretty name of the theme
Definition at line 3762 of file karambainterface.cpp.
|
slot |
RichText/getRichTextFont.
SYNOPSIS string getRichTextFont(widget, richtext) DESCRIPTION Gets the font name of a richtext meter. ARGUMENTS
- reference to widget – karamba
- reference to richtext – richtext RETURN VALUE font name
Definition at line 4738 of file karambainterface.cpp.
|
slot |
RichText/getRichTextFontSize.
SYNOPSIS integer getRichTextFontSize(widget, richtext) DESCRIPTION Gets the font size of the richtext meter. ARGUMENTS
- reference to widget – karamba
- reference to richtext – richtext RETURN VALUE font point size
Definition at line 4783 of file karambainterface.cpp.
|
slot |
RichText/getRichTextPos.
SYNOPSIS array getRichTextPos(widget, richtext) DESCRIPTION Given a reference to a richtext object, this will return an array containing the x and y coordinate of a richtext object. ARGUMENTS
- reference to widget – karamba
- reference to richtext – richtext RETURN VALUE x and y coordinates
Definition at line 4521 of file karambainterface.cpp.
|
slot |
RichText/getRichTextSensor.
SYNOPSIS string getRichTextSensor(widget, richtext) DESCRIPTION Gets the current sensor string ARGUMENTS
- reference to widget – karamba
- reference to richtext – richtext RETURN VALUE current sensor string
Definition at line 4823 of file karambainterface.cpp.
|
slot |
RichText/getRichTextSize.
SYNOPSIS array getRichTextSize(widget, richtext) DESCRIPTION Given a reference to a richtext object, this will return a tuple containing the height and width of a richtext object. ARGUMENTS
- long widget – karamba
- long richtext – richtext RETURN VALUE width and height of the richtext
Definition at line 4587 of file karambainterface.cpp.
|
slot |
RichText/getRichTextValue.
SYNOPSIS string getRichTextValue(widget, richtext) DESCRIPTION Returns the current richtext value. ARGUMENTS
- reference to widget – karamba
- reference to richtext – richtext RETURN VALUE text that is displayed in the rich text
Definition at line 4638 of file karambainterface.cpp.
|
slot |
Misc/getServiceGroups.
SYNOPSIS list getServiceGroups(path) DESCRIPTION This function returns a list of services and service groups that are in the user's KDE Menu. It is not a recursive function, so if there are submenus (service groups) in the returned results, you must call getServiceGroups with the path of the submenu in order to obtain the information in that submenu. The return result is complex: it's a list of tuples. The tuple contains two elements - a 1 if the second element is a service, and a 0 if it's a service group. The second element is a dictionary, with keys (if they exist) of caption, comment, icon, and relpath if it's a service group, and keys (if they exist) of exec, menuid, name, path, icon, library, comment, type and genericname. To fully understand the return results of this function, it is thoroughly recommended // that you look up the KDE documentation on KServiceGroup and KService. ARGUMENTS
- reference to widget – karamba
- string path – path to the Service Group you wish to retrieve RETURN VALUE List of Dictionaries of services and service groups
Definition at line 3798 of file karambainterface.cpp.
|
slot |
Task/getStartupInfo.
SYNOPSIS list getStartupInfo(widget, task) DESCRIPTION This returns all of the info about a certain starting task in the form of a list. Widget is a reference to the current widget. task is a reference to the window you want info about which you obtain by calling getStartupList(). ARGUMENTS
- reference to widget – karamba
- reference to task – task RETURN VALUE Here is the format of the returned list by index value:
- 0 = Task name (The full name of the window)
- 1 = Icon name
- 2 = Executable name
- 3 = A reference back to the task you got info on
Definition at line 4974 of file karambainterface.cpp.
|
slot |
Task/getStartupList.
SYNOPSIS list getTaskList(widget) DESCRIPTION This returns a list with references to all the current windows that are in the process of loading on this system. You can then call getStartupInfo() on any of the entries in the list. ARGUMENTS
- reference to widget – karamba RETURN VALUE list with references to startups
Definition at line 5004 of file karambainterface.cpp.
|
slot |
Definition at line 4937 of file karambainterface.cpp.
Task/getTaskInfo.
SYNOPSIS list getTaskInfo(widget, task) DESCRIPTION This returns all of the info about a certain task in the form of a list. widget is a reference to the current widget. Task is a reference to the window you want info about which you obtain by calling getTaskList(). ARGUMENTS
- reference to widget – karamba
- reference to task – task RETURN VALUE Here is the format of the returned list by index value:
- 0 = Task name (The full name of the window)
- 1 = Icon name
- 2 = Class name - This is for grouping tasks. All tasks with the same name can be grouped together because they are instances of the same program.
- 3 = Desktop number - The desktop number this window is on
- 4 = Is this window maximized? false = no, true = yes
- 5 = Is this window iconified (minimized)? false = no, true = yes
- 6 = Is this window shaded (rolled up)? false = no, true = yes
- 7 = Is this window focused? fale = no, true = yes
- 8 = A reference back to the task you got info on
Definition at line 5045 of file karambainterface.cpp.
|
slot |
Task/getTaskList.
SYNOPSIS list getTaskList(widget) DESCRIPTION This returns a list with references to all the current windows open on this system. You can then call performTaskAction() or getTaskInfo() on any of the entries in the list. ARGUMENTS
- reference to widget – karamba RETURN VALUE list with references to the tasks
Definition at line 5079 of file karambainterface.cpp.
|
slot |
Task/getTaskNames.
SYNOPSIS list getTaskNames(widget) DESCRIPTION This returns a list containing the String names of all open windows on the system. This is for convience if you want to list open windows or see if a window by a certain name exists. Anything else requires the reference to the window you would obtain from getTaskList() ARGUMENTS
- reference to widget – karamba RETURN VALUE list with task names
Definition at line 5110 of file karambainterface.cpp.
Text/getTextAlign.
SYNOPSIS string getTextAlign(widget, text) DESCRIPTION Gets the current text alignment. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter RETURN VALUE LEFT, CENTER or RIGHT as string
Definition at line 5675 of file karambainterface.cpp.
|
slot |
Text/getTextColor.
SYNOPSIS array getTextColor(widget, text) DESCRIPTION Gets the current text color ARGUMENTS
- reference to widget – karamba
- reference to text – text meter RETURN VALUE (red, green, blue, alpha)
Definition at line 5610 of file karambainterface.cpp.
Text/getTextFont.
SYNOPSIS string getTextFont(widget, text) DESCRIPTION Gets the current text font name ARGUMENTS
- reference to widget – karamba
- reference to text – text meter RETURN VALUE font name
Definition at line 5564 of file karambainterface.cpp.
Text/getTextFontSize.
SYNOPSIS integer getTextFontSize(widget, text) DESCRIPTION Gets the current text font size. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter RETURN VALUE text font size
Definition at line 5654 of file karambainterface.cpp.
Text/getTextPos.
SYNOPSIS array getTextPos(widget, text) DESCRIPTION Given a reference to a text object, this will return an array containing the x and y coordinate of the text object. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter RETURN VALUE x and y coordinate
Definition at line 5307 of file karambainterface.cpp.
Text/getTextSensor.
SYNOPSIS string getTextSensor(widget, text) DESCRIPTION Gets the current sensor string ARGUMENTS
- reference to widget – karamba
- reference to text – text meter RETURN VALUE currently used sensor string
Definition at line 5342 of file karambainterface.cpp.
Text/getTextShadow.
SYNOPSIS integer getTextShadow(widget, text) DESCRIPTION Gets the current shadow offset ARGUMENTS
- reference to widget – karamba
- reference to text – text meter RETURN VALUE shadow offset
Definition at line 5516 of file karambainterface.cpp.
Text/getTextSize.
SYNOPSIS array getTextSize(widget, text) DESCRIPTION Given a reference to a text object, this will return an array containing the height and width of a text object. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter RETURN VALUE height and width of the text
Definition at line 5379 of file karambainterface.cpp.
Text/getTextTextWidth.
SYNOPSIS integer getTextTextWidth(widget, text) DESCRIPTION Gets the current text width. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter RETURN VALUE text width
Definition at line 5733 of file karambainterface.cpp.
Text/getTextValue.
SYNOPSIS string getTextValue(widget, text) DESCRIPTION Returns there current text value. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter RETURN VALUE value
Definition at line 5472 of file karambainterface.cpp.
Bar/getThemeBar.
SYNOPSIS reference getThemeBar(widget, name) DESCRIPTION You can reference a bar in your python code that was created in the theme file. Basically, you just add a NAME= value to the BAR line in the .theme file. Then if you want to use that object, instead of calling createBar, you can call this function.
The name you pass to the function is the same one that you gave it for the NAME= parameter in the .theme file. ARGUMENTS
- reference to widget – karamba
- string name – name of the bar in the theme file RETURN VALUE reference to bar
Definition at line 780 of file karambainterface.cpp.
Graph/getThemeGraph.
SYNOPSIS reference getThemeGraph(widget, name) DESCRIPTION You can reference graph in your python code that was created in the theme file. Basically, you just add a NAME= value to the GRAPH line in the .theme file. Then if you want to use that object, instead of calling createGraph, you can call this function.
The name you pass to the function is the same one that you gave it for the NAME= parameter in the .theme file. ARGUMENTS
- reference to widget – karamba
- string name – name of the graph in the theme file RETURN VALUE reference to graph
Definition at line 1158 of file karambainterface.cpp.
Image/getThemeImage.
SYNOPSIS long getThemeImage(widget, name) DESCRIPTION You can reference an image in your python code that was created in the .theme file. Basically, you just add a NAME= value to the IMAGE line in the .theme file. Then if you want to use that object, instead of calling createImage, you can call this function.
The name you pass to the function is the same one that you gave it for the NAME= parameter in the .theme file. ARGUMENTS
- reference to widget – karamba
- string name – name of the image in the theme files RETURN VALUE reference to image
Definition at line 1690 of file karambainterface.cpp.
|
slot |
InputBox/getThemeInputBox.
SYNOPSIS reference getThemeInputBox(widget, name) DESCRIPTION You can reference text in your python code that was created in the theme file. Basically, you just add a NAME= value to the INPUT line in the .theme file. Then if you want to use that object, instead of calling createInputBox, you can call this function.
The name you pass to the function is the same one that you gave it for the NAME= parameter in the .theme file. ARGUMENTS
- reference to widget – karamba
- string name – name of the Input Box in the theme file RETURN VALUE reference to Input Box
Definition at line 2624 of file karambainterface.cpp.
|
slot |
Misc/getThemePath.
SYNOPSIS string getThemePath(widget) DESCRIPTION Returns a string containing the directory where your theme was loaded from. ARGUMENTS
- reference to widget – karamba RETURN VALUE path to theme
Definition at line 3876 of file karambainterface.cpp.
|
slot |
RichText/getThemeRichText.
SYNOPSIS reference getThemeRichText(widget, name) DESCRIPTION You can reference richtext in your python code that was created in the theme file. Basically, you just add a NAME= value to the GRAPH line in the .theme file. Then if you want to use that object, instead of calling createRichText, you can call this function.
The name you pass to the function is the same one that you gave it for the NAME= parameter in the .theme file. ARGUMENTS
- long widget – karamba
- string name – name of the richtext in the theme file RETURN VALUE reference to richtext
Definition at line 4661 of file karambainterface.cpp.
Text/getThemeText.
SYNOPSIS reference getThemeText(widget, name) DESCRIPTION You can reference text in your python code that was created in the theme file. Basically, you just add a NAME= value to the TEXT line in the .theme file. Then if you want to use that object, instead of calling createText, you can call this function.
The name you pass to the function is the same one that you gave it for the NAME= parameter in the .theme file. ARGUMENTS
- long widget – karamba
- string name – name of the text in the theme file RETURN VALUE reference to text
Definition at line 5402 of file karambainterface.cpp.
|
slot |
Misc/getUpdateTime.
SYNOPSIS decimal getUpdateTime(widget) DESCRIPTION Returns the last stored update time. Intended for use so that the next refresh interval can work out how long ago the mouse was last moved over the widget. ARGUMENTS
- reference to widget – karamba RETURN VALUE last stored update time (from setUpdateTime)
Definition at line 3902 of file karambainterface.cpp.
|
slot |
Widget/getWidgetPosition.
SYNOPSIS array getWidgetPosition(widget) DESCRIPTION Returns an array containing the x and y position of you widget. widget is a reference to the current widget. ARGUMENTS
- reference to widget – karamba RETURN VALUE position of the widget
Definition at line 5781 of file karambainterface.cpp.
|
slot |
Misc/hide.
SYNOPSIS boolean hide(widget) DESCRIPTION Hides the widget. ARGUMENTS
- reference to widget – karamba RETURN VALUE true if successful
Definition at line 3947 of file karambainterface.cpp.
Bar/hideBar.
SYNOPSIS boolean hideBar(widget, bar) DESCRIPTION This hides an bar. In other words, during subsequent calls to widgetUpdate(), this bar will not be drawn. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar RETURN VALUE true if successful
Definition at line 798 of file karambainterface.cpp.
Graph/hideGraph.
SYNOPSIS boolean hideGraph(widget, graph) DESCRIPTION This hides a graph. In other words, during subsequent calls to widgetUpdate(), this graph will not be drawn. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE true if successful
Definition at line 1176 of file karambainterface.cpp.
|
slot |
Image/hideImage.
SYNOPSIS boolean hideImage(widget, image) DESCRIPTION This hides an image. In other words, during subsequent calls to widgetUpdate(), this image will not be drawn. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE true if successful
Definition at line 1708 of file karambainterface.cpp.
InputBox/hideInputBox.
SYNOPSIS boolean hideInputBox(widget, inputBox) DESCRIPTION Hides a Input Box that is visible. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box RETURN VALUE true if successful
Definition at line 2641 of file karambainterface.cpp.
|
slot |
RichText/hideRichText.
SYNOPSIS boolean hideRichText(widget, richtext) DESCRIPTION This hides the richtext. In other words, during subsequent calls to widgetUpdate(), this richtext will not be drawn. ARGUMENTS
- reference to widget – karamba
- reference to richtext – richtext RETURN VALUE true if successful
Definition at line 4679 of file karambainterface.cpp.
|
slot |
Definition at line 4868 of file karambainterface.cpp.
Text/hideText.
SYNOPSIS boolean hideText(widget, text) DESCRIPTION Hides text that is visible. You need to call redrawWidget() afterwords to actually hide the text on screen. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter RETURN VALUE true if successful
Definition at line 5420 of file karambainterface.cpp.
bool KarambaInterface::initInterpreter | ( | ) |
Definition at line 92 of file karambainterface.cpp.
|
signal |
|
signal |
|
slot |
Misc/language.
SYNOPSIS string language(widget) DESCRIPTION Returns a string containing the default language of a translation file. ARGUMENTS
- reference to widget – karamba RETURN VALUE default language or empty string if no translation files found.
Definition at line 3991 of file karambainterface.cpp.
|
slot |
Misc/managementPopup.
SYNOPSIS boolean managementPopup(widget) DESCRIPTION The right click menu of SuperKaramba allows themes to be loaded, closed, moved to other screens. If you want this popup menu to appear, call this function. ARGUMENTS
- reference to widget – karamba RETURN VALUE true if successful
Definition at line 4014 of file karambainterface.cpp.
|
signal |
|
signal |
Bar/moveBar.
SYNOPSIS boolean moveBar(widget, bar, x, y) DESCRIPTION This will move the bar to new x and y coordinates. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar
- integer x – x coordinate
- integer y – y coordinate RETURN VALUE boolean (true if successful)
Definition at line 632 of file karambainterface.cpp.
|
slot |
CanvasWidget/moveCanvasWidget.
SYNOPSIS boolean moveCanvasWidget(widget, canvaswidget, x, y, w, h) DESCRIPTION Moves the canvaswidget object to a new position. ARGUMENTS
- reference to widget – karamba
- reference to widget – the QGraphicsProxyWidget canvaswidget object which should be moved.
- integer x – x coordinate
- integer y – y coordinate
- integer w – width
- integer h – height RETURN VALUE true if the move was successful done else false.
Definition at line 5970 of file karambainterface.cpp.
Graph/moveGraph.
SYNOPSIS boolean moveGraph(widget, graph, x, y) DESCRIPTION This will move the graph to the new x and y coordinates. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph
- integer x – x coordinate
- integer y – y coordinate RETURN VALUE true if successful
Definition at line 1010 of file karambainterface.cpp.
|
slot |
Image/moveImage.
SYNOPSIS boolean moveImage(widget, image, x, y) DESCRIPTION This moves an image to a new x, y relative to your widget. In other words, (0,0) is the top corner of your widget, not the screen. The imageToMove parameter is a reference to the image to move that you saved as the return value from createImage() ARGUMENTS
- reference to widget – karamba
- reference to image – image
- integer x – x coordinate
- integer y – y coordinate RETURN VALUE true if successful
Definition at line 1530 of file karambainterface.cpp.
InputBox/moveInputBox.
SYNOPSIS long moveInputBox(widget, inputBox, x, y) DESCRIPTION This moves a Input Box object to a new x, y relative to your widget. In other words, (0,0) is the top corner of your widget, not the screen. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box
- integer x – x coordinate
- integer y – y coordinate RETURN VALUE true if successful
Definition at line 2511 of file karambainterface.cpp.
|
slot |
RichText/moveRichText.
SYNOPSIS boolean moveRichText(widget, richtext, x, y) DESCRIPTION This moves the rich text to a new x, y relative to your widget. In other words, (0,0) is the top corner of your widget, not the screen. ARGUMENTS
- reference to widget – karamba
- reference richtext – richtext
- integer x – x coordinate
- integer y – y coordinate RETURN VALUE true if successful
Definition at line 4503 of file karambainterface.cpp.
|
slot |
Definition at line 4884 of file karambainterface.cpp.
Text/moveText.
SYNOPSIS boolean moveText(widget, text, x, y) DESCRIPTION This moves a text object to a new x, y relative to your widget. In other words, (0,0) is the top corner of your widget, not the screen. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter
- integer x – x coordinate
- integer y – y coordinate RETURN VALUE true if successful
Definition at line 5289 of file karambainterface.cpp.
|
slot |
Widget/moveWidget.
SYNOPSIS boolean moveWidget(widget, x, y) DESCRIPTION Moves the widget to the new screen location. ARGUMENTS
- reference to widget – karamba
- integer x – x coordinate
- integer y – y coordinate RETURN VALUE true if successful
Definition at line 5809 of file karambainterface.cpp.
|
slot |
Misc/openNamedTheme.
SYNOPSIS reference openNamedTheme(theme, pretty_name, is_sub_theme) DESCRIPTION Opens a new theme, giving it a pretty (alternative and by your own choice unique) name. If you do not want the theme to be loaded when SuperKaramba is first started up (but instead want it to only be opened by this function call) then set is_sub_theme to 1. Themes opened with openNamedTheme will be unique. If a theme with the same pretty name already exists, openNamedTheme will have no effect. If you want duplicate themes (and a bit of a mess), use openTheme, instead. ARGUMENTS
- string theme – path to new theme
- string pretty_name – the name to be associated with the new widget
- boolean is_sub_theme – sets persistence (save state) of the theme RETURN VALUE reference to the new widget
Definition at line 4046 of file karambainterface.cpp.
|
slot |
Misc/openTheme.
SYNOPSIS reference openTheme(theme) DESCRIPTION Opens a new theme. ARGUMENTS
- string theme – path to theme that will be opened RETURN VALUE reference to the new widget
Definition at line 4074 of file karambainterface.cpp.
Task/performTaskAction.
SYNOPSIS boolean performTaskAction(widget, task, action) DESCRIPTION This peforms the given action on a task object. widget is a reference to the current widget. Task is a reference to a task object you got from getTaskList(). Action is a number from 1 to 10. See the list below.
Possible actions:
- 1 = Maximize the window
- 2 = Restore the window (use on iconified windows)
- 3 = Iconify the window (minimize it)
- 4 = Close the window
- 5 = Activate (give focus to) the window
- 6 = Raise the window
- 7 = Lower the window
- 8 = Smart Focus/Minimize - This will what the KDE taskbar does when you click on a window. If it is iconified, raise it. If it has focus, iconify it.
- 9 = Toggle whether this window is always on top
- 10 = Toggle whether this window is shaded (rolled up) ARGUMENTS
- reference to widget – karamba
- reference to task – task
- integer action – action number RETURN VALUE boolean if successful
Definition at line 5156 of file karambainterface.cpp.
|
slot |
Menu/popupMenu.
SYNOPSIS boolean popupMenu(widget, menu, x, y) DESCRIPTION This pops up the given menu at the given co-ordinates. The co-ordinates are relative to the widget, not the screen. You can use negative co-ordinates to make a menu appear to the right of or above your theme. ARGUMENTS
- reference to widget – karamba
- reference to menu – menu
- integer x – x coordinate
- integer y – y coordinate RETURN VALUE true if the menu existed and was popped up, returns false otherwise.
Definition at line 3349 of file karambainterface.cpp.
|
slot |
Config/readConfigEntry.
SYNOPSIS string readConfigEntry(widget, key, value) DESCRIPTION This function reads an entry from the config file with the given key. ARGUMENTS
- reference to widget – karamba
- string key – key for config item RETURN VALUE config value for key
Definition at line 2330 of file karambainterface.cpp.
|
slot |
Config/readMenuConfigOption.
SYNOPSIS boolean readMenuConfigOption(widget, key) DESCRIPTION This returns whether or not the given option is checked in the theme's Configure Theme menu.
See addMenuConfigOption for a more detailed explanation. ARGUMENTS
- reference to widget – karamba
- string key – key for menu item RETURN VALUE false is returned if it is not checked and true is returned if it is.
Definition at line 2357 of file karambainterface.cpp.
|
slot |
Misc/readThemeFile.
SYNOPSIS string readThemeFile(widget, file) DESCRIPTION Returns a string with the contents of the theme file ARGUMENTS
- reference to widget – karamba
- string file – name of the file to read RETURN VALUE file contents
Definition at line 4098 of file karambainterface.cpp.
|
slot |
Widget/redrawWidget.
SYNOPSIS boolean redrawWidget(widget) DESCRIPTION This is THE most important function. After you do a bunch of other calls (moving images, adding images or text, etc), you call this to update the widget display area. You will not see your changes until you call this. Redraws all meters. ARGUMENTS
- reference to widget – karamba RETURN VALUE true if successful
Definition at line 5834 of file karambainterface.cpp.
|
slot |
Widget/redrawWidgetBackground.
SYNOPSIS boolean redrawWidgetBackground(widget) DESCRIPTION Redraws the widget background. WARNING This function does nothing in SuperKaramba 0.50 and later ARGUMENTS
- reference to widget – karamba RETURN VALUE true if successful
Definition at line 5857 of file karambainterface.cpp.
|
slot |
Misc/reloadTheme.
SYNOPSIS boolean reloadTheme(theme) DESCRIPTION Reloads the current theme. ARGUMENTS
- reference to widget – karamba RETURN VALUE true if successful
Definition at line 4118 of file karambainterface.cpp.
Misc/removeClickArea.
SYNOPSIS boolean removeClickArea(widget, clickarea) DESCRIPTION This function deletes a clickable area. ARGUMENTS
- reference to widget – karamba
- reference to clickarea – click area RETURN VALUE true if successful
Definition at line 3588 of file karambainterface.cpp.
|
slot |
Image/removeImageEffects.
SYNOPSIS boolean removeImageEffects(widget, image) DESCRIPTION If you have called image effect commands on your image (ex: changeImageIntensity), you can call this to restore your image to it's original form. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE true if successful
Definition at line 2025 of file karambainterface.cpp.
|
slot |
Image/removeImageTransformations.
SYNOPSIS boolean removeImageTransformations(widget, image) DESCRIPTION If you have rotated or resized your image, you can call this function to restore your image to its original form. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE true if successful
Definition at line 2049 of file karambainterface.cpp.
|
slot |
Menu/removeMenuItem.
SYNOPSIS boolean removeMenuItem(widget, menu, id) DESCRIPTION This removes the menu if it exists. ARGUMENTS
- reference to widget – karamba
- reference to menu – pointer to menu
- reference to menu item – menu item RETURN VALUE 1 if the menu item existed and was removed or returns zero otherwise.
Definition at line 3376 of file karambainterface.cpp.
Bar/resizeBar.
SYNOPSIS boolean resizeBar(widget, bar, w, h) DESCRIPTION This will resize bar to new height and width. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar
- integer w – new width
- integer h – new height RETURN VALUE true if successful
Definition at line 704 of file karambainterface.cpp.
|
slot |
Graph/resizeGraph.
SYNOPSIS boolean resizeGraph(widget, graph, width, height) DESCRIPTION This will resize graph to new height and width. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph
- integer width – new width
- integer height – new height RETURN VALUE true if successful
Definition at line 1082 of file karambainterface.cpp.
|
slot |
Image/resizeImage.
SYNOPSIS boolean resizeImage(widget, image, w, h) DESCRIPTION This resizes your image to width, height. The image parameter is a reference to an image that you saved as the return value from createImage() ARGUMENTS
- reference widget – karamba
- reference image – image
- integer w – width
- integer h – height RETURN VALUE true if successful
Definition at line 1605 of file karambainterface.cpp.
|
slot |
Image/resizeImageSmooth.
SYNOPSIS boolean resizeImageSmooth(widget, image, w, h) DESCRIPTION DEPRECATED: resizeImage now allows the user to pick whether to use fast or smooth resizing from the SuperKaramba menu - This resizes your image to width, height. The imageToResize parameter is a reference to an image that you saved as the return value from createImage()
In version 0.50 and later this function works exactly as resizeImage(). ARGUMENTS
- reference to widget – karamba
- reference to image – image
- integer w – width
- integer h – height RETURN VALUE true if successful
Definition at line 2079 of file karambainterface.cpp.
|
slot |
InputBox/resizeInputBox.
SYNOPSIS boolean resizeInputBox(widget, inputBox, w, h) DESCRIPTION This will resize Input Box to the new height and width. ARGUMENTS
- reference to widget – karamba
- referenc to inputBox – Input Box
- integer w – new width
- integer h – new height RETURN VALUE true if successful
Definition at line 2548 of file karambainterface.cpp.
|
slot |
RichText/resizeRichText.
SYNOPSIS boolean resizeRichText(widget, richtext, w, h) DESCRIPTION This will resize richtext to the new height and width. ARGUMENTS
- reference to widget – karamba
- reference to richtext – richtext
- integer w – new width
- integer h – new height RETURN VALUE true if successful
Definition at line 4540 of file karambainterface.cpp.
|
slot |
Text/resizeText.
SYNOPSIS boolean resizeText(widget, text, w, h) DESCRIPTION This will resize text to the new height and width. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter
- integer w – new width
- integer h – new height RETURN VALUE true if successful
Definition at line 5361 of file karambainterface.cpp.
|
slot |
Widget/resizeWidget.
SYNOPSIS boolean resizeWidget(widget, w, h) DESCRIPTION Resizes the widget to the new width and height. ARGUMENTS
- reference to widget – karamba
- integer w – width
- integer h – height RETURN VALUE true if successful
Definition at line 5877 of file karambainterface.cpp.
|
slot |
Image/rotateImage.
SYNOPSIS boolean rotateImage(widget, image, deg) DESCRIPTION This rotates your image to by the specified amount of degrees. The The image parameter is a reference to an image that you saved as the return value from createImage() ARGUMENTS
- reference to widget – karamba
- reference to image – image
- integer deg – degrees to rotate RETURN VALUE true if successful
Definition at line 2106 of file karambainterface.cpp.
|
slot |
Misc/run.
SYNOPSIS boolean run(name, command, icon, list_of_args) DESCRIPTION This command simply executes a program or command on the system. The difference between run and execute is that run takes arguments, and the name of the icon to be displayed. ARGUMENTS
- string name – name to be displayed
- string command – command to execute
- string icon – name of icon to be displayed
- string list_of_args – arguments to be passed to the command RETURN VALUE true if successful
Definition at line 4145 of file karambainterface.cpp.
|
slot |
Bar/setBarImage.
SYNOPSIS boolean setBarImage(widget, bar, image) DESCRIPTION Use the image in path for the background as the bar ARGUMENTS
- reference to widget – karamba
- reference to bar – bar
- string image – path to the new image RETURN VALUE true if successful
Definition at line 878 of file karambainterface.cpp.
Bar/setBarMinMax.
SYNOPSIS boolean setBarMinMax(widget, bar, min, max) DESCRIPTION Returns current bar value. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar
- integer min – min value
- integer max – max value RETURN VALUE true if successful
Definition at line 596 of file karambainterface.cpp.
Bar/setBarSensor.
SYNOPSIS boolean setBarSensor(widget, bar, sensor) DESCRIPTION Sets the sensor string of the bar. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar
- string sensor – new sensor string as in theme files RETURN VALUE true if successful
Definition at line 668 of file karambainterface.cpp.
Bar/setBarValue.
SYNOPSIS reference setBarValue(widget, bar, value) DESCRIPTION Sets current bar value. ARGUMENTS
- long widget – karamba
- long bar – pointer to bar
- long value – new value RETURN VALUE reference to bar
Definition at line 740 of file karambainterface.cpp.
Bar/setBarVertical.
SYNOPSIS boolean setBarVertical(widget, bar) DESCRIPTION Set bar vertical ARGUMENTS
- reference to widget – karamba
- reference to bar – bar
- boolean vertical – true to draw the bar vertically RETURN VALUE true if successful
Definition at line 834 of file karambainterface.cpp.
|
slot |
Graph/setGraphColor.
SYNOPSIS boolean setGraphColor(widget, graph, red, green, blue, alpha=255) DESCRIPTION Sets current graph color. ARGUMENTS
- reference to widget – karamba
- reference to graph – pointer to graph
- integer red – red component of color
- integer green – green component of color
- integer blue – blue component of color
- integer alpha – alpha component of color (optional) RETURN VALUE true if successful
Definition at line 1215 of file karambainterface.cpp.
|
slot |
Graph/setGraphFillColor.
SYNOPSIS boolean setGraphFillColor(widget, graph, red, green, blue, alpha=255) DESCRIPTION Sets current graph fill color. ARGUMENTS
- reference to widget – karamba
- reference to graph – pointer to graph
- integer red – red component of color
- integer green – green component of color
- integer blue – blue component of color
- integer alpha – alpha component of color (optional) RETURN VALUE true if successful
Definition at line 1254 of file karambainterface.cpp.
|
slot |
Graph/setGraphMinMax.
SYNOPSIS boolean setGraphMinMax(widget, graph, min, max) DESCRIPTION Sets the graphs min and max values. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph
- integer min – min value
- integer max – max value RETURN VALUE true if successful
Definition at line 974 of file karambainterface.cpp.
|
slot |
Graph/setGraphPlot.
SYNOPSIS bool setGraphPlot(widget, graph, plot) DESCRIPTION sets the direction that the graph plots positive numbers to be 'up' or 'down' ARGUMENTS
- reference to widget – karamba
- reference to graph – graph
- reference to new plot direction value – plot RETURN VALUE bool to indicate success
Definition at line 1372 of file karambainterface.cpp.
|
slot |
Graph/getGraphScroll.
SYNOPSIS bool getGraphScroll(widget, graph) DESCRIPTION get string description of the scroll direction of 'left' or 'right' ARGUMENTS
- reference to widget – karamba
- reference to graph – graph
- reference to new scroll value – scroll RETURN VALUE bool to indicate success
Definition at line 1328 of file karambainterface.cpp.
|
slot |
Graph/setGraphSensor.
SYNOPSIS boolean setGraphSensor(widget, graph, sensor) DESCRIPTION Sets current sensor string of the graph. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph
- string sensor – new sensor string as in theme files RETURN VALUE true if successful
Definition at line 1046 of file karambainterface.cpp.
|
slot |
Graph/setGraphShouldFill.
SYNOPSIS bool setGraphShouldFill(widget, graph, shouldFill) DESCRIPTION set whether or not the graph should draw itself filled ARGUMENTS
- reference to widget – karamba
- reference to graph – graph
- bool to dictate fill – shouldFill RETURN VALUE bool to indicate success
Definition at line 1416 of file karambainterface.cpp.
|
slot |
Graph/setGraphValue.
SYNOPSIS reference setGraphValue(widget, graph, value) DESCRIPTION Sets current graph value. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph
- integer value – new value RETURN VALUE reference to graph
Definition at line 1118 of file karambainterface.cpp.
|
slot |
Image/setImageElement.
SYNOPSIS boolean setImageElement(widget, image, element) DESCRIPTION This function will only draw the named element of an SVG image. ARGUMENTS
- reference to widget – karamba
- reference to image – image
- string element – ID of the element to draw RETURN VALUE true if the element is available or false if it is not an SVG image.
Definition at line 2211 of file karambainterface.cpp.
|
slot |
Image/setImageElementAll.
SYNOPSIS boolean setImageElementAll(widget, image) DESCRIPTION This function reset a previous set element. A call to this function will result in a completely drawn SVG image. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE true if successful
Definition at line 2234 of file karambainterface.cpp.
|
slot |
Image/setImagePath.
SYNOPSIS reference setImagePath(widget, image, path) DESCRIPTION This will change image of a image widget. Note, starting with SuperKaramba version 0.50 (KDE4.0) this function accepts SVG. ARGUMENTS
- reference to widget – karamba
- reference to image – image
- string path – new path of the image RETURN VALUE reference to the image
Definition at line 1649 of file karambainterface.cpp.
|
slot |
Image/setImageSensor.
SYNOPSIS long setImageSensor(widget, image, sensor) DESCRIPTION Sets the current sensor string. ARGUMENTS
- reference to widget – karamba
- reference to image – image
- string sensor – new sensor string as in theme files RETURN VALUE true if successful
Definition at line 1566 of file karambainterface.cpp.
|
slot |
Misc/setIncomingData.
SYNOPSIS boolean setIncomingData(widget, theme, info) DESCRIPTION Contacts a theme - identified by the pretty name - and stores a string to be associated with the remote theme. The difference between setIncomingData and callTheme is that the theme is not notified by setIncomingData that the data has arrived. Previous information, if any, is overwritten. Use getIncomingData to retrieve the last stored information. setIncomingData is not very sophisticated, and could benefit from having info passed to it put into a queue, instead of being overwritten. ARGUMENTS
- reference to widget – karamba
- string theme – path to theme to have information passed to it.
- string info – a string containing the info to be passed to the theme RETURN VALUE true if successful
Definition at line 4199 of file karambainterface.cpp.
InputBox/setInputFocus.
SYNOPSIS boolean setInputFocus(widget, inputBox) DESCRIPTION Sets the input focus to the Input Box. ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box RETURN VALUE true if successful
Definition at line 3060 of file karambainterface.cpp.
|
slot |
Config/setMenuConfigOption.
SYNOPSIS boolean setMenuConfigOption(widget, key, value) DESCRIPTION This sets whether or not the given option is checked in the theme's Configure Theme menu. Value should be false if key should not be checked and true if key should be checked.
See addMenuConfigOption for a more detailed explanation. ARGUMENTS
- referencce to widget – karamba
- string key – key for menu item
- boolean value – true for checked RETURN VALUE true if successful
Definition at line 2383 of file karambainterface.cpp.
|
slot |
Image/setPixel.
SYNOPSIS boolean setPixel(widget, image, x, y, r, g, b, a=255) DESCRIPTION This sets the pixel in the image at the position x, y to the color r, g, b with the alpha channel a. You can use this function to draw your own images with your theme script. ARGUMENTS
- reference to widget – karamba
- reference to image – image
- integer x – x coordinate of the pixel
- integer y – y coordinate of the pixel
- integer r – red component of color
- integer g – green component of color
- integer b – blue component of color
- integer a – alpha component of color (optional) RETURN VALUE true if successful
Definition at line 2138 of file karambainterface.cpp.
|
slot |
RichText/setRichTextSensor.
SYNOPSIS boolean setRichTextSensor(widget, richtext, sensor) DESCRIPTION Sets a new sensor string ARGUMENTS
- reference to widget – karamba
- reference to richtext – richtext
- string sensor – new sensor string as in theme files RETURN VALUE true if successful
Definition at line 4805 of file karambainterface.cpp.
|
slot |
RichText/setRichTextWidth.
SYNOPSIS boolean setRichTextWidth(widget, richtext, width) DESCRIPTION Given a reference to a rich text object, this function changes it's width to the specified value in pixels.
The height adjusts automatically as the contents are changed with changeRichText. ARGUMENTS
- reference to widget – karamba
- reference to richtext – richtext
- integer width – new width in pixels RETURN VALUE true if successful
Definition at line 4563 of file karambainterface.cpp.
|
slot |
Text/setTextAlign.
SYNOPSIS boolean setTextAlign(widget, text, align) DESCRIPTION Sets the text label align. ARGUMENTS
- reference to widget – karamba
- reference to text – text meter
- string align – LEFT, CENTER or RIGHT as string RETURN VALUE true if successful
Definition at line 5697 of file karambainterface.cpp.
|
slot |
Definition at line 5709 of file karambainterface.cpp.
|
slot |
Text/setTextSensor.
SYNOPSIS boolean setTextSensor(widget, text, sensor) DESCRIPTION Sets a new sensor string ARGUMENTS
- reference to widget – karamba
- reference to text – text meter
- string sensor – new sensor string as in theme files RETURN VALUE true if successful
Definition at line 5325 of file karambainterface.cpp.
|
slot |
Misc/setUpdateTime.
SYNOPSIS boolean getUpdateTime(widget, updated_time) DESCRIPTION Sets the update time. Intended for use so that the next refresh interval can work out how long ago the mouse was last moved over the widget. ARGUMENTS
- reference to widget – karamba
- integer updated_time – the update time to be associated with the widget RETURN VALUE true if successful
Definition at line 3925 of file karambainterface.cpp.
|
slot |
Misc/setWantRightButton.
SYNOPSIS boolean setWantRightButton(widget, want_receive_right_button) DESCRIPTION The right click menu of SuperKaramba allows themes to be loaded, closed, moved to other screens. Not all themes will want the right click menu. Call karamba.wantRightButton(widget, 1) if you want to receive MouseUpdate button notifications. ARGUMENTS
- reference to widget – karamba
- boolean want_receive_right_button – whether the widget will receive right clicks RETURN VALUE true if successful
Definition at line 4329 of file karambainterface.cpp.
|
slot |
Misc/show.
SYNOPSIS boolean show(widget) DESCRIPTION Shows the widget. ARGUMENTS
- reference to widget – karamba RETURN VALUE true if successful
Definition at line 3969 of file karambainterface.cpp.
Bar/showBar.
SYNOPSIS boolean showBar(widget, bar) DESCRIPTION This shows an bar. In other words, during subsequent calls to widgetUpdate(), this bar will be drawn. ARGUMENTS
- reference to widget – karamba
- reference to bar – bar RETURN VALUE true if successful
Definition at line 816 of file karambainterface.cpp.
Graph/showGraph.
SYNOPSIS boolean showGraph(widget, graph) DESCRIPTION This shows a graph. In other words, during subsequent calls to widgetUpdate(), this graph will be drawn. ARGUMENTS
- reference to widget – karamba
- reference to graph – graph RETURN VALUE true if successful
Definition at line 1194 of file karambainterface.cpp.
|
slot |
Image/showImage.
SYNOPSIS boolean showImage(widget, image) DESCRIPTION This shows a previously hidden image. It does not actually refresh the image on screen. That is what redrawWidget() does. ARGUMENTS
- reference to widget – karamba
- reference to image – image RETURN VALUE true if successful
Definition at line 1726 of file karambainterface.cpp.
InputBox/showInputBox.
SYNOPSIS boolean showInputBox(widget, inputBox) DESCRIPTION Shows Input Box that has been hidden with hideInputBox() ARGUMENTS
- reference to widget – karamba
- reference to inputBox – Input Box RETURN VALUE true if successful
Definition at line 2658 of file karambainterface.cpp.
|
slot |
RichText/showRichText.
SYNOPSIS boolean showRichText(widget, richtext) DESCRIPTION This shows the richtext. In other words, during subsequent calls to widgetUpdate(), this richtext will be drawn. ARGUMENTS
- reference to widget – karamba
- reference to richtext – richtext RETURN VALUE true if successful
Definition at line 4697 of file karambainterface.cpp.
|
slot |
Definition at line 4905 of file karambainterface.cpp.
Text/showText.
SYNOPSIS boolean showText(widget, text) DESCRIPTION Shows text that has been hidden with hideText(). ARGUMENTS
- reference to widget – karamba
- reference to text – text meter RETURN VALUE true if successful
Definition at line 5437 of file karambainterface.cpp.
void KarambaInterface::startInterpreter | ( | ) |
Definition at line 83 of file karambainterface.cpp.
|
signal |
|
slot |
Misc/toggleShowDesktop.
SYNOPSIS boolean toggleShowDesktop(widget) DESCRIPTION This shows/hides the current desktop just like the Show Desktop button on kicker. Basically, it minimizes all the windows on the current desktop. Call it once to show the desktop and again to hide it. ARGUMENTS
- reference to widget – karamba RETURN VALUE true if successful
Definition at line 4222 of file karambainterface.cpp.
|
slot |
Widget/toggleWidgetRedraw.
SYNOPSIS boolean toggleWidgetRedraw(widget, b) DESCRIPTION Toggles widget redraw. WARNING This function does nothing in SuperKaramba 0.50 and later ARGUMENTS
- reference to widget – karamba
- boolean b – 1 = widget is drawn RETURN VALUE true if successful
Definition at line 5902 of file karambainterface.cpp.
|
slot |
Misc/translateAll.
SYNOPSIS boolean translateAll(widget, relative_x, relative_y) DESCRIPTION Moves all widgets within a theme in a particular direction relative from the previous spot without moving the parent theme widget. ARGUMENTS
- reference to widget – karamba
- integer translate_x – move horizontally
- integer translate_y – move vertically RETURN VALUE true if successful
Definition at line 4247 of file karambainterface.cpp.
|
slot |
Definition at line 4921 of file karambainterface.cpp.
|
slot |
Misc/userLanguage.
SYNOPSIS string userLanguage(widget) DESCRIPTION Returns a string containing the global KDE user language. ARGUMENTS
- reference to widget – karamba RETURN VALUE user language or empty string
Definition at line 4278 of file karambainterface.cpp.
|
slot |
Misc/userLanguages.
SYNOPSIS array userLanguages(widget) DESCRIPTION Returns an array containing the language name abbreviations for the preferred interface languages user chose for KDE session in Region & Language settings. Having the whole array of preferred languages available is important for cases when you cannot provide interface translation for the 1st preferred language, but can for consecutive languages. (Implemented in version 0.42.) ARGUMENTS
- reference to widget – karamba RETURN VALUE list (array) with user languages in the order of preference.
Definition at line 4304 of file karambainterface.cpp.
|
slot |
Misc/version.
SYNOPSIS array version() DESCRIPTION Returns the (API) version of the running SuperKaramba. This allows theme writers to use new functionality with keeping backward compatibility. RETURN VALUE array with SuperKaramba version and API version
Definition at line 4351 of file karambainterface.cpp.
|
signal |
|
signal |
|
signal |
|
signal |
|
signal |
|
slot |
Config/writeConfigEntry.
SYNOPSIS boolean writeConfigEntry(widget, key, value) DESCRIPTION SuperKaramba automatically supports configuration files for each theme. These files will be saved in /your/home/dir/.superkaramba/ and will be named themenamerc where themename is the name of the theme.
This function writes an entry into the config file with the given key and value.
For example, to save my favorite color, I would do karamba.writeConfigEntry(widget, "FavColor", "Red") ARGUMENTS
- reference to widget – karamba
- string key – key for config item
- string value – config value RETURN VALUE true if successful
Definition at line 2413 of file karambainterface.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:07:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.