kgoldrunner
#include <kgrrunner.h>
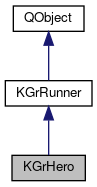
Signals | |
void | soundSignal (const int n, const bool onOff=true) |
![]() | |
void | incScore (const int n) |
void | startAnimation (const int spriteId, const bool repeating, const int i, const int j, const int time, const Direction dirn, const AnimationType type) |
Public Member Functions | |
KGrHero (KGrLevelPlayer *pLevelPlayer, KGrLevelGrid *pGrid, int i, int j, int pSpriteId, KGrRuleBook *pRules) | |
~KGrHero () | |
bool | dig (const Direction dirn, int &digI, int &digJ) |
HeroStatus | run (const int scaledTime) |
void | setNuggets (const int nGold) |
void | showState () |
![]() | |
KGrRunner (KGrLevelPlayer *pLevelPlayer, KGrLevelGrid *pGrid, int i, int j, const int pSpriteId, KGrRuleBook *pRules, const int startDelay) | |
virtual | ~KGrRunner () |
int | whereAreYou (int &x, int &y) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
void | getRules () |
char | nextCell () |
bool | setNextMovement (const char spriteType, const char cellType, Direction &dir, AnimationType &anim, int &interval) |
Situation | situation (const int scaledTime) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
AnimationType | currAnimation |
Direction | currDirection |
int | deltaX |
int | deltaY |
int | enemyFallTime |
bool | falling |
int | fallTime |
KGrLevelGrid * | grid |
int | gridI |
int | gridJ |
int | gridX |
int | gridY |
int | interval |
bool | leftRightSearch |
KGrLevelPlayer * | levelPlayer |
int | pointCtr |
int | pointsPerCell |
KGrRuleBook * | rules |
int | runTime |
int | spriteId |
QTime | t |
int | timeLeft |
int | trapTime |
bool | turnAnywhere |
![]() | |
objectName | |
Detailed Description
This class models the behaviour of the hero.
It inherits from KGrRunner.
The hero's main functions are running, digging holes in bricks and collecting gold. If he is caught by an enemy or trapped in a closing brick, he dies. If he collects all the gold and runs to the top row, he wins the level.
Definition at line 148 of file kgrrunner.h.
Constructor & Destructor Documentation
KGrHero::KGrHero | ( | KGrLevelPlayer * | pLevelPlayer, |
KGrLevelGrid * | pGrid, | ||
int | i, | ||
int | j, | ||
int | pSpriteId, | ||
KGrRuleBook * | pRules | ||
) |
The constructor of the KGrHero class.
The parameters are the same as for the KGrRunner constructor, which does most of the work, but this constructor also initialises the hero's timing, which depends on the rules being used.
- Parameters
-
pLevelPlayer The object that owns the hero and will destroy him if the KGoldrunner application is terminated during play. The object also provides helper functions to the hero. pGrid The grid on which the hero is playing. i The starting column-number (>=1). j The starting row-number (>=1). pSpriteId The sprite ID of the hero, as used in animation. pRules The rules that apply to this game and level.
Definition at line 198 of file kgrrunner.cpp.
KGrHero::~KGrHero | ( | ) |
Definition at line 210 of file kgrrunner.cpp.
Member Function Documentation
bool KGrHero::dig | ( | const Direction | dirn, |
int & | digI, | ||
int & | digJ | ||
) |
Decides whether the hero can dig as is required by pressing a key or a mouse-button.
If OK, the KGrLevelPlayer will control the dug brick.
- Parameters
-
dirn The direction in which to dig: L or R of the hero. digI The column-number of the brick (return by reference). digJ The row-number of the brick (return by reference).
- Returns
- If true, a hole can be dug in the direction required.
Definition at line 305 of file kgrrunner.cpp.
HeroStatus KGrHero::run | ( | const int | scaledTime | ) |
Makes the hero run, under control of a pointer or the keyboard and guided by the layout of the grid.
The method is invoked by a periodic timer and returns NORMAL status while play continues. If the hero is caught by an enemy or trapped in a brick, it returns DEAD status, or if he collects all the gold and reaches the top row, the method returns WON_LEVEL status. Otherwise it changes the hero's position as required and decides the type of animation to display (run left, climb, etc.).
- Parameters
-
scaledTime The amount by which to adjust the time, scaled according to the current game-speed setting. Smaller times cause slower running in real-time: larger times cause faster running.
- Returns
- The hero's status: NORMAL, DEAD or WON_LEVEL.
Definition at line 214 of file kgrrunner.cpp.
|
inline |
Tells the hero how many gold nuggets are remaining.
- Parameters
-
nGold The number of gold nuggets remaining.
Definition at line 207 of file kgrrunner.h.
void KGrHero::showState | ( | ) |
Implements the author's debugging aid that shows the hero's state.
Definition at line 344 of file kgrrunner.cpp.
|
signal |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:24 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.