libkdegames/libkdegamesprivate/kgame
#include <KGame/KGameChat>
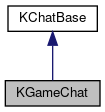
Public Types | |
enum | SendingIds { SendToGroup = 1 } |
Public Slots | |
virtual void | addMessage (const QString &fromName, const QString &text) |
virtual void | addMessage (int fromId, const QString &text) |
void | slotReceiveMessage (int, const QByteArray &, quint32 receiver, quint32 sender) |
Public Member Functions | |
KGameChat (KGame *game, int msgid, KPlayer *fromPlayer, QWidget *parent, KChatBaseModel *model=0, KChatBaseItemDelegate *delegate=0) | |
KGameChat (KGame *game, int msgId, QWidget *parent, KChatBaseModel *model=0, KChatBaseItemDelegate *delegate=0) | |
KGameChat (QWidget *parent) | |
virtual | ~KGameChat () |
virtual QString | fromName () const |
KPlayer * | fromPlayer () const |
KGame * | game () const |
int | messageId () const |
void | setFromPlayer (KPlayer *player) |
void | setKGame (KGame *g) |
void | setMessageId (int msgid) |
Protected Slots | |
void | slotAddPlayer (KPlayer *) |
void | slotPropertyChanged (KGamePropertyBase *, KPlayer *) |
void | slotReceivePrivateMessage (int msgid, const QByteArray &buffer, quint32 sender, KPlayer *me) |
void | slotRemovePlayer (KPlayer *) |
void | slotUnsetKGame () |
Protected Member Functions | |
bool | hasPlayer (int id) const |
bool | isSendToAllMessage (int id) const |
bool | isToGroupMessage (int id) const |
bool | isToPlayerMessage (int id) const |
int | playerId (int id) const |
virtual void | returnPressed (const QString &text) |
int | sendingId (int playerId) const |
virtual QString | sendToPlayerEntry (const QString &name) const |
Detailed Description
A Chat widget for KGame-based games.
Call setFromPlayer() first - this will be used as the "from" part of every message you will send. Otherwise it won't work! You can also use the fromPlayer parameter in the constructor though...
Definition at line 45 of file kgamechat.h.
Member Enumeration Documentation
Enumerator | |
---|---|
SendToGroup |
Definition at line 72 of file kgamechat.h.
Constructor & Destructor Documentation
KGameChat::KGameChat | ( | KGame * | game, |
int | msgid, | ||
KPlayer * | fromPlayer, | ||
QWidget * | parent, | ||
KChatBaseModel * | model = 0 , |
||
KChatBaseItemDelegate * | delegate = 0 |
||
) |
Construct a KGame chat widget on game
that used msgid
for the chat message.
The fromPlayer
is the local player (see setFromPlayer).
Definition at line 64 of file kgamechat.cpp.
KGameChat::KGameChat | ( | KGame * | game, |
int | msgId, | ||
QWidget * | parent, | ||
KChatBaseModel * | model = 0 , |
||
KChatBaseItemDelegate * | delegate = 0 |
||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. To make use of this widget you need to call setFromPlayer manually.
Definition at line 57 of file kgamechat.cpp.
|
explicit |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. This constructs a widget that is not usable.
You must call at least setGame, setFromPlayer and setMessageId manually.
Definition at line 72 of file kgamechat.cpp.
|
virtual |
Definition at line 79 of file kgamechat.cpp.
Member Function Documentation
|
inlinevirtualslot |
Definition at line 121 of file kgamechat.h.
|
virtualslot |
Definition at line 93 of file kgamechat.cpp.
|
virtual |
reimplemented from KChatBase
- Returns
- KPlayer::name() for the player set by setFromPlayer
Definition at line 192 of file kgamechat.cpp.
KPlayer * KGameChat::fromPlayer | ( | ) | const |
Definition at line 252 of file kgamechat.cpp.
KGame * KGameChat::game | ( | ) | const |
Definition at line 247 of file kgamechat.cpp.
|
protected |
- Returns
- True if the player with this ID was added before (see slotAddPlayer)
Definition at line 195 of file kgamechat.cpp.
|
protected |
- Parameters
-
id The ID of the sending entry, as returned by KChatBase::sendingEntry
- Returns
- True if the entry "send to all" was selected, otherwise false
Definition at line 159 of file kgamechat.cpp.
|
protected |
Used to indicate whether a message shall be sent to a group of players.
Note that this was not yet implemented when this doc was written so this description might be wrong. (FIXME)
- Parameters
-
id The ID of the sending entry, as returned by KChatBase::sendingEntry
- Returns
- True if the message is meant to be sent to a group (see KPlayer::group), e.g. if "send to my group" was selected.
Definition at line 162 of file kgamechat.cpp.
|
protected |
Used to indicate whether the message shall be sent to a single player only.
Note that you can also call isSendToAllMessage and isToGroupMessage - if both return false it must be a player message. This behaviour might be changed later - so don't depend on it.
See also toPlayerId
- Parameters
-
id The ID of the sending entry, as returned by KChatBase::sendingEntry
- Returns
- True if the message shall be sent to a special player, otherwise false.
Definition at line 165 of file kgamechat.cpp.
int KGameChat::messageId | ( | ) | const |
- Returns
- The id of the messages produced by KGameChat. The id will be used in KGame as parameter msgid in the method KGame::sendMessage
Definition at line 156 of file kgamechat.cpp.
|
protected |
- Parameters
-
id The ID of the sending entry, as returned by KChatBase::sendingEntry
- Returns
- The ID of the player (see KPlayer::id) the sending entry belongs to. Note that the parameter id is an id as returned by ref KChatBase::sendingEntry and the id this method returns is a KPlayer ID. If isToPlayerMessage returns false this method returns -1
Definition at line 172 of file kgamechat.cpp.
|
protectedvirtual |
Definition at line 110 of file kgamechat.cpp.
|
protected |
- Parameters
-
playerId The ID of the KPlayer object
- Returns
- The ID of the sending entry (see KChatBase) or -1 if the player id was not found.
Definition at line 181 of file kgamechat.cpp.
- Parameters
-
name The name of the added player
- Returns
- A string that will be added as sending entry in KChatBase. By default this is "send to name" where name is the name that you specify. See also KChatBase::addSendingEntry
Definition at line 169 of file kgamechat.cpp.
void KGameChat::setFromPlayer | ( | KPlayer * | player | ) |
This sets the fromPlayer to player
.
The fromPlayer is the player that will appear as "from" when you send messages through this widget.
- Parameters
-
player The player of this widget
Definition at line 200 of file kgamechat.cpp.
void KGameChat::setKGame | ( | KGame * | g | ) |
Set the KGame object for this chat widget.
All messages will be sent through this object. You don't have to implement any send functions, just call this function, call setFromPlayer and be done :-)
- Parameters
-
g The KGame object the messages will be sent through
Definition at line 223 of file kgamechat.cpp.
void KGameChat::setMessageId | ( | int | msgid | ) |
Change the message id of the chat widget.
It is recommended that you don't use this but prefer the constructor instead, but in certain situations (such as using this widget in Qt designer) it may be useful to change the message id.
See also messageId
Definition at line 153 of file kgamechat.cpp.
|
protectedslot |
Definition at line 272 of file kgamechat.cpp.
|
protectedslot |
Definition at line 309 of file kgamechat.cpp.
|
slot |
Definition at line 331 of file kgamechat.cpp.
|
protectedslot |
Called when KPlayer::signalNetworkData is emitted.
The message gets forwarded to slotReceiveMessage if me
equals fromPlayer.
Definition at line 322 of file kgamechat.cpp.
|
protectedslot |
Definition at line 292 of file kgamechat.cpp.
|
protectedslot |
Unsets a KGame object that has been set using setKGame before.
You don't have to call this - this is usually done automatically.
Definition at line 257 of file kgamechat.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.