libkdegames/libkdegamesprivate/kgame
#include <KGame/KGameNetwork>
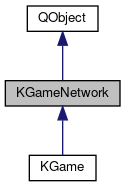
Signals | |
void | signalAdminStatusChanged (bool isAdmin) |
void | signalClientConnected (quint32 clientID) |
void | signalClientDisconnected (quint32 clientID, bool broken) |
void | signalConnectionBroken () |
void | signalNetworkErrorMessage (int error, QString text) |
Public Member Functions | |
KGameNetwork (int cookie=42, QObject *parent=0) | |
virtual | ~KGameNetwork () |
bool | connectToServer (const QString &host, quint16 port) |
bool | connectToServer (KMessageIO *connection) |
int | cookie () const |
virtual void | Debug () |
void | disconnect () |
void | electAdmin (quint32 clientID) |
quint32 | gameId () const |
QString | hostName () const |
bool | isAdmin () const |
bool | isMaster () const |
bool | isNetwork () const |
bool | isOfferingConnections () const |
virtual void | lock () |
KMessageClient * | messageClient () const |
KMessageServer * | messageServer () const |
virtual void | networkTransmission (QDataStream &, int, quint32, quint32, quint32 clientID)=0 |
bool | offerConnections (quint16 port) |
quint16 | port () const |
void | sendError (int error, const QByteArray &message, quint32 receiver=0, quint32 sender=0) |
bool | sendMessage (const QByteArray &buffer, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendMessage (const QDataStream &msg, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendMessage (const QString &msg, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendMessage (int data, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendSystemMessage (const QByteArray &buffer, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendSystemMessage (int data, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendSystemMessage (const QDataStream &msg, int msgid, quint32 receiver=0, quint32 sender=0) |
bool | sendSystemMessage (const QString &msg, int msgid, quint32 receiver=0, quint32 sender=0) |
void | setDiscoveryInfo (const QString &type, const QString &name=QString()) |
void | setMaxClients (int max) |
bool | stopServerConnection () |
virtual void | unlock () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Slots | |
void | aboutToLoseConnection (quint32 id) |
void | receiveNetworkTransmission (const QByteArray &a, quint32 clientID) |
void | slotAdminStatusChanged (bool isAdmin) |
void | slotResetConnection () |
Protected Member Functions | |
void | setMaster () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
The KGameNetwork class is the KGame class with network support.
All other features are the same but they are now network transparent. It is not used directly but only via a KGame object. So you do not really have to bother with this object.
The main KDE game object
Definition at line 46 of file kgamenetwork.h.
Constructor & Destructor Documentation
|
explicit |
Create a KGameNetwork object.
Definition at line 61 of file kgamenetwork.cpp.
|
virtual |
Definition at line 74 of file kgamenetwork.cpp.
Member Function Documentation
|
protectedslot |
Called when the network connection is about to terminate.
Is used to store the network parameter like the game id
Definition at line 335 of file kgamenetwork.cpp.
bool KGameNetwork::connectToServer | ( | const QString & | host, |
quint16 | port | ||
) |
Inits a network game as a network CLIENT.
- Parameters
-
host the host to which we want to connect port the port we want to connect to
- Returns
- true if connected
Definition at line 204 of file kgamenetwork.cpp.
bool KGameNetwork::connectToServer | ( | KMessageIO * | connection | ) |
}
Definition at line 221 of file kgamenetwork.cpp.
int KGameNetwork::cookie | ( | ) | const |
Application cookie.
this idendifies the game application. It help to distinguish between e.g. KPoker and KWin4
- Returns
- the application cookie
Definition at line 98 of file kgamenetwork.cpp.
|
virtual |
Gives debug output of the game status.
Reimplemented in KGame.
Definition at line 517 of file kgamenetwork.cpp.
void KGameNetwork::disconnect | ( | ) |
Disconnect the current connection and establish a new local one.
Definition at line 285 of file kgamenetwork.cpp.
void KGameNetwork::electAdmin | ( | quint32 | clientID | ) |
If you are the ADMIN of the game you can give the ADMIN status away to another client.
Use this e.g. if you want to quit the game or if you want another client to administrate the game (note that disconnect calls this automatically).
- Parameters
-
clientID the ID of the new ADMIN (note: this is the _client_ID which has nothing to do with the player IDs. See KMessageServer)
Definition at line 347 of file kgamenetwork.cpp.
quint32 KGameNetwork::gameId | ( | ) | const |
QString KGameNetwork::hostName | ( | ) | const |
- Returns
- The name of the host that we are currently connected to is isNetwork is TRUE and we are not the MASTER, i.e. if connectToServer was called. Otherwise this will return "localhost".
Definition at line 266 of file kgamenetwork.cpp.
bool KGameNetwork::isAdmin | ( | ) | const |
The admin of a game is the one who initializes newly connected clients using negotiateNetworkGame and is allowed to configure the game.
E.g. only the admin is allowed to use KGame::setMaxPlayers.
If one KGame object in the game is MASTER then this client is the admin as well. isMaster and isAdmin differ only if the KMessageServer is running in an own process.
- Returns
- Whether this client (KGame object) is the admin
Definition at line 104 of file kgamenetwork.cpp.
bool KGameNetwork::isMaster | ( | ) | const |
Is this the game MASTER (i.e.
has started theKMessageServer). A game has always exactly one MASTER. This is either a KGame object (i.e. a Client) or an own MessageServer-process. A KGame object that has the MASTER status is always admin.
You probably don't want to use this. It is a mostly internal method which will probably become protected. Better use isAdmin
- See also
- isAdmin
- Returns
- Whether this client has started the KMessageServer
Definition at line 101 of file kgamenetwork.cpp.
bool KGameNetwork::isNetwork | ( | ) | const |
- Returns
- TRUE if this is a network game - i.e. you are either MASTER or connected to a remote MASTER.
Definition at line 83 of file kgamenetwork.cpp.
bool KGameNetwork::isOfferingConnections | ( | ) | const |
Are we still offer offering server connections - only for game MASTER.
- Returns
- true/false
Definition at line 282 of file kgamenetwork.cpp.
|
virtual |
You should call this before doing thigs like, e.g.
qApp->processEvents(). Don't forget to call unlock once you are done!
- See also
- KMessageClient::lock
Definition at line 373 of file kgamenetwork.cpp.
KMessageClient * KGameNetwork::messageClient | ( | ) | const |
Don't use this unless you really know what youre doing! You might experience some strange behaviour if you send your messages directly through the KMessageClient!
- Returns
- a pointer to the KMessageClient used internally to send the messages. You should rather use one of the send functions!
Definition at line 107 of file kgamenetwork.cpp.
KMessageServer * KGameNetwork::messageServer | ( | ) | const |
Don't use this unless you really know what you are doing! You might experience some strange behaviour if you use the message server directly!
- Returns
- a pointer to the message server if this is the MASTER KGame object. Note that it might be possible that no KGame object contains the KMessageServer at all! It might even run stand alone!
Definition at line 110 of file kgamenetwork.cpp.
|
pure virtual |
bool KGameNetwork::offerConnections | ( | quint16 | port | ) |
Inits a network game as network MASTER.
Note that if the KMessageServer is not yet started it will be started here (see setMaster). Any existing connection will be disconnected.
If you already offer connections the port is changed.
- Parameters
-
port The port on which the service is offered
- Returns
- true if it worked
Definition at line 174 of file kgamenetwork.cpp.
quint16 KGameNetwork::port | ( | ) | const |
- Returns
- The port we are listening to if offerConnections was called or the port we are connected to if connectToServer was called. Otherwise 0.
Definition at line 254 of file kgamenetwork.cpp.
|
protectedslot |
Called by KMessageClient::broadcastReceived() and will check if the message format is valid.
If it is not, it will generate an error (see signalNetworkVersionError and signalNetworkErorrMessage). If it is valid, the pure virtual method networkTransmission() is called. (This one is overwritten in KGame.)
Definition at line 473 of file kgamenetwork.cpp.
void KGameNetwork::sendError | ( | int | error, |
const QByteArray & | message, | ||
quint32 | receiver = 0 , |
||
quint32 | sender = 0 |
||
) |
Sends a network message.
- Parameters
-
error The error code message The error message - use KGameError receiver the KGame / KPlayer this message is for. 0 For all sender The KGame / KPlayer this message is from (i.e. you). You probably want to leave this 0, then KGameNetwork will create the correct value for you. You might want to use this if you send a message from a specific player.
Definition at line 462 of file kgamenetwork.cpp.
bool KGameNetwork::sendMessage | ( | const QByteArray & | buffer, |
int | msgid, | ||
quint32 | receiver = 0 , |
||
quint32 | sender = 0 |
||
) |
Send a network message msg with a given message ID msgid to all clients.
You want to use this to send a message to the clients.
Note that a message is always sent to ALL clients! This is necessary so that all clients always have the same data and can easily be changed from network to non-network without restarting the game. If you want a specific KGame / KPlayer to react to the message use the receiver and sender parameters. See KGameMessage::calsMessageId
SendMessage differs from sendSystemMessage only by the msgid parameter. sendSystemMessage is thought as a KGame only mehtod while sendMessage is for public use. The msgid parameter will be +=KGameMessage::IdUser and in KGame::signalNetworkData msgid will be -= KGameMessage::IdUser again, so that one can easily distinguish between system and user messages.
Use sendSystemMessage to comunicate with KGame (e.g. by adding a player) and sendMessage for your own user message.
Note: a player should send messages through a KGameIO!
- Parameters
-
buffer the message which will be send. See messages.txt for contents msgid an id for this message. See KGameMessage::GameMessageIds receiver the KGame / KPlayer this message is for. sender The KGame / KPlayer this message is from (i.e. you). You probably want to leave this 0, then KGameNetwork will create the correct value for you. You might want to use this if you send a message from a specific player.
- Returns
- true if worked
Definition at line 459 of file kgamenetwork.cpp.
bool KGameNetwork::sendMessage | ( | const QDataStream & | msg, |
int | msgid, | ||
quint32 | receiver = 0 , |
||
quint32 | sender = 0 |
||
) |
This is an overloaded member function, provided for convenience.
Definition at line 456 of file kgamenetwork.cpp.
bool KGameNetwork::sendMessage | ( | const QString & | msg, |
int | msgid, | ||
quint32 | receiver = 0 , |
||
quint32 | sender = 0 |
||
) |
This is an overloaded member function, provided for convenience.
Definition at line 453 of file kgamenetwork.cpp.
bool KGameNetwork::sendMessage | ( | int | data, |
int | msgid, | ||
quint32 | receiver = 0 , |
||
quint32 | sender = 0 |
||
) |
This is an overloaded member function, provided for convenience.
Definition at line 450 of file kgamenetwork.cpp.
bool KGameNetwork::sendSystemMessage | ( | const QByteArray & | buffer, |
int | msgid, | ||
quint32 | receiver = 0 , |
||
quint32 | sender = 0 |
||
) |
Sends a network message msg with a given msg id msgid to all clients.
Use this to communicate with KGame (e.g. to add a player ot to configure the game - usually not necessary).
For your own messages use sendMessage instead! This is mostly internal!
- Parameters
-
buffer the message which will be send. See messages.txt for contents msgid an id for this message. See KGameMessage::GameMessageIds receiver the KGame / KPlayer this message is for. sender The KGame / KPlayer this message is from (i.e. you). You probably want to leave this 0, then KGameNetwork will create the correct value for you. You might want to use this if you send a message from a specific player.
- Returns
- true if worked
Definition at line 408 of file kgamenetwork.cpp.
bool KGameNetwork::sendSystemMessage | ( | int | data, |
int | msgid, | ||
quint32 | receiver = 0 , |
||
quint32 | sender = 0 |
||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 389 of file kgamenetwork.cpp.
bool KGameNetwork::sendSystemMessage | ( | const QDataStream & | msg, |
int | msgid, | ||
quint32 | receiver = 0 , |
||
quint32 | sender = 0 |
||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 405 of file kgamenetwork.cpp.
bool KGameNetwork::sendSystemMessage | ( | const QString & | msg, |
int | msgid, | ||
quint32 | receiver = 0 , |
||
quint32 | sender = 0 |
||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 397 of file kgamenetwork.cpp.
Definition at line 150 of file kgamenetwork.cpp.
|
protected |
Start a KMessageServer object and use it as the MASTER of the game. Note that you must not call this if there is already another master running!
Definition at line 114 of file kgamenetwork.cpp.
void KGameNetwork::setMaxClients | ( | int | max | ) |
Changes the maximal connection number of the KMessageServer to max.
-1 Means infinite connections are possible. Note that existing connections are not affected, so even if you set this to 0 in a running game no client is being disconnected. You can call this only if you are the ADMIN!
- See also
- KMessageServer::setMaxClients
- Parameters
-
max The maximal number of connections possible.
Definition at line 360 of file kgamenetwork.cpp.
|
signal |
This client gets or loses the admin status.
- Parameters
-
isAdmin True if this client gets the ADMIN status otherwise FALSE
|
signal |
This signal is emitted whenever the KMessageServer sends us a message that a new client connected.
KGame uses this to call KGame::negotiateNetworkGame for the newly connected client if we are admin (see isAdmin)
- Parameters
-
clientID the ID of the newly connected client
|
signal |
This signal is emitted whenever the KMessageServer sends us a message that a connection to a client was detached.
The second parameter can be used to distinguish between network errors or removing on purpose.
- Parameters
-
clientID the client that has disconnected broken true if the connection was lost because of a network error, false if the connection was closed by the message server admin.
|
signal |
Our connection to the KMessageServer has broken.
|
signal |
A network error occurred.
- Parameters
-
error the error code text the error text
|
protectedslot |
This KGame object receives or loses the admin status.
- Parameters
-
isAdmin Whether we are admin or not
Definition at line 510 of file kgamenetwork.cpp.
|
protectedslot |
Called when the network connection is terminated.
Used to clean up the disconnect parameter
Definition at line 341 of file kgamenetwork.cpp.
bool KGameNetwork::stopServerConnection | ( | ) |
Stops offering server connections - only for game MASTER.
- Returns
- true
Definition at line 271 of file kgamenetwork.cpp.
|
virtual |
- See also
- KMessageClient::unlock
Definition at line 380 of file kgamenetwork.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.