libkdegames/libkdegamesprivate/kgame
#include <KGame/KGamePropertyHandler>
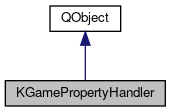
Signals | |
void | signalPropertyChanged (KGamePropertyBase *) |
void | signalRequestValue (KGamePropertyBase *property, QString &value) |
void | signalSendMessage (int msgid, QDataStream &, bool *sent) |
Public Member Functions | |
KGamePropertyHandler (QObject *parent=0) | |
KGamePropertyHandler (int id, const QObject *receiver, const char *sendf, const char *emitf, QObject *parent=0) | |
~KGamePropertyHandler () | |
bool | addProperty (KGamePropertyBase *data, const QString &name=QString()) |
void | clear () |
void | Debug () |
QMultiHash< int, KGamePropertyBase * > & | dict () const |
void | emitSignal (KGamePropertyBase *data) |
KGamePropertyBase * | find (int id) |
void | flush () |
int | id () const |
virtual bool | load (QDataStream &stream) |
void | lockDirectEmit () |
void | lockProperties () |
KGamePropertyBase::PropertyPolicy | policy () |
bool | processMessage (QDataStream &stream, int id, bool isSender) |
QString | propertyName (int id) const |
QString | propertyValue (KGamePropertyBase *property) |
void | registerHandler (int id, const QObject *receiver, const char *send, const char *emit) |
bool | removeProperty (KGamePropertyBase *data) |
virtual bool | save (QDataStream &stream) |
void | sendLocked (bool l) |
bool | sendProperty (QDataStream &s) |
void | setId (int id) |
void | setPolicy (KGamePropertyBase::PropertyPolicy p, bool userspace=true) |
int | uniquePropertyId () |
void | unlockDirectEmit () |
void | unlockProperties () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
A collection class for KGameProperty objects.
The KGamePropertyHandler class is some kind of a collection class for KGameProperty. You usually don't have to create one yourself, as both KPlayer and KGame provide a handler. In most cases you do not even have to care about the KGamePropertHandler. KGame and KPlayer implement all features of KGamePropertyHandler so you will rather use it there.
You have to use the KGamePropertyHandler as parent for all KGameProperty objects but you can also use KPlayer or KGame as parent - then KPlayer::dataHandler or KGame::dataHandler will be used.
Every KGamePropertyHandler must have - just like every KGameProperty - a unique ID. This ID is provided either in the constructor or in registerHandler. The ID is used to assign an incoming message (e.g. a changed property) to the correct handler. Inside the handler the property ID is used to change the correct property.
The constructor or registerHandler takes 3 addittional arguments: a receiver and two slots. The first slot is connected to signalSendMessage, the second to signalPropertyChanged. You must provide these in order to use the KGamePropertyHandler.
The most important function of KGamePropertyHandler is processMessage which assigns an incoming value to the correct property.
A KGamePropertyHandler is also used - indirectly using emitSignal - to emit a signal when the value of a property changes. This is done this way because a KGameProperty does not inherit QObject because of memory advantages. Many games can have dozens or even hundreds of KGameProperty objects so every additional variable in KGameProperty would be multiplied.
Definition at line 73 of file kgamepropertyhandler.h.
Constructor & Destructor Documentation
KGamePropertyHandler::KGamePropertyHandler | ( | QObject * | parent = 0 | ) |
Construct an unregistered KGamePropertyHandler.
You have to call registerHandler before you can use this handler!
Definition at line 62 of file kgamepropertyhandler.cpp.
KGamePropertyHandler::KGamePropertyHandler | ( | int | id, |
const QObject * | receiver, | ||
const char * | sendf, | ||
const char * | emitf, | ||
QObject * | parent = 0 |
||
) |
Construct a registered handler.
- See also
- registerHandler
Definition at line 56 of file kgamepropertyhandler.cpp.
KGamePropertyHandler::~KGamePropertyHandler | ( | ) |
Definition at line 67 of file kgamepropertyhandler.cpp.
Member Function Documentation
bool KGamePropertyHandler::addProperty | ( | KGamePropertyBase * | data, |
const QString & | name = QString() |
||
) |
Adds a KGameProperty property to the handler.
- Parameters
-
data the property name A description of the property, which will be returned by propertyName. This is used for debugging
- Returns
- true on success
Definition at line 144 of file kgamepropertyhandler.cpp.
void KGamePropertyHandler::clear | ( | ) |
Clear the KGamePropertyHandler.
Note that the properties are not deleted so if you created your KGameProperty objects dynamically like
you also have to delete it:
Definition at line 329 of file kgamepropertyhandler.cpp.
void KGamePropertyHandler::Debug | ( | ) |
Writes some debug output to the console.
Definition at line 386 of file kgamepropertyhandler.cpp.
QMultiHash< int, KGamePropertyBase * > & KGamePropertyHandler::dict | ( | ) | const |
Reference to the internal dictionary.
Definition at line 348 of file kgamepropertyhandler.cpp.
void KGamePropertyHandler::emitSignal | ( | KGamePropertyBase * | data | ) |
called by a property to emit a signal This call is simply forwarded to the parent object
Definition at line 296 of file kgamepropertyhandler.cpp.
KGamePropertyBase * KGamePropertyHandler::find | ( | int | id | ) |
- Parameters
-
id The ID of the property. See KGamePropertyBase::id
- Returns
- The KGameProperty this ID is assigned to
Definition at line 322 of file kgamepropertyhandler.cpp.
void KGamePropertyHandler::flush | ( | ) |
Sends all properties which are marked dirty over the network.
This will make a forced synchornisation of the properties and mark them all not dirty.
Definition at line 262 of file kgamepropertyhandler.cpp.
int KGamePropertyHandler::id | ( | ) | const |
- Returns
- the id of the handler
Definition at line 75 of file kgamepropertyhandler.cpp.
|
virtual |
Loads properties from the datastream.
- Parameters
-
stream the datastream to load from
- Returns
- true on success otherwise false
Definition at line 181 of file kgamepropertyhandler.cpp.
void KGamePropertyHandler::lockDirectEmit | ( | ) |
Called by the KGame or KPlayer object or the handler itself to delay emmiting of signals.
Lockign keeps a counter and unlock is only achieved when every lock is canceld by an unlock. While this is set signals are quequed and only emmited after this is reset. Its deeper meaning is to prevent inconsistencies in a game load or network transfer where a emit could access a property not yet loaded or transmitted. Calling this by yourself you better know what your are doing.
Definition at line 276 of file kgamepropertyhandler.cpp.
void KGamePropertyHandler::lockProperties | ( | ) |
Calls KGamePropertyBase::setReadOnly(true) for all properties of this handler.
Use with care! This will even lock the core properties, like name, group and myTurn!!
- See also
- unlockProperties
Definition at line 248 of file kgamepropertyhandler.cpp.
KGamePropertyBase::PropertyPolicy KGamePropertyHandler::policy | ( | ) |
Returns the default policy for this property handler.
All properties registered newly, will have this property.
Definition at line 220 of file kgamepropertyhandler.cpp.
bool KGamePropertyHandler::processMessage | ( | QDataStream & | stream, |
int | id, | ||
bool | isSender | ||
) |
Main message process function.
This has to be called by the parent's message event handler. If the id of the message agrees with the id of the handler, the message is extracted and processed. Otherwise false is returned. Example:
- Parameters
-
stream The data stream containing the message id the message id of the message isSender Whether the receiver is also the sender
- Returns
- true on message processed otherwise false
Definition at line 96 of file kgamepropertyhandler.cpp.
QString KGamePropertyHandler::propertyName | ( | int | id | ) | const |
- Parameters
-
id The ID of the property
- Returns
- A name of the property which can be used for debugging. Don't depend on this function! It it possible not to provide a name or to provide the same name for multiple properties!
Definition at line 165 of file kgamepropertyhandler.cpp.
QString KGamePropertyHandler::propertyValue | ( | KGamePropertyBase * | property | ) |
In several situations you just want to have a QString of a KGameProperty object.
This function will provide you with such a QString for all the types used inside of all KGame classes. If you have a non-standard property (probably a self defined class or something like this) you also need to connect to signalRequestValue to make this function useful.
- Parameters
-
property Return the value of this KGameProperty
- Returns
- The value of a KGameProperty
Definition at line 353 of file kgamepropertyhandler.cpp.
void KGamePropertyHandler::registerHandler | ( | int | id, |
const QObject * | receiver, | ||
const char * | send, | ||
const char * | emit | ||
) |
Register the handler with a parent.
This is to use if the constructor without arguments has been chosen. Otherwise you need not call this.
- Parameters
-
id The id of the message to listen for receiver The object that will receive the signals of KGamePropertyHandler send A slot that is being connected to signalSendMessage emit A slot that is being connected to signalPropertyChanged
Definition at line 85 of file kgamepropertyhandler.cpp.
bool KGamePropertyHandler::removeProperty | ( | KGamePropertyBase * | data | ) |
Removes a property from the handler.
- Parameters
-
data the property
- Returns
- true on success
Definition at line 134 of file kgamepropertyhandler.cpp.
|
virtual |
Saves properties into the datastream.
- Parameters
-
stream the datastream to save to
- Returns
- true on success otherwise false
Definition at line 203 of file kgamepropertyhandler.cpp.
void KGamePropertyHandler::sendLocked | ( | bool | l | ) |
bool KGamePropertyHandler::sendProperty | ( | QDataStream & | s | ) |
called by a property to send itself into the datastream.
This call is simply forwarded to the parent object
Definition at line 315 of file kgamepropertyhandler.cpp.
void KGamePropertyHandler::setId | ( | int | id | ) |
Use id as new ID for this KGamePropertyHandler.
This is used internally only.
Definition at line 80 of file kgamepropertyhandler.cpp.
void KGamePropertyHandler::setPolicy | ( | KGamePropertyBase::PropertyPolicy | p, |
bool | userspace = true |
||
) |
Set the policy for all kgame variables which are currently registerd in the KGame proeprty handler.
See KGamePropertyBase::setPolicy
- Parameters
-
p is the new policy for all properties of this handler userspace if userspace is true (default) only user properties are changed. Otherwise the system properties are also changed.
Definition at line 225 of file kgamepropertyhandler.cpp.
|
signal |
This is emitted by a property.
KGamePropertyBase::emitSignal calls emitSignal which emits this signal.
This signal is emitted whenever the property is changed. Note that you can switch off this behaviour using KGamePropertyBase::setEmittingSignal in favor of performance. Note that you won't experience any performance loss using signals unless you use dozens or hundreds of properties which change very often.
|
signal |
If you call propertyValue with a non-standard KGameProperty it is possible that the value cannot automatically be converted into a QString.
Then this signal is emitted and asks you to provide the correct value. You probably want to use something like this to achieve this:
- Parameters
-
property The KGamePropertyBase the value is requested for value The value of this property. You have to set this.
|
signal |
This signal is emitted when a property needs to be sent.
Only the parent has to react to this.
- Parameters
-
msgid The id of the handler sent set this to true if the property was sent successfully - otherwise don't touch
int KGamePropertyHandler::uniquePropertyId | ( | ) |
returns a unique property ID starting called usually with a base of KGamePropertyBase::IdAutomatic.
This is used internally by the property base to assign automtic id's. Not much need to call this yourself.
Definition at line 257 of file kgamepropertyhandler.cpp.
void KGamePropertyHandler::unlockDirectEmit | ( | ) |
Removes the lock from the emitting of property signals.
Corresponds to the lockIndirectEmits
Definition at line 281 of file kgamepropertyhandler.cpp.
void KGamePropertyHandler::unlockProperties | ( | ) |
Calls KGamePropertyBase::setReadOnly(false) for all properties of this player.
See also lockProperties
Definition at line 239 of file kgamepropertyhandler.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.