KDECore
#include <KConfigBackend>
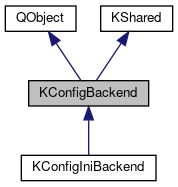
Public Types | |
enum | ParseInfo { ParseOk, ParseImmutable, ParseOpenError } |
enum | ParseOption { ParseGlobal = 1, ParseDefaults = 2, ParseExpansions = 4 } |
enum | WriteOption { WriteGlobal = 1 } |
Public Member Functions | |
virtual | ~KConfigBackend () |
virtual KConfigBase::AccessMode | accessMode () const =0 |
virtual void | createEnclosing ()=0 |
QString | filePath () const |
virtual bool | isLocked () const =0 |
virtual bool | isWritable () const =0 |
QDateTime | lastModified () const |
virtual bool | lock (const KComponentData &componentData)=0 |
virtual QString | nonWritableErrorMessage () const =0 |
virtual ParseInfo | parseConfig (const QByteArray &locale, KEntryMap &pWriteBackMap, ParseOptions options=ParseOptions())=0 |
virtual void | setFilePath (const QString &path)=0 |
qint64 | size () const |
virtual void | unlock ()=0 |
virtual bool | writeConfig (const QByteArray &locale, KEntryMap &entryMap, WriteOptions options, const KComponentData &data)=0 |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
QSharedData () | |
QSharedData (const QSharedData &other) | |
Static Public Member Functions | |
static KSharedPtr< KConfigBackend > | create (const KComponentData &componentData, const QString &fileName=QString(), const QString &system=QString()) |
static void | registerMappings (const KEntryMap &entryMap) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
KConfigBackend () | |
void | setLastModified (const QDateTime &dt) |
void | setLocalFilePath (const QString &file) |
void | setSize (qint64 sz) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Provides the implementation for accessing configuration sources.
KDELibs only provides an INI backend, but this class can be used to create plugins that allow access to other file formats and configuration systems.
Definition at line 55 of file kconfigbackend.h.
Member Enumeration Documentation
Return value from parseConfig()
Enumerator | |
---|---|
ParseOk | |
ParseImmutable |
the configuration was opened read/write |
ParseOpenError |
the configuration is immutable the configuration could not be opened |
Definition at line 109 of file kconfigbackend.h.
Allows the behaviour of parseConfig() to be tuned.
Enumerator | |
---|---|
ParseGlobal | |
ParseDefaults |
entries should be marked as global |
ParseExpansions |
entries should be marked as default |
Definition at line 93 of file kconfigbackend.h.
Allows the behaviour of writeConfig() to be tuned.
Enumerator | |
---|---|
WriteGlobal |
Definition at line 102 of file kconfigbackend.h.
Constructor & Destructor Documentation
|
virtual |
Destroys the backend.
Definition at line 98 of file kconfigbackend.cpp.
|
protected |
Definition at line 93 of file kconfigbackend.cpp.
Member Function Documentation
|
pure virtual |
- Returns
- the read/write status of the configuration object
- See also
- KConfigBase::AccessMode
Implemented in KConfigIniBackend.
|
static |
Creates a new KConfig backend.
If no system
is given, or the given system
is unknown, this method tries to determine the correct backend to use.
- Parameters
-
componentData the owning component fileName the absolute file name of the configuration file system the configuration system to use
- Returns
- a KConfigBackend object to be used with KConfig
Definition at line 64 of file kconfigbackend.cpp.
|
pure virtual |
Create the enclosing object of the configuration object.
For example, if the configuration object is a file, this should create the parent directory.
Implemented in KConfigIniBackend.
QString KConfigBackend::filePath | ( | ) | const |
- Returns
- the absolute path to the object
Definition at line 123 of file kconfigbackend.cpp.
|
pure virtual |
- Returns
true
if the file is locked,false
if it is not locked
Implemented in KConfigIniBackend.
|
pure virtual |
If isWritable() returns false, writeConfig() will always fail.
- Returns
true
if the configuration is writable,false
if it is immutable
Implemented in KConfigIniBackend.
QDateTime KConfigBackend::lastModified | ( | ) | const |
- Returns
- the date and time when the object was last modified
Definition at line 103 of file kconfigbackend.cpp.
|
pure virtual |
Lock the file.
Implemented in KConfigIniBackend.
|
pure virtual |
When isWritable() returns false
, return an error message to explain to the user why saving configuration will not work.
The return value when isWritable() returns true
is undefined.
- Returns
- a translated user-visible explanation for the configuration object not being writable
Implemented in KConfigIniBackend.
|
pure virtual |
Read persistent storage.
- Parameters
-
locale the locale to read entries for (if the backend supports localized entries) pWriteBackMap the KEntryMap where the entries are placed options
- See also
- ParseOptions
- Returns
- See also
- ParseInfo
Implemented in KConfigIniBackend.
|
static |
Registers mappings from directories/files to configuration systems.
Allows you to tell KConfigBackend that create() should use a particular backend for a particular file or directory.
- Warning
- currently does nothing
- Parameters
-
entryMap the KEntryMap to build the mappings from
Definition at line 60 of file kconfigbackend.cpp.
|
pure virtual |
Set the file path.
- Note
path
MUST be absolute.
- Parameters
-
path the absolute file path
Implemented in KConfigIniBackend.
|
protected |
Definition at line 108 of file kconfigbackend.cpp.
|
protected |
Definition at line 128 of file kconfigbackend.cpp.
|
protected |
Definition at line 118 of file kconfigbackend.cpp.
qint64 KConfigBackend::size | ( | ) | const |
- Returns
- the size of the object
Definition at line 113 of file kconfigbackend.cpp.
|
pure virtual |
Release the lock on the file.
Implemented in KConfigIniBackend.
|
pure virtual |
Write the dirty entries to permanent storage.
- Parameters
-
locale the locale to write entries for (if the backend supports localized entries) entryMap the KEntryMap containing the config object's entries. options
- See also
- WriteOptions
- Parameters
-
data the component that requested the write
- Returns
true
if the write was successful,false
if writing the configuration failed
Implemented in KConfigIniBackend.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.