KDEUI
#include <kdialog.h>
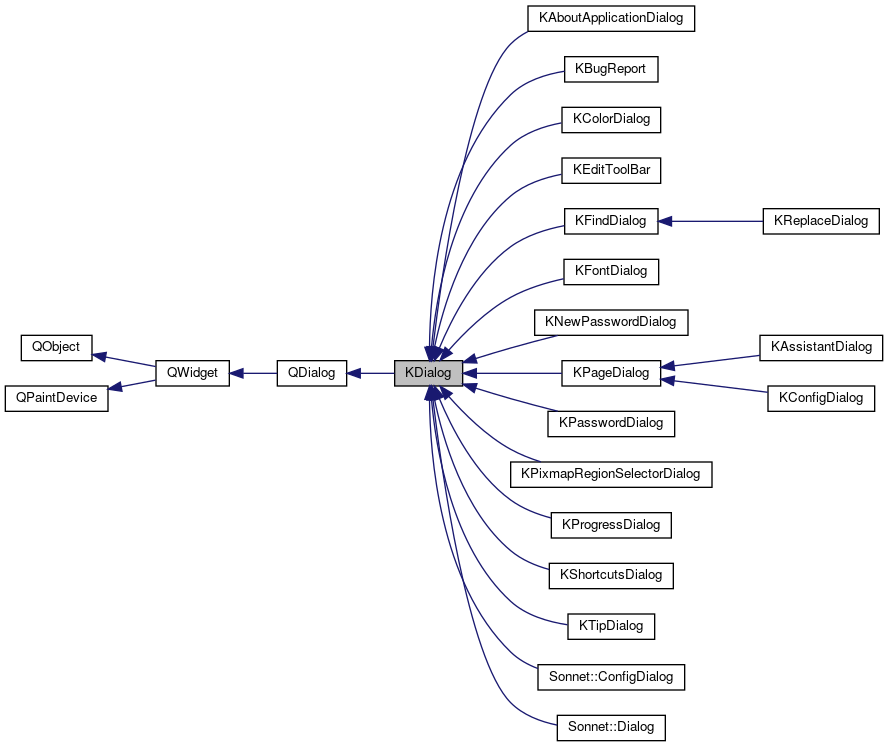
Public Types | |
enum | ButtonCode { None = 0x00000000, Help = 0x00000001, Default = 0x00000002, Ok = 0x00000004, Apply = 0x00000008, Try = 0x00000010, Cancel = 0x00000020, Close = 0x00000040, No = 0x00000080, Yes = 0x00000100, Reset = 0x00000200, Details = 0x00000400, User1 = 0x00001000, User2 = 0x00002000, User3 = 0x00004000, NoDefault = 0x00008000 } |
enum | ButtonPopupMode { InstantPopup = 0, DelayedPopup = 1 } |
enum | CaptionFlag { NoCaptionFlags = 0, AppNameCaption = 1, ModifiedCaption = 2, HIGCompliantCaption = AppNameCaption } |
Public Slots | |
void | delayedDestruct () |
void | enableButton (ButtonCode id, bool state) |
void | enableButtonApply (bool state) |
void | enableButtonCancel (bool state) |
void | enableButtonOk (bool state) |
void | enableLinkedHelp (bool state) |
bool | isDetailsWidgetVisible () const |
virtual void | setCaption (const QString &caption) |
virtual void | setCaption (const QString &caption, bool modified) |
void | setDetailsWidget (QWidget *detailsWidget) |
void | setDetailsWidgetVisible (bool visible) |
void | setHelp (const QString &anchor, const QString &appname=QString()) |
void | setHelpLinkText (const QString &text) |
virtual void | setPlainCaption (const QString &caption) |
Signals | |
void | aboutToShowDetails () |
void | applyClicked () |
void | buttonClicked (KDialog::ButtonCode button) |
void | cancelClicked () |
void | closeClicked () |
void | defaultClicked () |
void | finished () |
void | helpClicked () |
void | hidden () |
void | layoutHintChanged () |
void | noClicked () |
void | okClicked () |
void | resetClicked () |
void | tryClicked () |
void | user1Clicked () |
void | user2Clicked () |
void | user3Clicked () |
void | yesClicked () |
Public Member Functions | |
KDialog (QWidget *parent=0, Qt::WindowFlags flags=0) | |
~KDialog () | |
KPushButton * | button (ButtonCode id) const |
KIcon | buttonIcon (ButtonCode id) const |
QString | buttonText (ButtonCode id) const |
QString | buttonToolTip (ButtonCode id) const |
QString | buttonWhatsThis (ButtonCode id) const |
ButtonCode | defaultButton () const |
QString | helpLinkText () const |
void | incrementInitialSize (const QSize &size) |
bool | isButtonEnabled (ButtonCode id) const |
QWidget * | mainWidget () |
virtual QSize | minimumSizeHint () const |
void | restoreDialogSize (const KConfigGroup &config) |
void | saveDialogSize (KConfigGroup &config, KConfigGroup::WriteConfigFlags options=KConfigGroup::Normal) const |
void | setButtonFocus (ButtonCode id) |
void | setButtonGuiItem (ButtonCode id, const KGuiItem &item) |
void | setButtonIcon (ButtonCode id, const KIcon &icon) |
void | setButtonMenu (ButtonCode id, QMenu *menu, ButtonPopupMode popupmode=InstantPopup) |
void | setButtons (ButtonCodes buttonMask) |
void | setButtonsOrientation (Qt::Orientation orientation) |
void | setButtonText (ButtonCode id, const QString &text) |
void | setButtonToolTip (ButtonCode id, const QString &text) |
void | setButtonWhatsThis (ButtonCode id, const QString &text) |
void | setDefaultButton (ButtonCode id) |
void | setEscapeButton (ButtonCode id) |
void | setInitialSize (const QSize &size) |
void | setMainWidget (QWidget *widget) |
void | showButton (ButtonCode id, bool state) |
void | showButtonSeparator (bool state) |
virtual QSize | sizeHint () const |
![]() | |
QDialog (QWidget *parent, QFlags< Qt::WindowType > f) | |
QDialog (QWidget *parent, const char *name, bool modal, QFlags< Qt::WindowType > f) | |
~QDialog () | |
virtual void | accept () |
void | accepted () |
virtual void | done (int r) |
int | exec () |
QWidget * | extension () const |
void | finished (int result) |
bool | isSizeGripEnabled () const |
void | open () |
Qt::Orientation | orientation () const |
virtual void | reject () |
void | rejected () |
int | result () const |
void | setExtension (QWidget *extension) |
void | setModal (bool modal) |
void | setOrientation (Qt::Orientation orientation) |
void | setResult (int i) |
void | setSizeGripEnabled (bool) |
virtual void | setVisible (bool visible) |
void | showExtension (bool showIt) |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Static Public Member Functions | |
static bool | avoidArea (QWidget *widget, const QRect &area, int screen=-1) |
static void | centerOnScreen (QWidget *widget, int screen=-1) |
static int | groupSpacingHint () |
static QString | makeStandardCaption (const QString &userCaption, QWidget *window=0, CaptionFlags flags=HIGCompliantCaption) |
static int | marginHint () |
static void | resizeLayout (QWidget *widget, int margin, int spacing) |
static void | resizeLayout (QLayout *lay, int margin, int spacing) |
static void | setAllowEmbeddingInGraphicsView (bool allowEmbedding) |
static int | spacingHint () |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
Protected Slots | |
virtual void | slotButtonClicked (int button) |
void | updateGeometry () |
Protected Member Functions | |
KDialog (KDialogPrivate &dd, QWidget *parent, Qt::WindowFlags flags=0) | |
virtual void | closeEvent (QCloseEvent *e) |
virtual void | hideEvent (QHideEvent *) |
virtual void | keyPressEvent (QKeyEvent *) |
![]() | |
virtual void | contextMenuEvent (QContextMenuEvent *e) |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *o, QEvent *e) |
virtual void | resizeEvent (QResizeEvent *) |
virtual void | showEvent (QShowEvent *event) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | changeEvent (QEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEvent *event) |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual void | paintEvent (QPaintEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual void | wheelEvent (QWheelEvent *event) |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QPaintDevice () | |
Protected Attributes | |
KDialogPrivate *const | d_ptr |
Additional Inherited Members | |
![]() | |
typedef | RenderFlags |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
modal | |
sizeGripEnabled | |
![]() | |
acceptDrops | |
accessibleDescription | |
accessibleName | |
autoFillBackground | |
baseSize | |
childrenRect | |
childrenRegion | |
contextMenuPolicy | |
cursor | |
enabled | |
focus | |
focusPolicy | |
font | |
frameGeometry | |
frameSize | |
fullScreen | |
geometry | |
height | |
inputMethodHints | |
isActiveWindow | |
layoutDirection | |
locale | |
maximized | |
maximumHeight | |
maximumSize | |
maximumWidth | |
minimized | |
minimumHeight | |
minimumSize | |
minimumSizeHint | |
minimumWidth | |
modal | |
mouseTracking | |
normalGeometry | |
palette | |
pos | |
rect | |
size | |
sizeHint | |
sizeIncrement | |
sizePolicy | |
statusTip | |
styleSheet | |
toolTip | |
updatesEnabled | |
visible | |
whatsThis | |
width | |
windowFilePath | |
windowFlags | |
windowIcon | |
windowIconText | |
windowModality | |
windowModified | |
windowOpacity | |
windowTitle | |
x | |
y | |
![]() | |
objectName | |
Detailed Description
A dialog base class with standard buttons and predefined layouts.
Provides basic functionality needed by nearly all dialogs.
It offers the standard action buttons you'd expect to find in a dialog as well as the capability to define at most three configurable buttons. You can define a main widget that contains your specific dialog layout
The class takes care of the geometry management. You only need to define a minimum size for the widget you want to use as the main widget.
By default, the dialog is non-modal.
Standard buttons (action buttons):
You select which buttons should be displayed, but you do not choose the order in which they are displayed. This ensures a standard interface in KDE. The button order can be changed, but this ability is only available for a central KDE control tool. The following buttons are available: OK, Cancel/Close, Apply/Try, Default, Help and three user definable buttons: User1, User2 and User3. You must specify the text of the UserN buttons. Each button emit a signal, so you can choose to connect that signal.
The default action of the Help button will open the help system if you have provided a path to the help text. The default action of Ok and Cancel will run QDialog::accept() and QDialog::reject(), which you can override by reimplementing slotButtonClicked(). The default action of the Close button will close the dialog.
Note that the KDialog will animate a button press when the user presses Escape. The button that is enabled is either Cancel, Close or the button that is defined by setEscapeButton(). Your custom dialog code should reimplement the keyPressEvent and animate the cancel button so that the dialog behaves like regular dialogs.
Layout:
The dialog consists of a help area on top (becomes visible if you define a help path and use enableLinkedHelp()), the main area which is the built-in dialog face or your own widget in the middle and by default a button box at the bottom. The button box can also be placed at the right edge (to the right of the main widget). Use setButtonsOrientation() to control this behavior. A separator can be placed above the button box (or to the left when the button box is at the right edge).
Standard compliance:
The marginHint() and spacingHint() sizes shall be used whenever you lay out the interior of a dialog. One special note. If you make your own action buttons (OK, Cancel etc), the space between the buttons shall be spacingHint(), whereas the space above, below, to the right and to the left shall be marginHint(). If you add a separator line above the buttons, there shall be a marginHint() between the buttons and the separator and a marginHint() above the separator as well.
Example:
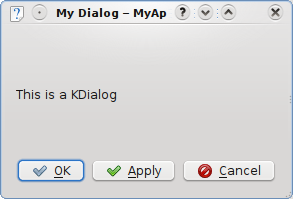
This class can be used in many ways. Note that most KDE ui widgets and many of KDE core applications use the KDialog so for more inspiration you should study the code for these.
- See also
- KPageDialog
Member Enumeration Documentation
enum KDialog::ButtonCode |
Enumerator | |
---|---|
None | |
Help |
Show Help button. (this button will run the help set with setHelp) |
Default |
Show Default button. |
Ok |
Show Ok button. (this button accept()s the dialog; result set to QDialog::Accepted) |
Apply |
Show Apply button. |
Try |
Show Try button. |
Cancel |
Show Cancel-button. (this button reject()s the dialog; result set to QDialog::Rejected) |
Close |
Show Close-button. (this button closes the dialog) |
No |
Show No button. (this button closes the dialog and sets the result to KDialog::No) |
Yes |
Show Yes button. (this button closes the dialog and sets the result to KDialog::Yes) |
Reset |
Show Reset button. |
Details |
Show Details button. (this button will show the detail widget set with setDetailsWidget) |
User1 |
Show User defined button 1. |
User2 |
Show User defined button 2. |
User3 |
Show User defined button 3. |
NoDefault |
Used when specifying a default button; indicates that no button should be marked by default. |
enum KDialog::CaptionFlag |
Constructor & Destructor Documentation
|
explicit |
Creates a dialog.
- Parameters
-
parent The parent of the dialog. flags The widget flags passed to the QDialog constructor
Definition at line 189 of file kdialog.cpp.
KDialog::~KDialog | ( | ) |
Destroys the dialog.
Definition at line 201 of file kdialog.cpp.
|
protected |
Definition at line 195 of file kdialog.cpp.
Member Function Documentation
|
signal |
The detailsWidget is about to get shown.
This is your last chance to call setDetailsWidget if you haven't done so yet.
|
signal |
The Apply button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
Places widget
so that it doesn't cover a certain area
of the screen.
This is typically used by the "find dialog" so that the match it finds can be read. For screen
, see centerOnScreen
- Returns
- true on success (widget doesn't cover area anymore, or never did), false on failure (not enough space found)
Definition at line 572 of file kdialog.cpp.
KPushButton * KDialog::button | ( | ButtonCode | id | ) | const |
Returns the button that corresponds to the id
.
Normally you should not use this function. Never delete the object returned by this function. See also enableButton(), showButton(), setButtonGuiItem().
- Parameters
-
id Identifier of the button.
- Returns
- The button or 0 if the button does not exist.
Definition at line 655 of file kdialog.cpp.
|
signal |
A button has been pressed.
This signal is only emitted if slotButtonClicked() is not replaced
- Parameters
-
button is the code of the pressed button.
KIcon KDialog::buttonIcon | ( | ButtonCode | id | ) | const |
Returns the icon of any button.
Definition at line 749 of file kdialog.cpp.
QString KDialog::buttonText | ( | ButtonCode | id | ) | const |
Returns the text of any button.
Definition at line 733 of file kdialog.cpp.
QString KDialog::buttonToolTip | ( | ButtonCode | id | ) | const |
Returns the tooltip of any button.
Definition at line 769 of file kdialog.cpp.
QString KDialog::buttonWhatsThis | ( | ButtonCode | id | ) | const |
Returns the "What's this?" text of any button.
Definition at line 789 of file kdialog.cpp.
|
signal |
The Cancel button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
|
static |
Centers widget
on the desktop, taking multi-head setups into account.
If screen
is -1, widget
will be centered on its current screen (if it was shown already) or on the primary screen. If screen
is -3, widget
will be centered on the screen that currently contains the mouse pointer. screen
will be ignored if a merged display (like Xinerama) is not in use, or merged display placement is not enabled in kdeglobals.
Definition at line 554 of file kdialog.cpp.
|
signal |
The Close button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
|
protectedvirtual |
Detects when a dialog is being closed from the window manager controls.
If the Cancel or Close button is present then the button is activated. Otherwise standard QDialog behavior will take place.
Reimplemented from QDialog.
Reimplemented in KBugReport.
Definition at line 1001 of file kdialog.cpp.
KDialog::ButtonCode KDialog::defaultButton | ( | ) | const |
Returns the button code of the default button, or NoDefault if there is no default button.
Definition at line 324 of file kdialog.cpp.
|
signal |
The Default button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
|
slot |
Destruct the dialog delayed.
You can call this function from slots like closeClicked() and hidden(). You should not use the dialog any more after calling this function.
Definition at line 868 of file kdialog.cpp.
|
slot |
Enable or disable (gray out) a general action button.
- Parameters
-
id Button identifier. state true
enables the button(s).
Definition at line 661 of file kdialog.cpp.
|
slot |
Enable or disable (gray out) the Apply button.
- Parameters
-
state true enables the button.
Definition at line 682 of file kdialog.cpp.
|
slot |
Enable or disable (gray out) the Cancel button.
- Parameters
-
state true enables the button.
Definition at line 687 of file kdialog.cpp.
|
slot |
Enable or disable (gray out) the OK button.
- Parameters
-
state true
enables the button.
Definition at line 677 of file kdialog.cpp.
|
slot |
Display or hide the help link area on the top of the dialog.
- Parameters
-
state true
will display the area.
- See also
- helpLinkText()
- setHelpLinkText()
- setHelp()
Definition at line 941 of file kdialog.cpp.
|
signal |
The dialog has finished.
A dialog emits finished after a user clicks a button that ends the dialog.
This signal is also emitted when you call hide()
If you have stored a pointer to the dialog do not try to delete the pointer in the slot that is connected to this signal.
You should use deleteLater() instead.
|
static |
Returns the number of pixels that should be used to visually separate groups of related options in a dialog according to the KDE standard.
- Since
- 4.2
Definition at line 437 of file kdialog.cpp.
|
signal |
The Help button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
QString KDialog::helpLinkText | ( | ) | const |
Returns the help link text.
If no text has been defined, "Get help..." (internationalized) is returned.
- Returns
- The help link text.
Definition at line 983 of file kdialog.cpp.
|
signal |
The dialog is about to be hidden.
A dialog is hidden after a user clicks a button that ends the dialog or when the user switches to another desktop or minimizes the dialog.
|
protectedvirtual |
Emits the hidden signal.
You can connect to that signal to detect when a dialog has been closed.
Reimplemented from QWidget.
Reimplemented in KEditToolBar.
Definition at line 993 of file kdialog.cpp.
void KDialog::incrementInitialSize | ( | const QSize & | size | ) |
Convenience method.
Add a size to the default minimum size of a dialog.
This method should only be called right before show() or exec().
- Parameters
-
size Size added to minimum size.
Definition at line 649 of file kdialog.cpp.
bool KDialog::isButtonEnabled | ( | ButtonCode | id | ) | const |
Returns whether any button is enabled.
Definition at line 668 of file kdialog.cpp.
|
slot |
Returns the status of the Details button.
Definition at line 824 of file kdialog.cpp.
|
protectedvirtual |
Reimplemented from QDialog.
Reimplemented in KColorDialog.
Definition at line 384 of file kdialog.cpp.
|
signal |
Emitted when the margin size and/or spacing size have changed.
Use marginHint() and spacingHint() in your slot to get the new values.
- Deprecated:
- This signal is not emitted. Listen to QEvent::StyleChange events instead.
QWidget * KDialog::mainWidget | ( | ) |
- Returns
- The current main widget. Will create a QWidget as the mainWidget if none was set before. This way you can write when using designer.ui.setupUi(mainWidget());
Definition at line 351 of file kdialog.cpp.
|
static |
Builds a caption that contains the application name along with the userCaption using a standard layout.
To make a compliant caption for your window, simply do: setWindowTitle(KDialog::makeStandardCaption(yourCaption))
;
To ensure that the caption is appropriate to the desktop in which the application is running, pass in a pointer to the window the caption will be applied to.
If using a KDialog or KMainWindow subclass, call setCaption instead and an appropraite standard caption will be created for you
- Parameters
-
userCaption The caption string you want to display in the window caption area. Do not include the application name! window a pointer to the window this application will apply to flags
- Returns
- the created caption
Definition at line 442 of file kdialog.cpp.
|
static |
Returns the number of pixels that should be used between a dialog edge and the outermost widget(s) according to the KDE standard.
- Deprecated:
- Use the style's pixelMetric() function to query individual margins. Different platforms may use different values for the four margins.
Definition at line 427 of file kdialog.cpp.
|
virtual |
|
signal |
The No button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
|
signal |
The OK button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
|
signal |
The Reset button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
|
static |
Resize every layout manager used in widget
and its nested children.
- Parameters
-
widget The widget used. margin The new layout margin. spacing The new layout spacing.
- Deprecated:
- Use QLayout functions where necessary. Setting margin and spacing values recursively for all children prevents QLayout from creating platform native layouts.
Definition at line 499 of file kdialog.cpp.
|
static |
Resize every layout associated with lay
and its children.
- Parameters
-
lay layout to be resized margin The new layout margin spacing The new layout spacing
- Deprecated:
- Use QLayout functions where necessary. Setting margin and spacing values recursively for all children prevents QLayout from creating platform native layouts.
Definition at line 513 of file kdialog.cpp.
void KDialog::restoreDialogSize | ( | const KConfigGroup & | config | ) |
Restores the dialog's size from the configuration according to the screen size.
- Note
- the group must be set before calling
- Parameters
-
config The config group to read from.
Definition at line 1018 of file kdialog.cpp.
void KDialog::saveDialogSize | ( | KConfigGroup & | config, |
KConfigGroup::WriteConfigFlags | options = KConfigGroup::Normal |
||
) | const |
Saves the dialog's size dependent on the screen dimension either to the global or application config file.
- Note
- the group must be set before calling
- Parameters
-
config The config group to read from. options passed to KConfigGroup::writeEntry()
Definition at line 1033 of file kdialog.cpp.
|
static |
Allow embedding the dialogs based on KDialog into a graphics view.
By default embedding is not allowed, dialogs will appear as separate windows.
- Since
- 4.6
Definition at line 1044 of file kdialog.cpp.
void KDialog::setButtonFocus | ( | ButtonCode | id | ) |
Sets the focus to the button of the passed id
.
Definition at line 798 of file kdialog.cpp.
void KDialog::setButtonGuiItem | ( | ButtonCode | id, |
const KGuiItem & | item | ||
) |
Sets the KGuiItem directly for the button instead of using 3 methods to set the text, tooltip and whatsthis strings.
This also allows to set an icon for the button which is otherwise not possible for the extra buttons beside Ok, Cancel and Apply.
- Parameters
-
id The button identifier. item The KGuiItem for the button.
Definition at line 699 of file kdialog.cpp.
void KDialog::setButtonIcon | ( | ButtonCode | id, |
const KIcon & | icon | ||
) |
Sets the icon of any button.
- Parameters
-
id The button identifier. icon Button icon.
Definition at line 742 of file kdialog.cpp.
void KDialog::setButtonMenu | ( | ButtonCode | id, |
QMenu * | menu, | ||
ButtonPopupMode | popupmode = InstantPopup |
||
) |
Sets the menu of any button.
- Parameters
-
id The button identifier. menu The menu. popupmode Choose if KPushButton setMenu or setDelayedMenu is used
Definition at line 708 of file kdialog.cpp.
void KDialog::setButtons | ( | ButtonCodes | buttonMask | ) |
Creates (or recreates) the button box and all the buttons in it.
Note that some combinations are not possible. That means, you can't have the following pairs of buttons in a dialog:
- Default and Details
- Cancel and Close
- Ok and Try
This will reset all default KGuiItem of all button.
- Parameters
-
buttonMask Specifies what buttons will be made.
Definition at line 206 of file kdialog.cpp.
void KDialog::setButtonsOrientation | ( | Qt::Orientation | orientation | ) |
Sets the orientation of the button box.
It can be Vertical
or Horizontal
. If Horizontal
(default), the button box is positioned at the bottom of the dialog. If Vertical
it will be placed at the right edge of the dialog.
- Parameters
-
orientation The button box orientation.
Definition at line 268 of file kdialog.cpp.
void KDialog::setButtonText | ( | ButtonCode | id, |
const QString & | text | ||
) |
Sets the text of any button.
- Parameters
-
id The button identifier. text Button text.
Definition at line 719 of file kdialog.cpp.
void KDialog::setButtonToolTip | ( | ButtonCode | id, |
const QString & | text | ||
) |
Sets the tooltip text of any button.
- Parameters
-
id The button identifier. text Button text.
Definition at line 758 of file kdialog.cpp.
void KDialog::setButtonWhatsThis | ( | ButtonCode | id, |
const QString & | text | ||
) |
Sets the "What's this?" text of any button.
- Parameters
-
id The button identifier. text Button text.
Definition at line 778 of file kdialog.cpp.
|
virtualslot |
Make a KDE compliant caption.
- Parameters
-
caption Your caption. Do not
include the application name in this string. It will be added automatically according to the KDE standard.
Definition at line 469 of file kdialog.cpp.
Makes a KDE compliant caption.
- Parameters
-
caption Your caption. Do not include the application name in this string. It will be added automatically according to the KDE standard. modified Specify whether the document is modified. This displays an additional sign in the title bar, usually "**".
Definition at line 475 of file kdialog.cpp.
void KDialog::setDefaultButton | ( | ButtonCode | id | ) |
Sets the button that will be activated when the Enter key is pressed.
By default, this is the Ok button if it is present
- Parameters
-
id The button code.
Definition at line 287 of file kdialog.cpp.
|
slot |
Sets the widget that gets shown when "Details" is enabled.
The dialog takes over ownership of the widget. Any previously set widget gets deleted.
Definition at line 806 of file kdialog.cpp.
|
slot |
Sets the status of the Details button.
Definition at line 829 of file kdialog.cpp.
void KDialog::setEscapeButton | ( | ButtonCode | id | ) |
Sets the button that will be activated when the Escape key is pressed.
By default, the Escape key is mapped to either the Cancel or the Close button if one of these buttons are defined. The user expects that Escape will cancel an operation so use this function with caution.
- Parameters
-
id The button code.
Definition at line 282 of file kdialog.cpp.
Sets the help path and topic.
- Parameters
-
anchor Defined anchor in your docbook sources appname Defines the appname the help belongs to If empty it's the current one
- Note
- The help button works differently for the class KCMultiDialog, so it does not make sense to call this function for Dialogs of that type. See KCMultiDialog::slotHelp() for more information.
Definition at line 967 of file kdialog.cpp.
|
slot |
Sets the text that is shown as the linked text.
If text is empty, the text "Get help..." (internationalized) is used instead.
- Parameters
-
text The link text.
Definition at line 975 of file kdialog.cpp.
void KDialog::setInitialSize | ( | const QSize & | size | ) |
Convenience method.
Sets the initial dialog size.
This method should only be called right before show() or exec(). The initial size will be ignored if smaller than the dialog's minimum size.
- Parameters
-
size Startup size.
Definition at line 643 of file kdialog.cpp.
void KDialog::setMainWidget | ( | QWidget * | widget | ) |
Sets the main widget of the dialog.
Definition at line 338 of file kdialog.cpp.
|
virtualslot |
Make a plain caption without any modifications.
- Parameters
-
caption Your caption. This is the string that will be displayed in the window title.
Definition at line 488 of file kdialog.cpp.
void KDialog::showButton | ( | ButtonCode | id, |
bool | state | ||
) |
Hide or display a general action button.
Only buttons that have been created in the constructor can be displayed. This method will not create a new button.
- Parameters
-
id Button identifier. state true display the button(s).
Definition at line 692 of file kdialog.cpp.
void KDialog::showButtonSeparator | ( | bool | state | ) |
Hide or display the separator line drawn between the action buttons an the main widget.
Definition at line 624 of file kdialog.cpp.
|
virtual |
Reimplemented from QDialog.
Reimplemented from QDialog.
Reimplemented in KShortcutsDialog.
Definition at line 359 of file kdialog.cpp.
|
protectedvirtualslot |
Activated when the button button
is clicked.
Sample that shows how to catch and handle button clicks within an own dialog;
- Parameters
-
button is the type KDialog::ButtonCode
Definition at line 877 of file kdialog.cpp.
|
static |
Returns the number of pixels that should be used between widgets inside a dialog according to the KDE standard.
- Deprecated:
- Use the style's layoutSpacing() function to query individual spacings. Different platforms may use different values depending on widget types and pairs.
Definition at line 432 of file kdialog.cpp.
|
signal |
The Try button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
|
protectedslot |
Updates the margins and spacings.
- Deprecated:
- KDialog respects the style's margins and spacings automatically. Calling this function has no effect.
Definition at line 989 of file kdialog.cpp.
|
signal |
The User1 button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
|
signal |
The User2 button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
|
signal |
The User3 button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
|
signal |
The Yes button was pressed.
This signal is only emitted if slotButtonClicked() is not replaced
Member Data Documentation
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.