KDEUI
#include <kmessagebox.h>
Public Types | |
enum | ButtonCode { Ok = 1, Cancel = 2, Yes = 3, No = 4, Continue = 5 } |
enum | DialogType { QuestionYesNo = 1, WarningYesNo = 2, WarningContinueCancel = 3, WarningYesNoCancel = 4, Information = 5, Sorry = 7, Error = 8, QuestionYesNoCancel = 9 } |
enum | Option { Notify = 1, AllowLink = 2, Dangerous = 4, PlainCaption = 8, NoExec = 16, WindowModal = 32 } |
Static Public Member Functions | |
static void | about (QWidget *parent, const QString &text, const QString &caption=QString(), Options options=Notify) |
static int | createKMessageBox (KDialog *dialog, QMessageBox::Icon icon, const QString &text, const QStringList &strlist, const QString &ask, bool *checkboxReturn, Options options, const QString &details=QString()) |
static int | createKMessageBox (KDialog *dialog, const QIcon &icon, const QString &text, const QStringList &strlist, const QString &ask, bool *checkboxReturn, Options options, const QString &details=QString(), QMessageBox::Icon notifyType=QMessageBox::Information) |
static void | detailedError (QWidget *parent, const QString &text, const QString &details, const QString &caption=QString(), Options options=Notify) |
static void | detailedErrorWId (WId parent_id, const QString &text, const QString &details, const QString &caption=QString(), Options options=Notify) |
static void | detailedSorry (QWidget *parent, const QString &text, const QString &details, const QString &caption=QString(), Options options=Notify) |
static void | detailedSorryWId (WId parent_id, const QString &text, const QString &details, const QString &caption=QString(), Options options=Notify) |
static void | enableAllMessages () |
static void | enableMessage (const QString &dontShowAgainName) |
static void | error (QWidget *parent, const QString &text, const QString &caption=QString(), Options options=Notify) |
static void | errorList (QWidget *parent, const QString &text, const QStringList &strlist, const QString &caption=QString(), Options options=Notify) |
static void | errorListWId (WId parent_id, const QString &text, const QStringList &strlist, const QString &caption=QString(), Options options=Notify) |
static void | errorWId (WId parent_id, const QString &text, const QString &caption=QString(), Options options=Notify) |
static void | information (QWidget *parent, const QString &text, const QString &caption=QString(), const QString &dontShowAgainName=QString(), Options options=Notify) |
static void | informationList (QWidget *parent, const QString &text, const QStringList &strlist, const QString &caption=QString(), const QString &dontShowAgainName=QString(), Options options=Notify) |
static void | informationListWId (WId parent_id, const QString &text, const QStringList &strlist, const QString &caption=QString(), const QString &dontShowAgainName=QString(), Options options=Notify) |
static void | informationWId (WId parent_id, const QString &text, const QString &caption=QString(), const QString &dontShowAgainName=QString(), Options options=Notify) |
static int | messageBox (QWidget *parent, DialogType type, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontShowAskAgainName=QString(), Options options=Notify) |
static int | messageBoxWId (WId parent_id, DialogType type, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontShowAskAgainName=QString(), Options options=Notify) |
static int | questionYesNo (QWidget *parent, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | questionYesNoCancel (QWidget *parent, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | questionYesNoCancelWId (WId parent_id, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | questionYesNoList (QWidget *parent, const QString &text, const QStringList &strlist, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | questionYesNoListWId (WId parent_id, const QString &text, const QStringList &strlist, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | questionYesNoWId (WId parent_id, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static void | queuedDetailedError (QWidget *parent, const QString &text, const QString &details, const QString &caption=QString()) |
static void | queuedDetailedErrorWId (WId parent_id, const QString &text, const QString &details, const QString &caption=QString()) |
static void | queuedMessageBox (QWidget *parent, DialogType type, const QString &text, const QString &caption, Options options) |
static void | queuedMessageBox (QWidget *parent, DialogType type, const QString &text, const QString &caption=QString()) |
static void | queuedMessageBoxWId (WId parent_id, DialogType type, const QString &text, const QString &caption, Options options) |
static void | queuedMessageBoxWId (WId parent_id, DialogType type, const QString &text, const QString &caption=QString()) |
static void | saveDontShowAgainContinue (const QString &dontShowAgainName) |
static void | saveDontShowAgainYesNo (const QString &dontShowAgainName, ButtonCode result) |
static void | setDontShowAskAgainConfig (KConfig *cfg) |
static bool | shouldBeShownContinue (const QString &dontShowAgainName) |
static bool | shouldBeShownYesNo (const QString &dontShowAgainName, ButtonCode &result) |
static void | sorry (QWidget *parent, const QString &text, const QString &caption=QString(), Options options=Notify) |
static void | sorryWId (WId parent_id, const QString &text, const QString &caption=QString(), Options options=Notify) |
static int | warningContinueCancel (QWidget *parent, const QString &text, const QString &caption=QString(), const KGuiItem &buttonContinue=KStandardGuiItem::cont(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | warningContinueCancelList (QWidget *parent, const QString &text, const QStringList &strlist, const QString &caption=QString(), const KGuiItem &buttonContinue=KStandardGuiItem::cont(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | warningContinueCancelListWId (WId parent_id, const QString &text, const QStringList &strlist, const QString &caption=QString(), const KGuiItem &buttonContinue=KStandardGuiItem::cont(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | warningContinueCancelWId (WId parent_id, const QString &text, const QString &caption=QString(), const KGuiItem &buttonContinue=KStandardGuiItem::cont(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | warningYesNo (QWidget *parent, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const QString &dontAskAgainName=QString(), Options options=Options(Notify|Dangerous)) |
static int | warningYesNoCancel (QWidget *parent, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | warningYesNoCancelList (QWidget *parent, const QString &text, const QStringList &strlist, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | warningYesNoCancelListWId (WId parent_id, const QString &text, const QStringList &strlist, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | warningYesNoCancelWId (WId parent_id, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontAskAgainName=QString(), Options options=Notify) |
static int | warningYesNoList (QWidget *parent, const QString &text, const QStringList &strlist, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const QString &dontAskAgainName=QString(), Options options=Options(Notify|Dangerous)) |
static int | warningYesNoListWId (WId parent_id, const QString &text, const QStringList &strlist, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const QString &dontAskAgainName=QString(), Options options=Options(Notify|Dangerous)) |
static int | warningYesNoWId (WId parent_id, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const QString &dontAskAgainName=QString(), Options options=Options(Notify|Dangerous)) |
Detailed Description
Easy message dialog box.
Provides convenience functions for some i18n'ed standard dialogs, as well as audible notification via KNotification
The text in message boxes is wrapped automatically. The text may either be plaintext or richtext. If the text is plaintext, a newline-character may be used to indicate the end of a paragraph.
- KDE 4 Porting Guide:
- Where applicable, the static functions now take an additional argument to specify the cancel button. Since a default argument is provided, this will affect your code only, if you specified dontAskAgainName and/or options. In those cases, adding an additional parameter KStandardGuiItem::cancel() leads to the old behavior. The following functions are affected (omitting function arguments in the list): questionYesNoCancel(), questionYesNoCancelWId(), warningContinueCancel(), warningContinueCancelWId(), warningContinueCancelList(), warningContinueCancelListWId(), warningYesNoCancel(), warningYesNoCancelWId(), warningYesNoCancelList(), warningYesNoCancelListWId(), messageBox(), messageBoxWId().
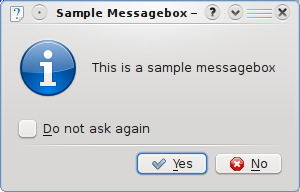
Definition at line 62 of file kmessagebox.h.
Member Enumeration Documentation
Enumerator | |
---|---|
QuestionYesNo | |
WarningYesNo | |
WarningContinueCancel | |
WarningYesNoCancel | |
Information | |
Sorry | |
Error | |
QuestionYesNoCancel |
Definition at line 77 of file kmessagebox.h.
enum KMessageBox::Option |
Enumerator | |
---|---|
Notify |
Emit a KNotify event. |
AllowLink |
The message may contain links. |
Dangerous |
The action to be confirmed by the dialog is a potentially destructive one. The default button will be set to Cancel or No, depending on which is available. |
PlainCaption |
Do not use KApplication::makeStdCaption() |
NoExec |
Do not call exec() in createKMessageBox() |
WindowModal |
The window is to be modal relative to its parent. By default, it is application modal. |
Definition at line 90 of file kmessagebox.h.
Member Function Documentation
|
static |
Display an "About" dialog.
- Parameters
-
parent Parent widget. text Message string. caption Message box title. The application name is added to the title. The default title is i18n("About \<appname\>"). options see Options
Your program wants to show some general information about the application like the authors's names and email addresses.
The default button is "&OK".
NOTE: The ok button will always have the i18n'ed text '&OK'.
Definition at line 1044 of file kmessagebox.cpp.
|
static |
Create content and layout of a standard dialog.
- Parameters
-
dialog The parent dialog base icon Which predefined icon the message box shall show. text Message string. strlist List of strings to be written in the listbox. If the list is empty, it doesn't show any listbox ask The text of the checkbox. If empty none will be shown. checkboxReturn The result of the checkbox. If it's initially true then the checkbox will be checked by default. May be 0. options see Options details Detailed message string.
- Returns
- A KDialog button code, not a KMessageBox button code, based on the buttonmask given to the constructor of the
dialog
(ie. will return KDialog::Yes [256] instead of KMessageBox::Yes [3]). Will return KMessageBox::Cancel if the message box is queued for display instead of exec()ed immediately or if the option NoExec is set.
- Note
- Unless NoExec is used, the
dialog
that is passed in is deleted by this function. Do not delete it yourself.
Definition at line 153 of file kmessagebox.cpp.
|
static |
Create content and layout of a standard dialog.
- Parameters
-
dialog The parent dialog base icon A QPixmap containing the icon to be displayed in the dialog next to the text. text Message string. strlist List of strings to be written in the listbox. If the list is empty, it doesn't show any listbox ask The text of the checkbox. If empty none will be shown. checkboxReturn The result of the checkbox. If it's initially true then the checkbox will be checked by default. May be 0. options see Options details Detailed message string. notifyType The type of notification to send when this message is presentend.
- Returns
- A KDialog button code, not a KMessageBox button code, based on the buttonmask given to the constructor of the
dialog
(ie. will return KDialog::Yes [256] instead of KMessageBox::Yes [3]). Will return KMessageBox::Cancel if the message box is queued for display instead of exec()ed immediately or if the option NoExec is set.
- Note
- Unless NoExec is used, the
dialog
that is passed in is deleted by this function. Do not delete it yourself.
Definition at line 163 of file kmessagebox.cpp.
|
static |
Displays an "Error" dialog with a "Details >>" button.
- Parameters
-
parent Parent widget. text Message string. details Detailed message string. caption Message box title. The application name is added to the title. The default title is i18n("Error"). options see Options
Your program messed up and now it's time to inform the user. To be used for important things like "Sorry, I deleted your hard disk."
The details
message can contain additional information about the problem and can be shown on request to advanced/interested users.
If your program detects the action specified by the user is somehow not allowed, this should never be reported with error(). Use sorry() instead to explain to the user that this action is not allowed.
The default button is "&OK". Pressing "Esc" selects the OK-button.
NOTE: The OK button will always have the i18n'ed text '&OK'.
Definition at line 858 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 865 of file kmessagebox.cpp.
|
static |
Displays a "Sorry" dialog with a "Details >>" button.
- Parameters
-
parent Parent widget. text Message string. details Detailed message string. caption Message box title. The application name is added to the title. The default title is i18n("Sorry"). options see Options
Either your program messed up and asks for understanding or your user did something stupid.
To be used for small problems like "Sorry, I can't find the file you specified."
And then details
can contain something like "foobar.txt was not found in any of the following directories: /usr/bin,/usr/local/bin,/usr/sbin"
The default button is "&OK". Pressing "Esc" selects the OK-button.
NOTE: The ok button will always have the i18n'ed text '&OK'.
Definition at line 931 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 938 of file kmessagebox.cpp.
|
static |
Enable all messages which have been turned off with the dontShowAgainName
feature.
Definition at line 1012 of file kmessagebox.cpp.
|
static |
Re-enable a specific dontShowAgainName
messages that had previously been turned off.
Definition at line 1031 of file kmessagebox.cpp.
|
static |
Display an "Error" dialog.
- Parameters
-
parent Parent widget. text Message string. caption Message box title. The application name is added to the title. The default title is i18n("Error"). options see Options
Your program messed up and now it's time to inform the user. To be used for important things like "Sorry, I deleted your hard disk."
If your program detects the action specified by the user is somehow not allowed, this should never be reported with error(). Use sorry() instead to explain to the user that this action is not allowed.
The default button is "&OK". Pressing "Esc" selects the OK-button.
NOTE: The OK button will always have the i18n'ed text '&OK'.
Definition at line 818 of file kmessagebox.cpp.
|
static |
Display an "Error" dialog with a listbox.
- Parameters
-
parent Parent widget. text Message string. strlist List of strings to be written in the listbox. If the list is empty, it doesn't show any listbox, working as error(). caption Message box title. The application name is added to the title. The default title is i18n("Error"). options see Options
Your program messed up and now it's time to inform the user. To be used for important things like "Sorry, I deleted your hard disk."
If your program detects the action specified by the user is somehow not allowed, this should never be reported with error(). Use sorry() instead to explain to the user that this action is not allowed.
The default button is "&OK". Pressing "Esc" selects the OK-button.
NOTE: The OK button will always have the i18n'ed text '&OK'.
Definition at line 830 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 836 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 824 of file kmessagebox.cpp.
|
static |
Display an "Information" dialog.
- Parameters
-
parent Parent widget. text Message string. caption Message box title. The application name is added to the title. The default title is i18n("Information"). dontShowAgainName If provided, a checkbox is added with which further notifications can be turned off. The string is used to lookup and store the setting in the applications config file. The setting is stored in the "Notification Messages" group. options see Options
Your program wants to tell the user something. To be used for things like: "Your bookmarks have been rearranged."
The default button is "&OK". Pressing "Esc" selects the OK-button.
NOTE: The OK button will always have the i18n'ed text '&OK'.
Definition at line 960 of file kmessagebox.cpp.
|
static |
Display an "Information" dialog with a listbox.
- Parameters
-
parent Parent widget. text Message string. strlist List of strings to be written in the listbox. If the list is empty, it doesn't show any listbox, working as information. caption Message box title. The application name is added to the title. The default title is i18n("Information"). dontShowAgainName If provided, a checkbox is added with which further notifications can be turned off. The string is used to lookup and store the setting in the applications config file. The setting is stored in the "Notification Messages" group. options see Options
Your program wants to tell the user something. To be used for things like: "The following bookmarks have been rearranged:"
The default button is "&OK". Pressing "Esc" selects the OK-button.
NOTE: The OK button will always have the i18n'ed text '&OK'.
Definition at line 972 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 979 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 966 of file kmessagebox.cpp.
|
static |
Alternate method to show a messagebox:
- Parameters
-
parent Parent widget. type type of message box: QuestionYesNo, WarningYesNo, WarningContinueCancel... text Message string. caption Message box title. buttonYes The text for the first button. The default is KStandardGuiItem::yes(). buttonNo The text for the second button. The default is KStandardGuiItem::no(). buttonCancel The text for the third button. The default is KStandardGuiItem::cancel(). dontShowAskAgainName If provided, a checkbox is added with which further questions/information can be turned off. If turned off all questions will be automatically answered with the last answer (either Yes or No), if the message box needs an answer. The string is used to lookup and store the setting in the applications config file. options see Options Note: for ContinueCancel, buttonYes is the continue button and buttonNo is unused. and for Information, none is used.
- Returns
- a button code, as defined in KMessageBox.
Definition at line 1068 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 1077 of file kmessagebox.cpp.
|
static |
Display a simple "question" dialog.
- Parameters
-
parent Parent widget. text Message string. caption Message box title. The application name is added to the title. The default title is i18n("Question"). buttonYes The text for the first button. The default is KStandardGuiItem::yes(). buttonNo The text for the second button. The default is KStandardGuiItem::no(). dontAskAgainName If provided, a checkbox is added with which further confirmation can be turned off. The string is used to lookup and store the setting in the applications config file. The setting is stored in the "Notification Messages" group. If dontAskAgainName
starts with a ':' then the setting is stored in the global config file.options see Option
- Returns
- 'Yes' is returned if the Yes-button is pressed. 'No' is returned if the No-button is pressed.
To be used for questions like "Do you have a printer?"
The default button is "Yes". Pressing "Esc" selects "No".
Definition at line 353 of file kmessagebox.cpp.
|
static |
Display a simple "question" dialog.
- Parameters
-
parent Parent widget. text Message string. caption Message box title. The application name is added to the title. The default title is i18n("Question"). buttonYes The text for the first button. The default is KStandardGuiItem::yes(). buttonNo The text for the second button. The default is KStandardGuiItem::no(). buttonCancel The text for the third button. The default is KStandardGuiItem::cancel(). dontAskAgainName If provided, a checkbox is added with which further confirmation can be turned off. The string is used to lookup and store the setting in the applications config file. The setting is stored in the "Notification Messages" group. If dontAskAgainName
starts with a ':' then the setting is stored in the global config file.options see Options
- Returns
- 'Yes' is returned if the Yes-button is pressed. 'No' is returned if the No-button is pressed.
To be used for questions like "Do you want to discard the message or save it for later?",
The default button is "Yes". Pressing "Esc" selects "Cancel".
Definition at line 495 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 508 of file kmessagebox.cpp.
|
static |
Display a "question" dialog with a listbox to show information to the user.
- Parameters
-
parent Parent widget. text Message string. strlist List of strings to be written in the listbox. If the list is empty, it doesn't show any listbox, working as questionYesNo. caption Message box title. The application name is added to the title. The default title is i18n("Question"). buttonYes The text for the first button. The default is KStandardGuiItem::yes(). buttonNo The text for the second button. The default is KStandardGuiItem::no(). dontAskAgainName If provided, a checkbox is added with which further confirmation can be turned off. The string is used to lookup and store the setting in the applications config file. The setting is stored in the "Notification Messages" group. If dontAskAgainName
starts with a ':' then the setting is stored in the global config file.options see Options
- Returns
- 'Yes' is returned if the Yes-button is pressed. 'No' is returned if the No-button is pressed.
To be used for questions like "Do you really want to delete these files?" And show the user exactly which files are going to be deleted in case he presses "Yes"
The default button is "Yes". Pressing "Esc" selects "No".
Definition at line 437 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 449 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 364 of file kmessagebox.cpp.
|
static |
Like detailedError.
This function will return immediately, the messagebox will be shown once the application enters an event loop and no other messagebox is being shown.
Note that if the parent gets deleted, the messagebox will not be shown.
Definition at line 887 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 894 of file kmessagebox.cpp.
|
static |
Like messageBox.
Only for message boxes of type Information, Sorry or Error.
This function will return immediately, the messagebox will be shown once the application enters an event loop and no other messagebox is being shown.
Note that if the parent gets deleted, the messagebox will not be shown.
Definition at line 1114 of file kmessagebox.cpp.
|
static |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.This is an overloaded member function, provided for convenience.
It behaves essentially like the above function.
Definition at line 1127 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 1119 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 1132 of file kmessagebox.cpp.
|
static |
Save the fact that the continue/cancel message box should not be shown again.
- Parameters
-
dontShowAgainName the name that identify the message box. If empty, this method does nothing.
Definition at line 418 of file kmessagebox.cpp.
|
static |
Save the fact that the yes/no message box should not be shown again.
- Parameters
-
dontShowAgainName the name that identify the message box. If empty, this method does nothing. result the value (Yes or No) that should be used as the result for the message box.
Definition at line 403 of file kmessagebox.cpp.
|
static |
Use cfg
for all settings related to the dontShowAgainName feature.
If cfg
is 0 (default) KGlobal::config() will be used.
Definition at line 432 of file kmessagebox.cpp.
- Returns
- true if the corresponding continue/cancel message box should be shown.
- Parameters
-
dontShowAgainName the name that identify the message box. If empty, true is always returned.
Definition at line 394 of file kmessagebox.cpp.
|
static |
- Returns
- true if the corresponding yes/no message box should be shown.
- Parameters
-
dontShowAgainName the name that identify the message box. If empty, true is always returned. result is set to the result (Yes or No) that was chosen the last time the message box was shown. Only meaningful, if the message box should not be shown.
Definition at line 375 of file kmessagebox.cpp.
|
static |
Display an "Sorry" dialog.
- Parameters
-
parent Parent widget. text Message string. caption Message box title. The application name is added to the title. The default title is i18n("Sorry"). options see OptionsType
Either your program messed up and asks for understanding or your user did something stupid.
To be used for small problems like "Sorry, I can't find the file you specified."
The default button is "&OK". Pressing "Esc" selects the OK-button.
NOTE: The ok button will always have the i18n'ed text '&OK'.
Definition at line 904 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 910 of file kmessagebox.cpp.
|
static |
Display a "warning" dialog.
- Parameters
-
parent Parent widget. text Message string. caption Message box title. The application name is added to the title. The default title is i18n("Warning"). buttonContinue The text for the first button. The default is KStandardGuiItem::cont(). buttonCancel The text for the second button. The default is KStandardGuiItem::cancel(). dontAskAgainName If provided, a checkbox is added with which further confirmation can be turned off. The string is used to lookup and store the setting in the applications config file. The setting is stored in the "Notification Messages" group. If dontAskAgainName
starts with a ':' then the setting is stored in the global config file.options see Options
- Returns
Continue
is returned if the Continue-button is pressed.Cancel
is returned if the Cancel-button is pressed.
To be used for questions like "You are about to Print. Are you sure?" the continueButton should then be labeled "Print".
The default button is buttonContinue. Pressing "Esc" selects "Cancel".
Definition at line 644 of file kmessagebox.cpp.
|
static |
Display a "warning" dialog with a listbox to show information to the user.
- Parameters
-
parent Parent widget. text Message string. strlist List of strings to be written in the listbox. If the list is empty, it doesn't show any listbox, working as warningContinueCancel. caption Message box title. The application name is added to the title. The default title is i18n("Warning"). buttonContinue The text for the first button. The default is KStandardGuiItem::cont(). buttonCancel The text for the second button. The default is KStandardGuiItem::cancel(). dontAskAgainName If provided, a checkbox is added with which further confirmation can be turned off. The string is used to lookup and store the setting in the applications config file. The setting is stored in the "Notification Messages" group. If dontAskAgainName
starts with a ':' then the setting is stored in the global config file.options see Options
- Returns
Continue
is returned if the Continue-button is pressed.Cancel
is returned if the Cancel-button is pressed.
To be used for questions like "You are about to Print. Are you sure?" the continueButton should then be labeled "Print".
The default button is buttonContinue. Pressing "Esc" selects "Cancel".
Definition at line 668 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 680 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 656 of file kmessagebox.cpp.
|
static |
Display a "warning" dialog.
- Parameters
-
parent Parent widget. text Message string. caption Message box title. The application name is added to the title. The default title is i18n("Warning"). buttonYes The text for the first button. The default is KStandardGuiItem::yes(). buttonNo The text for the second button. The default is KStandardGuiItem::no(). dontAskAgainName If provided, a checkbox is added with which further confirmation can be turned off. The string is used to lookup and store the setting in the applications config file. The setting is stored in the "Notification Messages" group. If dontAskAgainName
starts with a ':' then the setting is stored in the global config file.options see Options
- Returns
Yes
is returned if the Yes-button is pressed.No
is returned if the No-button is pressed.
To be used for questions "Shall I update your configuration?" The text should explain the implication of both options.
The default button is "No". Pressing "Esc" selects "No".
Definition at line 564 of file kmessagebox.cpp.
|
static |
Display a Yes/No/Cancel "warning" dialog.
- Parameters
-
parent Parent widget. text Message string. caption Message box title. The application name is added to the title. The default title is i18n("Warning"). buttonYes The text for the first button. The default is KStandardGuiItem::yes(). buttonNo The text for the second button. The default is KStandardGuiItem::no(). buttonCancel The text for the third button. The default is KStandardGuiItem::cancel(). dontAskAgainName If provided, a checkbox is added with which further questions can be turned off. If turned off all questions will be automatically answered with the last answer (either Yes or No). The string is used to lookup and store the setting in the applications config file. The setting is stored in the "Notification Messages" group. If dontAskAgainName
starts with a ':' then the setting is stored in the global config file.options see Options
- Returns
Yes
is returned if the Yes-button is pressed.No
is returned if the No-button is pressed.Cancel
is retunred if the Cancel- button is pressed.
To be used for questions "Do you want to save your changes?" The text should explain the implication of choosing 'No'.
The default button is "Yes". Pressing "Esc" selects "Cancel"
Definition at line 726 of file kmessagebox.cpp.
|
static |
Display a Yes/No/Cancel "warning" dialog with a listbox to show information to the user.
- Parameters
-
parent Parent widget. text Message string. strlist List of strings to be written in the listbox. If the list is empty, it doesn't show any listbox, working as warningYesNoCancel. caption Message box title. The application name is added to the title. The default title is i18n("Warning"). buttonYes The text for the first button. The default is KStandardGuiItem::yes(). buttonNo The text for the second button. The default is KStandardGuiItem::no(). buttonCancel The text for the third button. The default is KStandardGuiItem::cancel(). dontAskAgainName If provided, a checkbox is added with which further questions can be turned off. If turned off all questions will be automatically answered with the last answer (either Yes or No). The string is used to lookup and store the setting in the applications config file. The setting is stored in the "Notification Messages" group. If dontAskAgainName
starts with a ':' then the setting is stored in the global config file.options see Options
- Returns
Yes
is returned if the Yes-button is pressed.No
is returned if the No-button is pressed.Cancel
is retunred if the Cancel- button is pressed.
To be used for questions "Do you want to save your changes?" The text should explain the implication of choosing 'No'.
The default button is "Yes". Pressing "Esc" selects "Cancel"
Definition at line 750 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 763 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 738 of file kmessagebox.cpp.
|
static |
Display a "warning" dialog with a listbox to show information to the user.
- Parameters
-
parent Parent widget. text Message string. strlist List of strings to be written in the listbox. If the list is empty, it doesn't show any listbox, working as questionYesNo. caption Message box title. The application name is added to the title. The default title is i18n("Question"). buttonYes The text for the first button. The default is KStandardGuiItem::yes(). buttonNo The text for the second button. The default is KStandardGuiItem::no(). dontAskAgainName If provided, a checkbox is added with which further confirmation can be turned off. The string is used to lookup and store the setting in the applications config file. The setting is stored in the "Notification Messages" group. If dontAskAgainName
starts with a ':' then the setting is stored in the global config file.options see Options
- Returns
- 'Yes' is returned if the Yes-button is pressed. 'No' is returned if the No-button is pressed.
To be used for questions like "Do you really want to delete these files?" And show the user exactly which files are going to be deleted in case he presses "Yes"
The default button is "No". Pressing "Esc" selects "No".
Definition at line 586 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 598 of file kmessagebox.cpp.
|
static |
This function accepts the window id of the parent window, instead of QWidget*.
It should be used only when necessary.
Definition at line 575 of file kmessagebox.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.