KDEUI
#include <kselectaction.h>
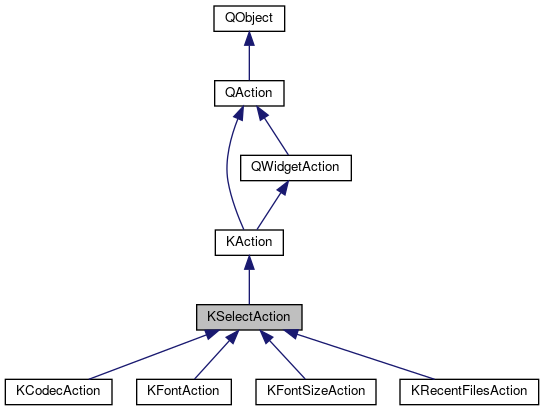
Public Types | |
enum | ToolBarMode { MenuMode, ComboBoxMode } |
![]() | |
enum | GlobalShortcutLoading { Autoloading = 0x0, NoAutoloading = 0x4 } |
enum | ShortcutType { ActiveShortcut = 0x1, DefaultShortcut = 0x2 } |
Signals | |
void | triggered (QAction *action) |
void | triggered (int index) |
void | triggered (const QString &text) |
![]() | |
void | authorized (KAuth::Action *action) |
void | globalShortcutChanged (const QKeySequence &) |
void | triggered (Qt::MouseButtons buttons, Qt::KeyboardModifiers modifiers) |
Protected Slots | |
virtual void | actionTriggered (QAction *action) |
void | slotToggled (bool) |
Protected Member Functions | |
KSelectAction (KSelectActionPrivate &dd, QObject *parent) | |
virtual QWidget * | createWidget (QWidget *parent) |
virtual void | deleteWidget (QWidget *widget) |
virtual bool | event (QEvent *event) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QList< QWidget * > | createdWidgets () const |
Protected Attributes | |
KSelectActionPrivate * | d_ptr |
Properties | |
int | comboWidth |
QAction | currentAction |
int | currentItem |
QString | currentText |
bool | editable |
QStringList | items |
ToolBarMode | toolBarMode |
QToolButton::ToolButtonPopupMode | toolButtonPopupMode |
![]() | |
KShortcut | globalShortcut |
bool | globalShortcutAllowed |
bool | globalShortcutEnabled |
KShortcut | shortcut |
bool | shortcutConfigurable |
![]() | |
autoRepeat | |
checkable | |
checked | |
enabled | |
font | |
icon | |
iconText | |
iconVisibleInMenu | |
menuRole | |
priority | |
shortcut | |
shortcutContext | |
softKeyRole | |
statusTip | |
text | |
toolTip | |
visible | |
whatsThis | |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
Action for selecting one of several items.
Action for selecting one of several items.
This action shows up a submenu with a list of items. One of them can be checked. If the user clicks on an item this item will automatically be checked, the formerly checked item becomes unchecked. There can be only one item checked at a time.
Definition at line 51 of file kselectaction.h.
Member Enumeration Documentation
Enumerator | |
---|---|
MenuMode |
Creates a button which pops up a menu when interacted with, as defined by toolButtonPopupMode(). |
ComboBoxMode |
Creates a combo box which contains the actions. This is the default. |
Definition at line 106 of file kselectaction.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a selection action with the specified parent.
- Parameters
-
parent The action's parent object.
Definition at line 56 of file kselectaction.cpp.
Constructs a selection action with text; a shortcut may be specified by the ampersand character (e.g.
"&Option" creates a shortcut with key O )
This is the most common KSelectAction used when you do not have a corresponding icon (note that it won't appear in the current version of the "Edit ToolBar" dialog, because an action needs an icon to be plugged in a toolbar...).
- Parameters
-
text The text that will be displayed. parent The action's parent object.
Definition at line 64 of file kselectaction.cpp.
Constructs a selection action with text and an icon; a shortcut may be specified by the ampersand character (e.g.
"&Option" creates a shortcut with key O )
This is the other common KSelectAction used. Use it when you do have a corresponding icon.
- Parameters
-
icon The icon to display. text The text that will be displayed. parent The action's parent object.
Definition at line 73 of file kselectaction.cpp.
|
virtual |
Destructor.
Definition at line 89 of file kselectaction.cpp.
|
protected |
Creates a new KSelectAction object.
- Parameters
-
dd the private d member parent The action's parent object.
Definition at line 81 of file kselectaction.cpp.
Member Function Documentation
QAction * KSelectAction::action | ( | int | index | ) | const |
Returns the action at index, if one exists.
Definition at line 162 of file kselectaction.cpp.
QAction * KSelectAction::action | ( | const QString & | text, |
Qt::CaseSensitivity | cs = Qt::CaseSensitive |
||
) | const |
Searches for an action with the specified text, using a search whose case sensitivity is defined by cs.
Definition at line 170 of file kselectaction.cpp.
Returns the list of selectable actions.
Definition at line 110 of file kselectaction.cpp.
|
protectedvirtualslot |
This function is called whenever an action from the selections is triggered.
Definition at line 309 of file kselectaction.cpp.
|
virtual |
Add action to the list of selectable actions.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
The newly created action is checkable and not user configurable.
Definition at line 230 of file kselectaction.cpp.
Definition at line 254 of file kselectaction.cpp.
Definition at line 271 of file kselectaction.cpp.
void KSelectAction::changeItem | ( | int | index, |
const QString & | text | ||
) |
Changes the text of item.
- Parameters
-
index to text .
Definition at line 337 of file kselectaction.cpp.
void KSelectAction::clear | ( | ) |
Clears up all the items in this action.
- Warning
- The actions will be deleted for backwards compatibility with KDE3. If you just want to remove all actions, use removeAllActions()
Definition at line 376 of file kselectaction.cpp.
int KSelectAction::comboWidth | ( | ) | const |
When this action is plugged into a toolbar, it creates a combobox.
This returns the maximum width set by setComboWidth
Reimplemented from.
- See also
- QWidgetAction.
Reimplemented from QWidgetAction.
Reimplemented in KFontAction.
Definition at line 510 of file kselectaction.cpp.
QAction* KSelectAction::currentAction | ( | ) | const |
Returns the current QAction.
- See also
- setCurrentAction
int KSelectAction::currentItem | ( | ) | const |
Returns the index of the current item.
- See also
- currentItem and currentAction
QString KSelectAction::currentText | ( | ) | const |
Returns the text of the currently selected item.
- See also
- currentItem and currentAction
|
protectedvirtual |
Reimplemented from.
- See also
- QWidgetAction.
Reimplemented from QWidgetAction.
Definition at line 578 of file kselectaction.cpp.
- Reimplemented from superclass.
Reimplemented from KAction.
Definition at line 588 of file kselectaction.cpp.
Reimplemented from QWidgetAction.
Definition at line 646 of file kselectaction.cpp.
bool KSelectAction::isEditable | ( | ) | const |
When this action is plugged into a toolbar, it creates a combobox.
- Returns
- true if the combo editable.
Definition at line 415 of file kselectaction.cpp.
QStringList KSelectAction::items | ( | ) | const |
Convenience function which returns the items that can be selected with this action.
It is the same as iterating selectableActionGroup()->actions() and looking at each action's text().
bool KSelectAction::menuAccelsEnabled | ( | ) | const |
Returns whether ampersands passed to methods using QStrings are interpreted as keyboard accelerator indicators or as literal ampersands.
Definition at line 504 of file kselectaction.cpp.
Remove the specified action from this action selector.
You take ownership here, so save or delete it in order to not leak the action.
Reimplemented in KRecentFilesAction.
Definition at line 278 of file kselectaction.cpp.
void KSelectAction::removeAllActions | ( | ) |
Definition at line 397 of file kselectaction.cpp.
QActionGroup * KSelectAction::selectableActionGroup | ( | ) | const |
The action group used to create exclusivity between the actions associated with this action.
Definition at line 104 of file kselectaction.cpp.
void KSelectAction::setComboWidth | ( | int | width | ) |
When this action is plugged into a toolbar, it creates a combobox.
This gives a maximum size to the combobox. The minimum size is automatically given by the contents (the items).
Definition at line 201 of file kselectaction.cpp.
KSelectAction::setCurrentAction | ( | QAction * | action | ) |
Sets the currently checked item.
- Parameters
-
action the QAction to become the currently checked item.
- Returns
- true if a corresponding action was found and successfully checked.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
If there is no action at that index, the currently checked action (if any) will be deselected.
- Returns
- true if a corresponding action was found and thus set to the current action, otherwise false
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
If there is no action at that index, the currently checked action (if any) will be deselected.
- Returns
- true if a corresponding action was found, otherwise false
Definition at line 133 of file kselectaction.cpp.
bool KSelectAction::setCurrentAction | ( | const QString & | text, |
Qt::CaseSensitivity | cs = Qt::CaseSensitive |
||
) |
Definition at line 195 of file kselectaction.cpp.
bool KSelectAction::setCurrentItem | ( | int | index | ) |
Definition at line 156 of file kselectaction.cpp.
void KSelectAction::setEditable | ( | bool | edit | ) |
When this action is plugged into a toolbar, it creates a combobox.
This makes the combo editable or read-only.
Definition at line 404 of file kselectaction.cpp.
void KSelectAction::setItems | ( | const QStringList & | lst | ) |
Convenience function to create the list of selectable items.
Any previously existing items will be cleared.
Definition at line 349 of file kselectaction.cpp.
void KSelectAction::setMaxComboViewCount | ( | int | n | ) |
Sets the maximum items that are visible at once if the action is a combobox, that is the number of items in the combobox's viewport.
Definition at line 215 of file kselectaction.cpp.
void KSelectAction::setMenuAccelsEnabled | ( | bool | b | ) |
Sets whether any occurrence of the ampersand character ( & ) in items should be interpreted as keyboard accelerator for items displayed in a menu or not.
Only applies to (overloaded) methods dealing with QStrings, not those dealing with QActions.
Defaults to true.
- Parameters
-
b true if ampersands indicate a keyboard accelerator, otherwise false.
Definition at line 498 of file kselectaction.cpp.
void KSelectAction::setToolBarMode | ( | ToolBarMode | mode | ) |
Set the type of widget to be inserted in a toolbar to mode.
Definition at line 434 of file kselectaction.cpp.
void KSelectAction::setToolButtonPopupMode | ( | QToolButton::ToolButtonPopupMode | mode | ) |
Set how this list of actions should behave when in popup mode and plugged into a toolbar.
Definition at line 446 of file kselectaction.cpp.
|
protectedslot |
For structured menu building.
Deselects all items if the action was unchecked by the top menu
Definition at line 421 of file kselectaction.cpp.
ToolBarMode KSelectAction::toolBarMode | ( | ) | const |
Returns which type of widget (combo box or button with drop-down menu) will be inserted in a toolbar.
QToolButton::ToolButtonPopupMode KSelectAction::toolButtonPopupMode | ( | ) | const |
Returns the style for the list of actions, when this action is plugged into a KToolBar.
The default value is QToolButton::InstantPopup
- See also
- QToolButton::setPopupMode()
|
signal |
This signal is emitted when an item is selected;.
- Parameters
-
action indicates the item selected.
|
signal |
This signal is emitted when an item is selected;.
- Parameters
-
index indicates the item selected.
|
signal |
This signal is emitted when an item is selected;.
- Parameters
-
text indicates the item selected.
Member Data Documentation
|
protected |
Definition at line 376 of file kselectaction.h.
Property Documentation
|
readwrite |
Definition at line 56 of file kselectaction.h.
|
readwrite |
Definition at line 54 of file kselectaction.h.
|
readwrite |
Definition at line 61 of file kselectaction.h.
|
read |
Definition at line 57 of file kselectaction.h.
|
readwrite |
Definition at line 55 of file kselectaction.h.
|
readwrite |
Definition at line 62 of file kselectaction.h.
|
readwrite |
Definition at line 59 of file kselectaction.h.
|
readwrite |
Definition at line 60 of file kselectaction.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.