KDEUI
#include <krecentfilesaction.h>
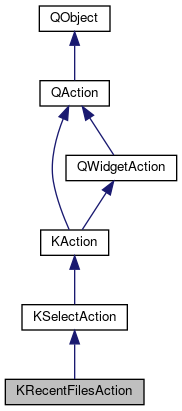
Public Slots | |
virtual void | clear () |
Signals | |
void | recentListCleared () |
void | urlSelected (const KUrl &url) |
![]() | |
void | triggered (QAction *action) |
void | triggered (int index) |
void | triggered (const QString &text) |
![]() | |
void | authorized (KAuth::Action *action) |
void | globalShortcutChanged (const QKeySequence &) |
void | triggered (Qt::MouseButtons buttons, Qt::KeyboardModifiers modifiers) |
Properties | |
int | maxItems |
![]() | |
int | comboWidth |
QAction | currentAction |
int | currentItem |
QString | currentText |
bool | editable |
QStringList | items |
ToolBarMode | toolBarMode |
QToolButton::ToolButtonPopupMode | toolButtonPopupMode |
![]() | |
KShortcut | globalShortcut |
bool | globalShortcutAllowed |
bool | globalShortcutEnabled |
KShortcut | shortcut |
bool | shortcutConfigurable |
![]() | |
autoRepeat | |
checkable | |
checked | |
enabled | |
font | |
icon | |
iconText | |
iconVisibleInMenu | |
menuRole | |
priority | |
shortcut | |
shortcutContext | |
softKeyRole | |
statusTip | |
text | |
toolTip | |
visible | |
whatsThis | |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
enum | ToolBarMode { MenuMode, ComboBoxMode } |
![]() | |
enum | GlobalShortcutLoading { Autoloading = 0x0, NoAutoloading = 0x4 } |
enum | ShortcutType { ActiveShortcut = 0x1, DefaultShortcut = 0x2 } |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | actionTriggered (QAction *action) |
void | slotToggled (bool) |
![]() | |
KSelectAction (KSelectActionPrivate &dd, QObject *parent) | |
virtual QWidget * | createWidget (QWidget *parent) |
virtual void | deleteWidget (QWidget *widget) |
virtual bool | event (QEvent *event) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QList< QWidget * > | createdWidgets () const |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
KSelectActionPrivate * | d_ptr |
Detailed Description
Recent files action.
This class is an action to handle a recent files submenu. The best way to create the action is to use KStandardAction::openRecent. Then you simply need to call loadEntries on startup, saveEntries on shutdown, addURL when your application loads/saves a file.
Definition at line 46 of file krecentfilesaction.h.
Constructor & Destructor Documentation
|
explicit |
Constructs an action with the specified parent.
- Parameters
-
parent The parent of this action.
Definition at line 47 of file krecentfilesaction.cpp.
Constructs an action with text; a shortcut may be specified by the ampersand character (e.g.
"&Option" creates a shortcut with key O )
This is the most common KAction used when you do not have a corresponding icon (note that it won't appear in the current version of the "Edit ToolBar" dialog, because an action needs an icon to be plugged in a toolbar...).
- Parameters
-
text The text that will be displayed. parent The parent of this action.
Definition at line 54 of file krecentfilesaction.cpp.
KRecentFilesAction::KRecentFilesAction | ( | const KIcon & | icon, |
const QString & | text, | ||
QObject * | parent | ||
) |
Constructs an action with text and an icon; a shortcut may be specified by the ampersand character (e.g.
"&Option" creates a shortcut with key O )
This is the other common KAction used. Use it when you do have a corresponding icon.
- Parameters
-
icon The icon to display. text The text that will be displayed. parent The parent of this action.
Definition at line 64 of file krecentfilesaction.cpp.
|
virtual |
Destructor.
Definition at line 91 of file krecentfilesaction.cpp.
Member Function Documentation
Adds action to the list of URLs, with url and title name.
Do not use addAction(QAction*), as no url will be associated, and consequently urlSelected() will not be emitted when action is selected.
Definition at line 200 of file krecentfilesaction.cpp.
Add URL to recent files list.
- Parameters
-
url The URL of the file name The user visible pretty name that appears before the URL
Create a deep copy here, because if _url is the parameter from urlSelected() signal, we will delete it in the removeAction() call below. but access it again in the addAction call... => crash
Definition at line 150 of file krecentfilesaction.cpp.
|
virtualslot |
Clears the recent files list.
Note that there is also an action shown to the user for clearing the list.
Definition at line 247 of file krecentfilesaction.cpp.
void KRecentFilesAction::loadEntries | ( | const KConfigGroup & | config | ) |
Loads the recent files entries from a given KConfigGroup object.
You can provide the name of the group used to load the entries. If the groupname is empty, entries are load from a group called 'RecentFiles'
Definition at line 265 of file krecentfilesaction.cpp.
int KRecentFilesAction::maxItems | ( | ) | const |
Returns the maximum of items in the recent files list.
|
signal |
This signal gets emitted when the user clear list.
So when user store url in specific config file it can saveEntry.
- Since
- 4.3
Reimplemented for internal reasons.
Reimplemented from KSelectAction.
Definition at line 220 of file krecentfilesaction.cpp.
void KRecentFilesAction::removeUrl | ( | const KUrl & | url | ) |
Remove an URL from the recent files list.
- Parameters
-
url The URL of the file
Definition at line 231 of file krecentfilesaction.cpp.
void KRecentFilesAction::saveEntries | ( | const KConfigGroup & | config | ) |
Saves the current recent files entries to a given KConfigGroup object.
You can provide the name of the group used to load the entries. If the groupname is empty, entries are saved to a group called 'RecentFiles'
Definition at line 322 of file krecentfilesaction.cpp.
void KRecentFilesAction::setMaxItems | ( | int | maxItems | ) |
Sets the maximum of items in the recent files list.
The default for this value is 10 set in the constructor.
If this value is lesser than the number of items currently in the recent files list the last items are deleted until the number of items are equal to the new maximum.
Definition at line 107 of file krecentfilesaction.cpp.
KUrl::List KRecentFilesAction::urls | ( | ) | const |
Retrieve a list of all URLs in the recent files list.
Definition at line 241 of file krecentfilesaction.cpp.
|
signal |
This signal gets emitted when the user selects an URL.
- Parameters
-
url The URL thats the user selected.
Property Documentation
|
readwrite |
Definition at line 49 of file krecentfilesaction.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.