KDEWebKit
#include <kwebpage.h>
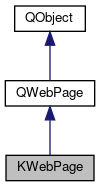
Public Types | |
enum | IntegrationFlags { NoIntegration = 0x01, KIOIntegration = 0x02, KPartsIntegration = 0x04, KWalletIntegration = 0x08 } |
Public Slots | |
virtual void | downloadRequest (const QNetworkRequest &request) |
void | downloadResponse (QNetworkReply *reply) |
virtual void | downloadUrl (const KUrl &url) |
Protected Member Functions | |
virtual bool | acceptNavigationRequest (QWebFrame *frame, const QNetworkRequest &request, NavigationType type) |
bool | handleReply (QNetworkReply *reply, QString *contentType=0, KIO::MetaData *metaData=0) |
void | removeRequestMetaData (const QString &key) |
void | removeSessionMetaData (const QString &key) |
QString | requestMetaData (const QString &key) const |
QString | sessionMetaData (const QString &key) const |
void | setRequestMetaData (const QString &key, const QString &value) |
void | setSessionMetaData (const QString &key, const QString &value) |
virtual QString | userAgentForUrl (const QUrl &url) const |
![]() | |
virtual QString | chooseFile (QWebFrame *parentFrame, const QString &suggestedFile) |
virtual QObject * | createPlugin (const QString &classid, const QUrl &url, const QStringList ¶mNames, const QStringList ¶mValues) |
virtual QWebPage * | createWindow (WebWindowType type) |
virtual void | javaScriptAlert (QWebFrame *frame, const QString &msg) |
virtual bool | javaScriptConfirm (QWebFrame *frame, const QString &msg) |
virtual void | javaScriptConsoleMessage (const QString &message, int lineNumber, const QString &sourceID) |
virtual bool | javaScriptPrompt (QWebFrame *frame, const QString &msg, const QString &defaultValue, QString *result) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | FindFlags |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
contentEditable | |
forwardUnsupportedContent | |
hasSelection | |
linkDelegationPolicy | |
modified | |
palette | |
preferredContentsSize | |
selectedHtml | |
selectedText | |
viewportSize | |
![]() | |
objectName | |
Detailed Description
An enhanced QWebPage that provides integration into the KDE environment.
This is a convenience class that provides full integration with KDE technologies such as KIO for network request handling, KCookiejar for cookie handling, KParts for embedding non-html content and KWallet for storing form data. It also sets standard icons for many of the actions provided by QWebPage.
Most of this integration happens behind the scenes. If you want KWallet integration, however, you will have to provide a mechanism for deciding whether to allow form data to be stored. To do this, you will need to connect to the KWebWallet::saveFormDataRequested signal and call either KWebWallet::acceptSaveFormDataRequest or KWebWallet::rejectSaveFormDataRequest, typically after asking the user whether they want to save the form data. If you do not do this, no form data will be saved.
KWebPage will also not automatically load form data for you. You should connect to QWebPage::loadFinished and, if the page was loaded successfully, call
- See also
- KIO::Integration
- KWebWallet
- Since
- 4.4
Definition at line 75 of file kwebpage.h.
Member Enumeration Documentation
Flags for setting the desired level of integration.
Enumerator | |
---|---|
NoIntegration |
Provide only very basic integration such as using KDE icons for the actions provided by QWebPage. |
KIOIntegration |
Use KIO to handle network requests.
|
KPartsIntegration |
Use KPart componenets, if available, to display content in <embed> and <object> tags. |
KWalletIntegration |
Use KWallet to store login credentials and other form data from web sites.
|
Definition at line 84 of file kwebpage.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a KWebPage with parent parent
.
Note that if no integration flags are set (the default), all integration options are activated. If you inherit from this class you can use the flags in IntegrationFlags to control how much integration should be used.
Definition at line 222 of file kwebpage.cpp.
KWebPage::~KWebPage | ( | ) |
Destroys the KWebPage.
Definition at line 285 of file kwebpage.cpp.
Member Function Documentation
|
protectedvirtual |
- Reimplemented from superclass.
This performs various integration-related actions when navigation is requested. If you override this method, make sure you call the parent's implementation unless you want to block the request outright.
If you do override acceptNavigationRequest and call this method, however, be aware of the effect of the page's linkDelegationPolicy on how * QWebPage::acceptNavigationRequest behaves.
Reimplemented from QWebPage.
Definition at line 445 of file kwebpage.cpp.
|
virtualslot |
Download request
using KIO.
This slot first prompts the user where to save the requested resource and then downloads it using KIO.
Definition at line 323 of file kwebpage.cpp.
|
slot |
Download the resource specified by reply
using KIO.
This slot first prompts the user where to save the requested resource and then downloads it using KIO.
In KDE 4.8 and higher, if reply
contains a QObject property called "DownloadManagerExe", then an attempt will be made to the command specified by that property to download the specified resource.
If the "DownloadManagerExe" property is not defined or the command specified by it could not be successfully executed, then the user will be prompted for the action to take.
- Since
- 4.5
- See also
- handleReply
Definition at line 340 of file kwebpage.cpp.
|
virtualslot |
Download url
using KIO.
This slot first prompts the user where to save the requested resource and then downloads it using KIO.
Definition at line 335 of file kwebpage.cpp.
|
protected |
Attempts to handle reply
and returns true on success, false otherwise.
In KDE 4.8 and higher, if reply
contains a QObject property called "DownloadManagerExe", then an attempt will be made to let the command specified by that property to download the requested resource.
If the "DownloadManagerExe" property is not defined or the command specified by it could not be successfully executed, then the user will be prompted for the action to take.
- Parameters
-
reply the QNetworkReply object to be handled. contentType if not null, it will be set to the content-type specified in reply
, if any.metaData if not null, it will be set to the KIO meta-data specified in reply
, if any.
- Since
- 4.6.3
Definition at line 477 of file kwebpage.cpp.
bool KWebPage::isExternalContentAllowed | ( | ) | const |
Whether access to remote content is permitted.
If this is false
, only resources on the local system can be accessed through this web page. By default access to remote content is allowed.
If KIO integration is disabled, this will always return true
.
- Returns
true
if access to remote content is permitted,false
otherwise
Definition at line 290 of file kwebpage.cpp.
|
protected |
Remove an item of request metadata.
Removes the temporary (per-request) metadata associated with key
.
- Parameters
-
key the key for the metadata to remove
Definition at line 416 of file kwebpage.cpp.
|
protected |
Remove an item of session metadata.
Removes the permanent (per-session) metadata associated with key
.
- Parameters
-
key the key for the metadata to remove
Definition at line 409 of file kwebpage.cpp.
Get an item of request metadata.
Retrieves the value of the temporary (per-request) metadata for key
.
If KIO integration is disabled, this will always return an empty string.
- Parameters
-
key the key of the metadata to retrieve
- Returns
- the value of the metadata associated with
key
, or an empty string if there is no such metadata
Definition at line 384 of file kwebpage.cpp.
Get an item of session metadata.
Retrieves the value of the permanent (per-session) metadata for key
.
If KIO integration is disabled, this will always return an empty string.
- Parameters
-
key the key of the metadata to retrieve
- Returns
- the value of the metadata associated with
key
, or an empty string if there is no such metadata
Definition at line 373 of file kwebpage.cpp.
void KWebPage::setAllowExternalContent | ( | bool | allow | ) |
Set whether to allow remote content.
If KIO integration is not enabled, this method will have no effect.
- See also
- isExternalContentAllowed()
- KIO::Integration::AccessManager::setAllowExternalContent(bool)
- Parameters
-
allow true
if access to remote content should be allowed,false
if only local content should be accessible
Definition at line 303 of file kwebpage.cpp.
Set an item of metadata to be sent to the KIO slave with the next request.
If KIO integration is disabled, this method will have no effect.
Metadata set using this method will be deleted after it has been sent once.
- Parameters
-
key the key for the metadata; any existing metadata associated with this key will be overwritten value the value to associate with key
Definition at line 402 of file kwebpage.cpp.
Set an item of metadata to be sent to the KIO slave with every request.
If KIO integration is disabled, this method will have no effect.
Metadata set using this method will be sent with every request.
- Parameters
-
key the key for the metadata; any existing metadata associated with this key will be overwritten value the value to associate with key
Definition at line 395 of file kwebpage.cpp.
void KWebPage::setWallet | ( | KWebWallet * | wallet | ) |
Set the KWebWallet that is used to store form data.
This KWebPage will take ownership of wallet
, so that the wallet is deleted when the KWebPage is deleted. If you do not want that to happen, you should call setParent() on wallet
after calling this function.
- See also
- KWebWallet
- Parameters
-
wallet the KWebWallet to be used for storing form data, or 0 to disable KWallet integration
Definition at line 310 of file kwebpage.cpp.
- Reimplemented from superclass.
This function is re-implemented to provide KDE user-agent management integration through KProtocolManager.
If a special user-agent has been configured for the host indicated by url
, that user-agent will be returned. Otherwise, QWebPage's default user agent is returned.
Reimplemented from QWebPage.
Definition at line 423 of file kwebpage.cpp.
KWebWallet * KWebPage::wallet | ( | ) | const |
The wallet integration manager.
If you wish to use KDE wallet integration, you will have to connect to signals emitted by this object and react accordingly. See KWebWallet for more information.
- Returns
- the wallet integration manager, or 0 if KDE wallet integration is disabled
Definition at line 298 of file kwebpage.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:26:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.