KDEWebKit
#include <kwebwallet.h>
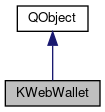
Classes | |
struct | WebForm |
Public Types | |
typedef QList< WebForm > | WebFormList |
Public Slots | |
void | acceptSaveFormDataRequest (const QString &key) |
void | rejectSaveFormDataRequest (const QString &key) |
Signals | |
void | fillFormRequestCompleted (bool ok) |
void | saveFormDataCompleted (const QUrl &url, bool ok) |
void | saveFormDataRequested (const QString &key, const QUrl &url) |
void | walletClosed () |
Public Member Functions | |
KWebWallet (QObject *parent=0, WId wid=0) | |
virtual | ~KWebWallet () |
void | fillFormData (QWebFrame *frame, bool recursive=true) |
WebFormList | formsWithCachedData (QWebFrame *frame, bool recursive=true) const |
void | removeFormData (QWebFrame *frame, bool recursive) |
void | removeFormData (const WebFormList &forms) |
void | saveFormData (QWebFrame *frame, bool recursive=true, bool ignorePasswordFields=false) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
virtual void | fillFormDataFromCache (const KUrl::List &list) |
void | fillWebForm (const KUrl &url, const WebFormList &forms) |
WebFormList | formsToDelete () const |
WebFormList | formsToFill (const KUrl &url) const |
WebFormList | formsToSave (const QString &key) const |
virtual bool | hasCachedFormData (const WebForm &form) const |
virtual void | removeFormDataFromCache (const WebFormList &forms) |
virtual void | saveFormDataToCache (const QString &key) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
A class that provides KDE wallet integration for QWebFrame.
Normally, you will use this class via KWebPage. In this case, you need to connect to the saveFormDataRequested signal and call either acceptSaveFormDataRequest or rejectSaveFormDataRequest, typically after asking the user whether they want to save the form data.
You will also need to call fillFormData when a QWebFrame has finished loading. To do this, connect to QWebPage::loadFinished and, if the page was loaded successfully, call
If you wish to use this directly with a subclass of QWebPage, you should call saveFormData from QWebPage::acceptNavigationRequest when a user submits a form.
- See also
- KWebPage
- Since
- 4.4
Definition at line 64 of file kwebwallet.h.
Member Typedef Documentation
A list of web forms.
Definition at line 94 of file kwebwallet.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a KWebWallet.
parent
is usually the QWebPage this wallet is being used for.
The wid
parameter is used to tell KDE's wallet manager which window is requesting access to the wallet.
- Parameters
-
parent the owner of this wallet wid the window ID of the window the web page will be embedded in
Definition at line 379 of file kwebwallet.cpp.
|
virtual |
Destructor.
Definition at line 394 of file kwebwallet.cpp.
Member Function Documentation
|
slot |
Accepts the save form data request associated with key
.
The key
parameter is the one sent through the saveFormDataRequested signal.
You must always call this function or rejectSaveFormDataRequest in order to complete the save form data request. Otherwise, the request will simply be ignored.
- See also
- saveFormDataRequested.
Definition at line 530 of file kwebwallet.cpp.
Attempts to fill forms contained in frame
with cached data.
If recursive
is set to true, the default, then this function will attempt to fill out forms in the specified frame and all its children frames.
Definition at line 419 of file kwebwallet.cpp.
|
protectedvirtual |
Fills form data from persistent storage.
If you reimplement this function, call formsToFill to obtain the list of forms pending to be filled. Once you fill the list with the cached data from the persistent storage, you must call fillWebForm
to fill out the actual web forms.
- See also
- formsToFill
Definition at line 584 of file kwebwallet.cpp.
|
signal |
This signal is emitted whenever a fill form data request is completed.
ok
will be set to true if any forms were successfully filled with cached data from the persistent storage.
- See also
- fillFormData
- Since
- 4.5
|
protected |
Fills the web forms in frame that point to url
with data from forms
.
- See also
- fillFormDataFromCache.
Definition at line 540 of file kwebwallet.cpp.
|
protected |
Returns forms to be removed from persistent storage.
|
protected |
Returns a list of forms for url
that are waiting to be filled.
This function returns an empty list if there is no pending requests for filling forms associated with url
.
Definition at line 567 of file kwebwallet.cpp.
|
protected |
Returns a list of for key
that are waiting to be saved.
This function returns an empty list if there are no pending requests for saving forms associated with key
.
Definition at line 572 of file kwebwallet.cpp.
KWebWallet::WebFormList KWebWallet::formsWithCachedData | ( | QWebFrame * | frame, |
bool | recursive = true |
||
) | const |
Returns a list of forms in frame
that have cached data in the peristent storage.
If recursive
is set to true, the default, then this function will will also return the cached form data for all the children frames of frame
.
If the site currently rendered in frame
does not contain any forms or there is no cached data for the forms found in frame
, then this function will return an empty list.
Note that this function will only return the information about the forms in frame
and not their cached data, i.e. the fields member variable in the returned WebForm list will always be empty.
Definition at line 399 of file kwebwallet.cpp.
Returns true when there is data associated with form
in the persistent storage.
Definition at line 577 of file kwebwallet.cpp.
|
slot |
Rejects the save form data request associated with key
.
The key
parameter is the one sent through the saveFormDataRequested signal.
- See also
- saveFormDataRequested.
Definition at line 535 of file kwebwallet.cpp.
Removes the form data specified by forms
from the persistent storage.
This function is provided for convenience and simply calls formsWithCachedData and removeFormData(WebFormList). Note that this function will remove all cached data for forms found in frame
. If recursive
is set to true, then all cached data for all of the child frames of frame
will be removed from the persistent storage as well.
- See also
- formsWithCachedData
- removeFormData
Definition at line 518 of file kwebwallet.cpp.
void KWebWallet::removeFormData | ( | const WebFormList & | forms | ) |
Removes the form data specified by forms
from the persistent storage.
Call formsWithCachedData to obtain a list of forms with data cached in persistent storage.
- See also
- formsWithCachedData
Definition at line 524 of file kwebwallet.cpp.
|
protectedvirtual |
Removes all cached form data associated with forms
from persistent storage.
If you reimplement this function, call formsToDelete to obtain the list of form data pending to be removed from persistent storage.
- See also
- formsToDelete
Definition at line 608 of file kwebwallet.cpp.
void KWebWallet::saveFormData | ( | QWebFrame * | frame, |
bool | recursive = true , |
||
bool | ignorePasswordFields = false |
||
) |
Attempts to save the form data from frame
and its children frames.
If recursive
is set to true, the default, then form data from all the child frames of frame
will be saved. Set ignorePasswordFields
to true if you do not want data from password fields to not be saved.
You must connect to the saveFormDataRequested signal and call either rejectSaveFormDataRequest or acceptSaveFormDataRequest signals in order to complete the save request. Otherwise, you request will simply be ignored.
Definition at line 478 of file kwebwallet.cpp.
This signal is emitted whenever a save form data request is completed.
ok
will be set to true if the save form data request for url
was completed successfully.
- See also
- saveFormDataRequested
This signal is emitted whenever a save form data request is received.
Unless you connect to this signal and and call acceptSaveFormDataRequest or rejectSaveFormDataRequest slots, the save form data requested through saveFormData will simply be ignored.
key
is a value that uniquely identifies the save request and url
is the address for which the form data is being saved.
|
protectedvirtual |
Stores form data associated with key
to a persistent storage.
If you reimplement this function, call formsToSave to obtain the list of form data pending to be saved to persistent storage.
- See also
- formsToSave
Definition at line 599 of file kwebwallet.cpp.
|
signal |
This signal is emitted whenever the current wallet is closed.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:26:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.