KIO
#include <jobclasses.h>
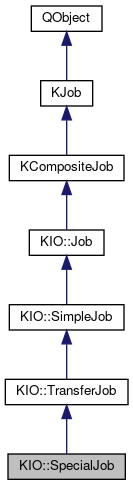
Public Member Functions | |
SpecialJob (const KUrl &url, const QByteArray &data=QByteArray()) | |
~SpecialJob () | |
QByteArray | arguments () const |
void | setArguments (const QByteArray &data) |
![]() | |
~TransferJob () | |
bool | isErrorPage () const |
QString | mimetype () const |
bool | reportDataSent () const |
void | sendAsyncData (const QByteArray &data) |
void | setAsyncDataEnabled (bool enabled) |
void | setModificationTime (const QDateTime &mtime) |
void | setReportDataSent (bool enabled) |
void | setTotalSize (KIO::filesize_t bytes) |
![]() | |
~SimpleJob () | |
bool | isRedirectionHandlingEnabled () const |
virtual void | putOnHold () |
void | setRedirectionHandlingEnabled (bool handle) |
const KUrl & | url () const |
![]() | |
virtual | ~Job () |
void | addMetaData (const QString &key, const QString &value) |
void | addMetaData (const QMap< QString, QString > &values) |
QStringList | detailedErrorStrings (const KUrl *reqUrl=0L, int method=-1) const |
QString | errorString () const |
bool | isInteractive () const |
void | mergeMetaData (const QMap< QString, QString > &values) |
MetaData | metaData () const |
MetaData | outgoingMetaData () const |
Job * | parentJob () const |
QString | queryMetaData (const QString &key) |
void | setMetaData (const KIO::MetaData &metaData) |
void | setParentJob (Job *parentJob) |
void | showErrorDialog (QWidget *parent=0) |
void | start () |
JobUiDelegate * | ui () const |
![]() | |
KCompositeJob (QObject *parent=0) | |
virtual | ~KCompositeJob () |
virtual | ~KJob () |
Capabilities | capabilities () const |
int | error () const |
QString | errorText () const |
bool | exec () |
bool | isAutoDelete () const |
bool | isSuspended () const |
KJob (QObject *parent=0) | |
unsigned long | percent () const |
qulonglong | processedAmount (Unit unit) const |
void | setAutoDelete (bool autodelete) |
void | setUiDelegate (KJobUiDelegate *delegate) |
qulonglong | totalAmount (Unit unit) const |
KJobUiDelegate * | uiDelegate () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
enum | Capability |
enum | KillVerbosity |
enum | Unit |
![]() | |
void | slotError (int, const QString &) |
![]() | |
bool | kill (KillVerbosity verbosity=Quietly) |
bool | resume () |
bool | suspend () |
![]() | |
void | canResume (KIO::Job *job, KIO::filesize_t offset) |
void | data (KIO::Job *job, const QByteArray &data) |
void | dataReq (KIO::Job *job, QByteArray &data) |
void | mimetype (KIO::Job *job, const QString &type) |
void | permanentRedirection (KIO::Job *job, const KUrl &fromUrl, const KUrl &toUrl) |
void | redirection (KIO::Job *job, const KUrl &url) |
![]() | |
void | canceled (KJob *job) |
void | connected (KIO::Job *job) |
![]() | |
void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1=qMakePair(QString(), QString()), const QPair< QString, QString > &field2=qMakePair(QString(), QString())) |
void | finished (KJob *job) |
void | infoMessage (KJob *job, const QString &plain, const QString &rich=QString()) |
void | percent (KJob *job, unsigned long percent) |
void | processedAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
void | processedSize (KJob *job, qulonglong size) |
void | result (KJob *job) |
void | resumed (KJob *job) |
void | speed (KJob *job, unsigned long speed) |
void | suspended (KJob *job) |
void | totalAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
void | totalSize (KJob *job, qulonglong size) |
void | warning (KJob *job, const QString &plain, const QString &rich=QString()) |
![]() | |
static void | removeOnHold () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | slotData (const QByteArray &data) |
virtual void | slotDataReq () |
virtual void | slotFinished () |
virtual void | slotMetaData (const KIO::MetaData &_metaData) |
virtual void | slotMimetype (const QString &mimetype) |
virtual void | slotRedirection (const KUrl &url) |
![]() | |
virtual void | slotFinished () |
virtual void | slotMetaData (const KIO::MetaData &_metaData) |
virtual void | slotWarning (const QString &) |
![]() | |
virtual void | slotInfoMessage (KJob *job, const QString &plain, const QString &rich) |
![]() | |
TransferJob (TransferJobPrivate &dd) | |
virtual bool | doResume () |
virtual void | slotResult (KJob *job) |
![]() | |
SimpleJob (SimpleJobPrivate &dd) | |
virtual bool | doKill () |
virtual bool | doSuspend () |
void | storeSSLSessionFromJob (const KUrl &m_redirectionURL) |
![]() | |
Job () | |
Job (JobPrivate &dd) | |
virtual bool | addSubjob (KJob *job) |
virtual bool | removeSubjob (KJob *job) |
![]() | |
KCompositeJob (KCompositeJobPrivate &dd, QObject *parent) | |
void | clearSubjobs () |
void | emitPercent (qulonglong processedAmount, qulonglong totalAmount) |
void | emitResult () |
void | emitSpeed (unsigned long speed) |
bool | hasSubjobs () |
KJob (KJobPrivate &dd, QObject *parent) | |
void | setCapabilities (Capabilities capabilities) |
void | setError (int errorCode) |
void | setErrorText (const QString &errorText) |
void | setPercent (unsigned long percentage) |
void | setProcessedAmount (Unit unit, qulonglong amount) |
void | setTotalAmount (Unit unit, qulonglong amount) |
const QList< KJob * > & | subjobs () const |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
KJobPrivate *const | d_ptr |
![]() | |
objectName | |
Detailed Description
A class that sends a special command to an ioslave.
This allows you to send a binary blob to an ioslave and handle its responses. The ioslave will receive the binary data as an argument to the "special" function (inherited from SlaveBase::special()).
Use this only on ioslaves that belong to your application. Sending special commands to other ioslaves may cause unexpected behaviour.
- See also
- KIO::special
Definition at line 1022 of file jobclasses.h.
Constructor & Destructor Documentation
|
explicit |
Creates a KIO::SpecialJob.
- Parameters
-
url the URL to be passed to the ioslave data the data to be sent to the SlaveBase::special() function.
Member Function Documentation
QByteArray SpecialJob::arguments | ( | ) | const |
Returns the QByteArray data that will be sent (or has been sent) to the ioslave.
void SpecialJob::setArguments | ( | const QByteArray & | data | ) |
Sets the QByteArray that is passed to SlaveBase::special() on the ioslave.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.