KParts
#include <browserrun.h>
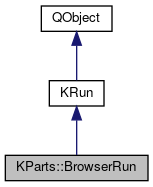
Public Types | |
enum | AskEmbedOrSaveFlags { InlineDisposition = 0, AttachmentDisposition = 1 } |
enum | AskSaveResult { Save, Open, Cancel } |
Public Member Functions | |
BrowserRun (const KUrl &url, const KParts::OpenUrlArguments &args, const KParts::BrowserArguments &browserArgs, KParts::ReadOnlyPart *part, QWidget *window, bool removeReferrer, bool trustedSource, bool hideErrorDialog=false) | |
virtual | ~BrowserRun () |
KParts::OpenUrlArguments & | arguments () |
KParts::BrowserArguments & | browserArguments () |
QString | contentDisposition () const |
bool | hideErrorDialog () const |
KParts::ReadOnlyPart * | part () const |
virtual void | save (const KUrl &url, const QString &suggestedFileName) |
bool | serverSuggestsSave () const |
KUrl | url () const |
![]() | |
KRun (const KUrl &url, QWidget *window, mode_t mode=0, bool isLocalFile=false, bool showProgressInfo=true, const QByteArray &asn=QByteArray()) | |
virtual | ~KRun () |
void | abort () |
bool | autoDelete () const |
bool | hasError () const |
bool | hasFinished () const |
void | setAutoDelete (bool b) |
void | setEnableExternalBrowser (bool b) |
void | setPreferredService (const QString &desktopEntryName) |
void | setRunExecutables (bool b) |
void | setSuggestedFileName (const QString &fileName) |
QString | suggestedFileName () const |
QWidget * | window () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static bool | allowExecution (const QString &mimeType, const KUrl &url) |
static AskSaveResult | askEmbedOrSave (const KUrl &url, const QString &mimeType, const QString &suggestedFileName=QString(), int flags=0) |
static AskSaveResult | askSave (const KUrl &url, KService::Ptr offer, const QString &mimeType, const QString &suggestedFileName=QString()) |
static bool | isTextExecutable (const QString &mimeType) |
static KUrl | makeErrorUrl (int error, const QString &errorText, const QString &initialUrl) |
static void | saveUrl (const KUrl &url, const QString &suggestedFileName, QWidget *window, const KParts::OpenUrlArguments &args) |
static void | saveUrlUsingKIO (const KUrl &srcUrl, const KUrl &destUrl, QWidget *window, const QMap< QString, QString > &metaData) |
static void | simpleSave (const KUrl &url, const QString &suggestedFileName, QWidget *window=0) |
![]() | |
static QString | binaryName (const QString &execLine, bool removePath) |
static bool | checkStartupNotify (const QString &binName, const KService *service, bool *silent_arg, QByteArray *wmclass_arg) |
static bool | displayOpenWithDialog (const KUrl::List &lst, QWidget *window, bool tempFiles=false, const QString &suggestedFileName=QString(), const QByteArray &asn=QByteArray()) |
static bool | isExecutable (const QString &serviceType) |
static bool | isExecutableFile (const KUrl &url, const QString &mimetype) |
static QStringList | processDesktopExec (const KService &_service, const KUrl::List &_urls, bool tempFiles=false, const QString &suggestedFileName=QString()) |
static bool | run (const QString &exec, const KUrl::List &urls, QWidget *window, const QString &name=QString(), const QString &icon=QString(), const QByteArray &asn=QByteArray()) |
static bool | run (const KService &service, const KUrl::List &urls, QWidget *window, bool tempFiles=false, const QString &suggestedFileName=QString(), const QByteArray &asn=QByteArray()) |
static bool | runCommand (const QString &cmd, QWidget *window) |
static bool | runCommand (const QString &cmd, const QString &execName, const QString &icon, QWidget *window, const QByteArray &asn=QByteArray()) |
static bool | runCommand (const QString &cmd, QWidget *window, const QString &workingDirectory) |
static bool | runCommand (const QString &cmd, const QString &execName, const QString &icon, QWidget *window, const QByteArray &asn, const QString &workingDirectory) |
static bool | runUrl (const KUrl &url, const QString &mimetype, QWidget *window, bool tempFile=false, bool runExecutables=true, const QString &suggestedFileName=QString(), const QByteArray &asn=QByteArray()) |
static void | shellQuote (QString &str) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Types | |
enum | NonEmbeddableResult { Handled, NotHandled, Delayed } |
Protected Slots | |
void | slotBrowserMimetype (KIO::Job *job, const QString &type) |
void | slotBrowserScanFinished (KJob *job) |
void | slotCopyToTempFileResult (KJob *job) |
virtual void | slotStatResult (KJob *job) |
![]() | |
void | mimeTypeDetermined (const QString &mimeType) |
void | slotScanFinished (KJob *) |
void | slotScanMimeType (KIO::Job *, const QString &type) |
virtual void | slotStatResult (KJob *) |
void | slotTimeout () |
Protected Member Functions | |
virtual void | handleError (KJob *job) |
NonEmbeddableResult | handleNonEmbeddable (const QString &mimeType) |
NonEmbeddableResult | handleNonEmbeddable (const QString &mimeType, KService::Ptr *pSelectedService) |
virtual void | init () |
virtual void | scanFile () |
![]() | |
bool | doScanFile () const |
virtual void | foundMimeType (const QString &type) |
bool | initializeNextAction () const |
bool | isDirectory () const |
bool | isLocalFile () const |
KIO::Job * | job () |
virtual void | killJob () |
mode_t | mode () const |
bool | progressInfo () const |
void | setDoScanFile (bool scanFile) |
void | setError (bool error) |
void | setFinished (bool finished) |
void | setInitializeNextAction (bool initialize) |
void | setIsDirecory (bool isDirectory) |
void | setIsLocalFile (bool isLocalFile) |
void | setJob (KIO::Job *job) |
void | setMode (mode_t mode) |
void | setProgressInfo (bool progressInfo) |
void | setUrl (const KUrl &url) |
QTimer & | timer () |
KUrl | url () const |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
void | error () |
void | finished () |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
This class extends KRun to provide additional functionality for browsers:
- "save or open" dialog boxes
- "save" functionality
- support for HTTP POST (including saving the result to a temp file if opening a separate application)
- warning before launching executables off the web
- custom error handling (i.e. treating errors as HTML pages)
- generation of SSL metadata depending on the previous URL shown by the part
Definition at line 39 of file browserrun.h.
Member Enumeration Documentation
Enumerator | |
---|---|
InlineDisposition | |
AttachmentDisposition |
Definition at line 98 of file browserrun.h.
Enumerator | |
---|---|
Save | |
Open | |
Cancel |
Definition at line 80 of file browserrun.h.
|
protected |
NotHandled means that foundMimeType should call KRun::foundMimeType, i.e.
launch an external app.
Enumerator | |
---|---|
Handled | |
NotHandled | |
Delayed |
Definition at line 176 of file browserrun.h.
Constructor & Destructor Documentation
BrowserRun::BrowserRun | ( | const KUrl & | url, |
const KParts::OpenUrlArguments & | args, | ||
const KParts::BrowserArguments & | browserArgs, | ||
KParts::ReadOnlyPart * | part, | ||
QWidget * | window, | ||
bool | removeReferrer, | ||
bool | trustedSource, | ||
bool | hideErrorDialog = false |
||
) |
- Parameters
-
url the URL we're probing args URL args - includes reload, metaData, etc. browserArgs browser-related args - includes data for a HTTP POST, etc. part the part going to open this URL - can be 0 if not created yet window the mainwindow - passed to KIO::Job::setWindow() removeReferrer if true, the "referrer" metadata from args
isn't passed ontrustedSource if false, a warning will be shown before launching an executable. Always pass false for trustedSource
, except for local directory views.hideErrorDialog if true, no dialog will be shown in case of errors.
Definition at line 57 of file browserrun.cpp.
|
virtual |
Definition at line 73 of file browserrun.cpp.
Member Function Documentation
Definition at line 344 of file browserrun.cpp.
KParts::OpenUrlArguments & KParts::BrowserRun::arguments | ( | ) |
Definition at line 595 of file browserrun.cpp.
|
static |
Similar to askSave but for the case where the current application is able to embed the url itself (instead of passing it to another app).
- Parameters
-
url the URL in question mimeType the mimetype of the URL suggestedFileName optional filename suggested by the server flags set to AttachmentDisposition if suggested by the server
- Returns
- Save, Open or Cancel.
- Deprecated:
- use BrowserOpenOrSaveQuestion const BrowserOpenOrSaveQuestion::Result res = dlg.askEmbedOrSave(flags);// Important: returns Embed now, not Open!
Definition at line 373 of file browserrun.cpp.
|
static |
Ask the user whether to save or open a url in another application.
- Parameters
-
url the URL in question offer the application that will be used to open the URL mimeType the mimetype of the URL suggestedFileName optional file name suggested by the server
- Returns
- Save, Open or Cancel.
- Deprecated:
- use BrowserOpenOrSaveQuestion const BrowserOpenOrSaveQuestion::Result res = dlg.askOpenOrSave();
Definition at line 359 of file browserrun.cpp.
KParts::BrowserArguments & KParts::BrowserRun::browserArguments | ( | ) |
Definition at line 600 of file browserrun.cpp.
QString BrowserRun::contentDisposition | ( | ) | const |
- Returns
- Suggested disposition by the server (e.g. HTTP content-disposition)
Definition at line 583 of file browserrun.cpp.
|
protectedvirtual |
Called when an error happens.
NOTE: job
could be 0L, if you passed hideErrorDialog=true. The default implementation shows a message box, but only when job != 0 .... It is strongly recommended to reimplement this method if you passed hideErrorDialog=true.
Definition at line 491 of file browserrun.cpp.
|
protected |
Helper for foundMimeType: call this if the mimetype couldn't be embedded.
Definition at line 265 of file browserrun.cpp.
|
protected |
Helper for foundMimeType: call this if the mimetype couldn't be embedded.
- Parameters
-
mimeType the mimetype found for the URL pSelectedService Output variable: pointer to a KService::Ptr, which will be set to the service selected in the BrowserOpenOrSaveQuestion dialog, if any.
How to handle this properly: if pSelectedService is non-zero, then the dialog will show additional "open with" buttons. In your code, you should write:
- Since
- 4.5
Definition at line 270 of file browserrun.cpp.
bool BrowserRun::hideErrorDialog | ( | ) | const |
Definition at line 578 of file browserrun.cpp.
|
protectedvirtual |
Definition at line 572 of file browserrun.cpp.
|
static |
KDE webbrowsing kparts support error urls to display errors in-line in the browser component.
This helper method creates the error URL from its parameters.
- Parameters
-
error the KIO error code (or KIO::ERR_SLAVE_DEFINED if not from KIO) errorText the text of the error message initialUrl the URL that we were trying to open (as a string, so that this can support invalid URLs as well)
- Since
- 4.6
Definition at line 520 of file browserrun.cpp.
KParts::ReadOnlyPart * BrowserRun::part | ( | ) | const |
Definition at line 78 of file browserrun.cpp.
Definition at line 385 of file browserrun.cpp.
|
static |
If kget integration is enabled, passes the url to kget.
Otherwise, asks the user for a destination url, and calls saveUrlUsingKIO.
- Since
- 4.4
Definition at line 397 of file browserrun.cpp.
|
static |
Starts the KIO file copy job to download srcUrl
into destUrl
.
- Since
- 4.4
Definition at line 465 of file browserrun.cpp.
|
protectedvirtual |
bool BrowserRun::serverSuggestsSave | ( | ) | const |
- Returns
- Wheter the returned disposition suggests saving or opening inline
Definition at line 588 of file browserrun.cpp.
|
static |
Definition at line 391 of file browserrun.cpp.
Definition at line 214 of file browserrun.cpp.
|
protectedslot |
Definition at line 182 of file browserrun.cpp.
|
protectedslot |
Definition at line 560 of file browserrun.cpp.
|
protectedvirtualslot |
Definition at line 482 of file browserrun.cpp.
KUrl BrowserRun::url | ( | ) | const |
Definition at line 83 of file browserrun.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:25:36 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.