KParts
#include <part.h>
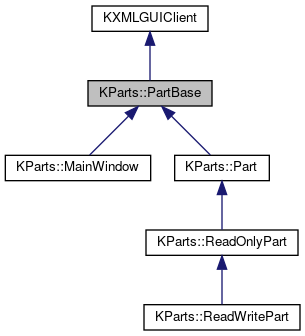
Protected Types | |
enum | PluginLoadingMode { DoNotLoadPlugins = 0, LoadPlugins = 1, LoadPluginsIfEnabled = 2 } |
Protected Member Functions | |
PartBase (PartBasePrivate &dd) | |
void | loadPlugins (QObject *parent, KXMLGUIClient *parentGUIClient, const KComponentData &componentData) |
virtual void | setComponentData (const KComponentData &componentData) |
virtual void | setComponentData (const KComponentData &componentData, bool loadPlugins) |
void | setPluginInterfaceVersion (int version) |
void | setPluginLoadingMode (PluginLoadingMode loadingMode) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
PartBasePrivate * | d_ptr |
Additional Inherited Members | |
![]() | |
enum | ReverseStateChange |
![]() | |
static QString | findMostRecentXMLFile (const QStringList &files, QString &doc) |
Detailed Description
Member Enumeration Documentation
|
protected |
We have three different policies, whether to load new plugins or not.
The value in the KConfig object of the KComponentData object always overrides LoadPlugins and LoadPluginsIfEnabled.
Constructor & Destructor Documentation
Member Function Documentation
|
protected |
Load the Plugins honoring the PluginLoadingMode.
If you call this method in an already constructed GUI (like when the user has changed which plugins are enabled) you need to add the new plugins to the KXMLGUIFactory:
|
protectedvirtual |
Set the componentData(KComponentData) for this part.
Call this first in the inherited class constructor, because it loads the i18n catalogs.
Reimplemented from KXMLGUIClient.
|
protectedvirtual |
Set the componentData(KComponentData) for this part.
Call this first in the inherited class constructor, because it loads the i18n catalogs.
It is recommended to call setComponentData with loadPlugins set to false, and to load plugins at the end of your part constructor (in the case of KParts::MainWindow, plugins are automatically loaded in createGUI anyway, so set loadPlugins to false for KParts::MainWindow as well).
void PartBase::setPartObject | ( | QObject * | object | ) |
Internal method.
Called by KParts::Part to specify the parent object for plugin objects.
|
protected |
If you change the binary interface offered by your part, you can avoid crashes from old plugins lying around by setting X-KDE-InterfaceVersion=2 in the .desktop files of the plugins, and calling setPluginInterfaceVersion( 2 ), so that the old plugins are not loaded.
Increase both numbers every time a binary incompatible change in the application's plugin interface is made.
- Parameters
-
version the interface version that plugins must have in order to be loaded.
For a KParts::Part: call this before setComponentData. For a KParts::MainWindow: call this before createGUI.
|
protected |
Set how plugins should be loaded.
- Parameters
-
loadingMode see PluginLoadingMode
For a KParts::Part: call this before setComponentData. For a KParts::MainWindow: call this before createGUI.
Member Data Documentation
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:25:36 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.