KParts
#include <plugin.h>
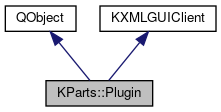
Classes | |
struct | PluginInfo |
Public Member Functions | |
Plugin (QObject *parent=0) | |
virtual | ~Plugin () |
virtual QString | localXMLFile () const |
virtual QString | xmlFile () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
KXMLGUIClient () | |
KXMLGUIClient (KXMLGUIClient *parent) | |
virtual | ~KXMLGUIClient () |
QAction * | action (const char *name) const |
virtual QAction * | action (const QDomElement &element) const |
virtual KActionCollection * | actionCollection () const |
void | addStateActionDisabled (const QString &state, const QString &action) |
void | addStateActionEnabled (const QString &state, const QString &action) |
void | beginXMLPlug (QWidget *) |
QList< KXMLGUIClient * > | childClients () |
KXMLGUIBuilder * | clientBuilder () const |
virtual KComponentData | componentData () const |
virtual QDomDocument | domDocument () const |
void | endXMLPlug () |
KXMLGUIFactory * | factory () const |
StateChange | getActionsToChangeForState (const QString &state) |
void | insertChildClient (KXMLGUIClient *child) |
KXMLGUIClient * | parentClient () const |
void | plugActionList (const QString &name, const QList< QAction * > &actionList) |
void | prepareXMLUnplug (QWidget *) |
void | reloadXML () |
void | removeChildClient (KXMLGUIClient *child) |
void | replaceXMLFile (const QString &xmlfile, const QString &localxmlfile, bool merge=false) |
void | setClientBuilder (KXMLGUIBuilder *builder) |
void | setFactory (KXMLGUIFactory *factory) |
void | setXMLGUIBuildDocument (const QDomDocument &doc) |
void | unplugActionList (const QString &name) |
QDomDocument | xmlguiBuildDocument () const |
Static Public Member Functions | |
static void | loadPlugins (QObject *parent, const KComponentData &instance) |
static void | loadPlugins (QObject *parent, const QList< PluginInfo > &pluginInfos) |
static void | loadPlugins (QObject *parent, const QList< PluginInfo > &pluginInfos, const KComponentData &instance) |
static void | loadPlugins (QObject *parent, KXMLGUIClient *parentGUIClient, const KComponentData &instance, bool enableNewPluginsByDefault=true, int interfaceVersionRequired=0) |
static QList< Plugin * > | pluginObjects (QObject *parent) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
static QString | findMostRecentXMLFile (const QStringList &files, QString &doc) |
Protected Member Functions | |
virtual void | setComponentData (const KComponentData &instance) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
virtual void | virtual_hook (int id, void *data) |
Static Protected Member Functions | |
static Plugin * | loadPlugin (QObject *parent, const char *libname) |
static Plugin * | loadPlugin (QObject *parent, const QByteArray &libname) |
static Plugin * | loadPlugin (QObject *parent, const QString &libname) |
static Plugin * | loadPlugin (QObject *parent, const QString &libname, const QString &keyword) |
static QList< Plugin::PluginInfo > | pluginInfos (const KComponentData &instance) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Additional Inherited Members | |
![]() | |
enum | ReverseStateChange |
![]() | |
objectName | |
Detailed Description
A plugin is the way to add actions to an existing KParts application, or to a Part.
The XML of those plugins looks exactly like of the shell or parts, with one small difference: The document tag should have an additional attribute, named "library", and contain the name of the library implementing the plugin.
If you want this plugin to be used by a part, you need to install the rc file under the directory "data" (KDEDIR/share/apps usually)+"/instancename/kpartplugins/" where instancename is the name of the part's instance.
You should also install a "plugin info" .desktop file with the same name.
- See also
- KPluginInfo
For a tutorial on how to write plugins, see http://developer.kde.org/documentation/tutorials/developing-a-plugin-structure/index.html#developing_plugins
Constructor & Destructor Documentation
Plugin::Plugin | ( | QObject * | parent = 0 | ) |
Construct a new KParts plugin.
Definition at line 48 of file plugin.cpp.
|
virtual |
Destructor.
Definition at line 54 of file plugin.cpp.
Member Function Documentation
- Returns
- The plugin created from the library
libname
Definition at line 172 of file plugin.cpp.
|
staticprotected |
, added only for source compatibility
- Returns
- The plugin created from the library
libname
Definition at line 184 of file plugin.cpp.
- Returns
- The plugin created from the library
libname
Definition at line 190 of file plugin.cpp.
|
staticprotected |
Definition at line 196 of file plugin.cpp.
|
static |
Load the plugin libraries from the directories appropriate to instance
and make the Plugin objects children of parent
.
It is recommended to use the last loadPlugins method instead, to support enabling and disabling of plugins.
Definition at line 136 of file plugin.cpp.
|
static |
Load the plugin libraries specified by the list docs
and make the Plugin objects children of parent
.
It is recommended to use the last loadPlugins method instead, to support enabling and disabling of plugins.
Definition at line 165 of file plugin.cpp.
|
static |
Load the plugin libraries specified by the list pluginInfos
, make the Plugin objects children of parent
, and use the given instance
.
It is recommended to use the last loadPlugins method instead, to support enabling and disabling of plugins.
Definition at line 141 of file plugin.cpp.
|
static |
Load the plugin libraries for the given instance
, make the Plugin objects children of parent
, and insert the plugin as a child GUI client of parentGUIClient
.
This method uses the KConfig object of the given instance, to find out which plugins are enabled and which are disabled. What happens by default (i.e. for new plugins that are not in that config file) is controlled by enableNewPluginsByDefault
. It can be overridden by the plugin if it sets the X-KDE-PluginInfo-EnabledByDefault key in the .desktop file (with the same name as the .rc file)
If a disabled plugin is already loaded it will be removed from the GUI factory and deleted.
If you change the binary interface offered by your part, you can avoid crashes from old plugins lying around by setting X-KDE-InterfaceVersion=2 in the .desktop files of the plugins, and passing 2 to interfaceVersionRequired
, so that the old plugins are not loaded. Increase both numbers every time a binary incompatible change in the application's plugin interface is made.
This method is automatically called by KParts::Part and by KParts::MainWindow.
If you call this method in an already constructed GUI (like when the user has changed which plugins are enabled) you need to add the new plugins to the KXMLGUIFactory:
Definition at line 255 of file plugin.cpp.
|
virtual |
Reimplemented for internal reasons.
Reimplemented from KXMLGUIClient.
Definition at line 71 of file plugin.cpp.
|
staticprotected |
Look for plugins in the instance's
"data" directory (+"/kpartplugins")
- Returns
- A list of QDomDocument s, containing the parsed xml documents returned by plugins.
Definition at line 84 of file plugin.cpp.
|
static |
Returns a list of plugin objects loaded for parent
.
This functions basically iterates over the children of the given object and returns those that inherit from KParts::Plugin.
Definition at line 212 of file plugin.cpp.
|
protectedvirtual |
Reimplemented from KXMLGUIClient.
Definition at line 249 of file plugin.cpp.
|
virtual |
Reimplemented for internal reasons.
Reimplemented from KXMLGUIClient.
Definition at line 59 of file plugin.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:25:36 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.