KBlog Client Library
#include <blog.h>
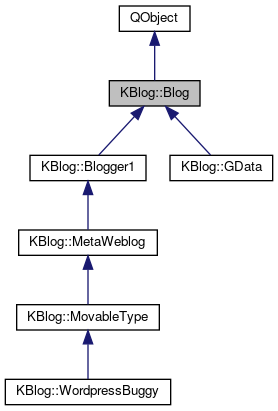
Public Types | |
enum | ErrorType { XmlRpc, Atom, ParsingError, AuthenticationError, NotSupported, Other } |
Signals | |
void | createdPost (KBlog::BlogPost *post) |
void | error (KBlog::Blog::ErrorType type, const QString &errorMessage) |
void | errorComment (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogPost *post, KBlog::BlogComment *comment) |
void | errorMedia (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogMedia *media) |
void | errorPost (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogPost *post) |
void | fetchedPost (KBlog::BlogPost *post) |
void | listedRecentPosts (const QList< KBlog::BlogPost > &posts) |
void | modifiedPost (KBlog::BlogPost *post) |
void | removedPost (KBlog::BlogPost *post) |
Public Member Functions | |
Blog (const KUrl &server, QObject *parent=0, const QString &applicationName=QString(), const QString &applicationVersion=QString()) | |
virtual | ~Blog () |
QString | blogId () const |
virtual void | createPost (KBlog::BlogPost *post)=0 |
virtual void | fetchPost (KBlog::BlogPost *post)=0 |
virtual QString | interfaceName () const =0 |
virtual void | listRecentPosts (int number)=0 |
virtual void | modifyPost (KBlog::BlogPost *post)=0 |
QString | password () const |
virtual void | removePost (KBlog::BlogPost *post)=0 |
virtual void | setBlogId (const QString &blogId) |
virtual void | setPassword (const QString &password) |
virtual void | setTimeZone (const KTimeZone &timeZone) |
virtual void | setUrl (const KUrl &url) |
void | setUserAgent (const QString &applicationName, const QString &applicationVersion) |
virtual void | setUsername (const QString &username) |
KTimeZone | timeZone () |
KUrl | url () const |
QString | userAgent () const |
QString | username () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
Blog (const KUrl &server, BlogPrivate &dd, QObject *parent=0, const QString &applicationName=QString(), const QString &applicationVersion=QString()) | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
BlogPrivate *const | d_ptr |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
A class that provides methods to call functions on a supported blog web application.
This is the main interface to the blogging client library.
Member Enumeration Documentation
Enumeration for possible errors.
Constructor & Destructor Documentation
|
explicit |
Constructor used by the remote interface implementations.
- Parameters
-
server URL for the blog's remote interface. parent the parent of this object, defaults to null. applicationName the client application's name to use in the HTTP user agent string, defaults to KBlog's own. applicationVersion the client application's version to use in the HTTP user agent string, defaults to KBlog's own.
|
protected |
Constructor needed to allow private inheritance of 'Private' classes.
- Parameters
-
server URL for the blog's XML-RPC interface. dd URL for the corresponding private class. parent the parent of this object, defaults to null. applicationName the client application's name to use in the HTTP user agent string, defaults to KBlog's own. applicationVersion the client application's version to use in the HTTP user agent string, defaults to KBlog's own.
Member Function Documentation
QString Blog::blogId | ( | ) | const |
Returns the unique ID for the specific blog on the server.
- See also
- setBlogId( const QString &blogId );
|
signal |
This signal is emitted when a createPost() job creates a new blog post on the blogging server.
- Parameters
-
post the created post.
- See also
- createPost()
|
pure virtual |
Create a new blog post on the server.
- Parameters
-
post the blog post to create.
- See also
- createdPost()
Implemented in KBlog::GData, KBlog::Blogger1, KBlog::MovableType, and KBlog::WordpressBuggy.
|
signal |
This signal is emitted when an error occurs with XML parsing or a structural problem.
- Parameters
-
type the type of the error. errorMessage the error message.
- See also
- ErrorType
|
signal |
This signal is emitted when an error occurs with XML parsing or a structural problem in an operation involving a blog post's comment.
- Parameters
-
type the type of the error. errorMessage the error message. post the post that caused the error. comment the comment that caused the error.
- See also
- ErrorType
|
signal |
This signal is emitted when an error occurs with XML parsing or a structural problem in an operation involving some blog media.
- Parameters
-
type the type of the error. errorMessage the error message. media the media that caused the error.
- See also
- ErrorType
|
signal |
This signal is emitted when an error occurs with XML parsing or a structural problem in an operation involving a blog post.
- Parameters
-
type the type of the error. errorMessage the error message. post the post that caused the error.
- See also
- ErrorType
|
signal |
This signal is emitted when a fetchPost() job fetches a post from the blogging server.
- Parameters
-
post the fetched post.
- See also
- fetchPost()
|
pure virtual |
Fetch a blog post from the server with a specific ID.
The ID of the existing post must be retrieved using getRecentPosts and then be modified and provided to this method or a new BlogPost created with the existing ID.
- Parameters
-
post a blog post with the ID identifying the blog post to fetch.
- See also
- fetchedPost()
- listedRecentPosts( int number )
Implemented in KBlog::GData, KBlog::Blogger1, and KBlog::MovableType.
|
pure virtual |
Returns the name of the blogging API this object implements.
Implemented in KBlog::GData, KBlog::WordpressBuggy, KBlog::Blogger1, KBlog::MetaWeblog, and KBlog::MovableType.
|
signal |
This signal is emitted when a listRecentPosts() job fetches a post from the blogging server.
- Parameters
-
posts the list of posts.
- See also
- listRecentPosts()
|
pure virtual |
List a number of recent posts from the server.
The posts are returned in descending chronological order.
- Parameters
-
number the number of posts to fetch.
Implemented in KBlog::GData, KBlog::Blogger1, and KBlog::MovableType.
|
signal |
This signal is emitted when a modifyPost() job modifies a post on the blogging server.
- Parameters
-
post the modified post.
- See also
- modifyPost()
|
pure virtual |
Modify an existing blog post on the server.
The ID of the existing post must be retrieved using getRecentPosts and then be modified and provided to this method or a new BlogPost created with the existing ID.
- Parameters
-
post the new blog post.
- See also
- modifiedPost()
- listedRecentPosts( int number )
Implemented in KBlog::GData, KBlog::Blogger1, KBlog::MovableType, and KBlog::WordpressBuggy.
QString Blog::password | ( | ) | const |
Returns the password of the blog.
- See also
- setPassword( const QString & );
|
signal |
This signal is emitted when a removePost() job removes a post from the blogging server.
- Parameters
-
post the removed post.
- See also
- removePost()
|
pure virtual |
Remove an existing blog post from the server.
The BlogPost object representing the existing post must be retrieved using getRecentPosts and then provided to this method.
- Parameters
-
post* the blog post to remove.
- See also
- removedPost()
- listedRecentPosts( int number )
Implemented in KBlog::GData, and KBlog::Blogger1.
|
virtual |
|
virtual |
Sets the password used in blog authentication.
- Parameters
-
password the blog's password.
- See also
- password();
|
virtual |
Sets the time zone of the blog's server.
- Parameters
-
timeZone the time zone of the server.
- See also
- timeZone()
|
virtual |
Sets the URL for the blog's XML-RPC interface.
- Parameters
-
url the blog's XML-RPC URL.
- See also
- url()
Reimplemented in KBlog::Blogger1.
Sets the HTTP user agent string used to make the HTTP requests.
- Parameters
-
applicationName the client application's name to use in the HTTP user agent string. applicationVersion the client application's version to use in the HTTP user agent string.
- See also
- userAgent()
|
virtual |
Sets the username used in blog authentication.
- Parameters
-
username the blog's username.
- See also
- username()
KTimeZone Blog::timeZone | ( | ) |
Get the time zone of the blog's server.
- See also
- void setTimeZone()
KUrl Blog::url | ( | ) | const |
Get the URL for the blog's XML-RPC interface.
- See also
- setUrl( const KUrl & )
QString Blog::userAgent | ( | ) | const |
QString Blog::username | ( | ) | const |
Returns the username used in blog authentication.
- See also
- setUsername( const QString & )
Member Data Documentation
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:36:50 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.