KBlog Client Library
#include <blogger1.h>
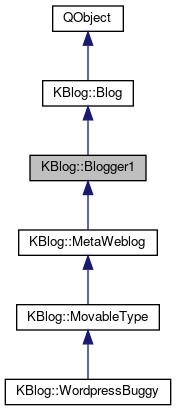
Signals | |
void | fetchedUserInfo (const QMap< QString, QString > &userInfo) |
void | listedBlogs (const QList< QMap< QString, QString > > &blogsList) |
![]() | |
void | createdPost (KBlog::BlogPost *post) |
void | error (KBlog::Blog::ErrorType type, const QString &errorMessage) |
void | errorComment (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogPost *post, KBlog::BlogComment *comment) |
void | errorMedia (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogMedia *media) |
void | errorPost (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogPost *post) |
void | fetchedPost (KBlog::BlogPost *post) |
void | listedRecentPosts (const QList< KBlog::BlogPost > &posts) |
void | modifiedPost (KBlog::BlogPost *post) |
void | removedPost (KBlog::BlogPost *post) |
Public Member Functions | |
Blogger1 (const KUrl &server, QObject *parent=0) | |
virtual | ~Blogger1 () |
void | createPost (KBlog::BlogPost *post) |
void | fetchPost (KBlog::BlogPost *post) |
virtual void | fetchUserInfo () |
QString | interfaceName () const |
virtual void | listBlogs () |
void | listRecentPosts (int number) |
void | modifyPost (KBlog::BlogPost *post) |
void | removePost (KBlog::BlogPost *post) |
void | setUrl (const KUrl &server) |
![]() | |
Blog (const KUrl &server, QObject *parent=0, const QString &applicationName=QString(), const QString &applicationVersion=QString()) | |
virtual | ~Blog () |
QString | blogId () const |
QString | password () const |
virtual void | setBlogId (const QString &blogId) |
virtual void | setPassword (const QString &password) |
virtual void | setTimeZone (const KTimeZone &timeZone) |
void | setUserAgent (const QString &applicationName, const QString &applicationVersion) |
virtual void | setUsername (const QString &username) |
KTimeZone | timeZone () |
KUrl | url () const |
QString | userAgent () const |
QString | username () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
Blogger1 (const KUrl &server, Blogger1Private &dd, QObject *parent=0) | |
![]() | |
Blog (const KUrl &server, BlogPrivate &dd, QObject *parent=0, const QString &applicationName=QString(), const QString &applicationVersion=QString()) | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | ErrorType { XmlRpc, Atom, ParsingError, AuthenticationError, NotSupported, Other } |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
BlogPrivate *const | d_ptr |
![]() | |
objectName | |
Detailed Description
A class that can be used for access to Blogger 1.0 blogs.
Almost every blog server supports Blogger 1.0. Compared to MetaWeblog it is not as functional and is obsolete on blogspot.com compared to GData which uses Atom instead of Xml-Rpc.
Definition at line 65 of file blogger1.h.
Constructor & Destructor Documentation
|
explicit |
Create an object for Blogger 1.0.
- Parameters
-
server is the url for the xmlrpc gateway. parent the parent object.
Definition at line 40 of file blogger1.cpp.
|
virtual |
Destroy the object.
Definition at line 54 of file blogger1.cpp.
|
protected |
Constructor needed for private inheritance.
Definition at line 47 of file blogger1.cpp.
Member Function Documentation
|
virtual |
Create a new post on server.
- Parameters
-
post is sent to the server.
Implements KBlog::Blog.
Reimplemented in KBlog::MovableType, and KBlog::WordpressBuggy.
Definition at line 148 of file blogger1.cpp.
This signal is emitted when a fetchUserInfo() job fetches the blog information from the blogging server.
- Parameters
-
userInfo The map with the keys: nickname, userid, url, email, lastname, firstname. Note: Not all keys are supported by all servers.
- See also
- fetchUserInfo()
|
virtual |
Fetch a post from the server.
- Parameters
-
post is the post. Note: Its id has to be set appropriately.
Implements KBlog::Blog.
Reimplemented in KBlog::MovableType.
Definition at line 108 of file blogger1.cpp.
|
virtual |
Get information about the user from the blog.
Note: This is not supported on the server side.
- See also
- void fetchedUserInfo( const QMap<QString,QString>& )
Definition at line 73 of file blogger1.cpp.
|
virtual |
Returns the of the inherited object.
Implements KBlog::Blog.
Reimplemented in KBlog::WordpressBuggy, KBlog::MetaWeblog, and KBlog::MovableType.
Definition at line 59 of file blogger1.cpp.
|
virtual |
List the blogs available for this authentication on the server.
- See also
- void listedBlogs( const QList<QMap<QString,QString> >& )
Definition at line 84 of file blogger1.cpp.
This signal is emitted when a listBlogs() job fetches the blog information from the blogging server.
- Parameters
-
blogsList The list of maps, in which each maps corresponds to a blog on the server. Each map has the keys id and name.
- See also
- listBlogs()
|
virtual |
List recent posts on the server.
The status of the posts will be Fetched.
- Parameters
-
number The number of posts to fetch. Latest first.
- See also
- void listedRecentPosts( QList<KBlog::BlogPost> & )
- void fetchPost( KBlog::BlogPost *post )
- BlogPost::Status
Implements KBlog::Blog.
Reimplemented in KBlog::MovableType.
Definition at line 95 of file blogger1.cpp.
|
virtual |
Modify a post on server.
- Parameters
-
post is used to send the modified post including the correct postId from it to the server.
- See also
- void modifiedPost( KBlog::BlogPost *post )
Implements KBlog::Blog.
Reimplemented in KBlog::MovableType, and KBlog::WordpressBuggy.
Definition at line 127 of file blogger1.cpp.
|
virtual |
Remove a post from the server.
- Parameters
-
post is the post. Note: Its id has to be set appropriately.
Implements KBlog::Blog.
Definition at line 169 of file blogger1.cpp.
|
virtual |
Set the Url of the server.
- Parameters
-
server is the server Url.
Reimplemented from KBlog::Blog.
Definition at line 64 of file blogger1.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:36:50 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.