KBlog Client Library
#include <metaweblog.h>
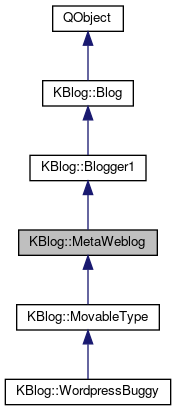
Signals | |
void | createdMedia (KBlog::BlogMedia *media) |
void | listedCategories (const QList< QMap< QString, QString > > &categories) |
![]() | |
void | fetchedUserInfo (const QMap< QString, QString > &userInfo) |
void | listedBlogs (const QList< QMap< QString, QString > > &blogsList) |
![]() | |
void | createdPost (KBlog::BlogPost *post) |
void | error (KBlog::Blog::ErrorType type, const QString &errorMessage) |
void | errorComment (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogPost *post, KBlog::BlogComment *comment) |
void | errorMedia (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogMedia *media) |
void | errorPost (KBlog::Blog::ErrorType type, const QString &errorMessage, KBlog::BlogPost *post) |
void | fetchedPost (KBlog::BlogPost *post) |
void | listedRecentPosts (const QList< KBlog::BlogPost > &posts) |
void | modifiedPost (KBlog::BlogPost *post) |
void | removedPost (KBlog::BlogPost *post) |
Public Member Functions | |
MetaWeblog (const KUrl &server, QObject *parent=0) | |
virtual | ~MetaWeblog () |
virtual void | createMedia (KBlog::BlogMedia *media) |
QString | interfaceName () const |
virtual void | listCategories () |
![]() | |
Blogger1 (const KUrl &server, QObject *parent=0) | |
virtual | ~Blogger1 () |
void | createPost (KBlog::BlogPost *post) |
void | fetchPost (KBlog::BlogPost *post) |
virtual void | fetchUserInfo () |
virtual void | listBlogs () |
void | listRecentPosts (int number) |
void | modifyPost (KBlog::BlogPost *post) |
void | removePost (KBlog::BlogPost *post) |
void | setUrl (const KUrl &server) |
![]() | |
Blog (const KUrl &server, QObject *parent=0, const QString &applicationName=QString(), const QString &applicationVersion=QString()) | |
virtual | ~Blog () |
QString | blogId () const |
QString | password () const |
virtual void | setBlogId (const QString &blogId) |
virtual void | setPassword (const QString &password) |
virtual void | setTimeZone (const KTimeZone &timeZone) |
void | setUserAgent (const QString &applicationName, const QString &applicationVersion) |
virtual void | setUsername (const QString &username) |
KTimeZone | timeZone () |
KUrl | url () const |
QString | userAgent () const |
QString | username () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
MetaWeblog (const KUrl &server, MetaWeblogPrivate &dd, QObject *parent=0) | |
![]() | |
Blogger1 (const KUrl &server, Blogger1Private &dd, QObject *parent=0) | |
![]() | |
Blog (const KUrl &server, BlogPrivate &dd, QObject *parent=0, const QString &applicationName=QString(), const QString &applicationVersion=QString()) | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | ErrorType { XmlRpc, Atom, ParsingError, AuthenticationError, NotSupported, Other } |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
BlogPrivate *const | d_ptr |
![]() | |
objectName | |
Detailed Description
A class that can be used for access to MetaWeblog blogs.
Almost every blog server supports MetaWeblog. Compared to Blogger 1.0 it is a superset of functions added to the its definition. MetaWeblog is much more functional, but has some drawbacks, e.g. security when compared to GData which is based on Atom API and is quite new.
Definition at line 65 of file metaweblog.h.
Constructor & Destructor Documentation
|
explicit |
Create an object for MetaWeblog.
- Parameters
-
server is the url for the xmlrpc gateway. parent is the parent object.
Definition at line 40 of file metaweblog.cpp.
|
virtual |
Destroy the object.
Definition at line 52 of file metaweblog.cpp.
|
protected |
Constructor needed for private inheritance.
Definition at line 46 of file metaweblog.cpp.
Member Function Documentation
|
signal |
This signal is emitted when a media has been created on the server.
- Parameters
-
media The created media.
|
virtual |
Create a new media object, e.g.
picture, on server.
- Parameters
-
media The media to send.
Definition at line 73 of file metaweblog.cpp.
|
virtual |
Returns the of the inherited object.
Reimplemented from KBlog::Blogger1.
Reimplemented in KBlog::WordpressBuggy, and KBlog::MovableType.
Definition at line 57 of file metaweblog.cpp.
|
virtual |
List the categories of the blog.
- See also
- listedCategories( const QList<QMap<QString,QString> >& )
Definition at line 62 of file metaweblog.cpp.
|
signal |
This signal is emitted when the last category of the listCategories() job has been fetched.
- Parameters
-
categories This list contains the categories. Each map has the keys: name, description, htmlUrl, rssUrl.
- See also
- listCategories()
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:36:50 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.