umbrello/umbrello
#include <rubywriter.h>
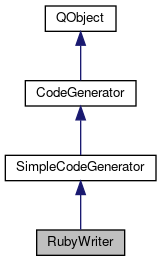
Public Member Functions | |
RubyWriter () | |
virtual | ~RubyWriter () |
virtual Uml::ProgrammingLanguage::Enum | language () const |
virtual QStringList | reservedKeywords () const |
virtual void | writeClass (UMLClassifier *c) |
![]() | |
SimpleCodeGenerator (bool createDirHierarchyForPackages=true) | |
virtual | ~SimpleCodeGenerator () |
CodeDocument * | newClassifierCodeDocument (UMLClassifier *classifier) |
void | writeCodeToFile (UMLClassifierList &concepts) |
void | writeCodeToFile () |
![]() | |
CodeGenerator () | |
virtual | ~CodeGenerator () |
bool | addCodeDocument (CodeDocument *add_object) |
void | connect_newcodegen_slots () |
virtual void | createDefaultStereotypes () |
virtual QStringList | defaultDatatypes () |
CodeDocument * | findCodeDocumentByClassifier (UMLClassifier *classifier) |
CodeDocument * | findCodeDocumentByID (const QString &id) |
QString | findFileName (CodeDocument *codeDocument) |
bool | forceDoc () const |
bool | forceSections () const |
CodeDocumentList * | getCodeDocumentList () |
virtual CodeViewerDialog * | getCodeViewerDialog (QWidget *parent, CodeDocument *doc, Settings::CodeViewerState state) |
virtual QString | getHeadingFile (const QString &file) |
QString | getUniqueID (CodeDocument *codeDoc) |
QString | headingFileDir () const |
bool | includeHeadings () const |
virtual bool | isReservedKeyword (const QString &keyword) |
virtual void | loadFromXMI (QDomElement &element) |
CodeGenerationPolicy::ModifyNamePolicy | modifyNamePolicy () const |
virtual CodeDocument * | newCodeDocument () |
bool | removeCodeDocument (CodeDocument *remove_object) |
virtual void | saveToXMI (QDomDocument &doc, QDomElement &root) |
void | setForceDoc (bool f) |
void | setForceSections (bool f) |
void | setHeadingFileDir (const QString &) |
void | setIncludeHeadings (bool i) |
void | setModifyNamePolicy (CodeGenerationPolicy::ModifyNamePolicy p) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
void | syncCodeToDocument () |
![]() | |
virtual void | checkAddUMLObject (UMLObject *obj) |
virtual void | checkRemoveUMLObject (UMLObject *obj) |
virtual void | syncCodeToDocument () |
![]() | |
void | codeGenerated (UMLClassifier *concept, bool generated) |
void | showGeneratedFile (const QString &filename) |
![]() | |
static QString | cleanName (const QString &name) |
static void | findObjectsRelated (UMLClassifier *c, UMLPackageList &cList) |
static QString | formatDoc (const QString &text, const QString &linePrefix=QLatin1String(" *"), int lineWidth=80) |
static QString | formatSourceCode (const QString &code, const QString &indentation) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QString | findFileName (UMLPackage *concept, const QString &ext) |
bool | hasAbstractOps (UMLClassifier *c) |
bool | hasDefaultValueAttr (UMLClassifier *c) |
QString | indent () |
void | initFromParentDocument () |
QString | overwritableName (UMLPackage *concept, const QString &name, const QString &ext) |
![]() | |
virtual void | finalizeRun () |
bool | openFile (QFile &file, const QString &name) |
QString | overwritableName (const QString &name, const QString &extension) |
void | writeListedCodeDocsToFile (CodeDocumentList *docs) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
QString | className_ |
QString | fileName_ |
bool | m_createDirHierarchyForPackages |
QString | m_endl |
QMap< UMLPackage *, QString > | m_fileMap |
QString | m_indentation |
int | m_indentLevel |
![]() | |
bool | m_applyToAllRemaining |
QHash< QString, CodeDocument * > | m_codeDocumentDictionary |
UMLDoc * | m_document |
![]() | |
objectName | |
Detailed Description
Class RubyWriter is a ruby code generator for UMLClassifier objects.
Just call writeClass and feed it a UMLClassifier.
Definition at line 26 of file rubywriter.h.
Constructor & Destructor Documentation
RubyWriter::RubyWriter | ( | ) |
Definition at line 30 of file rubywriter.cpp.
|
virtual |
Definition at line 34 of file rubywriter.cpp.
Member Function Documentation
|
virtual |
Returns "Ruby".
- Returns
- the programming language identifier
Implements CodeGenerator.
Definition at line 421 of file rubywriter.cpp.
|
virtual |
Get list of reserved keywords.
- Returns
- the list of reserved keywords
Reimplemented from CodeGenerator.
Definition at line 430 of file rubywriter.cpp.
|
virtual |
Call this method to generate C++ code for a UMLClassifier.
- Parameters
-
c the class you want to generate code for.
Implements SimpleCodeGenerator.
Definition at line 42 of file rubywriter.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:40:29 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.