umbrello/umbrello
#include <codegenerator.h>
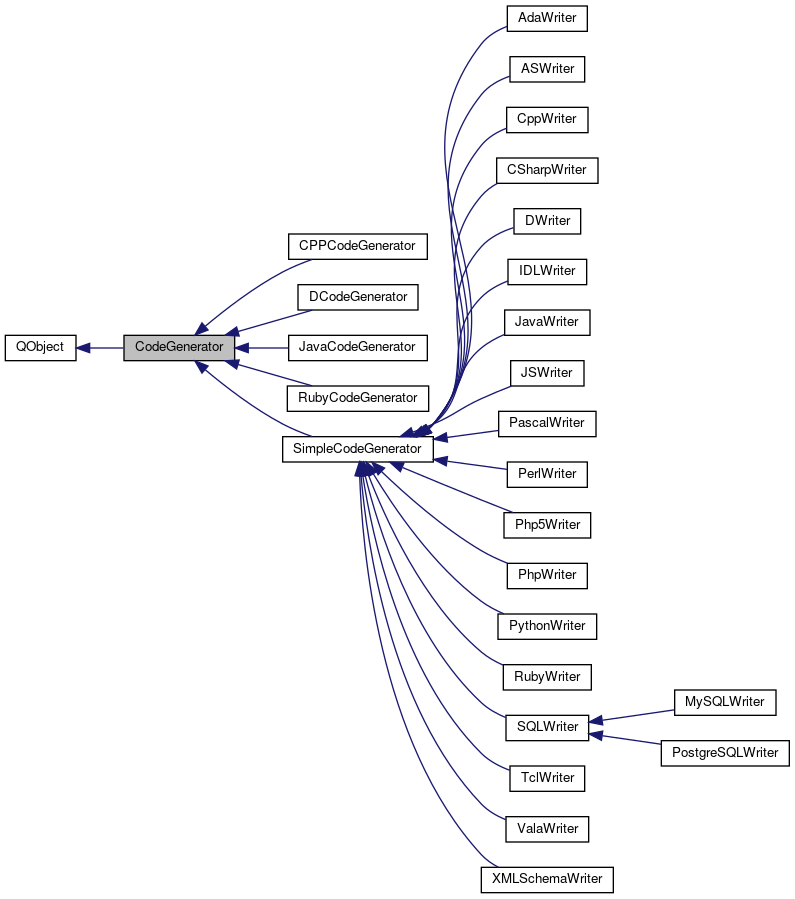
Public Slots | |
virtual void | checkAddUMLObject (UMLObject *obj) |
virtual void | checkRemoveUMLObject (UMLObject *obj) |
virtual void | syncCodeToDocument () |
Signals | |
void | codeGenerated (UMLClassifier *concept, bool generated) |
void | showGeneratedFile (const QString &filename) |
Static Public Member Functions | |
static QString | cleanName (const QString &name) |
static void | findObjectsRelated (UMLClassifier *c, UMLPackageList &cList) |
static QString | formatDoc (const QString &text, const QString &linePrefix=QLatin1String(" *"), int lineWidth=80) |
static QString | formatSourceCode (const QString &code, const QString &indentation) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
virtual void | finalizeRun () |
bool | openFile (QFile &file, const QString &name) |
QString | overwritableName (const QString &name, const QString &extension) |
void | writeListedCodeDocsToFile (CodeDocumentList *docs) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
bool | m_applyToAllRemaining |
QHash< QString, CodeDocument * > | m_codeDocumentDictionary |
UMLDoc * | m_document |
Additional Inherited Members | |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
This class collects together all of the code documents which form this project, and generates code for them in a given language.
CodeGenerator is the base class for all CodeGenerators. It provides the interface through which all Generators are invoked and the all the basic functionality. The only thing it doesn't do is to generate code =)
If you want to implement a CodeGenerator for some language follow these steps:
Create a class which inherits CodeGenerator. This class can have any name, I use names like CppCodeGenerator for the Cpp Generator, JavaCodeGenerator for the Java Generator and so on, but you can use what you want.
The code you generate should be output to "outputDirectory" and you should respect the OverwritePolicy specified. You should call findFileName(..) to get an appropriate file name, and then you can call openFile if you want, but if you want to do it yourself you
Finally put your generator in a library which can be dlopened together with a factory class (see below) and you are ready to go.
Note for "simple" code generators: Code can be entered into a QTextEdit widget in the ClassPropertiesDialog. This code is then stored in the respective UMLOperation, written to the xmi file, and also used when generating the source files. The code fragments are stored into the xmi file in the section "codegeneration" with the tag "sourcecode".
Definition at line 67 of file codegenerator.h.
Constructor & Destructor Documentation
CodeGenerator::CodeGenerator | ( | ) |
Constructor for a code generator.
Definition at line 54 of file codegenerator.cpp.
|
virtual |
Destructor.
Definition at line 69 of file codegenerator.cpp.
Member Function Documentation
bool CodeGenerator::addCodeDocument | ( | CodeDocument * | doc | ) |
Add a CodeDocument object to the m_codedocumentVector List.
- Returns
- boolean - will return false if it couldnt add a document
Definition at line 127 of file codegenerator.cpp.
|
virtualslot |
This function checks for adding objects to the UMLDocument.
Definition at line 343 of file codegenerator.cpp.
|
virtualslot |
This function checks for removing objects from the UMLDocument.
Definition at line 359 of file codegenerator.cpp.
Replaces spaces with underscores and capitalises as defined in m_modname.
- Returns
- QString
- Parameters
-
name
Definition at line 627 of file codegenerator.cpp.
|
signal |
void CodeGenerator::connect_newcodegen_slots | ( | ) |
Connect additional slots.
Only required for Advanced Code Generators. To be called after constructing the code generator (see CodeGenFactory).
Definition at line 839 of file codegenerator.cpp.
|
virtual |
Create the default stereotypes for your language (constructor, int etc).
Reimplemented in CPPCodeGenerator.
Definition at line 909 of file codegenerator.cpp.
|
virtual |
Return the default datatypes for your language (bool, int etc).
Default implementation returns empty list.
Reimplemented in CPPCodeGenerator, JavaCodeGenerator, DCodeGenerator, CppWriter, AdaWriter, DWriter, JavaWriter, PerlWriter, SQLWriter, ValaWriter, IDLWriter, PythonWriter, CSharpWriter, PascalWriter, MySQLWriter, and PostgreSQLWriter.
Definition at line 878 of file codegenerator.cpp.
|
protectedvirtual |
A single call to writeCodeToFile() usually entails processing many items (e.g.
as classifiers) for which code is generated. This method is called after all code of one call to writeCodeToFile() has been generated. It can be reimplemented by concrete code generators to perform additional cleanups or other actions that can only be performed once all code has been written.
Definition at line 465 of file codegenerator.cpp.
CodeDocument * CodeGenerator::findCodeDocumentByClassifier | ( | UMLClassifier * | classifier | ) |
Find a code document by the given classifier.
NOTE: FIX, this should be 'protected' or we could have problems with CPP code generator
- Returns
- CodeDocument
- Parameters
-
classifier
Definition at line 380 of file codegenerator.cpp.
CodeDocument * CodeGenerator::findCodeDocumentByID | ( | const QString & | tag | ) |
Find a code document by the given id string.
- Returns
- CodeDocument
Definition at line 112 of file codegenerator.cpp.
QString CodeGenerator::findFileName | ( | CodeDocument * | codeDocument | ) |
Finds an appropriate file name for the given CodeDocument, taking into account the Overwrite Policy and asking the user what to do if need be (if policy == Ask).
- Parameters
-
codeDocument the CodeDocument for which an output file name is desired.
- Returns
- the file name that should be used. (with extension) or NULL if none to be used
Definition at line 643 of file codegenerator.cpp.
|
static |
Finds all classes in the current document to which objects of class c are in some way related.
Possible relations are Associations (generalization, composition, etc) as well as parameters to methods and return values this is useful in deciding which classes/files to import/include in code generation
- Parameters
-
c the class for which relations are to be found cList a reference to the list into which return the result
Definition at line 700 of file codegenerator.cpp.
bool CodeGenerator::forceDoc | ( | ) | const |
Definition at line 859 of file codegenerator.cpp.
bool CodeGenerator::forceSections | ( | ) | const |
Definition at line 869 of file codegenerator.cpp.
|
static |
Format documentation for output in source files.
- Parameters
-
text the documentation which has to be formatted linePrefix the prefix which has to be added in the beginnig of each line lineWidth the line width used for word-wrapping the documentation
- Returns
- the formatted documentation text
Definition at line 790 of file codegenerator.cpp.
|
static |
Format source code for output in source files by adding the correct indentation to every line of code.
- Parameters
-
code the source code block which has to be formatted indentation the blanks to indent
Definition at line 821 of file codegenerator.cpp.
CodeDocumentList * CodeGenerator::getCodeDocumentList | ( | ) |
Get the list of CodeDocument objects held by m_codedocumentVector.
- Returns
- CodeDocumentList list of CodeDocument objects held by m_codedocumentVector
Definition at line 171 of file codegenerator.cpp.
|
virtual |
Get the editing dialog for this code document.
Reimplemented in CPPCodeGenerator, JavaCodeGenerator, RubyCodeGenerator, and DCodeGenerator.
Definition at line 181 of file codegenerator.cpp.
Gets the heading file (as a string) to be inserted at the beginning of the generated file.
you give the file type as parameter and get the string. if fileName starts with a period (.) then fileName is the extension (.cpp, .h, .java) if fileName starts with another character you are requesting a specific file (mylicensefile.txt). The files can have parameters which are denoted by parameter%.
current parameters are author% date% time% filepath%
- Returns
- QString
- Parameters
-
file
Definition at line 497 of file codegenerator.cpp.
QString CodeGenerator::getUniqueID | ( | CodeDocument * | codeDoc | ) |
Get a unique id for this codedocument.
- Returns
- id for the codedocument
Definition at line 80 of file codegenerator.cpp.
QString CodeGenerator::headingFileDir | ( | ) | const |
bool CodeGenerator::includeHeadings | ( | ) | const |
|
virtual |
Initialize this code generator from its parent UMLDoc.
When this is called, it will (re-)generate the list of code documents for this project (generator) by checking for new objects/attributes which have been added or changed in the document. One or more CodeDocuments will be created/overwritten/amended as is appropriate for the given language.
In this 'generic' version a ClassifierCodeDocument will exist for each and every classifier that exists in our UMLDoc. IF when this is called, a code document doesn't exist for the given classifier, then we will created and add a new code document to our generator.
IF you want to add non-classifier related code documents at this step, you will need to overload this method in the appropriate code generatator (see JavaCodeGenerator for an example of this).
Reimplemented in SimpleCodeGenerator, and CPPCodeGenerator.
Definition at line 308 of file codegenerator.cpp.
|
virtual |
Check whether the given string is a reserved word for the language of this code generator.
- Parameters
-
keyword string to check
Reimplemented in AdaWriter, and PascalWriter.
Definition at line 891 of file codegenerator.cpp.
|
pure virtual |
Return the unique language enum that identifies this type of code generator.
Implemented in JavaCodeGenerator, DCodeGenerator, RubyCodeGenerator, XMLSchemaWriter, CPPCodeGenerator, CppWriter, AdaWriter, DWriter, JavaWriter, TclWriter, SQLWriter, IDLWriter, PerlWriter, ValaWriter, PythonWriter, RubyWriter, ASWriter, JSWriter, PascalWriter, CSharpWriter, Php5Writer, MySQLWriter, PhpWriter, and PostgreSQLWriter.
|
virtual |
Load codegenerator data from xmi.
- Parameters
-
qElement the element from which to load
Definition at line 191 of file codegenerator.cpp.
CodeGenerationPolicy::ModifyNamePolicy CodeGenerator::modifyNamePolicy | ( | ) | const |
|
pure virtual |
A series of accessor method constructors that we need to define for any particular language.
Implemented in JavaCodeGenerator, CPPCodeGenerator, DCodeGenerator, RubyCodeGenerator, and SimpleCodeGenerator.
|
virtual |
Create a new Code document belonging to this package.
- Returns
- CodeDocument pointer to new code document.
Definition at line 473 of file codegenerator.cpp.
Opens a file named "name" for writing in the outputDirectory.
If something goes wrong, it informs the user if this function returns true, you know you can write to the file.
- Parameters
-
file file descriptor fileName the name of the file
- Returns
- success state
Definition at line 601 of file codegenerator.cpp.
|
protected |
Remove (and possibly delete) all AutoGenerated content type CodeDocuments but leave the UserGenerated (and any other type) documents in this generator alone.
Returns a name that can be written to in the output directory, respecting the overwrite policy.
If a file of the given name and extension does not exist, then just returns the name. If a file of the given name and extension does exist, then opens an overwrite dialog. In this case the name returned may be a modification of the input name. This method is invoked by findFileName().
- Parameters
-
name the proposed output file name extension the extension to use
- Returns
- the real file name that should be used (including extension) or QString() if none to be used
Definition at line 517 of file codegenerator.cpp.
bool CodeGenerator::removeCodeDocument | ( | CodeDocument * | remove_object | ) |
Replace (or possibly add a new) CodeDocument object to the m_codedocumentVector List.
Remove a CodeDocument object from m_codedocumentVector List.
As names must be unique and each code document must have a name.
- Returns
- boolean value which will be true if the passed document was able to replace some other document OR was added(no prior document existed..only when addIfPriorDocumentNotPresent is true). The document which was replaced will be deleted IF deleteReplacedDocument is true.
- boolean - will return false if it couldnt remove a document
Definition at line 152 of file codegenerator.cpp.
|
virtual |
Get list of reserved keywords.
Reimplemented in CPPCodeGenerator, JavaCodeGenerator, DCodeGenerator, RubyCodeGenerator, XMLSchemaWriter, AdaWriter, CppWriter, SQLWriter, IDLWriter, TclWriter, PascalWriter, PerlWriter, ValaWriter, PythonWriter, RubyWriter, ASWriter, JSWriter, CSharpWriter, Php5Writer, and PhpWriter.
Definition at line 900 of file codegenerator.cpp.
|
virtual |
Save the XMI representation of this object.
Reimplemented in CPPCodeGenerator.
Definition at line 258 of file codegenerator.cpp.
void CodeGenerator::setForceDoc | ( | bool | f | ) |
Definition at line 854 of file codegenerator.cpp.
void CodeGenerator::setForceSections | ( | bool | f | ) |
Definition at line 864 of file codegenerator.cpp.
void CodeGenerator::setHeadingFileDir | ( | const QString & | ) |
void CodeGenerator::setIncludeHeadings | ( | bool | i | ) |
void CodeGenerator::setModifyNamePolicy | ( | CodeGenerationPolicy::ModifyNamePolicy | p | ) |
|
signal |
|
virtualslot |
Force a synchronize of this code generator, and its present contents, to that of the parent UMLDocument.
"UserGenerated" code will be preserved, but Autogenerated contents will be updated/replaced or removed as is apppropriate.
Definition at line 328 of file codegenerator.cpp.
|
virtual |
This method is here to provide class wizard the ability to write out only those classes which are selected by the user.
Reimplemented in CPPCodeGenerator, and SimpleCodeGenerator.
Definition at line 390 of file codegenerator.cpp.
|
virtual |
This method is here to provide class wizard the ability to write out only those classes which are selected by the user.
Reimplemented in CPPCodeGenerator, and SimpleCodeGenerator.
Definition at line 401 of file codegenerator.cpp.
|
protected |
The actual internal routine which writes code documents.
Definition at line 421 of file codegenerator.cpp.
Member Data Documentation
|
protected |
Used by OverwriteDialog to know if the apply to all remaining files checkbox should be checked (is by default).
Definition at line 185 of file codegenerator.h.
|
protected |
Definition at line 179 of file codegenerator.h.
|
protected |
The document object.
Definition at line 190 of file codegenerator.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:40:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.